Java ‘Implements’ Keyword: A Detailed Guide
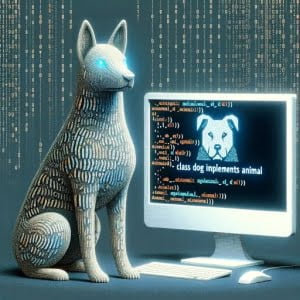
Are you finding it challenging to grasp the ‘implements’ keyword in Java? You’re not alone. Many developers find themselves puzzled when it comes to understanding and using this keyword effectively.
Think of the ‘implements’ keyword as a master key – a key that unlocks the door to interface-based programming in Java. It’s a powerful tool that, when used correctly, can significantly enhance your code’s flexibility and readability.
In this guide, we’ll walk you through the process of mastering the ‘implements’ keyword in Java, from its basic usage to more advanced techniques. We’ll cover everything from implementing a simple interface to dealing with multiple interfaces and even discuss alternative approaches.
So, let’s dive in and start mastering the ‘implements’ keyword in Java!
TL;DR: How Do I Use the ‘Implements’ Keyword in Java?
The
'implements'
keyword in Java is used to implement an interface, used with the syntaxclass Child implements Parent
. This keyword allows a class to inherit abstract methods from the interface, which it then has to provide implementations for. Here’s a simple example:
interface Animal {
void sound();
}
class Dog implements Animal {
public void sound() {
System.out.println("Woof!");
}
}
Dog dog = new Dog();
dog.sound();
# Output:
# 'Woof!'
In this example, we define an interface Animal
with a method sound()
. Then, we create a class Dog
that implements Animal
. By using the ‘implements’ keyword, we’re making a contract that Dog
will provide an implementation for all methods declared in Animal
. In this case, Dog
implements the sound()
method, which prints ‘Woof!’ when called.
This is a basic way to use the ‘implements’ keyword in Java, but there’s much more to learn about interface-based programming. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics of ‘Implements’ in Java
- Implementing Multiple Interfaces in Java
- Exploring Alternatives: Abstract Classes in Java
- Troubleshooting ‘Implements’ in Java
- Interfaces in Java and the Role of ‘Implements’
- The ‘Implements’ Keyword: Beyond Basics
- Wrapping Up: The ‘Implements’ Keyword in Java
Understanding the Basics of ‘Implements’ in Java
When starting with Java, one of the first things you’ll come across is the concept of interfaces and their implementation. The ‘implements’ keyword plays a crucial role in this. To put it simply, when a class ‘implements’ an interface, it provides the actual implementation of the methods declared in that interface.
Let’s look at a simple example:
interface Greeting {
void greet();
}
class EnglishGreeting implements Greeting {
public void greet() {
System.out.println("Hello!");
}
}
EnglishGreeting eg = new EnglishGreeting();
eg.greet();
# Output:
# 'Hello!'
In this code, we have an interface Greeting
with a method greet()
. The class EnglishGreeting
implements this interface, meaning it provides the actual implementation of the greet()
method. When we create an object of EnglishGreeting
and call the greet()
method, it prints ‘Hello!’.
This basic use of ‘implements’ allows us to create flexible and reusable code. With interfaces, we can define a set of methods that a class must have, without specifying how these methods should be implemented. This way, different classes can implement the same interface in different ways, providing flexibility and reusability.
However, there are some pitfalls to be aware of. One of the most common issues is forgetting to provide implementations for all methods declared in the interface. If a class doesn’t implement all methods from its interface, it must be declared as ‘abstract’. Otherwise, the code will not compile. We’ll discuss this and other issues in more detail in the ‘Troubleshooting and Considerations’ section.
Implementing Multiple Interfaces in Java
As you grow more comfortable with the ‘implements’ keyword in Java, you’ll discover that its power extends beyond implementing a single interface. A class in Java can actually implement multiple interfaces, opening the door to greater flexibility and complexity in your coding projects.
Consider the following example:
interface Eater {
void eat();
}
interface Sleeper {
void sleep();
}
class Human implements Eater, Sleeper {
public void eat() {
System.out.println("Eating...");
}
public void sleep() {
System.out.println("Sleeping...");
}
}
Human human = new Human();
human.eat();
human.sleep();
# Output:
# 'Eating...'
# 'Sleeping...'
In this example, we have two interfaces, Eater
and Sleeper
, each with their own method. The Human
class implements both these interfaces, meaning it provides implementations for both the eat()
and sleep()
methods.
This allows us to define more complex behavior in our classes. By implementing multiple interfaces, we can ensure that a class adheres to multiple ‘contracts’, each defined by an interface. It’s a powerful way to enhance the functionality of your classes and promote code reuse.
However, with this advanced usage comes a potential pitfall. What if the interfaces have methods with the same signature? In that case, the class can provide only one implementation that will be shared across all interfaces. This is something to keep in mind when designing your interfaces and classes.
Exploring Alternatives: Abstract Classes in Java
While the ‘implements’ keyword and interfaces are powerful tools in Java, they’re not the only ways to achieve code reuse and flexibility. An alternative approach to interface-based programming involves using abstract classes.
Abstract classes, like interfaces, can contain abstract methods that must be implemented by any class that extends the abstract class. However, unlike interfaces, abstract classes can also contain implemented methods, fields, and constructors.
Consider the following example:
abstract class Animal {
abstract void sound();
void eat() {
System.out.println("Eating...");
}
}
class Dog extends Animal {
public void sound() {
System.out.println("Woof!");
}
}
Dog dog = new Dog();
dog.sound();
dog.eat();
# Output:
# 'Woof!'
# 'Eating...'
In this code, Animal
is an abstract class with an abstract method sound()
and a concrete method eat()
. The Dog
class extends Animal
, providing an implementation for the sound()
method. When we create a Dog
object and call the sound()
and eat()
methods, it prints ‘Woof!’ and ‘Eating…’, respectively.
This approach offers some benefits over interfaces. For instance, it allows for code reuse through implemented methods in the abstract class. It also allows for defining fields and constructors, which can’t be done in interfaces.
However, it comes with a significant drawback: a class can extend only one abstract class, but it can implement multiple interfaces. This limits flexibility compared to interface-based programming.
When deciding between using interfaces with ‘implements’ or abstract classes, consider the needs of your project. If you need to define fields, constructors, or implemented methods, an abstract class might be the way to go. If you need more flexibility and want to adhere to multiple ‘contracts’, interfaces and ‘implements’ might be a better choice.
Troubleshooting ‘Implements’ in Java
While using the ‘implements’ keyword in Java, you might encounter some common issues. Let’s discuss these problems and how to resolve them.
Not Implementing All Methods of an Interface
One of the most common issues is not implementing all the methods of an interface. When a class implements an interface, it signs a ‘contract’ to provide implementations for all the methods declared in the interface. Failing to do so results in a compilation error.
Consider the following example:
interface Animal {
void sound();
void walk();
}
class Dog implements Animal {
public void sound() {
System.out.println("Woof!");
}
}
# Output:
# Compilation error: The type Dog must implement the inherited abstract method Animal.walk()
In this code, the Animal
interface has two methods: sound()
and walk()
. However, the Dog
class only implements the sound()
method. This results in a compilation error, as Dog
has not fulfilled its ‘contract’ to implement all methods from Animal
.
The solution is simple: ensure that your class provides implementations for all methods declared in the interface. If it can’t, you should declare the class as ‘abstract’.
abstract class Dog implements Animal {
public void sound() {
System.out.println("Woof!");
}
}
In this revised code, Dog
is declared as an abstract class. This indicates that Dog
is not providing implementations for all methods from Animal
, and that’s okay because it’s abstract. Any non-abstract class that extends Dog
will have to provide the missing implementations.
Understanding these common issues and their solutions will help you use the ‘implements’ keyword more effectively in your Java projects.
Interfaces in Java and the Role of ‘Implements’
To fully grasp the power of the ‘implements’ keyword in Java, it’s essential to understand the concept of interfaces and how they relate to other fundamental concepts like inheritance and polymorphism.
An interface in Java is a blueprint of a class. It’s a collection of abstract methods (and constants) that any class ‘implementing’ the interface agrees to provide implementations for. Unlike classes, interfaces don’t contain any implemented methods (except for default and static methods since Java 8).
Here’s a basic example of an interface in Java:
interface Animal {
void sound();
}
In this example, Animal
is an interface with a single method sound()
. Any class that implements Animal
will need to provide an implementation for sound()
.
The ‘implements’ keyword is used by a class to implement an interface. Once a class implements an interface, it inherits the abstract methods of the interface.
Moving on to inheritance, it’s a mechanism in Java that allows one class to inherit the fields and methods of another class. The ‘implements’ keyword is a form of inheritance, as the implementing class inherits the methods of the interface.
Polymorphism, on the other hand, is a concept that allows us to perform a single action in different ways. In Java, we can achieve polymorphism by interfaces. When a class implements an interface, you can use an instance of that class as an instance of the interface, opening the door to various forms of polymorphism such as method overriding or dynamic method dispatch.
In essence, the ‘implements’ keyword in Java is a powerful tool that ties together interfaces, inheritance, and polymorphism, allowing for more flexible, reusable, and organized code.
The ‘Implements’ Keyword: Beyond Basics
As we’ve discussed, the ‘implements’ keyword in Java is a potent tool, enabling us to use interface-based programming to create flexible, reusable, and organized code. But its relevance and utility extend beyond these basic uses, particularly when we consider larger projects and design patterns.
The Role of ‘Implements’ in Larger Projects
In larger projects, the ‘implements’ keyword becomes even more crucial. It allows us to define a common set of methods that different classes must implement, promoting consistency across the project. This can be incredibly beneficial in a team setting, where multiple developers are working on different parts of the project. By using interfaces and ‘implements’, we can ensure that everyone is on the same page about what methods certain classes must have.
‘Implements’ and Design Patterns
The ‘implements’ keyword also plays a significant role in various design patterns in Java. For instance, in the Factory pattern, we often use an interface to define a method for creating an object, and then have multiple classes implement this interface to provide different ways of creating the object. Similarly, in the Strategy pattern, we use an interface to define a method for performing a certain action, and then have multiple classes implement this interface to provide different strategies for performing the action.
Further Resources for ‘Implements’ Keyword in Java
To delve deeper into the ‘implements’ keyword and its uses in Java, consider exploring the following resources:
- Java Keywords Logic – Understand managing thread safety with keywords like “volatile” and “synchronized” in Java.
Volatile Usage in Java – Examples where ‘volatile’ is used to coordinate communication between threads in Java concurrency.
Transient Keyword in Java – Learn how ‘transient’ keyword prevents specific fields from being included during object persistence.
Java Interfaces and Inheritance – A detailed guide from Oracle’s official Java documentation.
Java Interface Tutorial – A comprehensive tutorial on Java interfaces at JavaTpoint.
Java Design Patterns – A collection of Java design patterns where ‘implements’ is often used.
Wrapping Up: The ‘Implements’ Keyword in Java
In this comprehensive guide, we’ve navigated the intricacies of the ‘implements’ keyword in Java, a crucial tool for interface-based programming.
We started with the basics, learning how to use ‘implements’ to provide concrete implementations for the methods declared in an interface. We then advanced to using ‘implements’ to make a class adhere to multiple interfaces, a powerful feature that enhances code flexibility and complexity.
We also explored alternative approaches, such as using abstract classes instead of interfaces. While this method has its own benefits, like allowing for implemented methods, fields, and constructors, it also has its limitations, such as only being able to extend one abstract class compared to implementing multiple interfaces.
We delved into common issues you might face when using ‘implements’, such as not implementing all methods of an interface, and provided solutions to these problems. We also discussed the role of ‘implements’ in larger projects and design patterns, demonstrating its relevance beyond basic usage.
Here’s a quick comparison of using ‘implements’ with interfaces versus using abstract classes:
Method | Flexibility | Code Reuse | Complexity |
---|---|---|---|
Implements with Interfaces | High | Moderate | Moderate |
Abstract Classes | Moderate | High | High |
Whether you’re just starting out with ‘implements’ in Java or looking to deepen your understanding, we hope this guide has served as a valuable resource. With ‘implements’, you can create flexible, reusable, and organized code, enhancing your Java programming skills.
The journey to mastering ‘implements’ in Java is a rewarding one, opening the door to powerful programming techniques. Happy coding!