Creating Files in Java: Step-by-Step Tutorial
~
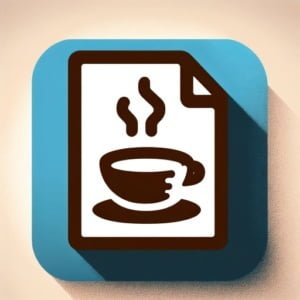
Are you finding it challenging to create files in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling file creation in Java, but we’re here to help.
Think of Java as a skilled craftsman, capable of creating and manipulating files with precision. It’s a powerful tool that allows us to store data, manage information, and build more complex applications.
This guide will walk you through the process of creating files in Java, from the basics using the File
class to more advanced techniques. We’ll cover everything from the creation, manipulation, and usage of files. We’ll also delve into the common issues and their solutions.
Let’s get started and master file creation in Java!
TL;DR: How Do I Create a File in Java?
In Java, you can create a new file using the
File
class, with the syntaxFile file = new File("filename.txt");
. Here’s a simple example:
File file = new File("filename.txt");
if (file.createNewFile()) {
System.out.println("File created: " + file.getName());
} else {
System.out.println("File already exists.");
}
# Output:
# 'File created: filename.txt'
In this example, we create a new File
object and pass the desired filename as a string to the constructor. The createNewFile()
method is then called, which attempts to create a file with the specified name. If the file is successfully created, it prints ‘File created: filename.txt’. If the file already exists, it prints ‘File already exists.’.
This is a basic way to create a file in Java, but there’s much more to learn about file handling in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic File Creation in Java
Java provides us with a built-in File
class to handle file operations. This class gives us the ability to create new files, read and write to files, delete files, and more. One of the most common methods used from this class is the createNewFile()
method, which allows us to create a new file.
Let’s dive into a step-by-step guide on how to use the File
class and the createNewFile()
method.
Step-by-Step Guide to Creating a File in Java
- Create a
File
Object: First, we need to create a newFile
object and specify the filename (and path, if needed) in the constructor.
File file = new File("filename.txt");
- Use the
createNewFile()
Method: Next, we call thecreateNewFile()
method on theFile
object. This method returns a boolean value – true if the file was successfully created and false if the file already exists.
if (file.createNewFile()) {
System.out.println("File created: " + file.getName());
} else {
System.out.println("File already exists.");
}
# Output:
# 'File created: filename.txt'
In this code, if the file is successfully created, ‘File created: filename.txt’ is printed. If the file already exists, ‘File already exists.’ is printed.
Pros and Cons of the File
Class Approach
Pros:
- It’s a simple and straightforward way to create a file in Java.
- It’s built into the Java language, so no additional libraries are required.
Cons:
- The
File
class does not allow for more complex file operations, such as setting file permissions or creating symbolic links. - The
createNewFile()
method can throw anIOException
, which must be caught or declared to be thrown.
Advanced File Creation in Java
As you become more comfortable with file handling in Java, you might find yourself needing more advanced features. This is where the Files
class and the Path
interface come into play.
The Files
Class and Path
Interface
The Files
class and the Path
interface were introduced in Java 7 as part of the new I/O system (NIO). They offer more advanced features compared to the File
class, such as setting file attributes, handling symbolic links, and more.
Let’s take a look at how to create a file using these tools.
Path path = Paths.get("filename.txt");
Files.createFile(path);
System.out.println("File created: " + path.getFileName());
# Output:
# 'File created: filename.txt'
In this example, we first create a Path
object that represents the path to the file we want to create. We then use the Files.createFile()
method to create the file. If the file is successfully created, ‘File created: filename.txt’ is printed.
Benefits and Pitfalls of the Files
Class and Path
Interface
Benefits:
- More advanced features: The
Files
class andPath
interface offer more advanced features compared to theFile
class, such as setting file attributes, handling symbolic links, and more. - Better error handling: Unlike the
File
class, which can only return a boolean value, theFiles
class can throw more specific exceptions, making error handling easier.
Pitfalls:
- Complexity: The
Files
class andPath
interface are more complex to use compared to theFile
class. They can be overkill for simple file operations. - Requires Java 7 or higher: These features are part of the new I/O system (NIO), which was introduced in Java 7. If you’re working with an older version of Java, you won’t be able to use them.
Exploring Alternative Methods for File Creation in Java
While the File
class and the Files
class with Path
interface are the built-in ways to create files in Java, there are also alternative methods. In particular, third-party libraries can offer additional features or more efficient ways to handle file creation.
Apache Commons IO
Apache Commons IO is a library that provides utility classes, stream implementations, file filters, and various other tools. It can be used to create a file in Java in a more concise way.
File file = FileUtils.getFile("filename.txt");
FileUtils.touch(file);
# Output:
# A file named 'filename.txt' is created
In this example, FileUtils.getFile()
is used to create a new File
object, and FileUtils.touch()
is used to create a new file. If the file already exists, the touch()
method will update the file’s last modified time.
Google Guava
Google Guava is another popular library that provides a lot of helpful methods to work with files.
File file = new File("filename.txt");
Files.touch(file);
# Output:
# A file named 'filename.txt' is created
In this example, Files.touch()
from Google Guava is used to create a new file or update the last modified time if the file already exists.
Comparing the Methods
Method | Complexity | Flexibility | Required Java Version |
---|---|---|---|
File class | Low | Low | All |
Files class with Path | Medium | High | 7 and above |
Apache Commons IO | Medium | High | All |
Google Guava | Medium | High | All |
As you can see, each method has its own advantages and disadvantages. The built-in File
class is simple to use but lacks flexibility. The Files
class with Path
interface provides a lot of advanced features but is more complex to use. Apache Commons IO and Google Guava offer a good balance between simplicity and flexibility.
Troubleshooting Common Issues in Java File Creation
While working with file creation in Java, you might encounter some common issues. One of the most frequent is handling an IOException
. This exception is thrown when an input-output operation fails or is interrupted.
Handling IOException
When you’re creating a file in Java, you have to deal with IOException
. This exception is thrown by the createNewFile()
method of the File
class, and by the createFile()
method of the Files
class.
Here’s how you can handle this exception:
try {
File file = new File("filename.txt");
if (file.createNewFile()) {
System.out.println("File created: " + file.getName());
} else {
System.out.println("File already exists.");
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
# Output:
# 'File created: filename.txt'
# OR
# 'File already exists.'
# OR
# 'An error occurred.'
# [Detailed error message]
In this example, we’ve wrapped our file creation code in a try
block. If an IOException
is thrown, the catch
block is executed, printing an error message and the stack trace of the exception.
Remember, it’s crucial to handle exceptions properly in your code to prevent your application from crashing and to provide meaningful error messages to your users.
Understanding File Handling in Java
Before we dive deeper into creating files in Java, it’s essential to understand the fundamental concepts of file handling in this language. In Java, we primarily use two classes for file handling – the File
class and the Files
class.
The File
Class
The File
class is part of the java.io package. It provides methods to work with files and directories. Here’s a simple example of using the File
class to create a file:
File file = new File("filename.txt");
if (file.createNewFile()) {
System.out.println("File created: " + file.getName());
} else {
System.out.println("File already exists.");
}
# Output:
# 'File created: filename.txt'
# OR
# 'File already exists.'
In this example, we create a File
object and use the createNewFile()
method to create a new file with the specified name.
The Files
Class
The Files
class is part of the java.nio.file package, introduced in Java 7. It provides static methods to work with files and directories. Here’s an example of creating a file using the Files
class:
Path path = Paths.get("filename.txt");
Files.createFile(path);
System.out.println("File created: " + path.getFileName());
# Output:
# 'File created: filename.txt'
In this example, we create a Path
object and use the createFile()
method of the Files
class to create a new file.
File Paths in Java
A file path is a string that provides the location of a file or directory in the file system. In Java, we can represent file paths using the Path
interface, part of the java.nio.file package. A Path
object can be used with the Files
class to create, move, or delete a file.
In our examples, we’ve used relative file paths (like “filename.txt”), which are relative to the current working directory. However, you can also use absolute file paths, which provide the full path to the file, starting from the root of the file system.
Understanding these concepts is crucial for effective file handling in Java. Whether you’re using the File
class for simple file operations or the Files
class for more advanced tasks, knowing how files and file paths work will help you write more robust and efficient code.
Extending File Creation to Larger Java Applications
Creating files is a fundamental part of programming, but it’s just the tip of the iceberg when it comes to file handling in Java. Once you’ve mastered file creation, you can explore more complex operations like reading from and writing to files, and working with file I/O streams.
Reading and Writing to Files in Java
After creating a file, you often need to write data to it or read data from it. Java provides several classes to do this, such as FileWriter
and FileReader
for text files, and FileOutputStream
and FileInputStream
for binary files.
File I/O Streams in Java
Streams are a fundamental concept in Java I/O operations. A stream can be thought of as a sequence of data. In Java, a Stream
is a sequence of objects. There are two main types of streams: byte streams (for binary data) and character streams (for text data).
Further Resources for Mastering Java File Handling
The Java File Class has much more to be discovered, Click Here for an in-depth Guide on how to utilize the class fully.
To delve deeper into specific File Class topics, here are some resources that provide in-depth tutorials and guides:
- How to Read Lines in Java – Master Java line reading methods for efficient text parsing and data extraction.
Exploring Java FileWriter – Master Java file writing operations using FileWriter for efficient data persistence.
Java I/O Tutorial by Oracle is the official Java tutorial by Oracle. It covers all aspects of Java I/O, including file handling.
Java File I/O (NIO.2) Tutorial by TutorialsPoint focuses on the NIO.2 API, which was introduced in Java 7.
Java File Class Tutorial by GeeksforGeeks provides a comprehensive guide to the
File
class in Java.
Wrapping Up: File Creation in Java
In this comprehensive guide, we’ve delved into the process of creating files in Java, from the simple usage of the File
class to more advanced techniques using the Files
class and Path
interface, and even explored third-party libraries like Apache Commons IO and Google Guava.
We began with the basics, understanding how to create a file using the File
class and its createNewFile()
method. We then progressed to more advanced techniques, exploring the Files
class and Path
interface, which offer more flexibility and advanced features. Finally, we ventured into the world of third-party libraries that provide additional features for file creation.
Along the way, we tackled common challenges you might face when creating files in Java, such as handling IOException
and choosing the right method for file creation based on your specific needs. We provided solutions and workarounds for each issue, helping you to navigate these challenges with ease.
Here’s a quick comparison of the methods we’ve discussed:
Method | Complexity | Flexibility | Required Java Version |
---|---|---|---|
File class | Low | Low | All |
Files class with Path | Medium | High | 7 and above |
Apache Commons IO | Medium | High | All |
Google Guava | Medium | High | All |
Whether you’re just starting out with file creation in Java or you’re looking to level up your skills, we hope this guide has equipped you with the knowledge and confidence to handle file creation in Java effectively.
With its balance of simplicity and flexibility, Java provides a variety of ways to create files. Now, you’re well equipped to choose the method that best suits your needs. Happy coding!