Understanding the Java += (Addition Assignment) Operator
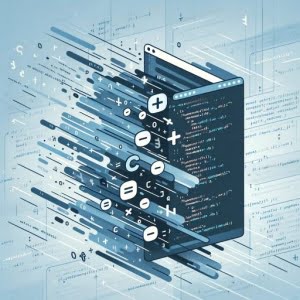
Stumped by the ‘+=’ operator in Java? You’re not alone. Many developers find this operator a bit puzzling, but it’s actually a handy tool that can simplify your code and make your programming tasks easier.
Think of the ‘+=’ operator in Java as a mathematical shortcut – a bridge that connects your variables and values in a more efficient way. It’s a powerful tool that can streamline your code, making it more readable and maintainable.
In this guide, we’ll walk you through the process of understanding and using the ‘+=’ operator in Java, from the basics to more advanced techniques. We’ll cover everything from simple assignments and calculations to its use with strings and arrays, and even discuss alternative approaches.
So, let’s dive in and start mastering the ‘+=’ operator in Java!
TL;DR: What Does ‘+=’ Mean in Java?
In Java, the ‘+=’ represents the additional assignment operator and is used to add the right operand to the left operand and assign the result back to the left operand, with the syntax,
operandA += operandB
. It’s a shorthand for a common operation that can make your code more concise and easier to read.
Here’s a simple example:
int a = 5;
a += 3;
System.out.println(a);
// Output:
// 8
In this example, we declare an integer a
and assign it a value of 5. Then, we use the ‘+=’ operator to add 3 to a
and assign the result back to a
. When we print out a
, the output is 8.
This is just a basic use of the ‘+=’ operator in Java, but there’s much more to learn about this operator and how it can simplify your code. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
The Basics of ‘+=’ in Java
The ‘+=’ operator in Java is a compound assignment operator. It’s a shorthand that combines the addition and assignment operations into a single operation. This operator adds the right operand to the left operand and then assigns the result back to the left operand.
Let’s look at a simple example:
int b = 10;
b += 5;
System.out.println(b);
// Output:
// 15
In this example, we declare an integer b
and assign it a value of 10. Then, we use the ‘+=’ operator to add 5 to b
and assign the result back to b
. When we print out b
, the output is 15.
Advantages of Using ‘+=’
One of the main advantages of using the ‘+=’ operator is that it can make your code more concise and easier to read. Instead of writing b = b + 5;
, you can simply write b += 5;
.
Pitfalls to Avoid
While the ‘+=’ operator can simplify your code, it’s important to be aware of potential pitfalls. For example, if you use the ‘+=’ operator with a null value, you’ll get a NullPointerException. It’s also important to remember that the ‘+=’ operator performs an implicit cast, which can lead to unexpected results if you’re not careful.
Here’s an example:
byte b = 10;
b += 130;
System.out.println(b);
// Output:
// -116
In this example, the ‘+=’ operator performs an implicit cast from int (the type of the right operand) to byte (the type of the left operand). The result is -116, which might not be what you expected.
Advanced Uses of ‘+=’ in Java
While the ‘+=’ operator is commonly used with numeric types, it can also be used with other types in Java, such as strings and arrays. This versatility can lead to more complex and interesting use cases.
‘+=’ with Strings
In Java, the ‘+=’ operator can be used to concatenate strings. Here’s an example:
String s = "Java";
s += " programming";
System.out.println(s);
// Output:
// Java programming
In this example, we declare a string s
and assign it a value of “Java”. Then, we use the ‘+=’ operator to append ” programming” to s
. When we print out s
, the output is “Java programming”.
‘+=’ with Arrays
The ‘+=’ operator can also be used with arrays in Java. However, it’s important to note that this usage is not as straightforward as with numeric types or strings. Here’s an example:
int[] array = {1, 2, 3};
for (int i = 0; i < array.length; i++) {
array[i] += 5;
}
for (int num : array) {
System.out.println(num);
}
// Output:
// 6
// 7
// 8
In this example, we declare an array and use a for loop to iterate through each element. We use the ‘+=’ operator to add 5 to each element in the array. When we print out the elements of the array, the output is 6, 7, 8.
These advanced uses of the ‘+=’ operator can help you write more efficient and concise code in Java, especially when working with strings and arrays.
Exploring Alternatives to ‘+=’ in Java
While the ‘+=’ operator is a powerful tool in Java, there are other related operators that you can use depending on the specific needs of your code. Understanding these alternatives can give you more flexibility and control over your code.
The ‘+’ Operator
The ‘+’ operator in Java is the most basic form of addition. It adds the right operand to the left operand.
int c = 5;
c = c + 3;
System.out.println(c);
// Output:
// 8
In this example, we’re doing the same thing as c += 3;
, but we’re writing it out in a longer form. The ‘+’ operator is straightforward and easy to understand, but it can make your code more verbose.
The ‘++’ Operator
The ‘++’ operator in Java is an increment operator. It increases the value of the variable by 1.
int d = 5;
d++;
System.out.println(d);
// Output:
// 6
In this example, we’re increasing the value of d
by 1. The ‘++’ operator is a concise way to increment a variable, but it’s limited to increasing the value by 1.
Decision-Making Considerations
When deciding which operator to use, consider the needs of your code and the readability of your code. The ‘+=’ operator can make your code more concise, but it might be less clear to someone who isn’t familiar with this operator. The ‘+’ and ‘++’ operators are more explicit, but they can make your code more verbose.
Understanding these alternatives to the ‘+=’ operator can help you write more efficient and readable code in Java.
Troubleshooting Java ‘+=’ Operator Issues
While the ‘+=’ operator in Java is a powerful tool, it’s not without its potential pitfalls. Understanding these common issues and how to address them can save you time and frustration.
Dealing with Null Values
One common issue is attempting to use the ‘+=’ operator with a null value. This will result in a NullPointerException.
String s = null;
s += " programming";
System.out.println(s);
// Output:
// Exception in thread "main" java.lang.NullPointerException
In this example, we attempt to use the ‘+=’ operator to append ” programming” to a null string. This results in a NullPointerException. To avoid this, always ensure that your variables are initialized before using them with the ‘+=’ operator.
Implicit Casting
Another common issue is the implicit casting that occurs when using the ‘+=’ operator. This can lead to unexpected results.
byte b = 10;
b += 130;
System.out.println(b);
// Output:
// -116
In this example, the ‘+=’ operator performs an implicit cast from int (the type of the right operand) to byte (the type of the left operand), resulting in an unexpected value. To avoid this, be mindful of the types of your variables and the potential for implicit casting.
Optimization Tips
While the ‘+=’ operator can make your code more concise, it’s not always the most efficient choice. For example, if you’re performing a large number of additions, it might be more efficient to use a StringBuilder when working with strings, or to use a loop or a built-in method when working with arrays.
Understanding these common issues and best practices can help you use the ‘+=’ operator more effectively in Java.
Digging into Java Operators
Operators in Java are special symbols that perform specific operations on one, two, or three operands, and then return a result. They are the building blocks of any Java program, allowing us to perform calculations, manipulate bits, compare values, and more.
The Role of Operators in Java
Operators play a pivotal role in Java programming. They allow us to perform basic mathematical operations like addition (+
), subtraction (-
), multiplication (*
), and division (/
). They also let us compare values and determine logic (==
, !=
, >
, <
, &&
, ||
), manipulate bits (<>
, &
, |
), and more.
The Importance of ‘+=’ Operator
Among these operators, ‘+=’ holds a special place due to its dual functionality. It’s a compound assignment operator that performs both addition and assignment in a single step. This not only makes our code more concise but also can lead to performance improvements in certain situations.
int x = 10;
x += 5; // Equivalent to x = x + 5;
System.out.println(x);
// Output:
// 15
In this example, we use the ‘+=’ operator to add 5 to x
and assign the result back to x
in a single step. This is more efficient than performing the addition and assignment in two separate steps.
The ‘+=’ Operator in the Broader Context of Java
The ‘+=’ operator is part of a family of compound assignment operators in Java, which also includes ‘-=’, ‘*=’, ‘/=’, and more. These operators all combine an arithmetic operation with an assignment, making our code more concise and potentially more efficient.
Understanding the ‘+=’ operator and its role in Java programming is key to mastering Java and writing efficient, readable code.
Applying ‘+=’ Operator in Larger Java Projects
The ‘+=’ operator is not just for small programs or quick scripts. It can be a valuable tool in larger Java projects, where code efficiency and readability become critical.
In large codebases, the ‘+=’ operator can help simplify complex calculations and assignments, making the code easier to understand and maintain. It can also reduce the chance of errors, as it reduces the need to repeat variable names.
Related Topics to Explore
The ‘+=’ operator often accompanies several related topics in typical use cases. Understanding these related concepts can provide a more holistic view of Java programming. These include other compound assignment operators like ‘-=’, ‘*=’, ‘/=’, and ‘%=’, as well as the broader topic of operator precedence in Java.
Further Resources for Mastering Java Operators
To further your understanding of the ‘+=’ operator and related concepts, here are some resources that offer more in-depth information:
- Java Operator Tutorial: Getting Started – Discover Java’s bitwise operators for manipulating binary data.
.equals Method in Java – Learn to compare the contents of objects for equality with the “.equals()” method in Java.
Exploring ! Operator Usage – Understand how the “!” operator performs logical negation, flipping true to false and vice versa.
Java Operators: Oracle Docs provides a comprehensive overview of all operators in Java, including the ‘+=’ operator.
Java Compound Assignment Operators discusses the usage of compound assignment operators in Java.
Java Operator Precedence explains operator precedence in Java, which determines how expressions involving the ‘+=’ operator are evaluated.
Wrapping Up: Additional Assignment ‘+=’ Operator
In this comprehensive guide, we’ve demystified the ‘+=’ operator in Java, a handy tool that can simplify your code and streamline your programming tasks.
We began with the basics, examining how the ‘+=’ operator functions as a mathematical shortcut in Java. We then delved into more advanced usage scenarios, such as using the ‘+=’ operator with strings and arrays. We also explored alternative approaches and related operators in Java, giving you a broader understanding of the Java operator landscape.
Throughout our exploration, we tackled common issues and pitfalls associated with the ‘+=’ operator, such as dealing with null values and understanding implicit casting. We provided solutions and best practices to help you overcome these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Operator | Use Case | Pros | Cons |
---|---|---|---|
+= | Addition and assignment | Simplifies code | Can lead to unexpected results with implicit casting |
+ | Addition | Easy to understand | More verbose |
++ | Incrementing by 1 | Concise for incrementing | Limited to increasing by 1 |
Whether you’re a beginner just starting out with Java or an experienced developer looking to brush up on your skills, we hope this guide has shed light on the ‘+=’ operator in Java and its usage.
The ‘+=’ operator is a powerful tool in your Java toolkit, offering a combination of simplicity, efficiency, and versatility. Now, you’re well equipped to use it in your Java projects. Happy coding!