Python Dotenv Guide | Using Python Environment Variables
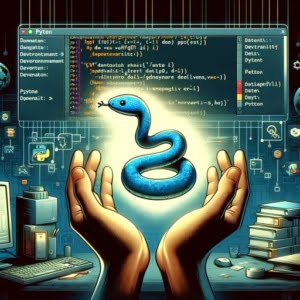
Are you wrestling with the task of managing sensitive data in your Python application? Do you feel like you’re treading on thin ice when it comes to handling confidential information? You’re not alone.
We have a powerful solution for you – the python-dotenv library. This library is a game-changer for securely managing environment variables in Python applications.
In this comprehensive guide, we will plunge into the world of python-dotenv, exploring its capabilities, peeling back the layers of its advanced features, and demonstrating how to use it to keep your sensitive data secure.
TL;DR: What is python-dotenv and how does it secure Python applications?
Python-dotenv is a library that enables secure management of environment variables in Python applications. It allows you to separate sensitive information from your application code, enhancing security. With python-dotenv, you can store sensitive data in a .env file in the user’s home directory, and access it when needed, keeping your code clean and maintainable. For a more advanced understanding and methods, continue reading the article.
Example usage for loading “SECRET KEY”:
from dotenv import load_dotenv
import os
load_dotenv()
secret_key = os.getenv('SECRET_KEY')
print(secret_key)
Table of Contents
Python-dotenv Basics
By keeping your secure data in your .env environment variable file, you can avoid accidentally including passwords, API keys, and other secrets in your program code. This is essential for making sure these secrets don’t accidentally get leaked on github, for example.
Python-dotenv allows you to separate sensitive information from your application code, providing an additional layer of security. This separation ensures that even if your code is compromised, your sensitive data remains protected.
In addition to enhanced security, python-dotenv also brings convenience to the table. The installation is straightforward, setting up the .env file is a breeze, and the separation of sensitive data makes your code cleaner and more maintainable.
Installing Python-dotenv
The first step in our journey is the installation of python-dotenv. It’s a straightforward process that only requires pip, Python’s package installer. Here’s how you do it:
pip install python-dotenv
With just one command, you’ve successfully installed python-dotenv. Simple, isn’t it?
Setting Up the .env File
After installing python-dotenv, the next step is to set up the .env file. This file will act as a safe where you store your sensitive information. Here’s how you can append a secret to a .env file:
echo SECRET_KEY=my_secret_key >> .env
In the command above, ‘SECRET_KEY’ is the name of the environment variable, and ‘my_secret_key’ is the value. You can replace these with your own variables and values.
By default, Python will load the .env file in
/home/username/.env
, and Python will have access to the .env file associated with the user running the Python script.
Example of using .env file in a Python application:
from dotenv import load_dotenv
import os
load_dotenv()
secret_key = os.getenv('SECRET_KEY')
print(secret_key)
The Advantages of Separation
By storing sensitive information in a separate .env file, you’re enhancing security and also making your code cleaner and more maintainable. You no longer have to hard-code sensitive data into your application code. Instead, you can store it in the .env file and access it when needed.
This separation also allows you to share your code without revealing your secrets.
Using the load_dotenv() Function
Having set up our .env file, the next step is to integrate it into our application. This is where the load_dotenv()
function comes into play. This function loads the variables from the .env file into the environment, making them accessible to our application.
For example, if your .env file looks like this:
DATABASE_URL=postgresql://localhost/db
SECRET_KEY=YourSecretKey
You can access these values like this:
import os
from dotenv import load_dotenv
# Load the dotenv file
load_dotenv()
# Access variables
database_url = os.getenv('DATABASE_URL', 'default_value_if_not_found')
secret_key = os.getenv('SECRET_KEY')
# Use these values in your application
print(f'Database URL: {database_url}')
print(f'Secret Key: {secret_key}')
In this script, ‘DATABASE_URL’ and ‘SECRET_KEY’ are strings that match the names of variables in your .env file.
If you think the .env file might have changed, you can refresh the variables inside of Python by running
load_dotenv()
again.
Loading All Environment Variables as a Dictionary
What if you want to load all the environment variables at once? Python-dotenv has a solution for that too. You can load all the variables from your .env file as a dictionary. Here’s how:
from dotenv import dotenv_values
# Get a dictionary of .env variables
config = dotenv_values()
# Get 'SECRET_KEY'
secret_key = config.get('SECRET_KEY')
# Now you can use the secret_key variable in your application
print(f'Secret Key: {secret_key}')
The dotenv_values()
function returns a dictionary containing all the environment variables from the specified .env file.
In the code snippet above, ‘SECRET_KEY’ is the name of the variable. You can replace it with the name of the variable you wish to refer to.
dotenv_values()
will load the .env file when you run it, making it unnecessary to runload_dotenv()
, and also takes the place of runningos.getenv
Loading Environment Variables via Streams
Python-dotenv goes a step further by supporting loading variables via streams. This means you can source your environment variables from non-filesystem entities, like network streams or in-memory files. This allows you to store your configurations in a database or a remote server and python-dotenv can still load them.
Example of loading variables via streams:
from dotenv import dotenv_values
from io import StringIO
stream = StringIO('SECRET_KEY=my_secret_key')
config = dotenv_values(stream=stream)
print(config)
Advanced Uses of dotenv()
Although the basic uses outlined above are already powerful, there are many ways to get more out of dotenv() with advanced use cases.
Loading Different Environment Variable Files
During the development of a Python application, it’s common to require different environment variables for different environments.
For instance, you might need to use a different database for development and production. Here’s how you can employ it:
import platform
if platform.node() == 'dev-machine':
load_dotenv('.env.dev')
else:
load_dotenv('.env.prod')
In the code snippet above, platform.node()
returns the network name of the machine. If the network name is ‘dev-machine’, the development environment variables are loaded. Otherwise, the production environment variables are loaded.
Example of loading different .env files based on the machine’s network name:
import platform
from dotenv import load_dotenv
if platform.node() == 'dev-machine':
load_dotenv('.env.dev')
else:
load_dotenv('.env.prod')
The capability to load different sets of variables based on the code environment can be a game-changer. It enables you to segregate your development and production environments, minimizing the risk of errors and enhancing the robustness of your application.
Excluding .env Files from Version Control
To further bolster security, it’s recommended to exclude your .env file from your version control system. This can be achieved by creating a .gitignore file. Here’s how you can do it:
echo .env >> .gitignore
The above command adds ‘.env’ to your .gitignore file, ensuring that your .env file is not tracked by Git.
Delving into Variable Expansions in Python-dotenv
Python-dotenv takes the management of environment variables a step further with the feature of variable expansions. This capability allows you to use the value of one environment variable within another. Here’s an illustrative example:
APP_PATH=/usr/app
LOG_PATH=${APP_PATH}/logs
In the example above, LOG_PATH
utilizes the value of APP_PATH
. This is referred to as variable expansion, and it’s an influential feature that can render your .env files more dynamic and flexible.
Example of variable expansion:
from dotenv import load_dotenv
import os
load_dotenv()
app_path = os.getenv('APP_PATH')
log_path = os.getenv('LOG_PATH')
print(app_path) # Output: /usr/app
print(log_path) # Output: /usr/app/logs
Python-dotenv extends support for POSIX variable expansion, paving the way for dynamic configuration. This means you can use special characters like
$
and:
in your environment variables, offering even more flexibility.
Integrating Python-dotenv in Django Projects
Python-dotenv isn’t limited to just standalone Python applications – it can also be integrated into Django projects. By adding load_dotenv()
to your Django settings.py file, you can load your environment variables directly into your Django project.
Example of integrating python-dotenv into a Django project:
# settings.py
from dotenv import load_dotenv
import os
load_dotenv()
SECRET_KEY = os.getenv('SECRET_KEY')
Python-dotenv’s Command-Line Interface
Python-dotenv also provides a command-line interface, enabling you to interact with your .env files directly from the command line. This can be a potent tool for managing your environment variables, particularly in development environments.
Here’s an example of how you can use it:
# Commands within your terminal
# Load the .env file
dotenv -f /path/to/your/.env list
# Set a new variable
dotenv -f /path/to/your/.env set NEW_VARIABLE "New Value"
# Get a variable value
dotenv -f /path/to/your/.env get VARIABLE_NAME
# Unset (delete) a variable
dotenv -f /path/to/your/.env unset VARIABLE_NAME
You can replace /path/to/your/.env
with the path to your .env file, VARIABLE_NAME
with the name of the variable you want to print or delete, and NEW_VARIABLE
and "New Value"
with the name and value of the variable you want to set.
If you have installed Python-dotenv and it’s available in your system’s PATH, then you should be able to utilize it as a command-line tool just like any other shell command.
However, on some systems, depending on how your Python environment is set up, you might need to use it via the python -m switch from the command line, like so:
# Load the .env file
python -m dotenv list
# Set a new variable
python -m dotenv set NEW_VARIABLE "New Value"
# Get a variable value
python -m dotenv get VARIABLE_NAME
# Unset (delete) a variable
python -m dotenv unset VARIABLE_NAME
This will ensure that you’re using the dotenv
module that’s associated with the particular Python interpreter you’re invoking.
Python-dotenv Summary: Flexibility, Security, and Convenience
As we’ve seen in this article, python-dotenv offers a high degree of flexibility when it comes to handling environment variables. Whether you want to load variables individually, load them all at once, or load them from diverse sources, python-dotenv has got you covered.
But python-dotenv is not just about flexibility – it’s also about security and convenience.
Feature | Description |
---|---|
Flexibility | Load variables individually, load them all at once, or load them from diverse sources |
Security | Separate sensitive data from application code and load it only when needed |
Convenience | Simple and intuitive API for managing environment variables |
By allowing you to separate your sensitive data from your application code and load it only when needed, python-dotenv enhances the security of your application. Its simple and intuitive API makes managing your environment variables a breeze.
Further Resources for Environment Variables
For more info on Environment Variables, Click Here for info on Python CLI best practices for clean interfaces, which can help further your usage of Environment Variables.
To further your understanding of Environment Variables we have sourced some resources that will assist you in deepening your knowledge:
- Function Definitions with args in Python – Learn how args simplifies passing multiple arguments to functions.
Function Parameter Handling with kwargs in Python – Learn how **kwargs simplifies passing keyword arguments.
Managing Environment Variables with Python-dotenv – Understand the concepts of managing environment variables using python-dotenv.
Python-dotenv on PyPi – Learn about python-dotenv, a module that allows you to specify environment variables.
Pieran Training’s Tutorial on Environment Variables is a guide on how to effectively use environment variables in Python.
Final Thoughts
In this comprehensive guide, we’ve journeyed through the world of python-dotenv, an influential library for managing environment variables in Python applications. We’ve highlighted how python-dotenv assists you in safeguarding your sensitive data, keeping it separate from your application code, and loading it only when necessary, thereby enhancing the security and integrity of your applications.
We’ve also ventured into the advanced features of python-dotenv, from variable expansions and dynamic configurations to its command-line interface and integration with Django projects. These features underscore python-dotenv as a versatile and flexible tool, capable of handling an extensive range of use cases.
To master Python’s subtleties, click here for a treasure trove of tips and tricks.
Whether you’re a novice just embarking on your Python development journey or a seasoned developer managing a large-scale project, python-dotenv is a tool you’ll want to have in your repertoire. So, why hold back? Start using python-dotenv today and experience the difference for yourself!