Python Union Operations: Guide (With Examples)
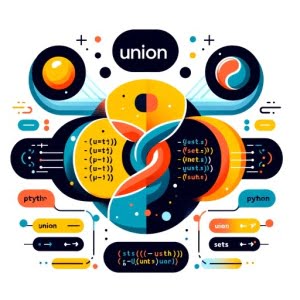
Are you finding it challenging to merge sets in Python? You’re not alone. Many developers find themselves puzzled when it comes to handling union operations in Python, but we’re here to help.
Think of Python’s union operations as a skilled diplomat – capable of uniting disparate sets into one. This provides a versatile and handy tool for various tasks, especially when dealing with data analysis and manipulation.
In this guide, we’ll walk you through the process of performing union operations in Python, from their creation, manipulation, and usage. We’ll cover everything from the basics of union operations to more advanced techniques, as well as alternative approaches.
Let’s dive in and start mastering Python union operations!
TL;DR: How Do I Perform a Union Operation in Python?
To perform a union operation in Python, you can use the
union()
method or the|
operator, such asunion_set = set1.union(set2)
. These tools allow you to merge sets, creating a new set that includes all unique elements from the original sets.
Here’s a simple example:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
print(union_set)
# Output:
# {1, 2, 3, 4, 5}
In this example, we have two sets: set1
and set2
. We use the union()
method to merge these sets into union_set
. The resulting set includes all unique elements from set1
and set2
. Notice that the number ‘3’, which appears in both sets, only appears once in the union set.
This is a basic way to perform a union operation in Python, but there’s much more to learn about manipulating sets in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Union Operations in Python: The Basics
- Python Union Operations: The Next Level
- Alternative Approaches to Python Union Operations
- Troubleshooting Python Union Operations
- Understanding Sets and Union Operations in Python
- Practical Applications and Further Learning
- Wrapping Up: Mastering Python Union Operations
Union Operations in Python: The Basics
Python provides two primary ways to perform union operations: the union()
method and the |
operator. Both methods serve the same purpose — merging sets — but they are used slightly differently.
Using the union()
Method
The union()
method is a built-in Python function that you can call on a set, passing in one or more sets that you want to merge with it.
Here’s a basic example:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
print(union_set)
# Output:
# {1, 2, 3, 4, 5}
In this code, set1.union(set2)
returns a new set that includes all unique elements from set1
and set2
. Notice that the number ‘3’, which appears in both sets, only appears once in the union set. This is because sets in Python, like in mathematical set theory, cannot have duplicate elements.
The union()
method is easy to read and understand, which makes it a good choice when you’re learning or when you want your code to be as clear as possible.
Using the |
Operator
The |
operator, also known as the pipe character, can also be used to perform union operations in Python. This operator merges two sets, similar to the union()
method.
Here’s the same operation as above, but using the |
operator instead:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1 | set2
print(union_set)
# Output:
# {1, 2, 3, 4, 5}
The |
operator is more concise than the union()
method, which can make your code cleaner and more efficient, especially in more complex operations. However, it may be slightly less readable to Python beginners or non-programmers.
In summary, both the union()
method and the |
operator can be used to perform union operations in Python. The union()
method is more explicit and readable, while the |
operator is more concise. You can choose the one that best fits your needs and coding style.
Python Union Operations: The Next Level
As you become more comfortable with Python union operations, you’ll find that they can be used in more complex ways to manipulate sets. In this section, we’ll explore how to perform union operations on more than two sets and how to use union operations in conjunction with other set operations like intersection and difference.
Union Operations with Multiple Sets
Both the union()
method and the |
operator can be used to merge more than two sets. You can simply chain them together in one line of code.
Here’s an example:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
set3 = {5, 6, 7}
union_set = set1.union(set2, set3)
print(union_set)
# Output:
# {1, 2, 3, 4, 5, 6, 7}
In this code, set1.union(set2, set3)
merges all three sets into union_set
. The resulting set includes all unique elements from set1
, set2
, and set3
.
The same operation can be performed using the |
operator:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
set3 = {5, 6, 7}
union_set = set1 | set2 | set3
print(union_set)
# Output:
# {1, 2, 3, 4, 5, 6, 7}
Using Union Operations with Other Set Operations
Python set operations are not just limited to union. There are also intersection (common elements between sets) and difference (elements present in one set but not in another) operations. You can use union operations in conjunction with these other operations to manipulate sets in more complex ways.
Here’s an example that combines union and intersection operations:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
set3 = {2, 3, 4}
union_set = set1.union(set2)
intersection_set = union_set.intersection(set3)
print(intersection_set)
# Output:
# {2, 3, 4}
In this code, we first merge set1
and set2
into union_set
using a union operation. We then find the common elements between union_set
and set3
using an intersection operation. The resulting intersection_set
includes all elements that are present in both union_set
and set3
.
These are just a few examples of the powerful ways you can use union operations in Python. With a solid understanding of these operations, you’ll be well-equipped to handle a wide range of data manipulation tasks.
Alternative Approaches to Python Union Operations
While the union()
method and the |
operator are the standard ways to perform union operations in Python, there are alternative approaches that can provide more flexibility or efficiency in certain situations. In this section, we’ll explore how to use list comprehension and the reduce()
function from the functools
module to perform union operations.
Using List Comprehension
List comprehension is a powerful feature in Python that allows you to create lists in a very concise way. It can be used as an alternative to the union()
method or the |
operator for merging sets.
Here’s an example:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = {x for x in (set1 | set2)}
print(union_set)
# Output:
# {1, 2, 3, 4, 5}
In this code, {x for x in (set1 | set2)}
creates a new set that includes all unique elements from set1
and set2
. This is essentially the same as the union operation, but done in a more Pythonic way using list comprehension.
Using the reduce()
Function
The reduce()
function from the functools
module is another powerful tool that can be used to perform union operations. This function applies a binary function (a function that takes two arguments) to all elements of an iterable in a cumulative way.
Here’s an example that uses reduce()
to perform a union operation:
from functools import reduce
set1 = {1, 2, 3}
set2 = {3, 4, 5}
set3 = {5, 6, 7}
union_set = reduce(lambda a, b: a | b, [set1, set2, set3])
print(union_set)
# Output:
# {1, 2, 3, 4, 5, 6, 7}
In this code, reduce(lambda a, b: a | b, [set1, set2, set3])
applies the |
operator to all sets in the list [set1, set2, set3]
in a cumulative way, effectively merging all sets into union_set
.
These alternative approaches to Python union operations can provide more flexibility and efficiency in certain situations. However, they may be less readable to Python beginners or non-programmers. You should choose the approach that best fits your needs and coding style.
Troubleshooting Python Union Operations
While Python’s union operations are generally straightforward, you may encounter some common issues, especially when dealing with non-set data types. In this section, we’ll discuss these issues and provide solutions and workarounds.
Type Errors with Non-Set Data Types
One common issue is trying to perform a union operation on non-set data types, such as lists or tuples. This will result in a TypeError.
Here’s an example that demonstrates this issue:
list1 = [1, 2, 3]
list2 = [3, 4, 5]
try:
union_set = list1.union(list2)
except TypeError as e:
print(f'Error: {e}')
# Output:
# Error: 'list' object has no attribute 'union'
In this code, we try to call the union()
method on list1
, which is a list, not a set. This raises a TypeError because the union()
method is only available for sets.
The solution to this issue is to convert the non-set data types to sets before performing the union operation. You can do this using the set()
function.
Here’s the corrected code:
list1 = [1, 2, 3]
list2 = [3, 4, 5]
union_set = set(list1).union(set(list2))
print(union_set)
# Output:
# {1, 2, 3, 4, 5}
In this code, set(list1).union(set(list2))
first converts list1
and list2
to sets, and then performs the union operation. The resulting union_set
includes all unique elements from both lists.
By understanding these common issues and their solutions, you’ll be better equipped to troubleshoot and optimize your Python union operations.
Understanding Sets and Union Operations in Python
To fully grasp the concept of union operations in Python, it’s essential to understand the fundamentals of sets and the theory behind union operations.
What are Sets in Python?
In Python, a set is an unordered collection of unique elements. Sets are mutable, meaning you can add or remove elements after the set is created. They are also iterable, so you can loop through the elements in a set.
Here’s an example of a set in Python:
my_set = {1, 2, 3, 4, 5}
print(my_set)
# Output:
# {1, 2, 3, 4, 5}
In this code, my_set
is a set that includes the numbers 1 through 5. Notice that the elements are unique and unordered.
What is a Union Operation?
In set theory, a union operation combines the elements of two or more sets into a new set that includes all unique elements from the original sets. In other words, if an element is in any of the original sets, it will be in the union set.
Here’s an example of a union operation in Python:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
print(union_set)
# Output:
# {1, 2, 3, 4, 5}
In this code, set1.union(set2)
performs a union operation on set1
and set2
, resulting in union_set
that includes all unique elements from set1
and set2
.
Understanding the basics of sets and union operations in Python is crucial to effectively using these tools in your code. With this knowledge, you’ll be better equipped to manipulate data and solve problems in Python.
Practical Applications and Further Learning
Python’s union operations are not just theoretical constructs; they have practical applications in a variety of real-world scenarios. Especially in fields like data analysis and manipulation, these operations can be invaluable tools.
Union Operations in Data Analysis
In data analysis, you often deal with large datasets that need to be manipulated and analyzed in various ways. Union operations can help you merge datasets, remove duplicates, and extract unique elements.
Here’s an example of how you might use a union operation in data analysis:
# Two sets representing customer IDs from two different months
month1_customers = {1, 2, 3, 4, 5}
month2_customers = {4, 5, 6, 7, 8}
# Use union operation to find all unique customers across both months
all_customers = month1_customers.union(month2_customers)
print(all_customers)
# Output:
# {1, 2, 3, 4, 5, 6, 7, 8}
In this code, we have two sets representing customer IDs from two different months. We use a union operation to merge these sets into all_customers
, which includes all unique customers across both months. This could be useful for understanding customer retention, acquisition, and overall reach.
Further Exploration: Other Set Operations and Data Structures
While this guide has focused on union operations, Python offers a variety of other set operations, such as intersection, difference, and symmetric difference. These operations can be equally useful in manipulating sets and can be combined with union operations in complex ways.
Python also offers other data structures, like lists, tuples, dictionaries, and more, each with their own unique properties and methods. Understanding these data structures and how to manipulate them is crucial to becoming proficient in Python.
Further Resources for Mastering Python Sets
For further information on Python topics, consider these resources:
- Python Built-In Functions: Your Coding Companion – Dive deep into functions for working with namespaces and scope.
Rounding Numbers in Python with the round() Function – Dive into precision control, floating-point arithmetic, and “round” in Python.
Introducing Delays in Python Code with sleep() – Dive into controlling program timing, timeouts, and concurrency with “sleep.”
Python’s Official Documentation on Sets offers info on Python’s built-in set data type and its methods.
Real Python’s Guide on Python Sets is a tutorial on how to use sets in Python, including union operations.
Python Course’s Chapter on Sets offers info on Python sets, including examples and exercises to test your knowledge.
By exploring these resources and practicing with real-world examples, you can deepen your understanding of Python union operations and sets, and become a more capable and versatile Python programmer.
Wrapping Up: Mastering Python Union Operations
In this comprehensive guide, we’ve delved into the concept and practical application of Python union operations, a powerful tool for merging sets in Python.
We began with the basics, explaining how to perform union operations using the union()
method and the |
operator. We then moved onto more advanced techniques, demonstrating how to merge more than two sets and use union operations in conjunction with other set operations like intersection and difference.
We also explored alternative approaches to performing union operations, such as using list comprehension and the reduce()
function from the functools
module.
Along the way, we tackled common issues you might face when performing union operations, such as type errors with non-set data types, providing solutions and workarounds for each issue.
We also provided a solid understanding of the fundamentals of sets and union operations in Python, and discussed the practical applications of union operations in real-world scenarios like data analysis.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
union() method | Easy to read and understand | Slightly less efficient with larger sets |
| operator | More concise and efficient | Less readable to beginners |
List comprehension | More Pythonic, flexible | Can be less efficient with larger sets |
reduce() function | Can handle multiple sets efficiently | Requires importing functools module |
Whether you’re just starting out with Python union operations or you’re looking to level up your set manipulation skills, we hope this guide has given you a deeper understanding of union operations and their capabilities.
With the knowledge and techniques covered in this guide, you’re now well-equipped to handle a wide range of data manipulation tasks using Python union operations. Happy coding!