Java Static Keyword: Proper Usage Guide
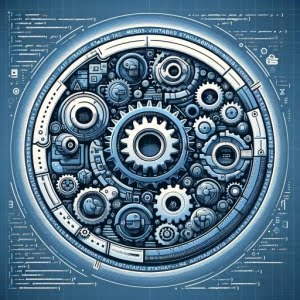
Are you puzzled by the ‘static’ keyword in Java? You’re not alone. Many developers find themselves in a similar quandary. Think of the ‘static’ keyword as a steadfast anchor, providing stability and consistency in your Java programs.
This guide will demystify ‘static’, showing you when and how to use it effectively. We’ll explore the ‘static’ keyword’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the ‘static’ keyword in Java!
TL;DR: What is the ‘static’ keyword in Java?
In Java, ‘static’ is a keyword that signifies a variable or method belongs to the class, rather than any instance of the class, such as
static int myVar = 10;
. It’s a fundamental concept in Java programming that plays a crucial role in memory management and code organization.
Here’s a simple example:
public class MyClass {
static int myVar = 10;
}
# Output:
# The variable 'myVar' is static, so it's the same across all instances of MyClass.
In this example, we declare a static variable ‘myVar’ within the class ‘MyClass’. This means ‘myVar’ is not tied to any specific instance of ‘MyClass’. Instead, it’s a class variable that remains the same across all instances of ‘MyClass’.
This is just a basic introduction to the ‘static’ keyword in Java. There’s much more to learn about its usage, benefits, and potential pitfalls. Continue reading for more detailed information and advanced usage examples.
Table of Contents
Static Keyword in Java: Basic Use for Beginners
In Java, the ‘static’ keyword is primarily used with variables and methods. When a variable is declared as static, it becomes a class variable. A class variable is common to all instances (or objects) of the class, meaning it is not tied to a specific instance.
Let’s look at a simple code example:
public class MyClass {
static int myVar = 10;
public static void main(String[] args) {
MyClass obj1 = new MyClass();
MyClass obj2 = new MyClass();
System.out.println(obj1.myVar);
System.out.println(obj2.myVar);
}
}
# Output:
# 10
# 10
In this example, we create two objects of the class ‘MyClass’, named ‘obj1’ and ‘obj2’. We then print the value of ‘myVar’ for both objects. Despite being accessed through different objects, ‘myVar’ prints the same value (10) for both. This is because ‘myVar’ is static and belongs to the class, not the individual instances.
The use of static methods is similar. A static method belongs to the class and not the instance. It can be called without creating an instance of the class.
There are several benefits to using the ‘static’ keyword. It helps in memory management as static variables are shared among all instances, reducing the amount of memory required. It also allows you to call methods without creating an instance.
However, there are potential pitfalls to consider. Static variables and methods can lead to problems if not used carefully. They can create issues with data consistency and state management, particularly in multi-threaded environments. It’s important to understand when to use static and when to use instance variables and methods, a topic we’ll delve into in the ‘Alternative Approaches’ section.
Static Java: Advanced Usage
As you gain more proficiency with the ‘static’ keyword in Java, you’ll encounter more complex uses such as static blocks and static nested classes.
Static Blocks in Java
A static block, also known as a static initialization block, is a block of code that is run when the class is loaded into memory. It’s often used to initialize static variables when their initialization requires more complex logic or error handling.
Here’s an example:
public class MyClass {
static int myVar;
// Static block
static {
myVar = 10;
System.out.println("Static block executed.");
}
public static void main(String[] args) {
System.out.println("myVar: " + myVar);
}
}
# Output:
# Static block executed.
# myVar: 10
In this example, the static block initializes ‘myVar’ and prints a message. This block is executed once, when the class is loaded, before the main method is called.
Static Nested Classes in Java
A static nested class is a static member of the outer class, similar to static variables and methods. It can access the static members of the outer class, but not its non-static members.
Here’s an example:
public class OuterClass {
static int outerVar = 10;
static class NestedClass {
void display() {
System.out.println("outerVar: " + outerVar);
}
}
public static void main(String[] args) {
OuterClass.NestedClass nested = new OuterClass.NestedClass();
nested.display();
}
}
# Output:
# outerVar: 10
In this example, the static nested class ‘NestedClass’ accesses the static variable ‘outerVar’ of the outer class.
While these advanced uses of ‘static’ provide more flexibility and control, they also come with their own challenges. Static blocks, for instance, can make code harder to read and debug if overused. Static nested classes, on the other hand, can lead to tight coupling if not used carefully. It’s important to use these features judiciously and consider alternatives when appropriate.
Exploring Alternatives to Static in Java
While the ‘static’ keyword in Java is powerful and versatile, it’s not always the best choice. There are scenarios where alternatives, like instance variables and methods, might be more appropriate.
Instance Variables and Methods
Instance variables and methods are tied to specific instances of a class. Unlike static members, they can access both static and non-static members of the class.
Here’s an example:
public class MyClass {
int instanceVar = 10; // Instance variable
void display() { // Instance method
System.out.println("instanceVar: " + instanceVar);
}
public static void main(String[] args) {
MyClass obj = new MyClass();
obj.display();
}
}
# Output:
# instanceVar: 10
In this example, ‘instanceVar’ is an instance variable and ‘display()’ is an instance method. They are accessed through an object (‘obj’) of the class.
Instance variables and methods provide more flexibility than static members as they can be manipulated independently for each object. They are essential for implementing object-oriented concepts like encapsulation and polymorphism.
However, they also consume more memory as each object gets its own copy of instance variables. They cannot be accessed directly from static context, which can sometimes limit their usability.
Making the Right Choice
Choosing between static and instance members depends on your specific needs. If you need a member to be common to all objects, use static. If you want each object to have its own copy of a member, use instance variables.
Remember, though, that overuse of static can lead to problems like memory leaks and threading issues. It can also make your code harder to test and debug. Always consider the pros and cons of each approach before making a decision.
Troubleshooting Static in Java
While the ‘static’ keyword in Java is incredibly useful, it’s not without its challenges. Two common issues related to ‘static’ are memory leaks and threading issues.
Memory Leaks with Static
Static variables live for the duration of the program, which can lead to memory leaks if not managed carefully. Here’s an example:
import java.util.ArrayList;
import java.util.List;
public class LeakExample {
static List<int[]> leakyList = new ArrayList<>();
public static void main(String[] args) {
while (true) {
leakyList.add(new int[1_000_000]);
}
}
}
# Output:
# (Program runs indefinitely, consuming more and more memory)
In this example, the program keeps adding large arrays to ‘leakyList’, consuming more and more memory. Because ‘leakyList’ is static, it doesn’t get garbage collected, leading to a memory leak.
Threading Issues with Static
Static variables are shared among all instances of a class, which can lead to data inconsistency in multi-threaded environments. Here’s an example:
public class Counter {
static int count = 0;
static void increment() {
count++;
}
public static void main(String[] args) throws InterruptedException {
Thread t1 = new Thread(Counter::increment);
Thread t2 = new Thread(Counter::increment);
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println("Count: " + count);
}
}
# Output:
# (May vary, but often less than 2)
In this example, two threads increment ‘count’ simultaneously. Because ‘count’ is static, the threads interfere with each other and ‘count’ may end up less than 2.
To prevent these issues, it’s important to use static judiciously and follow best practices. For instance, avoid using static variables to store data that changes over time or is specific to an instance. For thread safety, use synchronization or thread-safe classes from the Java Concurrency API.
Understanding Static in Programming
The term ‘static’ is a fundamental concept in many programming languages, including Java. It’s a modifier that changes the behavior of a particular element in a programming context. But what does it mean? And how does it apply to Java?
Static: A Closer Look
At its core, ‘static’ denotes something that remains constant or unchanged. In the context of programming, ‘static’ is used to denote class-level variables or methods that are shared among all instances of a class.
public class MyClass {
static int staticVar = 10;
int instanceVar = 20;
}
# Output:
# staticVar is a static variable, shared among all instances of MyClass.
# instanceVar is an instance variable, unique to each instance of MyClass.
In this example, ‘staticVar’ is a static variable, while ‘instanceVar’ is an instance variable. The difference between them lies in their scope and lifetime. The static variable ‘staticVar’ is shared among all instances of ‘MyClass’, while the instance variable ‘instanceVar’ is unique to each instance.
Class Variables vs Instance Variables
Class variables (static variables) and instance variables represent two distinct types of data in a class. Class variables are shared among all instances of a class, while instance variables are unique to each instance.
Static and Memory Management
One of the key advantages of static variables and methods is their impact on memory management. Because static variables are shared among all instances, they can help reduce the memory footprint of your program. However, they also persist for the lifetime of the program, which can lead to memory leaks if not managed carefully.
In conclusion, understanding the concept of ‘static’ in programming and how it applies to Java is crucial for effective Java programming. It affects how you design your classes, manage memory, and structure your code.
Static in Java: Beyond the Basics
The ‘static’ keyword in Java isn’t just a basic programming concept. It has wide-ranging implications that can significantly impact how you structure and manage your Java projects.
Static in Large-Scale Projects
In larger Java projects, effective use of the ‘static’ keyword can help improve memory management and code organization. However, it’s important to use ‘static’ judiciously to avoid potential issues like memory leaks and threading problems.
Delving into Related Topics
Understanding ‘static’ in Java can also pave the way for learning more advanced topics. Here are a few related concepts that you might find interesting:
- Object-Oriented Programming (OOP): Java is an object-oriented language, and understanding how ‘static’ fits into the OOP paradigm can deepen your understanding of both.
Java Memory Model: The Java Memory Model defines how Java programs interact with memory. The ‘static’ keyword plays a crucial role in this, especially in terms of memory management and garbage collection.
Garbage Collection in Java: Garbage collection is the automatic management of memory in Java. Static variables, being class-level variables, are not subject to garbage collection, which can lead to memory leaks if not managed carefully.
Further Resources for Mastering Static in Java
To better understand the role of keywords like “break,” “continue,” and “return” in Java control statements, Click Here for insights on Java Keywords.
To further your understanding of ‘static’ in Java and related topics, here are some other resources you might find helpful:
- Java Synchronized Keyword – Dive into Java synchronized keyword for achieving thread safety in concurrent programming.
Transient in Java – Understand the role of ‘transient’ keyword in Java for marking fields that should not be serialized.
Static Keyword in Java by GeeksforGeeks provides a thorough explanation of the static keyword in Java.
Understanding the static keyword in Java by Baeldung takes a detailed look at the static keyword in Java.
Oracle’s Java Tutorials covers a wide range of topics, including ‘static’, OOP, memory management, and more.
Wrapping Up:
In this comprehensive guide, we’ve journeyed through the world of the ‘static’ keyword in Java, exploring its usage, benefits, and potential pitfalls.
We began with the basics, learning how to use the ‘static’ keyword with variables and methods. We then ventured into more advanced territory, exploring complex uses of ‘static’, such as static blocks and static nested classes. Along the way, we tackled common issues you might face when using ‘static’, such as memory leaks and threading problems, providing you with solutions for each issue.
We also looked at alternative approaches to using ‘static’, discussing scenarios where instance variables and methods might be more appropriate. We provided examples and discussed the benefits, drawbacks, and decision-making considerations for each approach.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Static variables/methods | Memory efficient, shared among all instances | Can lead to memory leaks, threading issues |
Instance variables/methods | More flexible, unique to each instance | Consume more memory, cannot be accessed directly from static context |
Whether you’re just starting out with Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the ‘static’ keyword and its usage in Java.
With its balance of memory efficiency and shared access, ‘static’ is a powerful tool in Java programming. However, it’s important to use it judiciously and understand its potential pitfalls. Happy coding!