Python Exit | How To Terminate A Python Program At Will
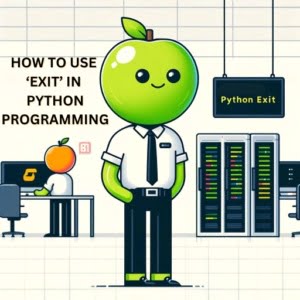
Python’s exit function is a crucial tool when developing software at IOFLOOD, as it facilitates graceful termination of programs and scripts. In our experience, Python’s exit function streamlines program termination and enhances control over script execution, particularly in scenarios requiring clean exits. Today’s article explores Python’s exit function, providing insights into its usage and best practices to empower our customers with effective scripting techniques for their custom server configurations.
In this blog post, we’ll delve into these questions to provide you with a comprehensive understanding of how sys.exit()
functions within the Python environment. We’ll explore its interactions with parent and child processes, and even discuss some alternative methods for terminating a process.
So, let’s dive into the world of Python process management together!
TL;DR: What is Python’s Exit function?
Python’s exit function,
sys.exit()
, is a built-in method used to terminate a Python script. It raises theSystemExit
exception which, if not caught, causes the Python interpreter to quit. It’s commonly used to manage the termination of Python programs, especially in multi-process applications.
import sys
sys.exit()
Table of Contents
Understanding sys.exit()
Let’s unravel the mystery of Python’s sys.exit()
. In the world of Python, sys.exit()
is a function that you can invoke to raise the SystemExit
exception. This exception, if not caught, prompts the Python interpreter to exit. It’s akin to pressing the ‘exit’ button programmatically.
So, how does sys.exit()
influence the exit status of a process? When you invoke sys.exit()
with an argument, that argument is taken as the exit status for the process. If you don’t provide an argument, the exit status defaults to zero, which conventionally denotes success.
The sys.exit()
function holds significant importance in multiprocessing programming. When dealing with multiple processes, having the ability to exit each one gracefully and with the appropriate status code is vital. sys.exit()
is a tool that enables you to do just that.
An intriguing aspect of sys.exit()
is its interaction with the try-except-finally
and try-finally
patterns.
Example of sys.exit() interaction with try-except-finally:
try:
sys.exit()
except SystemExit:
print('Caught the SystemExit exception')
finally:
print('Finally block is executed')
When sys.exit()
is invoked, it raises a SystemExit
exception which can be caught like any other exception. This implies that if sys.exit()
is invoked inside a try
or finally
block, the remainder of the block will be skipped, and any except
or finally
clauses will be executed.
Gaining a deep understanding of sys.exit()
is vital for managing the termination of Python programs in production code. It serves as a reliable exit door that allows your programs to complete their execution cleanly and predictably. So, whenever you’re contemplating how to gracefully terminate your Python programs, remember sys.exit()
– your dependable key to a smooth exit.
Exit Codes and sys.exit()
With a basic understanding of sys.exit()
under our belt, let’s delve deeper into the concept of exit codes in Python processes. Picture exit codes as the final words of a process before it takes a bow and exits the stage. These codes are essential in determining whether a process ended normally or encountered an error.
So, how do we set these exit codes? That’s where sys.exit()
comes into play. As we’ve already noted, when you call sys.exit()
with an argument, that argument is used as the exit status for the process. This means you can use sys.exit()
to set the exit code for your process. For instance, sys.exit(0)
signals a successful exit, while sys.exit(1)
typically indicates an abnormal termination.
import sys
# Successful exit
def success():
sys.exit(0)
# Abnormal termination
def failure():
sys.exit(1)
But what if we don’t provide an argument to sys.exit()
? In that scenario, Python automatically sets the exit code to zero. This is a useful feature as it allows us to have a default ‘success’ exit code without having to specify it every time.
However, it’s important to note that not all exit codes are created equal.
Here are some common exit codes and their meanings:
Exit Code | Meaning |
---|---|
0 | Success |
1 | General error or abnormal termination |
2 | Misuse of shell builtins |
126 | Command invoked cannot execute |
127 | Command not found |
128 | Invalid argument to exit |
130 | Script terminated by Control-C |
Different values can signify different outcomes. A zero exit code typically means the process was successful, while a non-zero exit code indicates an error. The exact meanings can vary depending on the system and the application, but the convention of zero for success and non-zero for failure is widely adopted.
In summary, exit codes are the silent communicators of process status. By understanding and correctly using exit codes, you can make your Python programs more robust and easier to debug. Remember, sys.exit()
is your ally when it comes to setting and understanding these vital status indicators.
Practical Application of sys.exit()
Having explored the theoretical aspects of sys.exit()
, it’s time to examine its practical applications. So, how do we actually employ sys.exit()
in Python? It’s quite straightforward – you simply invoke sys.exit()
at the point in your code where you wish your process to terminate. If you desire to specify an exit code, you can pass it as an argument, like sys.exit(0)
for a successful exit or sys.exit(1)
for an unsuccessful one.
Let’s consider an example. Assume we have a basic Python script that verifies if a certain condition is met. If the condition is not fulfilled, we want the script to terminate with an error code. Here’s how we can implement it:
import sys
if not condition:
sys.exit(1)
# rest of the code
In this example, if condition
is False
, sys.exit(1)
is invoked, and the process terminates with an exit code of 1, indicating an error. If condition
is True
, sys.exit(1)
is not invoked, and the rest of the code executes normally.
What if we pass a string as an argument to sys.exit()
? Python treats the string as an error message and prints it to stderr
before terminating the process. This can be handy for providing more information about why the process is terminating.
However, it’s crucial to understand that using sys.exit()
in different parts of your code can have varying implications. For instance, if you invoke sys.exit()
inside a try
block, it will raise a SystemExit
exception that can be caught by an except
clause.
Example of sys.exit() inside a try block:
try:
sys.exit()
except SystemExit:
print('Caught the SystemExit exception')
This allows you to perform cleanup actions or log an error message before the process terminates.
A remarkable feature of sys.exit()
is that it enables dynamic program termination based on user input. For example, you could have a script that asks the user for input, and if the user enters a specific command, the script invokes sys.exit()
and terminates.
In conclusion, sys.exit()
is a versatile tool that allows for controlled and dynamic termination of Python programs. By mastering its use, you can make your programs more robust, user-friendly, and easier to debug.
sys.exit() in Parent and Child Processes
Until now, our discussion has primarily revolved around the usage of sys.exit()
in a single process. But what transpires when we bring child processes into the equation? How does sys.exit()
operate in parent and child processes? Let’s delve into this.
Firstly, let’s examine how sys.exit()
functions in the main process. When you invoke sys.exit()
in the main process, it raises a SystemExit
exception. If this exception is not intercepted, the Python interpreter exits, leading to the termination of the process. While this is fairly straightforward, the scenario becomes a tad more complex when child processes are involved.
Imagine a scenario where a main process spawns a child process, and we invoke sys.exit()
in the child process. What’s the outcome? Here’s an illustrative example:
import os
import sys
from multiprocessing import Process
def child_process():
print('Starting child process')
sys.exit('Exiting child process')
if __name__ == '__main__':
p = Process(target=child_process)
p.start()
p.join()
print('Back in the main process')
In this scenario, the main process creates a new child process that invokes sys.exit()
. When sys.exit()
is invoked in the child process, it raises a SystemExit
exception, similar to the main process. However, this does not influence the main process. The main process continues to operate, and ‘Back in the main process’ is printed to the console.
But what if we invoke sys.exit()
in the main process while a child process is still operational? In that situation, the main process would terminate, but the child process would continue to operate until it completes its task. This is because each process operates in its own separate environment, and sys.exit()
only influences the process in which it is invoked.
Now, let’s discuss os._exit()
. Contrary to sys.exit()
, which raises an exception, os._exit()
terminates the process immediately without performing any cleanup operations or flushing stdio buffers. This can be beneficial in certain situations where you need to terminate a process immediately, but it should be used cautiously, as it can leave resources such as open files or network connections in an undefined state.
In conclusion, understanding how sys.exit()
and os._exit()
operate in parent and child processes is crucial for managing complex Python applications. By navigating the intricacies of these functions, you can ensure that your processes terminate gracefully and predictably, whether they are operating independently or as part of a multi-process application.
Alternative Termination Methods in Python
While sys.exit()
is a formidable tool for terminating Python processes, it’s not the only method available. Python offers several alternative methods for process termination, each with their unique uses and implications. Let’s examine some of these alternatives.
One such alternative to invoking sys.exit()
is to directly raise the SystemExit
exception. When you raise SystemExit
, Python behaves similarly to when sys.exit()
is invoked: it initiates the process of terminating the interpreter. Here’s how it’s done:
raise SystemExit
In this instance, the SystemExit
exception is raised, and unless it’s intercepted, the interpreter exits. This can be beneficial in scenarios where you wish to terminate the process from within an exception handler or a finally block.
However, using alternative termination methods like directly raising SystemExit
can have certain implications. For starters, it can make your code more challenging to comprehend, since it’s less explicit than invoking sys.exit()
. It also bypasses any cleanup operations that sys.exit()
performs, which can result in issues like resource leaks.
Another termination method in Python is to use the quit()
or exit()
functions. These functions operate similarly to sys.exit()
, but they are designed for use in the interactive interpreter, not in production code. When invoked, they raise the SystemExit
exception, which causes the interpreter to exit. However, they also have the side effect of printing a message to the console, which can be confusing in a production setting.
# Using quit()
quit()
# Using exit()
exit()
In conclusion, while sys.exit()
is the most prevalent method for terminating a Python process, it’s certainly not the only option. By understanding and utilizing alternative termination methods like directly raising SystemExit
or using quit()
or exit()
, you can gain more control over how your Python programs terminate. However, it’s crucial to use these alternatives judiciously, as they can impact your code’s clarity and robustness.
Further Resources for Python Iterator Mastery
To deepen your knowledge of Python control statements and loops, here are some free online guides:
- Python Loop Syntax Guide – Explore advanced loop patterns and their applications.
Python “for” Loop: Syntax and Usage – Dive into the world of “for” loops and their purpose in Python.
Python “for” Loop and Index – Learn about the benefits and challenges of using index-based “for” loops.
Tutorial on ‘For’ Loop in Python – Coursera presents a step-by-step tutorial on how to use ‘for’ loops in Python.
Generators in Python: Simplified – An article on Medium breaking down the concept and usage of generators in Python.
Python Control Flow Statements – A Medium article that simplifies the understanding of control flow statements in Python.
By immersing yourself in these resources and expanding your knowledge in these areas, you’ll enhance your ability to write efficient and effective Python code.
Python Exit Summary
In our exploration of Python’s sys.exit()
function, we’ve covered a vast terrain. We’ve understood its workings, its interaction with parent and child processes, and even its role in setting exit codes. We’ve also delved into alternative methods of process termination in Python, including directly raising the SystemExit
exception and using the quit()
and exit()
functions.
By employing sys.exit()
, we can gracefully terminate Python processes, providing an exit status that offers valuable information about the process’s outcome. In multiprocessing programming, sys.exit()
emerges as a crucial tool for managing the termination of individual processes. Furthermore, we’ve seen how sys.exit()
interacts with exception handling constructs like try-except-finally
blocks, giving us enhanced control over process termination.
Alternative termination methods like raising SystemExit
directly or using quit()
and exit()
can offer more control over process termination, but they come with their own caveats and should be used judiciously.
Transform your Python coding skills with our robust syntax resource guide.
In essence, understanding and properly utilizing sys.exit()
and its alternatives is critical for managing processes in Python. Whether you’re scripting a simple program or a complex multi-process application, these tools can aid in ensuring that your processes terminate gracefully and predictably. So, the next time you find yourself pondering over how to exit a Python process, remember the insights you’ve gathered here – and exit with confidence.