Mastering Lombok in Java: Streamline Your Code
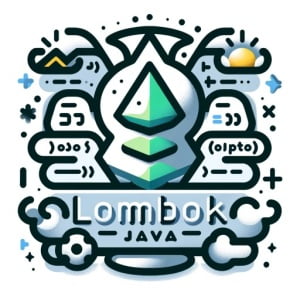
Are you constantly bogged down by the monotony of writing repetitive boilerplate code in Java? You’re not alone. Many developers find themselves tangled in this tedious task, but there’s a tool that can make this process a breeze.
Think of Lombok as a skilled artisan, capable of crafting common methods for you automatically. It’s a handy library that can significantly streamline your Java code, reducing the clutter and enhancing readability.
This guide will introduce you to Lombok, a tool that can revolutionize your Java coding experience. We’ll explore Lombok’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Lombok in Java!
TL;DR: What is Lombok in Java and How Do I Use It?
Lombok is a Java library that automatically generates boilerplate code, such as getters, setters, and constructors, using annotations such as:
@Data
,@Getter
, or@Setter
This helps to reduce the amount of repetitive code you need to write, making your code cleaner and easier to read.
Here’s a simple example:
import lombok.Data;
@Data
public class User {
private String name;
private int age;
}
In this example, we’ve used the @Data
annotation from Lombok on a User
class. This single annotation will automatically generate getters, setters, a constructor, equals()
, hashCode()
, and toString()
methods for the User
class. This saves you from having to manually write these methods, reducing the amount of boilerplate code in your project.
This is just a basic introduction to Lombok in Java, but there’s much more to learn about this powerful library. Continue reading for a more detailed guide on using Lombok, including its advanced features and common issues.
Table of Contents
- Lombok Annotations: A Beginner’s Guide
- Exploring Advanced Features of Lombok
- Alternative Methods to Reduce Boilerplate Code
- Troubleshooting Common Issues with Lombok
- Understanding Boilerplate Code in Java
- Lombok: A Solution to Boilerplate Code
- Lombok in Larger Projects: A Game Changer
- Wrapping Up: Mastering Lombok for Efficient Java Coding
Lombok Annotations: A Beginner’s Guide
Lombok provides a variety of annotations that can be used to generate boilerplate code automatically. Let’s explore some of the most commonly used ones.
@Data: The All-in-One Solution
The @Data
annotation is a convenient way to generate common methods for a class. Let’s see it in action:
@Data
public class User {
private String name;
private int age;
}
By simply adding the @Data
annotation, Lombok will generate getters, setters, a constructor, equals()
, hashCode()
, and toString()
methods for the User
class. This eliminates the need for you to write these methods manually.
@Getter and @Setter: Control Over Accessor Methods
If you only need getters and setters, Lombok has you covered with the @Getter
and @Setter
annotations. Here’s how to use them:
@Getter
@Setter
public class User {
private String name;
private int age;
}
In this example, Lombok will generate only the getter and setter methods for the User
class. This gives you more control over what methods are generated for your classes.
While Lombok’s annotations can greatly simplify your Java code, it’s important to be aware of potential pitfalls. For instance, if you have existing methods in your class that conflict with the ones Lombok generates, it could lead to unexpected behavior. As with any tool, understanding how it works and when to use it is key to leveraging its full potential.
Stay tuned for more advanced uses of Lombok in the next section.
Exploring Advanced Features of Lombok
As you become more comfortable with Lombok, you may want to explore its more advanced features. These can further enhance your code and provide additional functionality.
@Builder: Custom Constructors Made Easy
One such feature is the @Builder
annotation. This annotation allows you to implement the Builder pattern with minimal code. Here’s an example:
@Builder
public class User {
private String name;
private int age;
}
// Usage
User user = User.builder()
.name("John Doe")
.age(30)
.build();
In this example, the @Builder
annotation generates a builder for the User
class. This allows you to create instances of User
in a more readable and flexible way. The User.builder()
method returns a builder that you can use to set the properties of the User
object and then call build()
to create the object.
Handling Potential Conflicts
While Lombok can greatly simplify your code, it’s crucial to understand how it works to avoid potential conflicts. For instance, if you have an existing method in your class that has the same signature as a method generated by Lombok, the existing method will take precedence. This could lead to unexpected behavior if you’re not aware of it.
Here’s an example of a potential conflict:
@Getter
public class User {
private String name;
public String getName() {
return "Name: " + this.name;
}
}
In this example, the getName()
method in the User
class conflicts with the getter method that would be generated by the @Getter
annotation. Since the existing method takes precedence, calling getName()
on a User
object will return the string “Name: ” followed by the user’s name, rather than just the user’s name.
Understanding these nuances can help you use Lombok effectively and avoid potential pitfalls. In the next section, we’ll discuss alternative approaches to reducing boilerplate code in Java.
Alternative Methods to Reduce Boilerplate Code
While Lombok is a powerful tool for reducing boilerplate code in Java, there are other methods and libraries that you can consider. Let’s explore some of these alternatives.
Manual Method Writing
The most straightforward alternative to using Lombok is to manually write the methods. This gives you full control over the implementation and can avoid potential conflicts with existing methods.
public class User {
private String name;
private int age;
// Getter for name
public String getName() {
return this.name;
}
// Setter for name
public void setName(String name) {
this.name = name;
}
// Getter for age
public int getAge() {
return this.age;
}
// Setter for age
public void setAge(int age) {
this.age = age;
}
}
In this example, we’ve manually written the getter and setter methods for the User
class. While this gives you the most control, it can be time-consuming and tedious, especially for larger classes.
Using Other Libraries
There are also other libraries available that can help reduce boilerplate code in Java, such as Apache Commons Lang and Google’s AutoValue.
For instance, Apache Commons Lang provides a ToStringBuilder
class that can generate a toString()
method for you. Here’s how you can use it:
import org.apache.commons.lang3.builder.ToStringBuilder;
public class User {
private String name;
private int age;
@Override
public String toString() {
return new ToStringBuilder(this)
.append("name", name)
.append("age", age)
.toString();
}
}
In this example, the ToStringBuilder
class from Apache Commons Lang is used to generate a toString()
method for the User
class. This can be a useful alternative if you want more control over the toString()
method than Lombok’s @Data
annotation provides.
However, these libraries come with their own set of benefits and drawbacks. For instance, while they can provide more control than Lombok, they may also require more code and be less intuitive to use. As always, the best approach depends on your specific needs and circumstances.
Troubleshooting Common Issues with Lombok
While Lombok is a handy tool for reducing boilerplate code in Java, you may encounter some issues when using it. Let’s discuss some common problems and their solutions.
Installation Problems
One of the most common issues is problems with installing Lombok. If Lombok isn’t working as expected, you should first verify that it’s correctly installed and set up in your IDE. Here’s how you can do that in IntelliJ IDEA:
# Go to File > Settings > Plugins
# Search for 'Lombok Plugin'
# Click on 'Install' if it's not installed
# Restart IntelliJ IDEA
This will install the Lombok Plugin in IntelliJ IDEA, which is required for the IDE to recognize Lombok’s annotations.
Conflicts with Other Libraries
Lombok may also conflict with other libraries, especially those that also generate code. If you’re facing such issues, it’s recommended to check the compatibility of Lombok with the other libraries you’re using.
For instance, if you’re using both Lombok and MapStruct, you need to ensure that Lombok is executed before MapStruct during the compilation. You can do this by adding the following configuration to your pom.xml
:
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<annotationProcessorPaths>
<path>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>${lombok.version}</version>
</path>
<path>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-processor</artifactId>
<version>${mapstruct.version}</version>
</path>
</annotationProcessorPaths>
</configuration>
</plugin>
</plugins>
This ensures that Lombok’s annotation processing is done before MapStruct’s, preventing any conflicts.
As with any tool, understanding how to troubleshoot common issues can help you use Lombok more effectively. In the next section, we’ll discuss the fundamentals of boilerplate code in Java and how Lombok addresses it.
Understanding Boilerplate Code in Java
Before we delve deeper into how Lombok helps us, it’s essential to understand the problem it solves – boilerplate code.
Boilerplate code refers to the sections of code that have to be included in many places with little or no alteration. In Java, this often takes the form of getters, setters, equals()
, hashCode()
, and toString()
methods. Writing these methods manually for every class can be time-consuming and error-prone.
public class User {
private String name;
private int age;
// Getter for name
public String getName() {
return this.name;
}
// Setter for name
public void setName(String name) {
this.name = name;
}
// Getter for age
public int getAge() {
return this.age;
}
// Setter for age
public void setAge(int age) {
this.age = age;
}
}
In the example above, we’ve written the getters and setters for a simple User
class. This is boilerplate code – it’s repetitive, adds to the codebase’s size, and doesn’t contribute to the program’s functionality.
Lombok: A Solution to Boilerplate Code
This is where Lombok comes into the picture. Lombok is a library that uses annotations to automatically generate boilerplate code for you. It operates during the compilation process, meaning it doesn’t add any runtime overhead.
@Data
public class User {
private String name;
private int age;
}
In this example, by adding the @Data
annotation, Lombok automatically generates the getters, setters, equals()
, hashCode()
, and toString()
methods for the User
class. This reduces the amount of manual code, making your codebase cleaner and easier to manage.
By understanding the problem of boilerplate code and how Lombok addresses it, we can better appreciate the utility of this powerful library. In the next section, we’ll explore how Lombok can be used in larger projects and its impact on code maintainability.
Lombok in Larger Projects: A Game Changer
Lombok’s usefulness becomes even more evident when working on larger projects. As your codebase grows, the amount of boilerplate code can increase exponentially. Lombok can help manage this growth and maintain the readability and maintainability of your code.
Consider a project with dozens of classes, each with several properties. Without Lombok, you would need to write getters, setters, equals()
, hashCode()
, and toString()
methods for each class. This could result in hundreds or even thousands of lines of boilerplate code.
With Lombok, you can reduce this to a few lines of annotations, making your codebase significantly cleaner and easier to manage.
Further Resources for Mastering Lombok
If you’re interested in learning more about Lombok and how it can help you reduce boilerplate code in Java, here are some resources that you might find helpful:
- IOFlood’s Java Package Quick Guide explores best practices for naming and organizing packages in Java.
Working with Apache POI in Java – Explore Apache POI features and APIs for integration with Java applications.
Lombok Builder Annotation – Learn how Lombok’s @Builder annotation simplifies Java object creation.
Project Lombok’s Official Documentation provides a comprehensive overview of the features that Lombok offers.
Baeldung’s Guide to Lombok is a detailed guide that covers many of Lombok’s features with examples.
HowToDoInJava’s Lombok Tutorial explains how to use Lombok in Java with detailed explanations.
By utilizing Lombok in your Java projects, you can significantly reduce the amount of boilerplate code you need to write, making your code cleaner, easier to read, and easier to maintain.
Wrapping Up: Mastering Lombok for Efficient Java Coding
In this comprehensive guide, we’ve delved into the world of Lombok, a powerful library that helps reduce boilerplate code in Java. We’ve explored how to use Lombok’s annotations to automatically generate common methods, such as getters, setters, and constructors, making your Java code cleaner and easier to read.
We started with the basics, learning how to use the @Data
, @Getter
, and @Setter
annotations. We then delved into more advanced features of Lombok, such as the @Builder
annotation and how to handle potential conflicts with existing methods.
We also tackled common issues you might encounter when using Lombok, such as installation problems and conflicts with other libraries, providing you with solutions and workarounds for each issue. Additionally, we discussed alternative approaches to reducing boilerplate code in Java, comparing Lombok with manual method writing and other libraries like Apache Commons Lang.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Lombok | Reduces boilerplate code, improves code readability | Potential conflicts with existing methods |
Manual Method Writing | Full control over the implementation | Time-consuming, can lead to large codebase |
Other Libraries (e.g., Apache Commons Lang) | Provides more control than Lombok | May require more code, can be less intuitive |
Whether you’re just starting out with Lombok or looking to level up your Java coding skills, we hope this guide has given you a deeper understanding of Lombok and its capabilities.
With its ability to reduce boilerplate code, Lombok is a powerful tool for making your Java code cleaner, easier to read, and easier to maintain. Happy coding!