Ternary Operator in Java: An If…Else Shorthand Guide
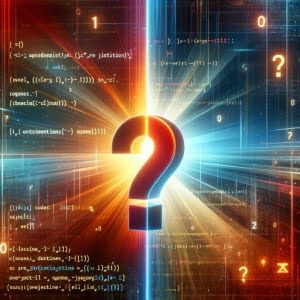
Ever felt like you’re wrestling with the ternary operator in Java? You’re not alone. Many developers find the ternary operator a bit daunting. Think of the ternary operator as a traffic signal – it directs the flow of your code based on certain conditions, making it a powerful tool in your Java toolkit.
The ternary operator is a shorthand for an if-else statement in Java. It takes three operands: a condition, a value if the condition is true, and a value if the condition is false. This operator can be a bit tricky to master, but once you do, it can make your code more concise and readable.
In this guide, we’ll help you understand and master the use of the ternary operator in Java, from its basic use to more advanced scenarios. We’ll cover everything from how the operator works, its advantages, potential pitfalls, to more complex uses such as nested ternary operations. We’ll also explore alternative approaches and provide tips for best practices and optimization.
So, let’s dive in and start mastering the ternary operator in Java!
TL;DR: What is the Ternary Operator in Java?
The ternary operator in Java is a concise way to perform conditional operations, defined by three operands: a condition, a value if the condition is true, and a value if the condition is false. Formatted with the syntax,
dataType variableName = condition ? value_if_true : value_if_false;
It’s main use is as shorthand for an if-else statement:
Here’s a simple example:
int result = (10 > 5) ? 1 : 0;
// Output:
// 1
In this example, the condition is 10 > 5
. Since this condition is true, the ternary operator returns the second operand 1
, and assigns it to the variable result
. If the condition was false, it would return the third operand 0
.
This is just a basic use of the ternary operator in Java. There’s much more to learn about this versatile operator, including its advanced usage and alternatives. Continue reading for a deeper dive.
Table of Contents
Java Ternary Operator: The Basics
The ternary operator in Java is a simple and efficient way to perform conditional operations. It’s a compact version of the if-else statement, and is often used to make code more concise and readable.
The ternary operator takes three operands: a condition to check, a result for when the condition is true, and a result for when the condition is false.
Here’s the basic syntax:
condition ? value_if_true : value_if_false;
Let’s break down a simple example:
int number = 15;
String result = (number > 10) ? "Greater than ten" : "Less than or equal to ten";
// Output:
// "Greater than ten"
In this example, the condition is number > 10
. The ternary operator checks if this condition is true. Since 15
is indeed greater than 10
, the operator returns the second operand ("Greater than ten"
), and assigns it to the variable result
. If number
was less than or equal to 10
, it would return the third operand ("Less than or equal to ten"
).
The ternary operator offers a more concise way to write if-else statements, making your code easier to read and write. However, it’s important to use this operator wisely. Overuse can lead to code that’s difficult to understand and maintain. Always prioritize readability and simplicity over brevity.
Advanced Usage: Nested Ternary Operators in Java
As you become more comfortable with the ternary operator, you might start to explore more complex uses, such as nested ternary operations. A nested ternary operation is when a ternary operator is used within another ternary operator.
Here’s an example of a nested ternary operation:
int number = 15;
String result = (number > 10) ? (number > 20 ? "Greater than twenty" : "Between ten and twenty") : "Less than or equal to ten";
// Output:
// "Between ten and twenty"
In this example, if the first condition number > 10
is true, it checks another condition number > 20
. If this second condition is also true, it returns "Greater than twenty"
. If the second condition is false (but the first is true), it returns "Between ten and twenty"
. If the first condition is false, it returns "Less than or equal to ten"
.
While nested ternary operations can be powerful, they can also make your code more complex and harder to read. It’s important to use nested ternary operations sparingly and only when it improves the readability or efficiency of your code.
Remember, the key to using the ternary operator effectively is to balance brevity with readability. Always strive to write code that’s easy to understand and maintain.
Alternative Approaches to the Ternary Operator
While the ternary operator is a powerful tool in Java, it’s not the only way to handle conditional logic. In fact, more traditional control flow structures like if-else
statements and switch
statements can often accomplish the same tasks. Understanding these alternatives is crucial for writing clean, readable, and efficient code.
The Traditional If-Else Statement
The if-else
statement is the most straightforward way to handle conditional logic in Java. It’s more verbose than the ternary operator, but it can be easier to read, especially for complex conditions.
Here’s how you might rewrite our previous example using an if-else
statement:
int number = 15;
String result;
if (number > 10) {
if (number > 20) {
result = "Greater than twenty";
} else {
result = "Between ten and twenty";
}
} else {
result = "Less than or equal to ten";
}
// Output:
// "Between ten and twenty"
In this example, the code is longer and more verbose, but it’s also arguably easier to understand at a glance. This is particularly true for cases with complex or nested conditions.
The Switch Statement
The switch
statement is another alternative to the ternary operator, particularly when dealing with multiple conditions. While it can’t directly replace the ternary operator, it’s a useful tool to have in your toolkit.
Here’s an example:
int number = 15;
String result;
switch (number) {
case 10:
result = "Exactly ten";
break;
case 20:
result = "Exactly twenty";
break;
default:
result = "Neither ten nor twenty";
break;
}
// Output:
// "Neither ten nor twenty"
In this example, the switch
statement checks if number
is 10
or 20
. If number
is neither 10
nor 20
, it defaults to the default
case.
While the ternary operator is a powerful and concise way to handle conditional logic in Java, it’s not always the best tool for the job. Understanding when to use the ternary operator, and when to use alternatives like if-else
or switch
statements, is a key skill for any Java developer.
Troubleshooting Java’s Ternary Operator
While the ternary operator in Java is quite handy, it’s not without its challenges. It’s essential to understand common pitfalls and how to avoid them.
Misunderstanding Operator Precedence
One common issue arises from misunderstanding operator precedence. The ternary operator has lower precedence than most other operators, which can lead to unexpected results.
Consider the following example:
int number = 5;
int result = number == 5 ? 1 : 0 + 1;
// Output:
// 1
At first glance, you might expect result
to be 2
when number
is 5
. But because the addition operator has higher precedence than the ternary operator, 0 + 1
is evaluated first, leading to a result
of 1
.
To avoid this, use parentheses to make your intentions clear:
int number = 5;
int result = number == 5 ? 1 : (0 + 1);
// Output:
// 1
Overusing Nested Ternary Operators
While nested ternary operators can be powerful, overusing them can lead to code that’s hard to read and debug. If you find yourself nesting multiple ternary operators, consider using traditional if-else
statements or switch
statements instead.
Ignoring Readability
Remember, the main purpose of the ternary operator is to make your code more concise and readable. If using the ternary operator makes your code harder to understand, it’s better to stick with if-else
statements.
In conclusion, while the ternary operator can be a powerful tool in your Java toolkit, it’s important to use it wisely. Always consider the readability and maintainability of your code, and don’t be afraid to use alternative approaches when they’re more suitable.
Understanding Java Operators and Control Flow
To fully grasp the ternary operator’s power and utility, it’s essential to understand the broader concepts of operators and control flow in Java.
Operators in Java
In Java, an operator is a special symbol that performs specific operations on one, two, or three operands, and then returns a result. The basic types of operators in Java include arithmetic, relational, bitwise, logical, and assignment operators.
The ternary operator falls under the category of conditional operators, which perform different computations depending on whether a programmer-specified boolean condition evaluates to true or false.
Control Flow in Java
Control flow, or flow of control, refers to the order in which the individual statements, instructions, or function calls of an imperative or a declarative program are executed or evaluated.
In Java, control flow statements break up the flow of execution by employing decision making, looping, and branching, enabling your program to conditionally execute particular blocks of code.
Here’s a simple example of control flow using an if-else statement:
int number = 10;
if (number > 5) {
System.out.println("Number is greater than 5");
} else {
System.out.println("Number is less than or equal to 5");
}
// Output:
// "Number is greater than 5"
In this example, the control flow of the program is determined by the if-else
statement. If the condition number > 5
is true, the program outputs "Number is greater than 5"
. Otherwise, it outputs "Number is less than or equal to 5"
.
Understanding operators and control flow is fundamental to mastering the ternary operator and other advanced features in Java. With this foundation, you’ll be better equipped to write efficient, readable, and maintainable code.
Applying the Ternary Operator in Larger Projects
The ternary operator’s real power shines when it’s used in larger programs or projects. It can help make your code more concise and readable, especially when dealing with complex conditional logic.
Consider a large program that involves multiple conditions. Using traditional if-else
statements could result in verbose and hard-to-read code. In such cases, the ternary operator can be a valuable tool for writing cleaner and more maintainable code.
However, as with any tool, it’s important to use the ternary operator wisely. Overusing the ternary operator, especially nested ternary operations, can make your code more complex and harder to debug. Always strive to balance brevity with readability.
Related Topics to Explore
The ternary operator often goes hand in hand with other concepts in Java. Here are a few related topics that you might find interesting:
- Control Flow in Java: The ternary operator is a part of Java’s control flow. Understanding other control flow structures, like loops and switch statements, can help you write more efficient and readable code.
Java Operators: The ternary operator is just one of many operators in Java. Learning about other operators, like arithmetic, relational, and logical operators, can deepen your understanding of Java.
Java Best Practices: Writing clean, efficient, and maintainable code is a key skill for any Java developer. Learning about Java best practices, including when and how to use the ternary operator, can help you become a better programmer.
Further Resources for Mastering Java’s Ternary Operator
Here are some additional resources that provide more in-depth information about the ternary operator and related topics:
- Java Operator Mastery Simplified – Explore Java’s arithmetic operators for mathematical calculations.
The Not Equals Operator in Java – Understand how “!=” differs from the
"=="
operator in Java for inequality comparison.Exploring || Operator Usage – Understand how the “||” operator evaluates to true if at least one of the operands is true.
Java Ternary Operator – Direct from Oracle, this is an official guide to Java’s ternary operator.
Java Operators – W3Schools provides an extensive overview of the different operators in Java.
Java Ternary Operator Guide – GeeksforGeeks offers a practical guide to using the ternary operator in Java.
Wrapping Up
In this comprehensive guide, we’ve delved deep into the world of Java’s ternary operator, a versatile tool for handling conditional logic in your code.
We started with the basics, understanding how the ternary operator works and how to use it in simple scenarios. We then delved into more complex uses, exploring nested ternary operations and how they can make your code more concise. Along the way, we tackled common challenges and pitfalls when using the ternary operator and provided solutions to help you write cleaner, more efficient code.
We also explored alternative approaches to handling conditional logic, comparing the ternary operator with traditional if-else
and switch
statements. Here’s a quick comparison of these methods:
Method | Conciseness | Readability | Complexity |
---|---|---|---|
Ternary Operator | High | Moderate | Moderate |
If-Else Statement | Low | High | Low |
Switch Statement | Moderate | High | Moderate |
Whether you’re just starting out with Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the ternary operator and its applications.
With its balance of conciseness and flexibility, the ternary operator is a powerful tool in any Java developer’s toolkit. Use it wisely, and it can help you write cleaner, more efficient code. Happy coding!