Understanding Java Primitive Data Types
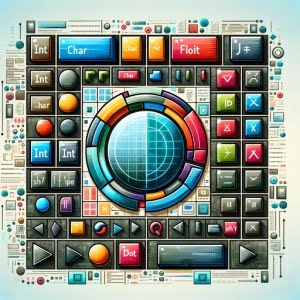
Have you ever pondered the fundamental building blocks of data in Java? Like the elemental particles that make up the universe, Java too has its own basic data types – the primitive types.
This guide will illuminate the eight primitive types in Java, helping you comprehend their characteristics and application. We’ll dive into each type, exploring its size, range, and usage, and provide practical examples to help you understand how to use them effectively.
So, let’s embark on this journey to unravel the mysteries of Java primitive types!
TL;DR: What Are the Primitive Types in Java?
Java has eight primitive types:
byte, short, int, long, float, double, char, and boolean.
Each type has its own unique characteristics and uses. For instance, ‘int’ is used for integers, while ‘boolean’ is used for true/false values.
Here’s a simple example:
int myNumber = 10;
boolean isJavaFun = true;
System.out.println(myNumber);
System.out.println(isJavaFun);
# Output:
# 10
# true
In this example, we’ve declared an integer variable ‘myNumber’ and assigned it the value 10. We’ve also declared a boolean variable ‘isJavaFun’ and assigned it the value true. The System.out.println
function is then used to print the values of these variables.
This is just a basic introduction to Java’s primitive types, but there’s much more to learn about them. Continue reading for a more detailed exploration of each type and their uses.
Table of Contents
- Understanding Java’s Primitive Types
- Applying Java Primitive Types in Complex Scenarios
- Exploring Wrapper Classes in Java
- Troubleshooting Common Issues with Java Primitive Types
- The Concept of Data Types in Programming
- The Need for Different Data Types
- Java’s Primitive Types: The Building Blocks of Data
- Going Beyond Java Primitive Types
- Wrapping Up: Mastering Java Primitive Types
Understanding Java’s Primitive Types
Java has eight primitive types, each with its own size, range, and usage. Let’s delve into each one:
Byte
The ‘byte’ data type in Java is an 8-bit signed two’s complement integer. It has a minimum value of -128 and a maximum value of 127 (inclusive). Here’s how you can declare and use a byte variable:
byte myByte = 10;
System.out.println(myByte);
# Output:
# 10
In this example, we’ve declared a byte variable ‘myByte’ and assigned it the value 10. The System.out.println
function is then used to print the value of this variable.
Short
The ‘short’ data type in Java is a 16-bit signed two’s complement integer. It has a minimum value of -32,768 and a maximum value of 32,767 (inclusive). Here’s how you can declare and use a short variable:
short myShort = 5000;
System.out.println(myShort);
# Output:
# 5000
In this example, we’ve declared a short variable ‘myShort’ and assigned it the value 5000. The System.out.println
function is then used to print the value of this variable.
Int
The ‘int’ data type in Java is a 32-bit signed two’s complement integer. It has a minimum value of -2,147,483,648 and a maximum value of 2,147,483,647 (inclusive). Here’s how you can declare and use an int variable:
int myInt = 100000;
System.out.println(myInt);
# Output:
# 100000
In this example, we’ve declared an int variable ‘myInt’ and assigned it the value 100000. The System.out.println
function is then used to print the value of this variable.
Long
The ‘long’ data type in Java is a 64-bit signed two’s complement integer. It has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive). Here’s how you can declare and use a long variable:
long myLong = 10000000000L;
System.out.println(myLong);
# Output:
# 10000000000
In this example, we’ve declared a long variable ‘myLong’ and assigned it the value 10000000000. Note that we’ve added an ‘L’ at the end of the value to indicate that it’s a long literal. The System.out.println
function is then used to print the value of this variable.
Float
The ‘float’ data type in Java is a single-precision 32-bit IEEE 754 floating point. It’s used to store fractional numbers and is sufficient for storing 6 to 7 decimal digits. Here’s how you can declare and use a float variable:
float myFloat = 10.99f;
System.out.println(myFloat);
# Output:
# 10.99
In this example, we’ve declared a float variable ‘myFloat’ and assigned it the value 10.99. Note that we’ve added an ‘f’ at the end of the value to indicate that it’s a float literal. The System.out.println
function is then used to print the value of this variable.
Double
The ‘double’ data type in Java is a double-precision 64-bit IEEE 754 floating point. It’s used to store fractional numbers and is sufficient for storing 15 decimal digits. Here’s how you can declare and use a double variable:
double myDouble = 20.99;
System.out.println(myDouble);
# Output:
# 20.99
In this example, we’ve declared a double variable ‘myDouble’ and assigned it the value 20.99. The System.out.println
function is then used to print the value of this variable.
Char
The ‘char’ data type in Java is a single 16-bit Unicode character. It has a minimum value of ” (or 0) and a maximum value of ‘’ (or 65,535 inclusive). Here’s how you can declare and use a char variable:
char myChar = 'A';
System.out.println(myChar);
# Output:
# A
In this example, we’ve declared a char variable ‘myChar’ and assigned it the value ‘A’. The System.out.println
function is then used to print the value of this variable.
Boolean
The ‘boolean’ data type in Java represents one bit of information, but its size isn’t precisely defined. It has only two possible values: true and false. Here’s how you can declare and use a boolean variable:
boolean isJavaFun = true;
System.out.println(isJavaFun);
# Output:
# true
In this example, we’ve declared a boolean variable ‘isJavaFun’ and assigned it the value true. The System.out.println
function is then used to print the value of this variable.
Applying Java Primitive Types in Complex Scenarios
Now that we have a basic understanding of each primitive type, let’s delve into more complex scenarios and discuss best practices for using these data types.
Choosing the Right Type
One of the key aspects of using primitive types effectively is choosing the right type for the right situation. For instance, if you’re dealing with large numbers that exceed the range of ‘int’, you should use ‘long’. If you’re dealing with fractional numbers, you should use ‘double’ or ‘float’ based on the precision required.
Using Primitive Types in Expressions
When using primitive types in expressions, it’s important to understand how type promotion works. For instance, in an expression involving different types of numbers, all numbers are promoted to the largest type present. Here’s an example:
int myInt = 5;
double myDouble = 6.5;
double result = myInt + myDouble;
System.out.println(result);
# Output:
# 11.5
In this example, the ‘int’ is promoted to a ‘double’ before the addition operation. The result is a ‘double’ as well.
Casting
Sometimes, you might need to convert a variable of one primitive type into another. This is known as casting. Here’s an example:
double myDouble = 9.78;
int myInt = (int) myDouble;
System.out.println(myInt);
# Output:
# 9
In this example, the ‘double’ is cast to an ‘int’. Note that the fractional part is lost in the process.
Best Practices
When working with primitive types, here are some best practices to keep in mind:
- Always choose the smallest type that can comfortably hold your data. This can help save memory.
- Avoid mixing types in an expression unless necessary. This can prevent unexpected results due to type promotion.
- When casting, be aware that you might lose information (like the fractional part when casting from ‘double’ to ‘int’).
- Always initialize your variables before using them. Java doesn’t initialize local variables automatically.
Exploring Wrapper Classes in Java
While primitive types are incredibly useful in Java, there are situations where you might need an object representation of these types. This is where wrapper classes come into play.
Understanding Wrapper Classes
Wrapper classes provide a way to use primitive data types (int, boolean, etc.) as objects. The Java library provides a wrapper class for each of the eight primitive data types. In essence, a wrapper class wraps (encloses) around a primitive data type and gives it an object appearance.
Here’s an example of how you can create a wrapper object for an ‘int’:
int myInt = 5;
Integer myInteger = Integer.valueOf(myInt);
System.out.println(myInteger);
# Output:
# 5
In this example, the Integer.valueOf
method is used to create an Integer
object from an ‘int’.
Autoboxing and Unboxing
Autoboxing is the automatic conversion of primitive types to their object wrapper classes. Unboxing is the reverse process of converting an object of a wrapper class to its corresponding primitive type.
Here’s an example of autoboxing and unboxing:
// Autoboxing
int myInt = 10;
Integer myInteger = myInt;
System.out.println(myInteger);
// Unboxing
int myNewInt = myInteger;
System.out.println(myNewInt);
# Output:
# 10
# 10
In this example, autoboxing occurs when the ‘int’ is assigned to the Integer
object. Unboxing occurs when the Integer
object is assigned to the ‘int’.
When to Use Wrapper Classes
While primitive types are more efficient in terms of memory and performance, there are situations where you might need to use wrapper classes instead:
- When working with collections (like
ArrayList
) that cannot store primitive types. - When you need to use methods provided by the wrapper classes to convert strings to numbers or vice versa.
- When you need
null
values, which are not allowed in primitive types.
Understanding the use of wrapper classes and the concept of autoboxing and unboxing is crucial for advanced Java programming.
Troubleshooting Common Issues with Java Primitive Types
While working with Java’s primitive types, you might encounter some common issues. Let’s discuss these problems and their solutions.
Type Overflow and Underflow
Type overflow occurs when a number is too large to be represented by a particular type. Conversely, underflow happens when a number is too small. In both cases, the results can be unpredictable.
Here’s an example of overflow with the ‘byte’ type:
byte myByte = 127;
myByte++;
System.out.println(myByte);
# Output:
# -128
In this example, we’ve incremented a ‘byte’ variable that’s already at its maximum value. Instead of getting an error, the value wraps around to the minimum value.
To prevent overflow and underflow, always make sure that the numbers you’re working with are within the range of your data type.
Precision Issues with Floating-Point Types
Floating-point types (‘float’ and ‘double’) can sometimes lead to precision issues due to the way they’re represented in memory. This can lead to unexpected results when performing calculations.
Here’s an example:
double myDouble = 0.1 + 0.2;
System.out.println(myDouble);
# Output:
# 0.30000000000000004
In this example, the result of the calculation is slightly more than 0.3 due to precision issues.
To avoid this, consider using the ‘BigDecimal’ class for high-precision calculations. However, keep in mind that ‘BigDecimal’ is slower and more memory-intensive than ‘double’ or ‘float’.
Understanding these common issues and their solutions can help you write more robust and accurate Java code.
The Concept of Data Types in Programming
In the world of programming, data types are fundamental. They define the kind of data that a variable can hold, such as integers, floating-point numbers, characters, or boolean values. Each data type requires different amounts of memory and has specific operations that can be performed on it.
Consider this example:
int myNumber = 5;
boolean isJavaFun = true;
System.out.println(myNumber);
System.out.println(isJavaFun);
# Output:
# 5
# true
In this example, ‘myNumber’ is a variable of type ‘int’, and ‘isJavaFun’ is a variable of type ‘boolean’. The type of these variables determines what kind of data they can hold and what operations can be performed on them.
The Need for Different Data Types
Different data types are needed to handle different kinds of data efficiently. For instance, if you’re dealing with whole numbers, you can use an integer type like ‘int’ or ‘long’. If you’re dealing with fractional numbers, you can use a floating-point type like ‘float’ or ‘double’. If you’re dealing with true/false values, you can use the ‘boolean’ type.
Having a variety of data types at your disposal allows you to choose the most appropriate type for your data, leading to more efficient and effective code.
Java’s Primitive Types: The Building Blocks of Data
In Java, the primitive types are the simplest and most fundamental data types. They form the building blocks of data manipulation in Java. As we’ve seen, Java has eight primitive types: byte, short, int, long, float, double, char, and boolean.
Each of these types serves a specific purpose and has a specific range of values it can represent. By understanding these types and how to use them, you can write more efficient and effective Java code.
Going Beyond Java Primitive Types
Understanding Java’s primitive types is not just about knowing what they are and how to use them. It’s about understanding how they underpin many of the more advanced aspects of Java programming, such as memory management and performance optimization.
Memory Management and Performance Optimization
Each primitive type in Java has a specific size, which determines how much memory it uses. By choosing the most appropriate type for your data, you can manage memory more efficiently and improve the performance of your programs.
For instance, if you’re dealing with small integers, you can use ‘byte’ or ‘short’ instead of ‘int’ or ‘long’ to save memory. Similarly, if you don’t need the precision of ‘double’, you can use ‘float’ instead.
The Power of Java Operators and Control Structures
Understanding primitive types also unlocks the power of Java operators and control structures. For instance, you can use arithmetic operators to perform calculations on numeric types, relational operators to compare values, and logical operators to combine boolean values.
Here’s an example of using operators with primitive types:
int num1 = 10;
int num2 = 20;
boolean result = (num1 < num2) && (num1 + num2 > 30);
System.out.println(result);
# Output:
# false
In this example, we’re using the less than operator (<
) to compare ‘num1’ and ‘num2’, the addition operator (+
) to add ‘num1’ and ‘num2’, and the logical AND operator (&&
) to combine the results. The result is ‘false’ because ‘num1’ is less than ‘num2’ but ‘num1’ + ‘num2’ is not greater than 30.
Further Resources for Mastering Java Primitive Types
To help you delve deeper into Java’s primitive types, here are some resources that you might find useful:
- Oracle’s Java Tutorials – A comprehensive guide to Java’s data types, including primitive types.
- GeeksforGeeks Java Primitive Data Types – This tutorial goes into more detail about each of Java’s primitive types.
- Java Primitive Data Types by TutorialsPoint: This is a free and in-depth guide to understanding Java primitive data types. It provides clear definitions, detailed explanations of how each type is used, and uses examples to demonstrate different scenarios, making it a great resource for both beginners and those looking to refresh their knowledge.
Wrapping Up: Mastering Java Primitive Types
In this comprehensive guide, we’ve explored the fundamental building blocks of data in Java – the primitive types. We’ve delved into the eight primitive types in Java, understanding their characteristics, size, range, and usage, and how these aspects influence the memory management and performance optimization of your Java programs.
We began with the basics, discussing each primitive type in detail, with practical examples illustrating their declaration and usage. We then delved into more complex scenarios, discussing how and when to use each primitive type, and providing best practices for their effective application. We also explored the concept of wrapper classes, their advantages, and their role in autoboxing and unboxing.
We tackled common issues related to primitive types, such as type overflow and underflow, and precision issues with floating-point types, providing solutions to these challenges. We also discussed the concept of data types in programming, the need for different data types, and how Java’s primitive types fit into this picture.
Here’s a quick comparison of the Java primitive types we’ve discussed:
Type | Size | Minimum Value | Maximum Value |
---|---|---|---|
byte | 8-bit | -128 | 127 |
short | 16-bit | -32,768 | 32,767 |
int | 32-bit | -2,147,483,648 | 2,147,483,647 |
long | 64-bit | -9,223,372,036,854,775,808 | 9,223,372,036,854,775,807 |
float | 32-bit | 1.4e-45 | 3.4028235e+38 |
double | 64-bit | 4.9e-324 | 1.7976931348623157e+308 |
char | 16-bit | ” | ‘’ |
boolean | N/A | false | true |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of its fundamental data types, we hope this guide has given you a solid foundation in Java’s primitive types and their effective application.
Understanding these fundamental building blocks of data in Java is crucial for writing efficient and effective Java code. Keep exploring, keep learning, and as always, happy coding!