Lombok @Builder | Streamlining Java Object Creation
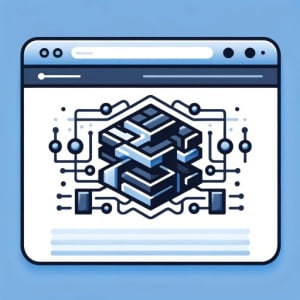
Are you finding it challenging to manage boilerplate code in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled craftsman, Lombok’s builder pattern is a handy utility that can seamlessly mold your Java code into a more elegant and readable format. This pattern can help you construct objects in a more efficient way, reducing the amount of repetitive code you need to write.
This guide will walk you through the process of using Lombok’s builder pattern, from basic usage to advanced techniques. We’ll explore Lombok builder’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the Lombok Builder!
TL;DR: How do I Create Objects with Lombok @Builder Annotation?
To use the Lombok builder annotation in Java, you need to add the
@Builder
annotation to your class. Then, you can use the generatedbuilder()
method to create objects. An example for a ‘User’ class would beUser user = User.builder().name('John')
It’s a powerful tool that can help you reduce boilerplate code and improve code readability.x
Here’s a simple example:
@Builder
public class User {
private String name;
private int age;
}
User user = User.builder().name('John').age(30).build();
# Output:
# User{name='John', age=30}
In this example, we’ve used the @Builder
annotation on the User
class. This enables us to use the builder()
method to create a new User
object. We’ve set the name
to ‘John’ and the age
to 30 using the builder pattern. The build()
method then constructs and returns the User
object.
This is a basic way to use the Lombok builder pattern in Java, but there’s much more to learn about it. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with Lombok Builder
Lombok’s @Builder
annotation is a powerful tool that can help you reduce boilerplate code in Java. It’s a popular choice among developers due to its simplicity and efficiency.
How to Use the @Builder
Annotation
To use the @Builder
annotation, you simply need to add it to your class. Lombok will then automatically generate a builder()
method for your class.
Here’s an example:
@Builder
public class Employee {
private String firstName;
private String lastName;
private int age;
}
Employee employee = Employee.builder().firstName('Alice').lastName('Smith').age(25).build();
# Output:
# Employee{firstName='Alice', lastName='Smith', age=25}
In this example, we’ve used the @Builder
annotation on the Employee
class. This allows us to use the generated builder()
method to create a new Employee
object. We’ve set the firstName
to ‘Alice’, the lastName
to ‘Smith’, and the age
to 25. The build()
method then constructs and returns the Employee
object.
Benefits and Potential Pitfalls of Lombok Builder
The main benefit of using the Lombok builder pattern is that it can significantly reduce the amount of boilerplate code you need to write. This can make your code more efficient and easier to read. However, it’s important to be aware of potential pitfalls. For example, if you’re not careful, you could accidentally create objects with missing or incorrect values. It’s also worth noting that Lombok’s @Builder
annotation may not be suitable for all use cases, and there may be situations where other design patterns are more appropriate.
Advanced Lombok Builder Techniques
While the basic use of Lombok builder can be quite straightforward, there are also more advanced techniques that can further enhance your coding efficiency. In this section, we’ll discuss two key advanced uses of the Lombok builder pattern: using it with inheritance and adding custom methods to the builder.
Lombok Builder with Inheritance
One of the powerful features of Lombok’s @Builder
annotation is its compatibility with inheritance. This means you can use it in a superclass and have the builder pattern available in its subclasses.
Here’s an example to illustrate this point:
@Builder
public class Animal {
private String name;
}
public class Dog extends Animal {
private String breed;
}
Dog dog = Dog.builder().name('Rover').breed('Labrador').build();
# Output:
# Dog{name='Rover', breed='Labrador'}
In this example, we’ve used the @Builder
annotation in the Animal
superclass. This allows us to use the builder pattern in the Dog
subclass, setting both the name
inherited from Animal
and the breed
specific to Dog
.
Adding Custom Methods to Lombok Builder
Another advanced technique is adding custom methods to the builder. This can be useful when you want to perform additional operations or checks when constructing an object.
Here’s an example of how you can add a custom method to the builder:
@Builder
public class Employee {
private String firstName;
private String lastName;
private int age;
@Builder.Default
private String department = 'Unassigned';
public EmployeeBuilder assignToDepartment(String department) {
this.department = department;
return this;
}
}
Employee employee = Employee.builder().firstName('Alice').lastName('Smith').age(25).assignToDepartment('Marketing').build();
# Output:
# Employee{firstName='Alice', lastName='Smith', age=25, department='Marketing'}
In this example, we’ve added a assignToDepartment
method to the builder of the Employee
class. This allows us to assign an employee to a department when constructing the object. Note that we’ve used the @Builder.Default
annotation to set a default value for the department
field.
These advanced techniques can make the Lombok builder pattern even more powerful and flexible, allowing you to write more efficient and readable code.
Exploring Alternative Builder Pattern Implementations
While Lombok’s builder pattern is a powerful tool for reducing boilerplate code, it’s not the only way to implement the builder pattern in Java. In this section, we’ll explore two alternative approaches: using plain Java and using other libraries.
Builder Pattern with Plain Java
Before libraries like Lombok came along, developers implemented the builder pattern manually in Java. This approach provides more control but requires more code.
Here’s an example of how you can implement the builder pattern in plain Java:
public class Employee {
private String firstName;
private String lastName;
private int age;
public static class Builder {
private String firstName;
private String lastName;
private int age;
public Builder firstName(String firstName) {
this.firstName = firstName;
return this;
}
public Builder lastName(String lastName) {
this.lastName = lastName;
return this;
}
public Builder age(int age) {
this.age = age;
return this;
}
public Employee build() {
return new Employee(this);
}
}
private Employee(Builder builder) {
this.firstName = builder.firstName;
this.lastName = builder.lastName;
this.age = builder.age;
}
}
Employee employee = new Employee.Builder().firstName('Alice').lastName('Smith').age(25).build();
# Output:
# Employee{firstName='Alice', lastName='Smith', age=25}
In this example, we’ve created a static inner Builder
class inside the Employee
class. This Builder
class has methods for setting the fields of Employee
, and a build()
method that constructs and returns an Employee
object.
Builder Pattern with Other Libraries
There are also other libraries that offer similar functionality to Lombok’s builder pattern, such as Google’s AutoValue. These libraries can provide additional features or a different approach to implementing the builder pattern.
Here’s an example of how you can use AutoValue to implement the builder pattern:
@AutoValue
public abstract class Employee {
abstract String firstName();
abstract String lastName();
abstract int age();
static Builder builder() {
return new AutoValue_Employee.Builder();
}
@AutoValue.Builder
abstract static class Builder {
abstract Builder firstName(String firstName);
abstract Builder lastName(String lastName);
abstract Builder age(int age);
abstract Employee build();
}
}
Employee employee = Employee.builder().firstName('Alice').lastName('Smith').age(25).build();
# Output:
# Employee{firstName='Alice', lastName='Smith', age=25}
In this example, we’ve used AutoValue’s @AutoValue
and @AutoValue.Builder
annotations to implement the builder pattern. The @AutoValue
annotation generates an implementation of the Employee
class, and the @AutoValue.Builder
annotation generates a builder for that implementation.
Comparing the Advantages and Disadvantages
Each of these approaches has its own advantages and disadvantages. Lombok’s builder pattern is simple and efficient, but it requires adding a dependency to your project and may not be suitable for all use cases. The plain Java approach gives you more control, but it requires more code and can be more error-prone. Other libraries like AutoValue offer additional features, but they also require adding dependencies and may have a steeper learning curve.
Ultimately, the best approach depends on your specific needs and preferences. It’s always a good idea to understand the different options available to you, so you can make an informed decision.
Troubleshooting Common Lombok Builder Issues
While Lombok’s builder pattern is a powerful tool, it’s not without its potential pitfalls. In this section, we’ll discuss some common issues you might encounter when using the Lombok builder pattern, such as dealing with null values or optional fields, and provide solutions to these problems.
Dealing with Null Values
One common issue when using the Lombok builder pattern is dealing with null values. If a field in your class is not set using the builder, it will default to null. This can potentially lead to NullPointerExceptions
.
To mitigate this, you can use Lombok’s @Builder.Default
annotation to set default values for your fields. Here’s an example:
@Builder
public class Employee {
@Builder.Default
private String firstName = 'Unknown';
@Builder.Default
private String lastName = 'Unknown';
@Builder.Default
private int age = 0;
}
Employee employee = Employee.builder().build();
# Output:
# Employee{firstName='Unknown', lastName='Unknown', age=0}
In this example, we’ve used the @Builder.Default
annotation to set default values for the fields of the Employee
class. This means that if these fields are not set using the builder, they will default to these values instead of null.
Handling Optional Fields
Another common issue is handling optional fields. In some cases, you might have fields in your class that are not always required.
You can handle this by using Java’s Optional
class in combination with the Lombok builder pattern. Here’s an example:
@Builder
public class Employee {
private String firstName;
private String lastName;
private Optional<String> middleName;
}
Employee employee = Employee.builder().firstName('Alice').lastName('Smith').middleName(Optional.of('Beth')).build();
# Output:
# Employee{firstName='Alice', lastName='Smith', middleName=Optional[Beth]}
In this example, we’ve used Java’s Optional
class for the middleName
field of the Employee
class. This means that the middleName
field is optional and can be omitted when constructing an Employee
object using the builder.
While these are some of the common issues you might encounter when using the Lombok builder pattern, there could be others depending on your specific use case. Always be sure to thoroughly test your code to ensure it behaves as expected.
The Builder Pattern: Core Concepts and Benefits
The builder pattern is a design pattern designed to provide a flexible solution to various object creation problems in object-oriented programming. The intent of the builder pattern is to isolate the construction of a complex object from its representation. By doing so, the same construction process can create different representations.
Understanding the Builder Pattern
In essence, the builder pattern allows us to write readable, understandable code to set up complex objects. It is especially useful when there are numerous properties with varying configurations.
Consider a scenario where you need to instantiate an object, and that object has numerous properties, some of which are mandatory, and others optional. The traditional way to do this would be to use a constructor. However, when an object has more than a few properties, constructor parameter lists can get out of hand and become hard to understand.
Here’s an example of a class with many constructor parameters:
public class Employee {
private String firstName;
private String lastName;
private int age;
private String department;
private String address;
private String phoneNumber;
public Employee(String firstName, String lastName, int age, String department, String address, String phoneNumber) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
this.department = department;
this.address = address;
this.phoneNumber = phoneNumber;
}
}
Employee employee = new Employee('Alice', 'Smith', 25, 'Marketing', '123 Main St', '555-555-5555');
# Output:
# Employee{firstName='Alice', lastName='Smith', age=25, department='Marketing', address='123 Main St', phoneNumber='555-555-5555'}
In this example, the Employee
class has many properties, making the constructor quite complex. It’s easy to mix up the order of the parameters, leading to bugs that can be hard to track down.
The Power of Lombok’s Builder Pattern
This is where Lombok’s builder pattern shines. It allows you to build up your object property by property, making the code more readable and less prone to errors. This is particularly beneficial when dealing with classes that have a large number of properties.
Using Lombok’s builder pattern, the previous example can be rewritten as follows:
@Builder
public class Employee {
private String firstName;
private String lastName;
private int age;
private String department;
private String address;
private String phoneNumber;
}
Employee employee = Employee.builder().firstName('Alice').lastName('Smith').age(25).department('Marketing').address('123 Main St').phoneNumber('555-555-5555').build();
# Output:
# Employee{firstName='Alice', lastName='Smith', age=25, department='Marketing', address='123 Main St', phoneNumber='555-555-5555'}
Here, we used the @Builder
annotation from Lombok to automatically generate the builder. The Employee
object is then constructed in a more readable and maintainable way.
In conclusion, Lombok’s builder pattern is a powerful tool for making your code more readable and maintainable. It allows you to construct complex objects in a way that’s easy to understand, reducing the likelihood of bugs and making your code easier to work with.
Scaling Up with Lombok Builder
The Lombok builder pattern is not just a tool for simplifying individual classes; it’s a strategy that can be employed across larger projects to enhance code quality and maintainability.
Lombok Builder in Large-Scale Projects
In large-scale projects, the number of classes and their complexity can grow significantly. Managing this complexity is one of the key challenges in such projects. The Lombok builder pattern can be a valuable tool in this regard.
By using the builder pattern, you can reduce the amount of boilerplate code in your project, making the codebase cleaner and easier to navigate. It also makes the code more readable, which is particularly beneficial when working in a team where multiple developers need to understand the code.
Impact on Code Quality
Code quality is a critical factor in the success of a project. High-quality code is easier to read, understand, and maintain, which ultimately leads to more stable and reliable software.
The Lombok builder pattern contributes to code quality by reducing boilerplate code and improving readability. It also promotes immutability, which is a key principle in writing robust and reliable code.
Exploring Other Lombok Annotations and Java Design Patterns
While the builder pattern is a powerful tool, it’s just one of many strategies you can use to improve your Java code. Lombok provides several other annotations, such as @Getter
, @Setter
, @EqualsAndHashCode
, and @AllArgsConstructor
, which can also help to reduce boilerplate code.
Furthermore, there are many other design patterns in Java that can help you write better code. Some of these include the singleton pattern, the factory pattern, and the strategy pattern. Each of these patterns has its own strengths and use cases, and understanding them can make you a more effective Java developer.
Further Resources for Mastering Lombok Builder
To continue your journey in mastering the Lombok builder pattern and other related topics, here are some resources that you might find helpful:
- Beginner’s Guide to Java Packages – Dive into the benefits of using packages for Java applications.
Understanding Lombok Annotations – Discover how to integrate Lombok into your Java code projects.
Exploring Lombok Data Features – Discover how to use Lombok @Data effectively in Java projects.
Project Lombok’s official documentation explains features provided by Lombok, including the builder pattern.
Baeldung’s guide on Lombok covers various Lombok annotations, including the builder pattern.
Java Design Patterns book covers many design patterns in Java and how to write effective Java code.
Wrapping Up: Lombok Builder Annotation
In this comprehensive guide, we’ve delved into the world of Lombok’s builder pattern, a powerful tool for reducing boilerplate code and enhancing readability in Java.
We began with the basics, exploring how to use the @Builder
annotation to create objects in a more elegant and readable way. We then moved on to more advanced usage scenarios, discussing how to use the builder pattern with inheritance and how to add custom methods to the builder. We also covered some common issues you might encounter when using the Lombok builder pattern, such as dealing with null values or optional fields, and provided solutions to these problems.
In addition to Lombok’s builder pattern, we also discussed alternative approaches to implementing the builder pattern in Java, such as using plain Java or other libraries like Google’s AutoValue. Each of these approaches has its own advantages and disadvantages, and understanding them can help you make an informed decision on which approach is best for your specific use case.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Lombok Builder | Reduces boilerplate code, enhances readability | Requires adding a dependency, may not be suitable for all use cases |
Plain Java | Provides more control | Requires more code, can be more error-prone |
Other Libraries (e.g., AutoValue) | Provides additional features | Requires adding dependencies, may have a steeper learning curve |
Whether you’re just starting out with the Lombok builder pattern or looking to deepen your understanding, we hope this guide has provided you with a comprehensive overview of how to use the Lombok builder pattern, its benefits, and its potential pitfalls. With this knowledge, you’re well-equipped to write more efficient and readable Java code. Happy coding!