npm-run-all Usage | Run Multiple npm Scripts in Parallel
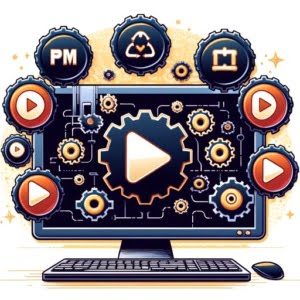
Struggling to manage multiple npm scripts concurrently? You’re not alone. Many developers face the challenge of handling various build processes, tests, and other scripts in a Node.js environment. To aid with this, today’s article will discuss how to run npm scripts in parallel.
When developing software for IOFLOOD’s dedicated servers, consistently dependable npm script execution is essential for smooth deployments. To streamline this process, we’ve utilized the npm-run-all
package as a solution. To help other developers facing similar obstacles, we’ve put together our tips and tricks into this comprehensive tutorial.
This guide will walk you through the basics to advanced usage of npm-run-all, making your development process smoother and more efficient. Whether you’re a beginner looking to understand the fundamentals or an experienced developer seeking to optimize your workflow, this guide has something for everyone.
Let’s dive in and explore how npm-run-all can transform your script execution strategy, making it more efficient and manageable.
TL;DR: How Do I Use npm-run-all to Run Multiple NPM Scripts?
npm-run-all is a CLI tool that lets you run multiple npm scripts in parallel or sequentially. To run
script1
andscript2
concurrently, you would use the following command:
npm-run-all --parallel script1 script2
# Output:
# 'script1' and 'script2' run concurrently.
This command simplifies the process of managing multiple scripts, making your development workflow more efficient. By allowing scripts to be run in parallel, npm-run-all reduces the total execution time, streamlining your project’s build process.
Curious to learn more? Keep reading to discover the full capabilities of npm-run-all, including advanced configurations and tips for optimizing your script execution strategy.
Table of Contents
Getting Started with npm-run-all
Your First Steps Towards Efficient Script Management
Embarking on your journey with npm-run-all starts with its installation. It’s a straightforward process that integrates seamlessly into your existing Node.js and npm environment. Let’s begin by installing npm-run-all globally, allowing you to use it across all your projects.
npm install -g npm-run-all
Once installed, you’re ready to simplify your script execution. Imagine you have two scripts in your package.json
: build
which compiles your project, and test
which runs your test suite. Traditionally, you might run these scripts one after another, but npm-run-all changes the game.
To run these scripts sequentially, ensuring build
completes before test
starts, you can use:
npm-run-all build test
# Output:
# 'build' script executes first followed by 'test'.
This command executes the build
script and, upon completion, starts the test
script. It’s a simple yet powerful way to manage script execution in a sequence, ensuring that your project is built and tested in the correct order.
Running Scripts in Parallel
What if you want to run scripts concurrently? npm-run-all makes this equally straightforward. If you have a lint
script to check your code quality and a watch
script to monitor file changes, running them in parallel can boost your productivity.
npm-run-all --parallel lint watch
# Output:
# 'lint' and 'watch' scripts run concurrently.
This command runs the lint
and watch
scripts at the same time, allowing you to simultaneously check code quality and monitor for changes. It’s an excellent example of how npm-run-all simplifies script management while catering to the needs of modern development workflows.
Pros: npm-run-all drastically simplifies the management of npm scripts, making it easier to run multiple scripts either sequentially or in parallel. This flexibility is a significant advantage in streamlining development processes.
Cons: For those new to npm or Node.js, there’s an initial learning curve in understanding script execution and how npm-run-all fits into your workflow. However, the benefits of mastering this tool far outweigh the initial effort required to learn it.
Advanced npm-run-all Techniques
Elevate Your Scripting Game
As you grow more comfortable with npm-run-all, it’s time to leverage its advanced features for even greater efficiency and flexibility. One powerful feature is the use of glob patterns to select and run scripts. This allows for a more dynamic script execution strategy, especially in larger projects with numerous scripts.
Imagine you have a series of scripts named test:unit
, test:integration
, and test:e2e
. Running all these tests individually can be tedious. Instead, you can use a glob pattern with npm-run-all to run them all in one go.
npm-run-all "test:*"
# Output:
# Executes 'test:unit', 'test:integration', and 'test:e2e' sequentially.
This command uses a glob pattern "test:*"
to match and run all scripts starting with test:
. It’s an elegant solution to run related scripts without manually listing each one, showcasing the tool’s flexibility.
Controlling Script Execution Order
Another advanced feature is controlling the order of script execution. While npm-run-all allows for parallel and sequential runs, sometimes you need more granular control, especially when scripts have dependencies on each other.
Consider a scenario where you need to clean your build directory before rebuilding your project. You have clean
and build
scripts in your package.json
. You can ensure clean
runs before build
by using the following command:
npm-run-all clean build
# Output:
# 'clean' script runs first, followed by 'build'.
This ensures that the clean
operation completes before the build
process begins, maintaining the necessary order for your scripts.
Integrating with Other Tools
npm-run-all’s versatility extends to its integration capabilities with other tools. This can significantly enhance your development workflow, allowing npm-run-all to act as a central hub for script execution.
For example, integrating npm-run-all with a tool like webpack
for bundling and jest
for testing can streamline your development process. Here’s an example package.json
file:
"scripts": {
"build": "webpack --config webpack.config.js",
"test": "jest",
"start": "node server.js",
"dev": "npm-run-all --parallel build test",
"prod": "npm-run-all --sequential build start"
}
In this example:
build
runs webpack to bundle the project.test
runs jest for testing.dev
runs bothbuild
andtest
concurrently usingnpm-run-all --parallel
.prod
runsbuild
first and then starts the server usingstart
sequentially usingnpm-run-all --sequential
.
Other tools that can integrate with npm-run-all
include:
- Gulp: npm-run-all can be used to run multiple gulp tasks sequentially or concurrently.
- ESLint: npm-run-all can execute linting tasks before running the main build task.
Here’s an example of how to integrate gulp
, and npm-run-all
within a package.json
file:
"scripts": {
"lint": "gulp lint",
"build": "gulp build",
"watch": "gulp watch",
"dev": "npm-run-all --parallel lint watch",
"prod": "npm-run-all --sequential lint build"
}
In this example:
lint
runs the linting task using Gulp.build
runs the build task using Gulp.watch
runs the watch task using Gulp.dev
runs both thelint
andwatch
tasks concurrently usingnpm-run-all --parallel
.prod
runs thelint
task first and then thebuild
task sequentially usingnpm-run-all --sequential
.
Here’s an example of how to integrate eslint
, and npm-run-all
within a package.json
file:
"scripts": {
"lint": "eslint .",
"build": "webpack",
"prebuild": "npm run lint",
"dev": "npm-run-all build",
"prod": "npm-run-all build"
}
In this example:
lint
runs the linting task using ESLint.build
runs the main build task using webpack.prebuild
is a special script that npm-run-all recognizes as a pre-hook. It runs thelint
task before thebuild
task.dev
andprod
are examples of tasks that run thebuild
task, withdev
typically used for development environments and prod for production.
Pros: The advanced features of npm-run-all, such as using glob patterns and controlling the order of execution, offer increased flexibility and efficiency. This makes it an invaluable tool for developers looking to optimize their script management.
Cons: With advanced features comes a complexity in configuration. It may take time to fully understand and utilize these features effectively. However, the investment in learning these advanced techniques pays off in the form of a more streamlined and efficient development process.
Exploring Alternatives to npm-run-all
Expand Your Scripting Toolkit
While npm-run-all is a powerful tool for managing npm scripts, it’s not the only player in the game. The Node.js ecosystem is rich with alternatives that offer unique features and benefits. Let’s dive into some of these alternatives, understand their advantages, and see how they compare to npm-run-all.
Concurrently: Run Commands Simultaneously
One popular alternative is concurrently
. It allows you to run multiple commands concurrently, similar to npm-run-all’s parallel feature. Here’s how you can use concurrently
to run two scripts at the same time:
concurrently "npm:script1" "npm:script2"
# Output:
# Both 'script1' and 'script2' run concurrently.
This command demonstrates concurrently
‘s ability to handle multiple scripts simultaneously. Its syntax differs slightly from npm-run-all, offering a different approach to script execution. The key advantage here is the flexibility in defining and running commands, making it a strong contender for managing complex script scenarios.
Cross-env: Environment Variable Management
Another tool worth mentioning is cross-env
, which simplifies the management of environment variables across different platforms. While not a direct alternative to running scripts like npm-run-all, it complements any script execution strategy by ensuring consistency in environment variables.
cross-env NODE_ENV=production npm run build
# Output:
# Sets NODE_ENV to 'production' and runs 'build' script.
This command sets the NODE_ENV
variable to production
before running the build
script, showcasing cross-env
‘s utility in creating a consistent scripting environment, especially when working across different development environments.
Custom Node.js Scripts: Ultimate Flexibility
For those seeking ultimate control and flexibility, writing custom Node.js scripts to manage npm tasks is an option. This approach allows for intricate script logic that goes beyond what npm-run-all and its alternatives offer.
const { exec } = require('child_process');
exec('npm run build && npm run test', (error, stdout, stderr) => {
if (error) {
console.error(`exec error: ${error}`);
return;
}
console.log(`stdout: ${stdout}`);
console.error(`stderr: ${stderr}`);
});
# Output:
# Executes 'build' then 'test', showing command outputs.
This example demonstrates a custom script that runs two npm scripts sequentially. By using Node.js’s child_process
module, you gain the flexibility to implement complex execution logic tailored to your project’s needs.
Pros and Cons: Each of these alternatives to npm-run-all has its unique advantages. Concurrently
offers a different syntax for running scripts simultaneously, cross-env
ensures environmental consistency, and custom Node.js scripts offer unparalleled flexibility. However, these tools also introduce additional complexity and learning curves, which might not suit all projects or developers.
Choosing the right tool for managing npm scripts depends on your specific project requirements and workflow preferences. Whether it’s the simplicity and efficiency of npm-run-all, the concurrency of concurrently
, the environment management of cross-env
, or the customizability of Node.js scripts, the Node.js ecosystem provides a wealth of options to streamline your development process.
Troubleshooting npm-run-all
Overcome Common Pitfalls
Using npm-run-all can significantly streamline your development process, but like any tool, it comes with its own set of challenges and considerations. Let’s explore some common issues and their solutions to ensure your npm scripts run smoothly.
Script Execution Order
One common pitfall is scripts executing in an unintended order, especially when running scripts in parallel. Although npm-run-all allows parallel execution, it’s crucial to understand which scripts can run concurrently without causing issues.
Consider you have a clean
script that clears your build directory and a build
script that compiles your source code. Running these in parallel could lead to the build
script executing before clean
has finished, resulting in unexpected behavior.
npm-run-all --parallel clean build
# Output:
# 'clean' and 'build' may run in an overlapping manner, leading to potential build issues.
To avoid this, ensure that scripts that must run in a specific sequence are executed using the sequential option or by carefully structuring your npm scripts to manage dependencies.
Handling Environment Variables
Another challenge is the proper handling of environment variables, particularly when moving between different development environments. npm-run-all itself does not directly manage environment variables, which can lead to inconsistencies if not handled correctly.
To ensure consistent environment variables across scripts, consider using cross-env
in conjunction with npm-run-all. This approach allows you to set and use environment variables reliably across platforms.
cross-env NODE_ENV=production npm-run-all build
# Output:
# Sets NODE_ENV to 'production' and runs 'build' script with the environment variable applied.
This command demonstrates setting the NODE_ENV
variable to production
before running the build
script, ensuring that your script runs with the correct environment settings.
Debugging Scripts
Debugging scripts run with npm-run-all can sometimes be more complex than running them individually. If a script fails, it might not be immediately clear which script caused the issue or why.
To facilitate debugging, use the --continue-on-error
option to prevent npm-run-all from stopping all scripts when one fails. This allows you to see the output of all scripts, making it easier to identify the source of the problem.
npm-run-all --continue-on-error script1 script2
# Output:
# Runs 'script1' and 'script2', continuing even if one script fails. This helps in isolating the failing script.
This command ensures that even if script1
or script2
encounters an error, the execution of other scripts continues, allowing for easier isolation and troubleshooting of the failing script.
Best Practices: To optimize your use of npm-run-all, familiarize yourself with its options and capabilities, structure your scripts with dependencies in mind, and incorporate tools like cross-env
for environment variable management. By anticipating and addressing these common pitfalls, you can harness the full power of npm-run-all to streamline your development workflow.
The Heart of Node.js Development
Why Script Management Matters
In the bustling world of Node.js development, managing scripts is akin to conducting an orchestra. Each script, whether it’s for building, testing, or deploying, plays a crucial role in the symphony of development tasks. But as projects grow in complexity, so does the challenge of managing these scripts efficiently. This is where tools like npm-run-all come into play, acting as the conductor to harmonize these tasks into a cohesive workflow.
Node.js, with its event-driven architecture, is designed for scalability and efficiency. npm, Node’s package manager, further extends this efficiency through scripts that automate repetitive tasks. However, as the number of scripts increases, developers often find themselves juggling multiple commands, leading to potential errors and inefficiencies.
npm-run-all: Simplifying Complexity
Enter npm-run-all, a command-line tool designed to run multiple npm scripts in parallel or sequentially. Its significance in the Node.js ecosystem cannot be overstated. By simplifying script execution, npm-run-all enhances development workflows, making them more predictable and efficient.
Consider a common scenario where you need to run a linting script followed by a series of test scripts. Instead of manually executing each script, npm-run-all streamlines the process:
npm-run-all lint "test:*"
# Output:
# 'lint' script runs first, followed by all scripts prefixed with 'test:' in sequence.
This command demonstrates the tool’s ability to manage script execution order, ensuring that code quality checks are completed before any tests are run. The use of a glob pattern ("test:*"
) further illustrates npm-run-all’s flexibility in handling multiple scripts with a single command, a feature that becomes invaluable in larger projects.
Impact on Development Workflows
The integration of npm-run-all into Node.js development workflows brings several benefits. It reduces the likelihood of human error in script execution, saves time by automating repetitive tasks, and ensures consistency across development environments. Moreover, by facilitating parallel execution, it can significantly decrease the time required for build and test processes, accelerating the development cycle.
In summary, npm-run-all represents more than just a utility for running scripts; it embodies a shift towards more efficient, reliable, and scalable Node.js development practices. By understanding the fundamentals of Node.js and npm, and leveraging tools like npm-run-all, developers can enhance their productivity and focus more on creating innovative solutions rather than managing the minutiae of script execution.
npm-run-all in CI/CD Pipelines
Enhancing Automation and Efficiency
The utility of npm-run-all extends far beyond local development environments. It plays a pivotal role in continuous integration (CI) and continuous deployment (CD) pipelines, automating tasks to ensure that software is efficiently built, tested, and deployed. Integrating npm-run-all into CI/CD pipelines with tools like Jenkins or GitHub Actions can significantly streamline these processes.
Consider a scenario where you need to run linting, unit tests, and integration tests as part of your CI pipeline. With npm-run-all, you can configure a single command in your CI configuration file to handle this sequence:
npm-run-all lint "test:unit" "test:integration"
# Output:
# Executes 'lint', 'test:unit', and 'test:integration' scripts sequentially, ensuring code quality and functionality before deployment.
This command showcases npm-run-all’s capability to simplify pipeline configurations, making your CI/CD processes more maintainable and less error-prone.
Complementing Tools and Practices
npm-run-all’s flexibility makes it an excellent companion to other development and deployment tools. For instance, Docker can be used to containerize your applications, ensuring consistency across environments, while npm-run-all manages the scripts that build and test your Docker images.
Additionally, incorporating Git hooks with npm-run-all can automate scripts to run before commits, pushes, or merges, enhancing code quality and consistency. This integration ensures that only code that meets your project’s standards is moved forward in the development process.
Further Resources for Mastering npm-run-all
To deepen your understanding of npm-run-all and its applications in modern development workflows, here are three external resources that provide valuable insights and examples:
- npm-run-all on GitHub – The official GitHub repository offers comprehensive documentation, usage examples, and the latest updates.
Automating with npm Scripts – An article that explores the power of npm scripts and how npm-run-all can be used to orchestrate them for complex tasks.
Explore CI/CD Integration – A guide on leveraging npm-run-all for efficient CI/CD pipelines, including practical examples with popular CI tools.
These resources offer a wealth of knowledge for developers looking to harness the full potential of npm-run-all in their projects. Whether you’re streamlining local development tasks or automating CI/CD pipelines, npm-run-all and its complementary tools and practices can elevate your development workflow to new heights.
Recap: The npm-run-all tool
In this comprehensive guide, we’ve explored the power of npm-run-all, a CLI tool that enhances the management of npm scripts, making your Node.js development process more efficient and streamlined. From the basic installation and usage to leveraging its advanced features for complex script management, npm-run-all proves to be an invaluable asset in the Node.js ecosystem.
We began with the basics, illustrating how to install npm-run-all and use it to run scripts sequentially or in parallel. This foundational knowledge sets the stage for more efficient development practices, reducing the complexity and time involved in script execution. We then advanced to more sophisticated uses, such as employing glob patterns for script selection and controlling the execution order, providing you with the tools to tackle more complex project requirements.
Exploring alternative tools and methods for managing npm scripts, such as concurrently, cross-env, and custom Node.js scripts, we compared their benefits and drawbacks. This comparative analysis aids in understanding the broader landscape of script management tools and selecting the best fit for your project needs.
Tool | Flexibility | Complexity | Use Case |
---|---|---|---|
npm-run-all | High | Moderate | Managing multiple npm scripts efficiently |
concurrently | High | Low | Running commands simultaneously |
cross-env | Moderate | Low | Handling environment variables across platforms |
Custom Node.js Scripts | Very High | High | Tailored script execution logic |
Whether you’re just starting out with npm-run-all or you’re an experienced developer looking to refine your script execution strategy, we hope this guide has provided you with valuable insights and practical tips. npm-run-all offers a blend of simplicity and power, enabling developers to focus more on building great applications and less on the intricacies of script management.
With its ability to run scripts in parallel or sequentially, and its compatibility with various Node.js projects, npm-run-all is a tool that can significantly enhance your development workflow. Experiment with npm-run-all in different project setups and discover how it can streamline your npm script execution, from basic tasks to complex build processes. Happy coding!