Understanding ‘&&’ Java (AND) Logical Operator
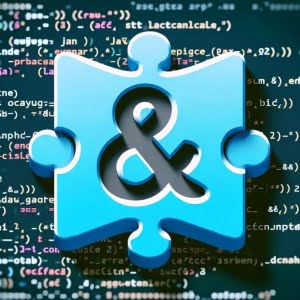
Are you finding it challenging to understand the ‘&&’ operator in Java? You’re not alone. Many developers grapple with this task, but there’s a method to the madness.
Think of the ‘&&’ operator in Java as a traffic signal – it controls the flow of your code, directing it based on certain conditions. It’s a logical AND operator that returns true if both operands are true, acting as a gatekeeper for your code execution.
This guide will explain how to use the ‘&&’ operator in Java, from basic usage to advanced techniques. We’ll explore its core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the ‘&&’ operator in Java!
TL;DR: What is the ‘&&’ Operator in Java?
The ‘&&’ operator in Java is a logical AND operator that returns true if both operands are true. It is declared with the syntax,
boolean result = (operandA) && (operandB)
It’s a fundamental part of controlling the flow of your code, allowing you to create complex logical conditions.
Here’s a simple example:
boolean result = (5 > 3) && (8 > 4);
System.out.println(result);
# Output:
# true
In this example, we have two conditions: 5 > 3
and 8 > 4
. Both of these conditions are true, so the ‘&&’ operator returns true. If either of these conditions were false, the ‘&&’ operator would return false.
This is just a basic usage of the ‘&&’ operator in Java. There’s much more to learn about using this operator effectively, especially in more complex scenarios. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
Understanding the ‘&&’ Operator in Java
The ‘&&’ operator in Java is a logical operator, specifically a logical AND operator. It’s used in conditional statements to create more complex conditions. The ‘&&’ operator will only return true if both conditions on either side of it are true.
Let’s look at a simple example:
boolean result = (2 > 1) && (3 > 2);
System.out.println(result);
# Output:
# true
In this example, the conditions 2 > 1
and 3 > 2
are both true, so the ‘&&’ operator returns true. If either of these conditions were false, the ‘&&’ operator would return false.
One of the key advantages of the ‘&&’ operator is that it allows you to create more complex conditions in your code. For example, you can use the ‘&&’ operator to check multiple conditions before executing a piece of code.
However, there are potential pitfalls when using the ‘&&’ operator. One common mistake is misunderstanding how the ‘&&’ operator works with non-boolean values. In Java, the ‘&&’ operator can only be used with boolean values, unlike some other languages where it can be used with other types of values.
Remember, the ‘&&’ operator in Java is a powerful tool in your coding toolbox, but it’s important to understand how it works to use it effectively.
Advanced Use of ‘&&’ Operator in Control Flow
As you become more comfortable with the ‘&&’ operator in Java, you can start to use it in more complex scenarios. One of the most common advanced uses of the ‘&&’ operator is in control flow statements, like ‘if’ and ‘while’ loops.
Using ‘&&’ in ‘if’ Statements
In an ‘if’ statement, the ‘&&’ operator can be used to check multiple conditions. If all conditions are true, the code block inside the ‘if’ statement will execute.
Here’s an example:
int age = 20;
boolean hasDriverLicense = true;
if (age >= 16 && hasDriverLicense) {
System.out.println("You can drive!");
} else {
System.out.println("You cannot drive.");
}
# Output:
# You can drive!
In this code, the ‘if’ statement checks two conditions: age >= 16
and hasDriverLicense
. Because both conditions are true, the message “You can drive!” is printed.
Using ‘&&’ in ‘while’ Loops
The ‘&&’ operator can also be used in ‘while’ loops to create more complex conditions. Here’s an example:
int counter = 0;
while (counter < 10 && hasDriverLicense) {
System.out.println("Counter: " + counter);
counter++;
}
# Output:
# Counter: 0
# Counter: 1
# Counter: 2
# ...
# Counter: 9
In this code, the ‘while’ loop continues as long as counter < 10
and hasDriverLicense
is true. If either condition becomes false, the loop will stop.
Understanding how to use the ‘&&’ operator in control flow statements is a key part of mastering Java. It allows you to create more complex and flexible code.
Exploring ‘||’ and ‘!’ Operators: Alternatives to ‘&&’
While ‘&&’ is a powerful tool in Java, it’s not the only operator you can use to control the flow of your code. The ‘||’ (OR) and ‘!’ (NOT) operators offer alternative ways to create complex conditions.
The ‘||’ (OR) Operator
The ‘||’ operator is a logical OR operator. It returns true if at least one of the operands is true. Here’s an example:
boolean result = (5 < 3) || (8 > 4);
System.out.println(result);
# Output:
# true
In this example, the condition 5 4
is true. Because at least one condition is true, the ‘||’ operator returns true.
The ‘||’ operator can be useful when you want a block of code to execute if at least one condition is met, rather than requiring all conditions to be true as with the ‘&&’ operator.
The ‘!’ (NOT) Operator
The ‘!’ operator is a logical NOT operator. It inverts the value of a boolean. Here’s an example:
boolean hasDriverLicense = true;
boolean cannotDrive = !hasDriverLicense;
System.out.println(cannotDrive);
# Output:
# false
In this example, the ‘!’ operator inverts the value of hasDriverLicense
, so cannotDrive
is false.
The ‘!’ operator can be useful when you want to check if a condition is not true.
While ‘&&’, ‘||’, and ‘!’ all have their uses, it’s important to choose the right tool for the job. Understanding the differences between these operators and when to use each one is a key part of mastering Java.
Troubleshooting Common Issues with ‘&&’
While the ‘&&’ operator is a powerful tool in Java, it’s not without its challenges. Let’s discuss some common issues that you might encounter when using the ‘&&’ operator and how to solve them.
Logical Errors with ‘&&’
One common issue is logical errors. These occur when your code runs without errors, but it doesn’t produce the expected result. This often happens when the conditions in your ‘&&’ operator aren’t set up correctly.
Consider this example:
boolean result = (5 > 3) && (4 > 8);
System.out.println(result);
# Output:
# false
In this example, the condition 4 > 8
is false, so the ‘&&’ operator returns false, even though 5 > 3
is true. To avoid logical errors, make sure to double-check your conditions.
Short-Circuiting with ‘&&’
Another issue to consider is short-circuiting. In Java, the ‘&&’ operator uses short-circuit evaluation, meaning it evaluates the left operand first and only evaluates the right operand if necessary.
Here’s an example:
boolean result = false && (3 > 2);
System.out.println(result);
# Output:
# false
In this example, because the left operand is false, Java doesn’t bother evaluating the right operand, since the ‘&&’ operator can only return true if both operands are true.
While short-circuiting can improve performance, it can also lead to unexpected results if you’re not aware of it. Always keep in mind that the ‘&&’ operator uses short-circuit evaluation when designing your conditions.
Understanding these common issues with the ‘&&’ operator can help you write more effective and reliable Java code.
Fundamentals of Logical Operators in Java
Before diving deeper into the ‘&&’ operator, let’s take a step back and understand the basics of logical operators in Java. Logical operators are used to create more complex conditions by combining boolean expressions.
There are three main logical operators in Java:
- ‘&&’ (Logical AND)
- ‘||’ (Logical OR)
- ‘!’ (Logical NOT)
Logical AND (‘&&’) Operator
As we’ve discussed, the ‘&&’ operator returns true if both operands are true. It’s used to create a condition that’s only true when all individual conditions are met.
Logical OR (‘||’) Operator
The ‘||’ operator returns true if at least one of the operands is true. It’s used to create a condition that’s true when any of the individual conditions are met.
Logical NOT (‘!’) Operator
The ‘!’ operator inverts the value of a boolean, turning true to false and vice versa. It’s used to create a condition that’s true when a certain condition is not met.
Understanding these fundamental concepts is crucial for mastering the ‘&&’ operator and other logical operators in Java. They form the building blocks for creating complex conditions and controlling the flow of your code.
The ‘&&’ Operator in Larger Projects and Complex Logic Building
The ‘&&’ operator in Java is not just for simple conditions. As your projects grow in size and complexity, you’ll find that the ‘&&’ operator becomes an essential tool for building complex logic.
For instance, in a large project, you might need to check multiple conditions before executing a piece of code. The ‘&&’ operator allows you to do this in a concise and readable way.
Moreover, understanding the ‘&&’ operator is a stepping stone to mastering other related concepts in Java, such as bitwise operators.
Bitwise Operators in Java
Bitwise operators are used for performing operations on bits. While they might seem intimidating at first, they are incredibly powerful and can help you solve problems more efficiently.
For example, the bitwise AND operator (&) is similar to the logical ‘&&’ operator, but it operates on the binary representations of integers. This can be useful in scenarios where you need to manipulate individual bits of an integer.
int a = 12; // Binary: 1100
int b = 10; // Binary: 1010
int result = a & b; // Binary: 1000, Decimal: 8
System.out.println(result);
# Output:
# 8
In this example, the bitwise AND operator (&) performs a binary AND operation on the binary representations of 12 and 10, resulting in 8.
Further Resources for Mastering Java Operators
To deepen your understanding of the ‘&&’ operator and related concepts in Java, here are some additional resources:
- Best Practices for Using Operators in Java – Explore Java’s equality and inequality operators for comparing objects.
Understanding Or Operator in Java – Learn about short-circuit evaluation when using the “||” operator in Java.
Exploring Operator Precedence in Java – Learn how to use parentheses to ensure proper evaluation of expressions.
Java Operators – A comprehensive tutorial from Oracle on logical and bitwise Java operators.
Java Logical Operators: A detailed guide from W3Schools on logical operators in Java.
Bitwise and Bit Shift Operators from GeeksforGeeks offers examples and exercises on bitwise operators in Java.
Wrapping Up:
In this comprehensive guide, we’ve journeyed through the world of the ‘&&’ operator in Java, a fundamental tool for controlling the flow of your code.
We began with the basics, understanding what the ‘&&’ operator is and how to use it in simple scenarios. We then ventured into more advanced territory, using the ‘&&’ operator in control flow statements like ‘if’ and ‘while’ loops, and even exploring alternative operators like ‘||’ and ‘!’.
Along the way, we tackled common issues that you might encounter when using the ‘&&’ operator, such as logical errors and short-circuiting, providing you with solutions and workarounds for each issue.
We also compared the ‘&&’ operator with the ‘||’ and ‘!’ operators, giving you a sense of the broader landscape of logical operators in Java. Here’s a quick comparison of these operators:
Operator | Description | Use Case |
---|---|---|
‘&&’ | Returns true if both operands are true | When you want a condition to be true only if all individual conditions are met |
‘ | ‘ | Returns true if at least one operand is true | When you want a condition to be true if any individual condition is met | |
‘!’ | Inverts the value of a boolean | When you want to check if a condition is not true |
Whether you’re just starting out with Java or you’re looking to level up your coding skills, we hope this guide has given you a deeper understanding of the ‘&&’ operator and its capabilities.
With its ability to create complex conditions and control the flow of your code, the ‘&&’ operator is a powerful tool in your Java toolbox. Happy coding!