Learn Python: How To Read CSV Formatted Text
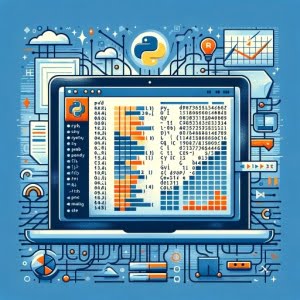
Are you grappling with reading CSV files in Python? Don’t worry. Python, akin to a skilled librarian, can open and process any CSV ‘book’ you hand it.
This comprehensive guide is designed to walk you through Python’s built-in capabilities to read CSV files, from the simplest to the most complex scenarios.
We’ll explore the power of Python’s built-in csv
module, delve into more advanced techniques, and even discuss alternative methods. So, let’s turn the page and start our journey in the world of CSV files with Python.
TL;DR: How Can I Read a CSV File in Python?
Python’s built-in
csv
module simplifies reading CSV files. Here’s a quick example:
import csv
with open('file.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
# Output:
# ['Column1', 'Column2', 'Column3']
# ['Data1', 'Data2', 'Data3']
# ['Data4', 'Data5', 'Data6']
This short script opens a file named ‘file.csv’, reads it line by line, and prints each row as a list. The output shows the column headers and data rows of the CSV file.
This is just the tip of the iceberg! Dive deeper into the rest of this guide for more detailed explanations, advanced usage scenarios, and alternative approaches to reading CSV files with Python.
Table of Contents
- Mastering the Basics: Reading CSV Files in Python
- Taking it Up a Notch: Advanced CSV Reading in Python
- Exploring Alternatives: Reading CSV with Pandas
- Troubleshooting Python CSV Reading Issues
- Understanding CSV Files and Python’s CSV Module
- CSV Reading: A Stepping Stone to Larger Python Projects
- Wrapping Up: Python and CSV Files
Mastering the Basics: Reading CSV Files in Python
Python’s built-in csv
module is your go-to tool for reading CSV files. Here, we’ll explore how to use it with a simple code example and explain how the code works. We’ll also discuss potential pitfalls and how to avoid them.
Python’s CSV Module: A Simple Code Example
Let’s start with a basic example. You have a CSV file named ‘file.csv’, and you want to read and print its content. Here’s how you can do it:
import csv
with open('file.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
# Output:
# ['Column1', 'Column2', 'Column3']
# ['Data1', 'Data2', 'Data3']
# ['Data4', 'Data5', 'Data6']
In this code, we first import the csv
module. We then open the CSV file using Python’s built-in open()
function with ‘r’ indicating that we want to read the file. The with
statement ensures the file is properly closed after it is no longer needed.
We then create a reader
object using the csv.reader()
function, which returns an iterable reader object. The for
loop iterates through this object, printing each row of the CSV file as a list.
Potential Pitfalls and How to Avoid Them
While the csv
module simplifies reading CSV files, there are a few potential pitfalls to be aware of. One common issue is handling CSV files with different delimiters. By default, the csv.reader()
function assumes the delimiter is a comma. If your CSV file uses a different delimiter, such as a semicolon, you’ll need to specify this when calling the csv.reader()
function. Here’s how you can do it:
import csv
with open('file.csv', 'r') as file:
reader = csv.reader(file, delimiter=';')
for row in reader:
print(row)
# Output:
# ['Column1;Column2;Column3']
# ['Data1;Data2;Data3']
# ['Data4;Data5;Data6']
In this modified code, we’ve added the delimiter
parameter to the csv.reader()
function. This tells Python to use a semicolon as the delimiter when reading the CSV file.
Taking it Up a Notch: Advanced CSV Reading in Python
Once you’ve mastered the basics, you’re ready to tackle more complex scenarios in Python CSV handling. In this section, we’ll discuss reading large CSV files, handling different delimiters, and dealing with special characters.
Handling Large CSV Files
Python’s csv
module can read any size of CSV file, but for large files, it’s more memory-efficient to read the file line by line. Here’s how you can do it:
import csv
with open('large_file.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
# Output:
# ['Column1', 'Column2', 'Column3']
# ['Data1', 'Data2', 'Data3']
# ['Data4', 'Data5', 'Data6']
# ...
This code works exactly like the basic example, but it doesn’t load the entire file into memory at once, making it more efficient for large files.
Dealing with Different Delimiters
As we’ve seen in the basic section, the csv.reader()
function can handle different delimiters. But what if your CSV file uses a more unusual delimiter, like a pipe (|) or a tab? No problem. You can specify any character as a delimiter in the csv.reader()
function. Here’s an example with a pipe delimiter:
import csv
with open('pipe_delimited_file.csv', 'r') as file:
reader = csv.reader(file, delimiter='|')
for row in reader:
print(row)
# Output:
# ['Column1|Column2|Column3']
# ['Data1|Data2|Data3']
# ['Data4|Data5|Data6']
Reading Files with Special Characters
Sometimes, CSV files contain special characters. Python’s csv
module can handle these files as well. You just need to specify the quotechar
parameter in the csv.reader()
function. By default, quotechar
is ‘”‘, but you can specify any character. Here’s an example:
import csv
with open('special_characters_file.csv', 'r') as file:
reader = csv.reader(file, quotechar='"')
for row in reader:
print(row)
# Output:
# ['Column1', 'Column2', 'Column3']
# ['Data1', '"Data2"', 'Data3']
# ['Data4', 'Data5', '"Data6"']
In this code, we’ve set quotechar
to ‘”‘. This tells Python to consider anything enclosed in double quotes as a single field, even if it contains commas.
Exploring Alternatives: Reading CSV with Pandas
Python’s csv
module is a powerful tool for reading CSV files, but it’s not the only option. For those who frequently work with data, the pandas
library offers a more robust and feature-rich alternative.
Reading CSV Files with Pandas
Pandas is a popular data manipulation library in Python. It provides a function called read_csv()
that makes reading CSV files a breeze. Here’s how you can use it:
import pandas as pd
data = pd.read_csv('file.csv')
print(data)
# Output:
# Column1 Column2 Column3
# 0 Data1 Data2 Data3
# 1 Data4 Data5 Data6
In this code, we first import the pandas
library as pd
. We then use the read_csv()
function to read the CSV file and store the data in a DataFrame, a two-dimensional labeled data structure in pandas. The print()
function then prints the DataFrame, showing the data in a tabular format.
Benefits and Drawbacks of Using Pandas
Using pandas to read CSV files has several benefits. It’s faster and more memory-efficient than the csv
module, especially for large files. It also provides powerful data manipulation and cleaning functions, making it a great choice for data analysis tasks.
However, pandas is a large library and can be overkill for simple tasks. It also has a steeper learning curve than the csv
module. Therefore, if you’re just starting out or if you’re working on a small project, sticking to the csv
module might be a better choice.
Decision-Making Considerations
When deciding whether to use the csv
module or pandas to read CSV files in Python, consider the size and complexity of your task. If you’re dealing with large files or complex data manipulation, pandas might be the way to go. On the other hand, if you’re just reading a simple CSV file, the csv
module is a simpler and more straightforward option.
Troubleshooting Python CSV Reading Issues
While Python simplifies the process of reading CSV files, you may still encounter some common issues. In this section, we’ll discuss these issues, such as dealing with missing values and handling errors, and provide solutions with code examples.
Handling Missing Values
Missing values in CSV files can cause problems when you’re trying to analyze data. Python’s csv
module doesn’t have built-in support for handling missing values, but you can manage them manually or use the pandas
library.
Here’s how you can handle missing values manually:
import csv
with open('file.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
row = ['N/A' if value == '' else value for value in row]
print(row)
# Output:
# ['Column1', 'Column2', 'Column3']
# ['Data1', 'N/A', 'Data3']
# ['Data4', 'Data5', 'Data6']
In this code, we replace any missing value (represented as an empty string ”) with ‘N/A’.
Alternatively, you can use pandas
to handle missing values. The read_csv()
function in pandas treats missing values as NaN by default, and you can easily fill them with any value using the fillna()
function:
import pandas as pd
data = pd.read_csv('file.csv')
data = data.fillna('N/A')
print(data)
# Output:
# Column1 Column2 Column3
# 0 Data1 N/A Data3
# 1 Data4 Data5 Data6
Handling Errors
Errors can occur when reading CSV files in Python for various reasons, such as a file not found error or a permission error. To handle these errors, you can use Python’s built-in error handling mechanism, the try-except block. Here’s an example:
import csv
try:
with open('file.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
except FileNotFoundError:
print('File not found. Please check the file name and try again.')
# Output:
# File not found. Please check the file name and try again.
In this code, we use a try
block to attempt to open and read the CSV file. If a FileNotFoundError
occurs, we catch it in the except
block and print a helpful error message.
Understanding CSV Files and Python’s CSV Module
Before diving further into reading CSV files with Python, it’s essential to understand what CSV files are and how Python’s csv
module handles them.
The Structure of CSV Files
CSV (Comma-Separated Values) files are a common format for storing tabular data. They are plain-text files where each line represents a data record. Each field (or column) in the record is separated by a comma, hence the name ‘comma-separated values’. Here’s an example of how data is structured in a CSV file:
# Contents of example.csv
'Name, Age, Occupation
John Doe, 35, Software Engineer'
# Output:
# Name, Age, Occupation
# John Doe, 35, Software Engineer
In this example, the CSV file contains three fields: Name, Age, and Occupation. Each field is separated by a comma. Note that the first line of a CSV file often contains the column headers.
Python’s CSV Module: The Workhorse for CSV Files
Python’s csv
module is specifically designed to handle CSV files. It provides functions to read and write CSV files, handling the parsing and formatting of CSV data behind the scenes. This means you can focus on working with the data itself, without worrying about the intricacies of the CSV format.
The csv
module includes the reader
function, which we’ve been using to read CSV files. This function returns an iterable object that you can loop through to access each row of the CSV file. Each row is returned as a list of strings, making it easy to work with the data.
By understanding the structure of CSV files and the role of Python’s csv
module, you’re well-equipped to handle CSV data in Python. Armed with this knowledge, let’s continue our journey into reading CSV files with Python.
CSV Reading: A Stepping Stone to Larger Python Projects
Understanding how to read CSV files in Python is a fundamental skill that opens the door to more advanced Python projects. CSV files are a common data format in many fields, including data analysis and machine learning. Therefore, being able to handle CSV files efficiently can significantly streamline your workflow in these areas.
Python CSV Reading in Data Analysis
In data analysis, you often deal with large datasets stored in CSV files. Python’s csv
module, and especially the pandas
library, are invaluable tools for importing and cleaning this data. For example, you can use the pandas
function read_csv()
to import a CSV file into a DataFrame, and then use pandas’ powerful data manipulation functions to analyze the data.
Python CSV Reading in Machine Learning
In machine learning, CSV files are often used to store training and testing data. Again, Python’s csv
module and the pandas
library can simplify the process of importing and preparing this data. For example, you can use the read_csv()
function to import the data, and then use pandas’ functions to normalize the data, handle missing values, and split the data into training and testing sets.
Further Reading
If you’re interested in further expanding your skills with data handling in Python, Click Here for JSON Insights and explore real-world examples of using JSON in Python programming.
For more information on CSV handling in Python, you may want to explore the following articles:
- Python CSV File Writing: Saving Data to CSV – Dive into the world of CSV file creation and data export in Python.
A Quick Intro: CSV Handling in Python explores Python’s built-in support for CSV file handling and manipulation.
Efficient Ways to Import CSV Data in Python – Discover the most time saving methods for importing CSV data into Python.
Python CSV Library Documentation – Access the official Python documentation for a comprehensive guide to the CSV module.
Reading and Writing CSV Files in Python – A step-by-step guide to reading and writing CSV files using Python.
By mastering the art of reading CSV files in Python, you’re not just learning a single skill. You’re laying a solid foundation that will support you in many different Python projects.
Wrapping Up: Python and CSV Files
In this guide, we’ve explored various aspects of reading CSV files in Python. We’ve started with the basics, using Python’s built-in csv
module, and gradually moved to more complex scenarios and alternative approaches.
We’ve seen how Python can act as your personal librarian, reading any CSV file you hand it. We’ve demonstrated how to use Python’s csv
module to read CSV files, handle different delimiters, and deal with special characters. We’ve also discussed potential pitfalls, such as handling missing values and errors, and provided solutions for these issues.
For more advanced use cases, we’ve explored how the pandas
library can provide a more robust and feature-rich alternative to the csv
module. We’ve shown how to use pandas
to read CSV files, handle missing values, and prepare data for data analysis or machine learning projects.
Here’s a quick comparison of the methods we’ve discussed:
Method | Use Case | Pros | Cons |
---|---|---|---|
csv module | Simple CSV reading | Easy to use, built into Python | Limited functionality for complex tasks |
pandas | Advanced CSV reading, data analysis | Powerful, efficient, handles missing values | Larger library, steeper learning curve |
Reading CSV files in Python is a fundamental skill that can be a stepping stone to larger Python projects. Whether you’re interested in data analysis, machine learning, or just need to handle CSV data in your projects, mastering Python CSV reading is a valuable asset.
Remember, the journey of learning doesn’t stop here. There’s always more to explore, such as writing to CSV files or handling Excel files in Python. Happy coding!