Using Java Operators: From Basics to Advanced
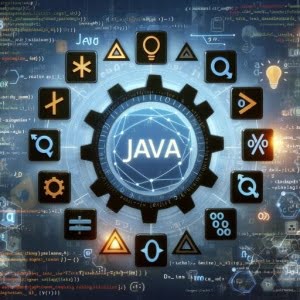
Are you finding it challenging to understand Java operators? You’re not alone. Many developers find themselves puzzled when it comes to handling operators in Java, but we’re here to help.
Just like a skilled mathematician, Java uses operators to perform operations on variables and values. These operators are the building blocks of any Java program, allowing us to perform a variety of tasks, from simple arithmetic to complex logical operations.
This guide will walk you through the different types of operators in Java, from basic arithmetic to advanced logical operators. We’ll cover everything from the basics of Java operators to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering Java operators!
TL;DR: What is an Operator in Java?
An operator in Java is a special symbol that is used to perform operations. For example,
+
is an arithmetic operator that adds two numbers.
Here’s a simple example:
int sum = 5 + 10;
// Output:
// 15
In this example, we’ve used the ‘+’ operator to add two numbers, 5 and 10, resulting in the output ’15’.
But Java’s operator capabilities go far beyond this. Continue reading for more detailed examples and advanced operator techniques.
Table of Contents
- Understanding Basic Arithmetic Operators in Java
- Diving Deeper: Relational, Logical, and Bitwise Operators in Java
- Exploring Alternative Approaches to Java Operations
- Navigating Common Issues with Java Operators
- The Concept of Operators in Programming
- The Importance of Operators in Java
- The Relevance of Java Operators in Complex Applications
- Wrapping Up: Mastering Java Operators
Understanding Basic Arithmetic Operators in Java
Java provides several basic arithmetic operators that allow us to perform simple mathematical operations. Let’s explore each one of them:
- Addition (+): This operator adds two numbers.
- Subtraction (-): This operator subtracts one number from another.
- Multiplication (*): This operator multiplies two numbers.
- Division (/): This operator divides one number by another.
- Modulus (%): This operator returns the remainder of a division operation.
Let’s look at an example of how these operators work in Java:
int a = 10;
int b = 5;
int sum = a + b; // Addition
int diff = a - b; // Subtraction
int product = a * b; // Multiplication
int quotient = a / b; // Division
int remainder = a % b; // Modulus
// Output:
// sum: 15
// diff: 5
// product: 50
// quotient: 2
// remainder: 0
In this example, we’ve performed various arithmetic operations using Java operators. The ‘+’ operator adds a
and b
, the ‘-‘ operator subtracts b
from a
, the ‘*’ operator multiplies a
and b
, the ‘/’ operator divides a
by b
, and the ‘%’ operator returns the remainder of the division of a
by b
.
While these operators are simple to use, they can be powerful tools in your Java programming toolkit. However, it’s important to be aware of potential pitfalls. For instance, the division operator ‘/’ performs integer division if both operands are integers. This means it will not return a floating-point number, even if the mathematical result of the division is not a whole number. To get a floating-point result, at least one of the operands must be a floating-point number.
Diving Deeper: Relational, Logical, and Bitwise Operators in Java
As you progress in your Java journey, you’ll encounter more complex operators that can add depth to your code. These include relational, logical, and bitwise operators.
Relational Operators
Relational operators in Java are used to compare two values. Here are the main ones:
- Equal to (
==
) - Not equal to (!=)
- Greater than (>)
- Less than (=)
- Less than or equal to (<=)
Let’s see these operators in action:
int a = 10;
int b = 5;
boolean isEqual = a == b; // Equal to
boolean isNotEqual = a != b; // Not equal to
boolean isGreater = a > b; // Greater than
boolean isLess = a < b; // Less than
boolean isGreaterOrEqual = a >= b; // Greater than or equal to
boolean isLessOrEqual = a <= b; // Less than or equal to
// Output:
// isEqual: false
// isNotEqual: true
// isGreater: true
// isLess: false
// isGreaterOrEqual: true
// isLessOrEqual: false
In the above example, we’ve compared a
and b
using various relational operators. The result of each operation is a boolean value that indicates whether the comparison is true or false.
Logical Operators
Logical operators in Java are used to combine two or more conditions. Java offers three logical operators:
- Logical AND (&&)
- Logical OR (||)
- Logical NOT (!)
Here’s an example of how these operators work:
boolean isAdult = true;
boolean hasLicense = true;
boolean canDrive = isAdult && hasLicense; // Logical AND
boolean eitherCondition = isAdult || hasLicense; // Logical OR
boolean reverseCondition = !isAdult; // Logical NOT
// Output:
// canDrive: true
// eitherCondition: true
// reverseCondition: false
In this example, canDrive
is true
because both isAdult
and hasLicense
are true
. The eitherCondition
is true
because at least one of isAdult
or hasLicense
is true
. The reverseCondition
is false
because it’s the opposite of isAdult
, which is true
.
Bitwise Operators
Bitwise operators in Java operate on bits and perform bit-level operations. The following are the bitwise operators:
- Bitwise AND (&)
- Bitwise OR (|)
- Bitwise XOR (^)
- Bitwise Complement (~)
- Left shift (<>)
- Zero fill right shift (>>>)
Here’s an example of bitwise operators:
int a = 5; // Binary: 0101
int b = 3; // Binary: 0011
int and = a & b; // Bitwise AND
int or = a | b; // Bitwise OR
int xor = a ^ b; // Bitwise XOR
int not = ~a; // Bitwise Complement
int leftShift = a <> 1; // Right shift
int zeroFillRightShift = a >>> 1; // Zero fill right shift
// Output:
// and: 1
// or: 7
// xor: 6
// not: -6
// leftShift: 10
// rightShift: 2
// zeroFillRightShift: 2
In this example, we’ve performed various bitwise operations on a
and b
. The result of each operation is an integer that represents the result of the bit-level operation.
Understanding these advanced operators can significantly enhance your Java skills. However, it’s essential to use them wisely and understand their implications to avoid potential pitfalls.
Exploring Alternative Approaches to Java Operations
While operators are a fundamental part of Java, there are alternative approaches to perform operations that can offer more flexibility or efficiency, especially in complex applications. These alternatives include using methods instead of operators and leveraging third-party libraries.
Using Methods Instead of Operators
Java provides built-in methods that can replace some operators. These methods can offer advantages like improved readability and increased functionality. Let’s consider an example where we replace the ‘+’ operator with the Math.addExact()
method:
int a = 5;
int b = 10;
// Using '+' operator
int sum1 = a + b;
// Using 'Math.addExact()' method
int sum2 = Math.addExact(a, b);
// Output:
// sum1: 15
// sum2: 15
In this example, both the ‘+’ operator and the Math.addExact()
method produce the same result. However, Math.addExact()
throws an exception if the result overflows an int, providing an additional layer of safety.
Leveraging Third-Party Libraries
Third-party libraries can offer powerful tools that go beyond Java’s built-in operators and methods. For instance, the Apache Commons Math library provides a host of mathematical and statistical functions that can simplify complex operations.
Here’s an example of using the ArithmeticUtils.addAndCheck()
method from the Apache Commons Math library, which adds two integers and checks for overflow:
import org.apache.commons.math3.util.ArithmeticUtils;
int a = 5;
int b = 10;
// Using Apache Commons Math
int sum = ArithmeticUtils.addAndCheck(a, b);
// Output:
// sum: 15
In this example, ArithmeticUtils.addAndCheck()
adds a
and b
and throws an exception if the result overflows an int. This method provides a similar functionality to Math.addExact()
, but within a library that offers many more mathematical and statistical tools.
While these alternative approaches can be beneficial, they also come with trade-offs. Using methods instead of operators can make your code more verbose, and third-party libraries introduce additional dependencies to your project. Therefore, it’s crucial to choose the approach that best fits your specific needs and constraints.
While Java operators are powerful tools, they can sometimes lead to unexpected results. Let’s discuss some common issues you might encounter when using operators in Java, and how to navigate them.
Type Compatibility Issues
Java is a statically-typed language, meaning that variables must be declared before they can be used, and their type can’t change. This can lead to issues when performing operations between incompatible types.
Consider the following example:
int a = 5;
double b = 10.5;
int result = a + b; // This line will cause a compile error
// Output:
// error: incompatible types: possible lossy conversion from double to int
In this case, the ‘+’ operator tries to add an integer (a
) and a double (b
), resulting in a double. However, we’re trying to assign the result to an integer variable (result
), which causes a compile error.
The solution is to use a variable of a compatible type to store the result:
int a = 5;
double b = 10.5;
double result = a + b; // This line will now compile successfully
// Output:
// result: 15.5
In this corrected example, we’re using a double variable (result
) to store the result of the addition, which is also a double.
Operator Precedence Issues
Java operators have a specific order of precedence, which determines the order in which they’re evaluated. For example, multiplication and division operators have higher precedence than addition and subtraction operators.
Consider the following example:
int result = 1 + 2 * 3; // The multiplication will be performed first
// Output:
// result: 7
In this case, the ‘*’ operator has higher precedence than the ‘+’ operator, so 2 * 3
is evaluated first, resulting in 1 + 6
, and thus result
is 7
.
If you want to change the order of operations, you can use parentheses:
int result = (1 + 2) * 3; // The addition will be performed first due to the parentheses
// Output:
// result: 9
In this corrected example, 1 + 2
is evaluated first due to the parentheses, resulting in 3 * 3
, and thus result
is 9
.
Understanding these common issues can help you avoid pitfalls and write effective and error-free code with Java operators.
The Concept of Operators in Programming
Operators are fundamental to any programming language, not just Java. They are special symbols that tell the compiler or interpreter to perform specific mathematical, relational, or logical operations.
Consider this simple arithmetic operation:
int a = 5;
int b = 10;
int sum = a + b;
// Output:
// sum: 15
In this example, ‘+’ is an operator that instructs Java to add a
and b
. The result is then stored in the sum
variable.
The Importance of Operators in Java
Operators play a crucial role in Java, contributing to the language’s efficiency and flexibility. They allow developers to manipulate data and make decisions based on conditions, making Java a powerful tool for creating complex applications.
For instance, logical operators can be used to create complex conditional statements:
int a = 5;
int b = 10;
if (a < b && a > 0) {
System.out.println("a is less than b and greater than 0");
}
// Output:
// "a is less than b and greater than 0"
In this example, we’re using the ‘&&’ (logical AND) operator to create a compound condition. The message is only printed if both conditions are true: a
is less than b
and a
is greater than 0.
This ability to combine and manipulate data and conditions is what makes operators such a vital part of Java.
The Relevance of Java Operators in Complex Applications
Java operators are not just for simple mathematical calculations or basic data manipulation. They play a significant role in complex Java applications, contributing to the development of efficient and robust software. From managing data flow with logical operators to manipulating binary data with bitwise operators, Java operators are indispensable.
Exploring Related Concepts
To fully leverage the power of Java operators, it’s beneficial to understand related concepts, such as control statements and loops.
Control statements like if
, else if
, and else
often work in tandem with relational operators to create conditions that guide the flow of your program. Similarly, loops like for
, while
, and do-while
frequently utilize arithmetic operators to control the number of iterations.
Here’s an example that combines control statements, loops, and operators:
for (int i = 0; i < 10; i++) {
if (i % 2 == 0) {
System.out.println(i + " is even");
} else {
System.out.println(i + " is odd");
}
}
// Output:
// "0 is even"
// "1 is odd"
// "2 is even"
// "3 is odd"
// "4 is even"
// "5 is odd"
// "6 is even"
// "7 is odd"
// "8 is even"
// "9 is odd"
In this example, we’re using a for
loop to iterate over numbers from 0 to 9. Inside the loop, we’re using an if
statement with the modulus operator ‘%’ to check if the current number is even or odd, and then printing the result.
Further Resources for Mastering Java Operators
To deepen your understanding of Java operators and related concepts, consider exploring these resources:
- Exploring Java Syntax – Explore the syntax conventions in Java, including statements, expressions, and declarations.
Java += Operator Usage – Explore examples and usage scenarios of the “+=” operator in Java programming.
Order of Operations in Java – Understand the rules governing the order of operations in Java expressions.
Oracle’s Java Operators Guide covers all aspects of the language, including operators, in detail.
GeeksforGeeks’ Java Operators provides a deep dive into Java operators, complete with examples and explanations.
W3Schools’ Java Operators offers a practical, hands-on approach to learning Java operators.
Wrapping Up: Mastering Java Operators
In this comprehensive guide, we’ve delved into the world of Java operators, exploring their usage, types, and the common issues that can arise when using them. We’ve also discussed alternative approaches to handle operations in Java, providing you with a well-rounded understanding of Java operators.
We began with the basics, exploring the arithmetic operators in Java, such as addition (+), subtraction (-), multiplication (*), division (/), and modulus (%). We then ventured into more advanced territory, looking at relational, logical, and bitwise operators, and how they can be used to add depth to your Java code.
Along the way, we tackled common challenges that you might encounter when using Java operators, such as type compatibility and operator precedence issues, providing solutions and workarounds for each issue. We also explored alternative approaches to Java operations, such as using methods instead of operators and leveraging third-party libraries, giving you a broader perspective on Java programming.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantages | Disadvantages |
---|---|---|
Using Operators | Straightforward, efficient | Can lead to type compatibility and precedence issues |
Using Methods | Improved readability, increased functionality | Can make code more verbose |
Using Third-Party Libraries | Offers powerful tools, can simplify complex operations | Introduces additional dependencies |
Whether you’re just starting out with Java, or you’re an experienced developer looking to brush up on your skills, we hope this guide has deepened your understanding of Java operators and their alternatives. Armed with this knowledge, you’re well-equipped to write efficient and robust Java code. Happy coding!