Python Write to CSV | Guide (With Examples)
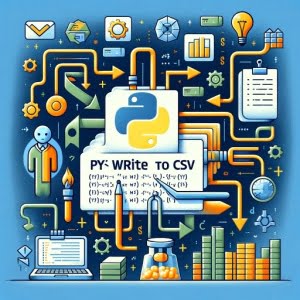
Are you finding it challenging to write data to CSV files in Python? You’re not alone. Many developers grapple with this task, but Python, like a skilled scribe, can easily record your data into CSV files.
Think of Python’s ability to write to CSV files as a bridge – a bridge that connects your Python code to a universal data format that can be opened by almost any data processing tool.
This guide will walk you through the process of writing data to CSV files in Python, from basic usage to advanced techniques. We’ll cover everything from using Python’s built-in csv module, handling different delimiters, quoting options, to alternative approaches and troubleshooting common issues.
Let’s dive in and start mastering writing to CSV files in Python!
TL;DR: How Do I Write Data to a CSV File in Python?
To write data to a CSV file in Python, you can use Python’s built-in
csv
module with thewriter()
andwriterow()
functions. Here’s a simple example:
import csv
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['name', 'age'])
writer.writerow(['John Doe', 30])
# Output:
# The CSV file named 'file.csv' will be created and two rows of data will be written to it.
In this example, we import the csv module and open a file named ‘file.csv’ in write mode. We then create a writer object and use it to write two rows of data to the file.
This is a basic way to write data to a CSV file in Python, but there’s much more to learn about handling CSV files in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Writing to CSV with Python’s Built-in CSV Module
- Advanced CSV Writing Techniques in Python
- Alternative Ways to Write CSV Files in Python
- Troubleshooting Common Issues with Python CSV Writing
- Unveiling CSV Files and Their Importance
- Python’s CSV Module Uncovered
- Going Beyond: Python and CSV Files in Larger Projects
- Wrapping Up: Master Python’s CSV Writing Capabilities
Writing to CSV with Python’s Built-in CSV Module
Python’s built-in csv module is a powerful tool for writing data to CSV files. It’s straightforward to use and perfect for beginners. Let’s break down the process into a step-by-step guide.
Step 1: Import the CSV Module
First, we need to import the csv module. The csv module comes pre-installed with Python, so you don’t need to install anything extra.
import csv
Step 2: Open a File in Write Mode
Next, we need to open the file we want to write to. We’ll use Python’s built-in open() function with the ‘w’ parameter, which stands for ‘write’.
with open('file.csv', 'w', newline='') as file:
pass
Step 3: Create a CSV Writer Object
Once the file is open, we create a csv writer object. This object has methods we can use to write data to the CSV file.
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file)
Step 4: Write Data to the CSV File
Finally, we use the writerow() method of the writer object to write data to the file. Each call to writerow() writes one row of data. The data should be in the form of a list.
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['name', 'age'])
writer.writerow(['John Doe', 30])
# Output:
# The CSV file named 'file.csv' will be created and two rows of data will be written to it.
In this example, we write two rows of data to the CSV file. The first row is a header row with the column names, and the second row contains some actual data.
And that’s it! You’ve just written data to a CSV file in Python. In the next section, we’ll delve into some more advanced usage scenarios.
Advanced CSV Writing Techniques in Python
Once you’re comfortable with the basics of writing to a CSV file in Python, you can start exploring some of the more advanced features of the csv module. These features give you greater control over how your data is written to the CSV file.
Custom Delimiters and Quoting Options
By default, the csv module uses a comma as the delimiter between fields and double quotes to enclose fields that contain special characters. However, you can customize these settings to suit your needs.
Let’s say you want to use a semicolon as the delimiter and single quotes to enclose fields. Here’s how you can do it:
import csv
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file, delimiter=';', quotechar=''', quoting=csv.QUOTE_MINIMAL)
writer.writerow(['name', 'age'])
writer.writerow(['John Doe', 30])
# Output:
# The CSV file named 'file.csv' will be created and two rows of data will be written to it. The fields are separated by semicolons and enclosed in single quotes.
In this example, we use the ‘delimiter’ and ‘quotechar’ parameters of the csv.writer() function to specify our custom delimiter and quote character. We also use the ‘quoting’ parameter to control when fields should be enclosed in quotes. The csv.QUOTE_MINIMAL option means that quotes are only used when necessary, i.e., when the field contains the delimiter, the quotechar, or a newline.
Writing Multiple Rows at Once
If you have a large amount of data to write to the CSV file, it can be more efficient to write multiple rows at once instead of one row at a time. You can do this with the writerows() method of the csv writer object.
Here’s an example:
import csv
data = [
['name', 'age'],
['John Doe', 30],
['Jane Doe', 28]
]
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
# Output:
# The CSV file named 'file.csv' will be created and three rows of data will be written to it.
In this example, we first create a list of lists, where each sub-list is a row of data. We then use the writerows() method to write all the rows to the CSV file at once.
These advanced techniques can make your Python CSV writing code more flexible and efficient. In the next section, we’ll explore some alternative approaches to writing CSV files in Python.
Alternative Ways to Write CSV Files in Python
While Python’s built-in csv module is a powerful tool for writing CSV files, there are alternative approaches that offer even more features and flexibility. One of the most popular alternatives is the pandas library.
Writing CSV Files with Pandas
Pandas is a powerful data analysis library that provides a high-level interface for manipulating structured data, including CSV files. Here’s how you can write data to a CSV file with pandas:
import pandas as pd
data = {
'name': ['John Doe', 'Jane Doe'],
'age': [30, 28]
}
df = pd.DataFrame(data)
df.to_csv('file.csv', index=False)
# Output:
# A CSV file named 'file.csv' will be created. The data from the DataFrame will be written to it.
In this example, we first create a dictionary where the keys are the column names and the values are lists of data. We then create a pandas DataFrame from the dictionary and use the to_csv() method of the DataFrame to write the data to a CSV file. The ‘index=False’ parameter is used to prevent pandas from writing row indices to the CSV file.
Comparing the csv Module and Pandas
While both the csv module and pandas can write data to CSV files, they have different strengths. The csv module is simpler and doesn’t have any dependencies, making it a good choice for small, straightforward tasks. On the other hand, pandas offers a wider range of features and is better suited for complex data manipulation tasks.
In conclusion, while Python’s built-in csv module is a reliable tool for writing CSV files, alternative approaches like pandas offer additional flexibility and features. The best approach depends on your specific needs and the complexity of your data.
Troubleshooting Common Issues with Python CSV Writing
Writing data to CSV files in Python is generally straightforward, but you might encounter some issues along the way. Let’s discuss some common problems and how to solve them.
Issue: Newlines Between Rows
If you’re seeing extra newlines between rows in your CSV file, it’s likely because you’re working on Windows, which uses a different newline character than Unix-based systems. To solve this issue, you can pass ‘newline=”’ to the open() function when opening the file:
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['name', 'age'])
writer.writerow(['John Doe', 30])
# Output:
# The CSV file named 'file.csv' will be created and two rows of data will be written to it, without extra newlines between rows.
Issue: Non-ASCII Characters
If your data contains non-ASCII characters, you might encounter an error when trying to write it to a CSV file. To handle this, you can open the file in utf-8 encoding:
with open('file.csv', 'w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(['name', 'age'])
writer.writerow(['Jöhn Döe', 30])
# Output:
# The CSV file named 'file.csv' will be created and two rows of data will be written to it. The non-ASCII characters in the data are handled correctly.
Issue: Data Contains Commas or Quotes
If your data contains commas or quotes, they can interfere with the CSV format. However, the csv module automatically handles these characters by enclosing the affected fields in quotes.
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['name', 'age'])
writer.writerow(['John, Doe', 30])
# Output:
# The CSV file named 'file.csv' will be created and two rows of data will be written to it. The comma in the data is handled correctly.
In this example, the comma in ‘John, Doe’ doesn’t interfere with the CSV format because the csv module automatically encloses it in quotes.
By being aware of these common issues and knowing how to solve them, you can ensure that your Python CSV writing code is robust and reliable.
Unveiling CSV Files and Their Importance
Comma-Separated Values (CSV) files are a type of file that stores tabular data. They are simple and compact, making them a popular choice for data exchange between programs and systems that support different formats. In a CSV file, each line represents a row of the table, and commas separate the fields (or columns) within each row.
# Example of CSV data:
'name', 'age'
'John Doe', 30
'Jane Doe', 28
# Output:
# This CSV data represents a table with two rows and two columns. The first row is a header row with the column names.
This simplicity and universality of CSV files make them a crucial tool in the world of data analysis and machine learning.
Python’s CSV Module Uncovered
Python’s csv module is a part of the standard library that provides functionality to read from and write to CSV files. It defines the writer
and reader
objects that encapsulate the logic for reading and writing CSV data.
The writer
object has two main methods:
writerow()
: Writes a single row to the CSV file.writerows()
: Writes multiple rows to the CSV file.
import csv
with open('file.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['name', 'age']) # Writing a single row
writer.writerows([['John Doe', 30], ['Jane Doe', 28]]) # Writing multiple rows
# Output:
# The CSV file named 'file.csv' will be created and three rows of data will be written to it.
Understanding the fundamentals of CSV files and Python’s csv module is the first step towards mastering the art of writing data to CSV files in Python. With this foundation, you can start to explore more advanced topics like custom delimiters, quoting options, and alternative libraries for handling CSV data.
Going Beyond: Python and CSV Files in Larger Projects
Writing data to CSV files in Python is not just an isolated task. It’s often a part of larger Python projects, especially those involving data analysis and machine learning.
Integrating CSV Writing in Data Analysis Projects
In data analysis projects, you might need to preprocess your data and save the cleaned data to a CSV file for further analysis. Or, you might need to save the results of your analysis to a CSV file for reporting or sharing with your team.
Exploring Related Topics
If you’re interested in expanding your Python CSV knowledge, there are several related topics you could explore. For example, you might want to learn about reading data from CSV files in Python. This is the reverse process of writing data to CSV files and is equally important in data analysis projects.
Another related topic is working with pandas, a powerful data analysis library in Python. As we’ve discussed earlier, pandas provides a high-level interface for manipulating CSV data and can be a good alternative to the csv module for complex tasks.
Further Resources for Mastering Python CSV Handling
If you’re interested in diving deeper into the world of Python and CSV files, here are some resources that might be helpful:
- IOFlood’s Python JSON Article dives into JSON schema validation and data integrity checks in Python.
Python JSON File Writing Guide details serializing Python objects into a JSON file.
Reading CSV Files in Python – A quick tutorial on reading and processing CSV files in Python.
Python’s official documentation on the csv module is a comprehensive resource that covers all the features of the csv module.
Real Python’s guide on working with CSV files provides practical examples and tips for working with CSV files in Python.
Pandas’ official documentation on the to_csv function covers the function in detail, which is useful for CSV data manipulation.
Wrapping Up: Master Python’s CSV Writing Capabilities
In this comprehensive guide, we’ve delved into the process of writing data to CSV files in Python. We’ve explored the built-in csv module, examined its methods, and learned how to use it to write data to CSV files efficiently.
We embarked with the basics, understanding how to use Python’s csv module to write data to a CSV file. We then stepped into advanced territory, discussing how to use the csv module to write data with different delimiters, quoting options, and even how to write multiple rows at once.
We also tackled common issues that you might encounter when writing data to a CSV file in Python, such as handling newlines between rows, non-ASCII characters, and data containing commas or quotes. We provided solutions to help you overcome these challenges.
we also discussed some alternatives to using the CSV module, as below:
Method | Flexibility | Ease of Use | Suitable For |
---|---|---|---|
csv module | Moderate | High | Small to medium-sized tasks |
pandas | High | Moderate | Complex data manipulation tasks |
Whether you’re a beginner just starting out with Python’s csv module or an experienced developer looking for advanced techniques, we hope this guide has equipped you with the knowledge and skills to write data to CSV files efficiently in Python.
With the power of Python’s csv module and the flexibility of pandas, you’re now well-prepared to tackle any CSV writing task that comes your way. Happy coding!