Get Length of Array in Python: Guide and Examples
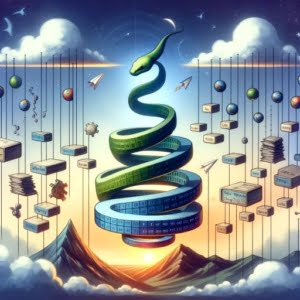
Imagine a train, with each carriage representing an element. In Python programming, this train is what we call an array. The length of the train, or the number of carriages, is analogous to the length of an array – the number of elements it contains.
In this guide, we’ll walk you through different methods to determine the length of an array in Python. We’ll also delve into the concept of arrays and how they operate within Python. So, if you’re ready to expand your Python programming skills, let’s get started!
TL;DR: How do I determine the length of an array in Python?
The simplest way to determine the length of an array in Python is by using the built-in
len()
function. Simply pass the array as an argument to the function like so:length = len(array)
. This will return the number of elements in the array. For more advanced methods and a deeper understanding, continue reading this comprehensive guide.
In Python, what would be called an array in other languages is typically implemented as a list or dictionary, which you can get the length of with len()
:
array = [1, 2, 3, 4, 5]
length = len(array)
print(length) # Outputs: 5
Table of Contents
- Grasping the Concept of Array Length
- The len() Function: A Simple Approach to Array Length Calculation in Python
- Illustrative Examples of the len() Function
- Alternative Methods for Calculating Array Length in Python
- Navigating Memory Management: The Significance of Array Length
- Understanding Arrays in Python: A Versatile Data Structure
- Further Resources for Python Arrays
- Conclusion
Grasping the Concept of Array Length
In the realm of Python programming, the length of an array refers to the total number of data elements it contains. Consider a scenario where you need to loop through an array to perform a specific operation on each element. Here, understanding the length of the array is crucial as it enables you to set up your loop correctly and prevent potential errors.
Python arrays are zero-indexed. This means that the first element is at index 0, the second at index 1, and so forth. Consequently, the index of the last item in an array is always one less than the length of the array. For instance, if you have an array of length 5, the last item’s index will be 4.
The len() Function: A Simple Approach to Array Length Calculation in Python
When it comes to calculating the length of an array in Python, the built-in len()
function is one of the simplest and most efficient tools at your disposal. The len()
function is designed to return the number of items in an object. When applied to a Python array, it returns the number of elements that the array contains.
The len()
function is remarkably easy to use. All you need to do is pass the array as an argument to the function. The following is the basic syntax for using the len()
function:
length = len(array)
In this code snippet, array
is the array whose length you’re interested in, and length
is a variable that will store the returned length.
Illustrative Examples of the len() Function
To provide a clearer understanding, let’s consider an example. Suppose we have the following array:
array = [1, 2, 3, 4, 5]
To determine the length of this array, we would use the len()
function as follows:
length = len(array)
print(length)
Running this code will output 5
, which represents the number of elements in the array.
The len()
function is a powerful and handy tool in Python. Its simplicity, straightforwardness, and efficiency make it an excellent choice for calculating array length.
Alternative Methods for Calculating Array Length in Python
While the len()
function is the most direct approach to calculating an array’s length in Python, it’s by no means the only option. Python offers an array of other methods that can be utilized to determine the length of an array. Let’s delve into some of these alternative methods.
Method | Syntax | Remarks |
---|---|---|
len() function | length = len(array) | Simplest and most efficient method |
__len__() method | length = array.__len__() | Less commonly used due to decreased readability |
For loop | count = 0 / for element in array: / count += 1 | Less efficient as it requires iterating over the entire array |
count() method | length = array.count(element) | Not recommended due to lower efficiency and readability |
Method 1: Using the __len__()
Method
Python’s objects come equipped with several built-in methods that can be used to manipulate and access the object’s data. Among these is the __len__()
method, which can be employed to ascertain the length of an array.
Here’s a demonstration of how you can use it:
array = [1, 2, 3, 4, 5]
length = array.__len__()
print(length)
Executing this code will output 5
, mirroring the len()
function.
While __len__()
accomplishes the same job as len()
, it’s less commonly employed in Python code due to its decreased readability. As such, it’s generally recommended to use len()
instead.
Method 2: Using a For Loop
An alternative method to calculate the length of an array involves using a for loop to iterate over the array and count the number of elements. Here’s an illustration:
array = [1, 2, 3, 4, 5]
count = 0
for element in array:
count += 1
print(count)
In this code, we initialize a counter variable count
to 0. Then, we use a for loop to iterate over each element in the array. For each iteration, we increment count
by 1. At the end of the loop, count
will hold the total number of elements in the array.
While this method can prove useful in certain scenarios, it’s less efficient than len()
as it necessitates iterating over the entire array. Therefore, it’s generally advisable to use len()
when you simply want to determine the length of an array.
Method 3: Using the count()
Method
The count()
method can be utilized to count the number of times a specific element appears in an array. If you know that all elements in your array are unique, you can use count()
to get the length of the array by counting the occurrence of one unique item. However, this method is not recommended due to its lower efficiency and readability compared to len()
.
While Python offers several ways to determine the length of an array, the len()
function is generally the most effective choice. Its simplicity, efficiency, and high readability make it the preferred tool for this task. However, it’s always beneficial to be aware of the other options available, should you ever need them.
In Python programming, a clear understanding of the relationship between array length and memory usage can significantly enhance your code’s efficiency and improve your system’s resource management. This section will delve into this relationship and provide you with insights on how to effectively manage memory when working with arrays in Python.
Unraveling the Link Between Array Length and Memory Usage
Every element in an array occupies a specific amount of memory. Thus, the total memory consumed by an array is directly proportional to its length. Simply put, the longer the array, the more memory it consumes.
Leveraging Array Length and Python’s itemsize Attribute to Calculate Memory Usage
Python comes with an attribute known as itemsize
that can be utilized to determine the memory usage of an array’s elements. The itemsize
attribute returns the length (in bytes) of one array item in the internal representation.
You can calculate the total memory usage of an array by combining the itemsize
attribute with the length of the array. Here’s an example of how you can do it:
import numpy as np
# Create a numpy array with 1,000,000 integers
array = np.array([i for i in range(1, 1000001)])
# Calculate the memory usage
memory_usage = array.itemsize * len(array)
print(memory_usage)
This code creates a numpy array with 1,000,000 integers and calculates the memory usage of this array. The result is the total memory usage of the array in bytes.
import numpy as np
# Create a numpy array
array = np.array([1, 2, 3, 4, 5])
# Calculate the memory usage
memory_usage = array.itemsize * len(array)
print(memory_usage)
In this code, we first import the numpy module and create a numpy array. We then calculate the memory usage by multiplying the itemsize
of the array by the length of the array. The result is the total memory usage of the array in bytes.
Practical Application: Calculating Memory Usage
To better understand this, let’s consider a practical example. Suppose you have an array of 1,000,000 integers. Given that an integer in Python occupies 4 bytes of memory, the total memory used by the array would be 4 bytes * 1,000,000 = 4,000,000 bytes
, or approximately 4 MB.
Gaining an understanding of how array length impacts memory usage can equip you with the knowledge to manage your system’s resources more effectively. For instance, if you’re dealing with large arrays and encountering memory issues, you might consider strategies to reduce the length of your arrays or employ more memory-efficient data types.
In conclusion, comprehending the length of an array goes beyond merely knowing the number of elements it contains. It also involves understanding how your code impacts your system’s memory usage. By mastering these concepts, you can develop more efficient Python code and enhance your programming skills.
Understanding Arrays in Python: A Versatile Data Structure
Arrays are among the most versatile and frequently used data structures in Python. They allow for the storage of multiple items of the same type within a single variable. This feature makes arrays incredibly useful when you need to execute operations on various items simultaneously.
The Array Module in Python
Python offers a built-in module named array
that you can utilize to create and manipulate arrays. The array
module defines an object type that can efficiently represent an array of basic values. Such values are stored in the machine’s memory as densely packed binary data, including characters, integers, and floating-point numbers.
Here’s an illustration of how you can create an array using the array
module:
import array as arr
# Create an array of integers
array = arr.array('i', [1, 2, 3, 4, 5])
In this code, we initially import the array
module. We then establish an array of integers using the array()
function. The ‘i’ argument indicates the type of the elements (in this instance, integers), and the list [1, 2, 3, 4, 5]
specifies the array’s elements.
Alternatives to Arrays: Python Lists and the NumPy Module
Although arrays are potent and flexible, Python offers other data structures that can serve as alternatives to arrays. One such alternative is Python lists.
Python lists bear similarities to arrays, but they provide more flexibility since they can accommodate items of various types. However, this flexibility comes at the expense of memory efficiency. Hence, if you’re handling large volumes of data and memory usage is a concern, you might opt to use arrays instead of lists.
Another alternative to arrays is the NumPy module. NumPy provides a robust object known as a NumPy array that is more versatile and efficient than Python’s built-in arrays and lists. NumPy arrays are particularly beneficial for scientific computing and other tasks that demand mathematical operations on large datasets.
Arrays are a fundamental component of Python programming. Whether you’re employing Python’s built-in arrays, lists, or the NumPy module, understanding how to operate with these data structures is critical for crafting effective Python code. Therefore, invest time in mastering these concepts, and you’ll be well on your journey to becoming a proficient Python programmer.
Further Resources for Python Arrays
To deepen your understanding of Python arrays, consider examining the following resources that may be immensely valuable:
- Python Data Types: A Quick Overview – Delve into Python’s set data type for handling unique elements and set operations.
Python Set: Exploring Sets in Python – Understand the concept of sets in Python and their properties for handling collections of unique elements.
Exploring Named Tuples in Python – Explore Python named tuple examples and use cases.
Python List Pop Method – Detailed guide on Python’s list pop method by GeeksforGeeks.
Real Python’s Guide on Python Append explains how to use Python’s append method.
W3Schools’ Python List Reference is a great reference for Python’s list functions.
By enriching your knowledge of Python arrays and delving into pertinent topics, you can amplify your skills and productivity as a Python developer.
Conclusion
Throughout this comprehensive guide, we’ve embarked on a journey into the world of Python arrays, with a particular emphasis on determining their length. We began by elucidating the concept of array length in Python and its significance for efficient data manipulation.
We then delved into the fundamental method of obtaining the length of an array using Python’s built-in len()
function, providing a detailed guide on its usage along with practical examples.
Our exploration didn’t end there. We also ventured into alternative methods for calculating array length, such as employing the __len__()
method, using a for loop, and the count()
method. While these methods might not be as commonly used, they offer unique approaches that could prove useful in certain scenarios.
Furthermore, we discussed the intricate relationship between array length and memory usage. By comprehending how the length of an array impacts memory usage, you can craft more efficient code and manage your system’s resources more effectively.
We concluded our discussion with a brief overview of arrays in Python, shedding light on the Array module and alternatives like Python lists and the NumPy module. These data structures form the backbone of Python programming, and understanding how to interact with them is crucial for any Python programmer.
Whether you’re a beginner or advanced coder, our Python syntax cheat sheet is an invaluable resource.
Remember, the journey to becoming a proficient programmer is paved with practice. So, don’t just read about these concepts – immerse yourself in them. Write some code, create some arrays, and calculate their length. The more you practice, the more these concepts will become second nature. Here’s to your journey in coding!