Uses of ‘Npm Run Dev’ | A How-To Development Guide
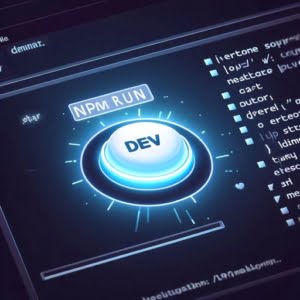
Utilizing the ‘npm run dev’ command is essential for setting up and running development environments effectively at IOFLOOD. As we utilize various in-house bare metal cloud servers for development, we’ve found that using ‘npm run dev’ is crucial for automating tasks related to startup, code compiling, and live reloading. To assist our customers and other developers with similar questions, we’ve formulated today’s article, complete with examples and tips.
This guide will walk you through using ‘npm run dev’ effectively, enhancing your development workflow in Node.js projects. By mastering this command, you’ll unlock a more efficient and streamlined development experience, allowing you to focus on crafting your application’s logic rather than wrestling with setup and configuration.
Let’s explore how ‘npm run dev’ simplifies the setup and management of development environments, allowing you to focus on building and testing your software projects
TL;DR: What Does ‘npm run dev’ Do?
npm run dev
executes thedev
script defined in your project’s package.json file, typically starting a development server with live reloading. This command is a cornerstone for Node.js developers, streamlining the process of testing and debugging applications in real-time.
Here’s a basic example:
"scripts": {
"dev": "node server.js"
}
# Output:
# Server running at http://localhost:3000
In this example, when you run npm run dev
in your terminal, it executes the dev
script specified in your project’s package.json file. This script starts a Node.js server using node server.js
. It’s a straightforward way to kickstart your development environment without manually starting your server each time.
Dive deeper into this guide for more insights on setting up and leveraging ‘npm run dev’ for your projects, including advanced configurations and troubleshooting tips.
Table of Contents
Script Setup: ‘npm run dev’
Understanding npm Scripts
In the heart of every Node.js project lies a package.json
file, a manifest that holds various metadata relevant to the project. Among its many uses, it defines scripts that can automate tasks, such as starting a development server. For beginners, setting up a ‘dev’ script is your first step towards automating your development workflow.
To define a ‘dev’ script in your package.json
, you simply add an entry under the scripts
section. This script usually starts your project’s development server. Here’s how you can set it up:
"scripts": {
"dev": "node app.js"
}
In this example, running npm run dev
in your terminal will execute the node app.js
command, starting your Node.js application. Here’s what you might see as output:
# Output:
# Listening on http://localhost:3000
This output indicates that your server is up and running, and you can now view your application by navigating to http://localhost:3000
in your browser. The beauty of using npm run dev
lies in its simplicity. With just a single command, you’ve started your development server, bypassing the need to manually execute node app.js
every time you want to run your application.
Pros and Cons for Beginners
Pros:
– Easy Setup: Just a few lines in package.json
and you’re ready to go.
– Quick Start: Jump straight into development without fiddling with commands.
Cons:
– Limited Customization: At this level, there’s minimal room for tweaking how your development environment behaves. For more advanced features like live reloading, you’ll need to explore further.
Mastering the npm run dev
command is a significant first step in optimizing your development process. It not only saves time but also introduces you to the world of npm scripts, an essential tool for any Node.js developer.
Automation Tips for ‘npm run dev’
Automatic Server Restarts
After mastering the basic use of npm run dev
, it’s time to enhance your development workflow. A common pain point in development is having to manually restart your server after making changes to your code. This is where Nodemon comes into play. Nodemon is a utility that monitors for any changes in your source and automatically restarts your server, saving you a significant amount of time and effort.
To integrate Nodemon into your workflow, you first need to install it as a development dependency:
npm install --save-dev nodemon
Next, update your ‘dev’ script in package.json
to use Nodemon instead of node:
"scripts": {
"dev": "nodemon app.js"
}
Running npm run dev
now utilizes Nodemon. Here’s what you might encounter as output when making a change:
# Output:
# [nodemon] starting `node app.js`
# [nodemon] restarting due to changes...
# [nodemon] starting `node app.js`
This output indicates that Nodemon is actively monitoring your project. Upon detecting a file change, it automatically restarts your server, ensuring that your latest changes are always in effect without the need for manual intervention.
Environment Variables Made Easy
Another aspect of advancing your ‘npm run dev’ setup involves the use of environment variables for configuring your development environment. Environment variables allow you to manage application settings outside of your code, such as database connection strings or API keys, enhancing security and flexibility.
To set up environment variables, you can use a package like dotenv
:
npm install dotenv
Then, create a .env
file in your project root and define your variables:
PORT=3000
DB_STRING=mongodb://localhost/devdb
In your application, you can access these variables using process.env
:
require('dotenv').config();
console.log(process.env.PORT); // Output: 3000
Pros and Cons
Pros:
– Increased Efficiency: Automatic restarts with Nodemon save time.
– Convenience: Environment variables streamline configuration management.
Cons:
– Additional Setup: Integrating Nodemon and environment variables requires initial setup effort.
By incorporating these advanced techniques into your ‘npm run dev’ command, you not only improve your development efficiency but also take a significant step towards a more professional and scalable Node.js project setup.
Exploring Beyond ‘npm run dev’
Embrace Docker for Consistent Environments
As your projects grow in complexity, you might seek more robust solutions to manage your development environments. Docker emerges as a powerful ally, enabling you to containerize your applications and their dependencies. This ensures that your application runs consistently across all environments.
To leverage Docker in your workflow, start by creating a Dockerfile
in your project root:
FROM node:14
WORKDIR /app
COPY . .
RUN npm install
CMD ["npm", "run", "dev"]
This Dockerfile
sets up a Node.js environment, copies your project files, installs dependencies, and specifies npm run dev
as the default command. To build and run your Docker container, execute:
docker build -t my-node-app .
docker run -p 3000:3000 my-node-app
# Output:
# > node app.js
# Listening on http://localhost:3000
This setup encapsulates your development environment within a Docker container, ensuring that it remains consistent regardless of where it’s deployed. It’s an excellent step towards achieving development-production parity.
Integrate Task Runners for Automation
Task runners like Gulp or Webpack can further automate and enhance your development process. These tools can compile your code, minify assets, and even refresh your browser automatically upon file changes, integrating seamlessly with npm run dev
.
For example, integrating Webpack might involve adding a new script in your package.json
:
"scripts": {
"dev": "webpack serve --mode development"
}
This command uses Webpack to bundle your application and serve it in development mode, offering features like hot module replacement.
Pros and Cons of Alternative Approaches
Pros:
– Consistency: Docker ensures your environment is the same everywhere.
– Automation: Task runners like Gulp and Webpack streamline repetitive tasks.
Cons:
– Complexity: Setting up Docker and integrating task runners can introduce additional complexity.
– Learning Curve: There’s a learning curve involved in mastering these tools.
By exploring these alternative approaches, you can tailor your development environment to your project’s specific needs, ensuring efficiency and consistency across all stages of development.
Overcoming ‘npm run dev’ Hurdles
Tackling ‘Script Not Found’
One of the most common issues developers encounter with ‘npm run dev’ is the dreaded ‘script not found’ error. This typically occurs when the ‘dev’ script is missing or incorrectly defined in your package.json
. To resolve this, ensure your ‘dev’ script is properly set up:
"scripts": {
"dev": "nodemon app.js"
}
After adding or correcting the ‘dev’ script, running npm run dev
should successfully start your development server. If the problem persists, double-check for typos or syntax errors in your package.json
.
Resolving Environment Variable Issues
Environment variables are crucial for configuring your application without hard-coding sensitive information. If npm run dev
isn’t recognizing your environment variables, ensure you’ve loaded them correctly using dotenv
or a similar package:
require('dotenv').config();
console.log(process.env.MY_VARIABLE); // Replace MY_VARIABLE with your actual variable name
# Output:
# Your variable value
This code block demonstrates how to load and access environment variables. If you don’t see the expected output, verify that your .env
file is in the root directory of your project and that it’s correctly formatted.
Avoiding Port Conflicts
Port conflicts occur when another process is using the port your application is trying to bind to. This is common when running multiple projects simultaneously. To avoid this, you can configure your application to use a different port if the default one is unavailable:
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3001; // Fallback to 3001 if process.env.PORT is unavailable
app.listen(PORT, () => {
console.log(`Server running on http://localhost:${PORT}`);
});
# Output:
# Server running on http://localhost:3001
In this example, we use a fallback port (3001) if the preferred port is not available. This simple strategy can help mitigate port conflicts, ensuring your development server runs smoothly.
Best Practices and Optimization
- Keep your
package.json
clean and organized: Regularly review your scripts and dependencies to avoid errors. - Use environment variables wisely: Store sensitive information in environment variables and keep your
.env
file out of version control. - Handle port conflicts gracefully: Implement fallback strategies to ensure your application always has a port to run on.
By addressing these common issues and adhering to best practices, you can optimize your development process and minimize disruptions when using ‘npm run dev’.
Npm and package.json Essentials
The Heart of Node.js Projects
Understanding npm
(Node Package Manager) is crucial for every Node.js developer. It’s not just a tool for installing packages; it’s the backbone of managing project dependencies, scripts, and more. The package.json
file, often considered the heart of a Node.js project, plays a pivotal role in this ecosystem.
At its core, package.json
serves multiple purposes:
- Dependency Management: It lists all the project dependencies, ensuring consistency across environments.
- Script Automation: It allows the definition of scripts for common tasks, such as starting the development server.
Here’s a minimal package.json
example:
{
"name": "my-app",
"version": "1.0.0",
"scripts": {
"start": "node app.js"
},
"dependencies": {
"express": "^4.17.1"
}
}
In this example, npm start
would execute node app.js
, starting the application. The dependencies section ensures that anyone working on the project uses the same version of Express.
Scripts: Beyond ‘npm run dev’
The scripts
section of package.json
is where npm run dev
comes into play. It’s a custom script, typically used to start a development server with features like hot reloading or debugging tools enabled. This is different from the start
script, which is often used in production environments.
"scripts": {
"dev": "nodemon app.js",
"start": "node app.js"
}
This setup illustrates the distinction between development (dev
) and production (start
) environments. Using nodemon
for the dev
script automates the process of restarting the server upon file changes, enhancing development efficiency.
Development vs. Production Environments
The difference between development and production environments is crucial. Development environments are configured for ease of use and debugging, often with additional tools like live reloading. Production environments, on the other hand, are optimized for performance and stability.
Understanding and utilizing the appropriate scripts and configurations for each environment is essential for a smooth workflow. This ensures that developers can work efficiently in the development phase, while the application runs optimally in production.
By grasping these fundamentals, developers can leverage npm
and package.json
to streamline their development process, making npm run dev
a powerful command in their development arsenal.
‘npm run dev’ in Complex Projects
Integrating Front-End Build Tools
As projects grow in complexity, integrating front-end build tools like Webpack or Parcel with npm run dev
becomes essential. These tools offer a plethora of features such as module bundling, asset optimization, and hot module replacement, significantly enhancing the development experience.
Consider a scenario where you integrate Webpack with your npm run dev
setup. Your package.json
might include a script like this:
"scripts": {
"dev": "webpack-dev-server --mode development"
}
This command starts the Webpack development server in development mode, providing features like live reloading and hot module replacement out of the box. Here’s a simplified output you might see:
# Output:
# Project is running at http://localhost:8080/
# webpack output is served from /dist/
This setup not only streamlines your development process but also ensures that your front-end assets are efficiently managed and served.
Related Commands and Functions
Besides npm run dev
, several related commands and functions can enhance your workflow. For instance, npm run build
is commonly used in conjunction with npm run dev
for preparing the application for production by compiling and minifying assets.
"scripts": {
"build": "webpack --mode production"
}
Executing npm run build
compiles your application with Webpack in production mode, optimizing your assets for performance. This command is crucial for preparing your application for deployment.
Further Resources for ‘npm run dev’ Mastery
To deepen your understanding and mastery of npm run dev
and its ecosystem, here are three invaluable resources:
- Visit Node.js Documentation to explore the fundamentals and advanced topics in Node.js.
Explore Webpack Concepts for a comprehensive understanding of Webpack and how it can be integrated with Node.js projects.
Learn about Parcel, a fast, zero-configuration web application bundler, and how to use it in your Node.js projects.
These resources provide a wealth of information that can help you leverage npm run dev
more effectively in your projects, ensuring a robust and efficient development workflow.
Wrapping Up: Mastering ‘npm run dev’
In this comprehensive guide, we’ve explored the vital command ‘npm run dev’, a cornerstone in the development of Node.js projects. This command initiates your development environment, setting the stage for efficient and streamlined workflow.
We began with the basics, understanding the role of package.json
and how to set up a simple ‘dev’ script to start our Node.js server. This foundational knowledge is crucial for anyone stepping into the world of Node.js development.
Moving forward, we delved into more advanced usage, integrating tools like Nodemon for automatic server restarts and employing environment variables for more dynamic configurations. These intermediate steps not only make the development process more efficient but also introduce best practices for managing application settings.
Exploring alternative approaches, we discussed the integration of Docker and the use of task runners like Gulp and Webpack. These expert-level configurations offer a glimpse into the possibilities for scaling and optimizing Node.js projects, ensuring consistency across environments and automating repetitive tasks.
Approach | Efficiency | Complexity |
---|---|---|
Basic ‘npm run dev’ | High | Low |
Nodemon Integration | Higher | Moderate |
Docker & Task Runners | Highest | High |
Whether you’re just starting out with ‘npm run dev’ or looking to enhance your current setup, we hope this guide has provided you with valuable insights and practical knowledge. The command ‘npm run dev’ is more than just a way to start your development server; it’s a gateway to optimizing your development workflow and embracing the full potential of Node.js.
With the right setup and understanding, ‘npm run dev’ can significantly reduce development time and increase productivity. Embrace these techniques, and you’ll find yourself navigating the Node.js ecosystem with greater ease and confidence. Happy coding!