Order of Operations in Java: A Step-by-Step Guide
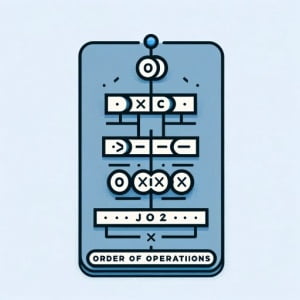
Ever felt like you’re wrestling with the order of operations in Java? You’re not alone. Many developers find Java’s order of operations a bit perplexing, but we’re here to help.
Think of Java’s order of operations as a mathematical equation – a set of rules that dictate the sequence in which operations are carried out. These rules are crucial for writing efficient and error-free code.
In this guide, we’ll walk you through the process of understanding and applying the order of operations in Java, from the basics to more advanced techniques. We’ll cover everything from operator precedence and associativity to common pitfalls and their solutions.
Let’s dive in and start mastering the order of operations in Java!
TL;DR: What is the Order of Operations in Java?
In Java, the order of operations is determined by operator precedence and associativity. Operators with higher precedence are evaluated before operators with lower precedence. If operators have the same precedence, associativity rules are used. For instance, in the expression
int result = 3 + 4 * 2;
, the multiplication operation is performed first due to its higher precedence, resulting inresult
being 11, not 14.
Here’s a simple example:
int a = 3;
int b = 4;
int c = 2;
int result = a + b * c;
System.out.println(result);
// Output:
// 11
In this example, we have three variables a
, b
, and c
with values 3, 4, and 2 respectively. The expression a + b * c
is evaluated using Java’s order of operations. The multiplication operation b * c
is performed first due to its higher precedence, resulting in 8. Then, the addition operation a + 8
is performed, resulting in 11. The result is printed to the console.
This is just a basic example of how the order of operations works in Java. But there’s much more to learn about operator precedence, associativity, and how to manipulate the order of operations for efficient coding. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Operator Precedence and Associativity in Java
- The Impact of Order of Operations on Program Outcomes
- Manipulating Java’s Order of Operations with Parentheses
- Avoiding Common Mistakes with Java’s Order of Operations
- Digging Deeper into Java’s Order of Operations
- Enhancing Code Efficiency with Java’s Order of Operations
- Exploring Related Topics
- Wrapping Up: Mastering the Order of Operations in Java
Operator Precedence and Associativity in Java
In Java, just like in mathematics, certain operations have higher precedence than others. This is known as operator precedence. When an expression involves multiple operations, Java evaluates them in the order of their precedence. For instance, multiplication and division operations have higher precedence than addition and subtraction.
Let’s take a look at a simple example:
int a = 20;
int b = 10;
int c = 2;
int result = a + b / c;
System.out.println(result);
// Output:
// 25
In this example, the division operation b / c
is performed first due to its higher precedence, resulting in 5. Then, the addition operation a + 5
is performed, resulting in 25. The result is printed to the console.
Associativity comes into play when two operators of the same precedence appear in an expression. Java uses associativity rules to determine the order in which operations of the same precedence are performed. All binary operators except for assignment operators are left-associative, meaning they are evaluated from left to right.
Here’s an example to illustrate this:
int a = 20;
int b = 10;
int c = 2;
int result = a / b * c;
System.out.println(result);
// Output:
// 4
In this example, the expression a / b * c
is evaluated from left to right due to the left associativity of the division and multiplication operators. First, the division operation a / b
is performed, resulting in 2. Then, the multiplication operation 2 * c
is performed, resulting in 4. The result is printed to the console.
Understanding operator precedence and associativity is crucial for writing correct and efficient code in Java.
The Impact of Order of Operations on Program Outcomes
The order of operations in Java isn’t just a theoretical concept. It has a direct impact on the outcome of your programs. Misunderstanding or overlooking the order of operations can lead to unexpected results and bugs that are hard to track down.
Consider the following example:
int a = 5;
int b = 2;
int c = 3;
int result = a + b * c;
System.out.println(result);
// Output:
// 11
You might expect the result to be 21 (because 5 + 2 * 3 equals 21 if evaluated from left to right), but the actual result is 11. This is because the multiplication operation b * c
is performed first due to its higher precedence, resulting in 6. Then, the addition operation a + 6
is performed, resulting in 11.
This pitfall can be avoided by using parentheses to explicitly specify the order of operations. In Java, operations in parentheses are performed first, regardless of their precedence. Here’s how you can modify the previous example to get the desired result:
int a = 5;
int b = 2;
int c = 3;
int result = (a + b) * c;
System.out.println(result);
// Output:
// 21
In this modified example, the expression (a + b) * c
is evaluated as follows: First, the operation in parentheses a + b
is performed, resulting in 7. Then, the multiplication operation 7 * c
is performed, resulting in 21. The result is printed to the console.
By understanding and applying the order of operations correctly, you can ensure your Java programs produce the expected results and avoid subtle bugs.
Manipulating Java’s Order of Operations with Parentheses
In Java, parentheses aren’t just for grouping code or enhancing readability; they’re a powerful tool for manipulating the order of operations. By using parentheses, you can override Java’s default operator precedence and associativity rules and dictate the exact order in which operations are performed.
Let’s consider a more complex example to illustrate this:
int a = 10;
int b = 20;
int c = 30;
int d = 40;
int result = a + b * c - d;
System.out.println(result);
// Output:
// 570
In this example, the multiplication operation b * c
is performed first due to its higher precedence, resulting in 600. Then, the addition operation a + 600
is performed, resulting in 610. Finally, the subtraction operation 610 - d
is performed, resulting in 570.
But what if you want to perform the addition operation first, then the multiplication, and finally the subtraction? You can achieve this by using parentheses as follows:
int a = 10;
int b = 20;
int c = 30;
int d = 40;
int result = (a + b) * c - d;
System.out.println(result);
// Output:
// 860
In this modified example, the operation in the first set of parentheses a + b
is performed first, resulting in 30. Then, the multiplication operation 30 * c
is performed, resulting in 900. Finally, the subtraction operation 900 - d
is performed, resulting in 860.
By using parentheses, you can manipulate the order of operations in Java to achieve the desired results. This technique is particularly useful in complex expressions where the default order of operations would lead to incorrect results.
Avoiding Common Mistakes with Java’s Order of Operations
Understanding the order of operations in Java is crucial, but it’s equally important to be aware of the common mistakes that developers make and how to avoid them. Let’s discuss a few of these pitfalls and their solutions.
Misunderstanding Operator Precedence
One common mistake is misunderstanding or forgetting the precedence of operators. This can lead to unexpected results. For instance, consider the following code:
int a = 10;
int b = 5;
int c = 2;
int result = a + b / c;
System.out.println(result);
// Output:
// 12
You might expect the result to be 7.5 (because 10 + 5 / 2 equals 7.5 if evaluated from left to right), but the actual result is 12. This is because the division operation b / c
is performed first due to its higher precedence, resulting in 2. Then, the addition operation a + 2
is performed, resulting in 12.
Ignoring Associativity Rules
Another common pitfall is ignoring the associativity rules when operators of the same precedence appear in an expression. Consider the following example:
int a = 20;
int b = 10;
int c = 2;
int result = a - b - c;
System.out.println(result);
// Output:
// 8
You might expect the result to be 10 (because 20 – 10 – 2 equals 10 if evaluated from right to left), but the actual result is 8. This is because the subtraction operations are evaluated from left to right due to the left associativity of the subtraction operator. First, the operation a - b
is performed, resulting in 10. Then, the operation 10 - c
is performed, resulting in 8.
By understanding and applying the order of operations correctly, you can avoid these common mistakes and write more accurate and efficient Java code.
Digging Deeper into Java’s Order of Operations
To truly master the order of operations in Java, it’s important to understand two fundamental concepts: operator precedence and associativity.
Operator Precedence in Java
Operator precedence determines the order in which operations are performed in an expression. Operators with higher precedence are evaluated before operators with lower precedence. For instance, multiplication and division have higher precedence than addition and subtraction.
Consider the following example:
int a = 10;
int b = 20;
int c = 2;
int result = a + b / c;
System.out.println(result);
// Output:
// 20
In this example, the division operation b / c
is performed first due to its higher precedence, resulting in 10. Then, the addition operation a + 10
is performed, resulting in 20.
Associativity in Java
Associativity determines the order in which operations of the same precedence are performed. All binary operators except for assignment operators are left-associative, meaning they are evaluated from left to right.
Here’s an example to illustrate this:
int a = 10;
int b = 20;
int c = 30;
int result = a - b - c;
System.out.println(result);
// Output:
// -40
In this example, the subtraction operations are evaluated from left to right due to the left associativity of the subtraction operator. First, the operation a - b
is performed, resulting in -10. Then, the operation -10 - c
is performed, resulting in -40.
Understanding operator precedence and associativity is crucial for correctly interpreting and writing Java code. By mastering these concepts, you can ensure your code behaves as expected and avoid subtle bugs.
Enhancing Code Efficiency with Java’s Order of Operations
Mastering the order of operations in Java not only helps you write correct code but also plays a crucial role in debugging and optimizing your code. When you understand the order in which operations are performed, you can better predict the behavior of your code and identify potential issues.
For instance, if a complex expression in your code is producing unexpected results, understanding the order of operations can help you break down the expression and identify the cause of the issue. Similarly, by manipulating the order of operations, you can often simplify complex expressions and make your code more efficient and readable.
Exploring Related Topics
Once you’ve mastered the order of operations in Java, there are several related topics that you might find interesting:
- Operator Overloading: While Java does not support operator overloading, many other languages do. Understanding this concept can broaden your programming skills and help you transition to other languages.
Order of Operations in Other Languages: The order of operations can vary between programming languages. Exploring how it works in languages like Python, C++, and JavaScript can provide valuable insights and make you a more versatile developer.
Further Resources for Mastering Java’s Order of Operations
To learn more about Java’s Operators and their usage, Click Here for a Complete Java Operators Guide.
And if you’re interested in diving deeper into Java’s order of operations, here are a few resources that you might find useful:
- Understanding And Operator in Java – Explore examples of the “&&” operator for combining multiple conditions in Java.
Java Expressions Overview explains expressions in Java, variables, operators, and method calls combined to produce a single value.
Java Operators provides a comprehensive overview of Java operators and their precedence.
Java Operator Precedence on JavaTpoint offers a detailed guide on Java operator precedence with examples.
Java Programming Tutorial on Programiz covers Java operators in depth and includes a handy operator precedence chart.
Wrapping Up: Mastering the Order of Operations in Java
In this comprehensive guide, we’ve journeyed through the intricate world of Java’s order of operations. We’ve explored how understanding and applying these rules can make your code more efficient and bug-free.
We started with the basics, explaining the concepts of operator precedence and associativity, and how they dictate the order of operations in Java. We then delved into more advanced topics, discussing the impact of the order of operations on program outcomes and how to manipulate it using parentheses.
Along the way, we addressed common pitfalls that developers often encounter when dealing with the order of operations, providing practical solutions to these issues. We also looked at how understanding the order of operations can aid in debugging and optimizing your code.
Here’s a quick comparison of the concepts we’ve discussed:
Concept | Importance | Common Pitfalls | Solutions |
---|---|---|---|
Operator Precedence | Determines the order of operations | Misunderstanding or forgetting the precedence of operators | Use parentheses to explicitly specify the order of operations |
Associativity | Determines the order of operations when operators have the same precedence | Ignoring the associativity rules | Understand that all binary operators except for assignment operators are left-associative |
Whether you’re a beginner just starting out with Java or an experienced developer looking to brush up on your skills, we hope this guide has deepened your understanding of the order of operations in Java.
Mastering the order of operations is a fundamental skill for writing correct and efficient code in Java. Now, you’re well equipped to tackle any challenges that come your way. Happy coding!