Java .equals() Method: A Detailed Tutorial
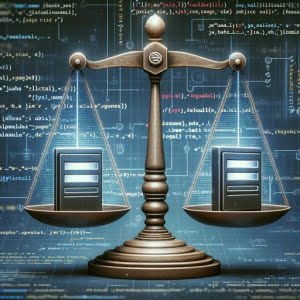
Ever found yourself puzzled over how to compare objects in Java? You’re not alone. Many developers find themselves in a maze when it comes to object comparison in Java, but we’re here to help.
Think of the .equals() method in Java as a detective – it helps you uncover whether two objects are identical or not. It’s a crucial tool in your Java toolkit, especially when dealing with object-oriented programming.
In this guide, we’ll walk you through the process of using .equals() in Java, from the basics to more advanced usage. We’ll cover everything from simple object comparison to dealing with custom objects and even troubleshooting common issues.
So, let’s dive in and start mastering the .equals() method in Java!
TL;DR: How Do I Use .equals() in Java?
The .equals() method in Java is used to compare two objects for equality, for example:
System.out.println(str1.equals(str2));
. It’s a powerful tool for checking if two objects hold the same value.
Here’s a simple example:
String str1 = new String('Hello');
String str2 = new String('Goodbye');
System.out.println(str1.equals(str2));
# Output:
# false
In this example, we’ve created two new String objects, str1
and str2
, both holding different values. When we use the .equals() method to compare str1
and str2
, it returns false
, indicating that the two objects are not equal in value.
This is just a basic way to use the .equals() method in Java, but there’s much more to learn about object comparison in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding .equals() in Java: The Basics
- Delving Deeper: .equals() with Custom Objects
- Exploring Alternatives: '==' Operator and ‘compareTo()’ Method
- Navigating Common Pitfalls with .equals()
- Unraveling Object Equality in Java
- The Bigger Picture: .equals() in Data Structures and Collections
- Exploring Related Concepts
- Wrapping Up: Mastering .equals() in Java
Understanding .equals() in Java: The Basics
In Java, the .equals() method is primarily used to compare the ‘value’ of two objects. It’s an instance method that’s part of the Object class, which is the parent class of all classes in Java. This means you can use .equals() to compare any two objects in Java.
Let’s look at a simple example to understand how .equals() works:
String str1 = new String('Hello');
String str2 = new String('Hello');
System.out.println(str1.equals(str2));
# Output:
# true
In this example, we’re creating two new String objects, str1
and str2
. Both of these objects hold the same value, which is ‘Hello’. When we use the .equals() method to compare str1
and str2
, it returns true
. This indicates that the two objects are equal in value, even though they’re two distinct objects in memory.
Advantages and Potential Pitfalls
The primary advantage of the .equals() method is its ability to compare the ‘value’ of two objects, rather than their ‘reference’. This is particularly useful when you’re dealing with objects like Strings, where you’re more interested in comparing their textual value rather than whether they refer to the same object in memory.
However, a potential pitfall of .equals() is that its behavior can vary depending on the class of the objects you’re comparing. For instance, when comparing two custom objects, the .equals() method might not work as expected, unless you override it in your class definition. We’ll cover more about this in the ‘Advanced Use’ section.
Remember, when using the .equals() method, it’s crucial to understand what you’re comparing and how the method behaves with different types of objects. This understanding will help you avoid common pitfalls and make the most of this powerful method.
Delving Deeper: .equals() with Custom Objects
As you progress in your Java journey, you’ll often find yourself working with custom objects. Here, the .equals() method can behave a bit differently. Let’s explore this with an example.
Consider a simple Person
class with properties name
and age
:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
}
We create two Person
objects with the same name
and age
:
Person person1 = new Person('Alice', 25);
Person person2 = new Person('Alice', 25);
System.out.println(person1.equals(person2));
# Output:
# false
Surprisingly, the output is false
! This is because the default .equals() method checks if the two references point to the exact same object, not their individual properties.
Customizing .equals() for Custom Objects
To get around this, we can override the .equals() method in our Person
class to compare the name
and age
properties:
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null || getClass() != obj.getClass())
return false;
Person person = (Person) obj;
return age == person.age && name.equals(person.name);
}
Now, if we run the same comparison:
System.out.println(person1.equals(person2));
# Output:
# true
This time, the output is true
. By overriding .equals(), we’ve customized it to compare the name
and age
properties of our Person
objects, giving us the desired result.
Remember, when working with custom objects, you’ll often need to override the .equals() method to ensure it behaves as expected. This allows you to define what ‘equality’ means for your custom objects, providing flexibility and control.
Exploring Alternatives: '=='
Operator and ‘compareTo()’ Method
While .equals() is a powerful method for object comparison in Java, it’s not the only tool at your disposal. Let’s explore two other alternatives: the '=='
operator and the ‘compareTo()’ method.
The '=='
Operator
The '=='
operator in Java is used to compare the references of two objects, not their contents. It checks if both references point to the exact same object in memory.
Let’s see it in action with a simple example:
String str1 = new String('Hello');
String str2 = new String('Hello');
System.out.println(str1 == str2);
# Output:
# false
Even though str1
and str2
contain the same text, the '=='
operator returns false
. This is because str1
and str2
are distinct objects in memory.
The ‘compareTo()’ Method
The ‘compareTo()’ method, on the other hand, is used to compare two objects based on their natural ordering. It’s part of the Comparable interface in Java.
Here’s a basic example of how ‘compareTo()’ works with String objects:
String str1 = 'Hello';
String str2 = 'Hello';
System.out.println(str1.compareTo(str2));
# Output:
# 0
The ‘compareTo()’ method returns 0
when the two strings are equal. If str1
is lexicographically greater than str2
, it returns a positive number, and if it’s less, it returns a negative number.
Choosing the Right Tool
Each of these methods has its own use cases and advantages. The '=='
operator is fast and straightforward, but it only checks reference equality. The .equals() method is more flexible and can be overridden to check value equality, but it can be tricky with custom objects. Finally, the ‘compareTo()’ method is useful for sorting objects but requires the objects to have a natural ordering.
In the end, the method you choose depends on your specific needs. Understanding how each method works will help you make an informed choice.
While the .equals() method is a powerful tool in Java, it’s not without its quirks. Let’s discuss some common issues you might encounter when using .equals() and how to navigate them.
Null Pointer Exceptions
One common issue when using .equals() is encountering a Null Pointer Exception. This happens when you try to use .equals() on a null reference. For example:
String str1 = null;
String str2 = 'Hello';
System.out.println(str1.equals(str2));
# Output:
# Exception in thread 'main' java.lang.NullPointerException
To avoid this, you can add a null check before using .equals(). Here’s how you can do it:
if (str1 != null && str1.equals(str2)) {
System.out.println('The strings are equal.');
} else {
System.out.println('The strings are not equal.');
}
Class Cast Exceptions
Another issue could be Class Cast Exceptions, which occur when you try to compare objects of different classes. For instance:
String str = 'Hello';
Integer num = 5;
System.out.println(str.equals(num));
# Output:
# false
Even though this doesn’t throw an error, it’s not a valid comparison. To avoid this, make sure the objects you’re comparing are of the same class or can be logically compared.
Remember, understanding these common issues and how to navigate them can help you use the .equals() method more effectively and avoid unnecessary headaches in your Java journey.
Unraveling Object Equality in Java
Before diving deeper into the .equals() method, it’s important to understand the concept of object equality in Java. What does it mean for two objects to be ‘equal’? In Java, there are two key concepts: ‘identity’ and ‘equality’.
Identity vs Equality
Identity (==
) checks if two references point to the exact same object in memory. On the other hand, equality (.equals()) checks if two objects are equal in value. For example:
String str1 = new String('Hello');
String str2 = new String('Hello');
System.out.println(str1 == str2);
System.out.println(str1.equals(str2));
# Output:
# false
# true
Here, str1
and str2
are identical in content, but they are not the same object in memory. Therefore, str1 == str2
is false
, but str1.equals(str2)
is true
.
Overriding .equals() and .hashCode()
In Java, it’s a common practice to override both .equals() and .hashCode() methods together. This is because these methods are often used in conjunction with each other, especially when dealing with collections.
The contract between .equals() and .hashCode() is that if two objects are equal according to the .equals() method, they must have the same hash code. This is crucial for the correct functioning of collections like HashSet and HashMap.
Here’s a simple example of overriding .equals() and .hashCode() in a Person
class:
@Override
public boolean equals(Object obj) {
// ... your equality logic here ...
}
@Override
public int hashCode() {
// ... your hash code logic here ...
}
By understanding these fundamental concepts, you’ll be better equipped to use the .equals() method effectively in your Java programming journey.
The Bigger Picture: .equals() in Data Structures and Collections
The .equals() method isn’t just for comparing simple or custom objects. It also plays a crucial role in data structures and collections in Java. Let’s explore this further.
Equality in Data Structures
Data structures like arrays, lists, or sets often rely on the .equals() method for operations like searching or sorting. For instance, the ArrayList’s contains()
method uses .equals() to check if an element exists in the list.
ArrayList<String> list = new ArrayList<>();
list.add('Hello');
System.out.println(list.contains('Hello'));
# Output:
# true
Equality in Collections
Similarly, in collections like HashSet or HashMap, the .equals() method is used in conjunction with .hashCode() to store and retrieve elements efficiently.
HashSet<String> set = new HashSet<>();
set.add('Hello');
System.out.println(set.contains('Hello'));
# Output:
# true
Exploring Related Concepts
The .equals() method is just one piece of the puzzle. There are other related concepts like hashcode, comparators, and the Comparable interface that are worth exploring to gain a deeper understanding of object comparison in Java.
Further Resources for Mastering .equals()
To continue your journey in mastering the .equals() method and related concepts in Java, check out these resources:
- Comprehensive Guide to Java Operators – Explore Java operators for arithmetic, comparison, and logical operations.
Understanding Addition Assignment in Java – Learn how the “+=” operator works for incremental addition in Java.
Modulo Operation in Java – Learn how the modulus operator is used to solve various computational problems.
Oracle’s Java Documentation covers all aspects of Java, including the Object class and the .equals() method.
Java Equality and Relational Operators by Baeldung, on the different ways to compare objects in Java.
Java Object Equality Article by JavaWorld covers the .equals() method, hashcode, and more.
Wrapping Up: Mastering .equals() in Java
In this comprehensive guide, we’ve delved into the intricacies of the .equals() method in Java, a fundamental tool for comparing objects.
We embarked on this journey with the basics, understanding how .equals() compares the ‘value’ of objects rather than their ‘reference’. We then expanded our horizons, exploring how .equals() behaves with custom objects and the importance of overriding it in our class definitions. We also navigated through common pitfalls, such as Null Pointer Exceptions and Class Cast Exceptions, equipping you with the knowledge to troubleshoot these issues.
Along the way, we looked at alternative approaches to object comparison. We examined the '=='
operator, which checks reference equality, and the ‘compareTo()’ method, which compares objects based on their natural ordering. Here’s a quick comparison of these methods:
Method | Object Comparison | Use Case |
---|---|---|
.equals() | Value | When you want to compare the values of two objects |
'==' Operator | Reference | When you want to check if two references point to the same object |
‘compareTo()’ Method | Natural Ordering | When you want to compare objects based on their natural ordering |
Whether you’re a beginner just starting out with Java or an intermediate developer looking to solidify your understanding of object comparison, we hope this guide has shed light on the .equals() method in Java and its alternatives.
Understanding these fundamental concepts is key to mastering Java. With this knowledge, you’re now well-equipped to handle object comparison in your Java projects. Happy coding!