Java File Class: Handling Files and Directories with Ease
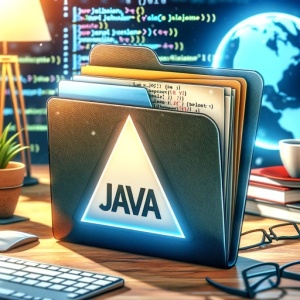
Are you finding it challenging to manage files in your Java applications? You’re not alone. Many developers find themselves puzzled when it comes to handling files in Java, but we’re here to help.
Think of Java’s File class as a skilled librarian – it’s a powerful utility that helps you manage your files efficiently. From creating, reading, writing, to deleting files, the File class in Java is a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of mastering the File class in Java, from the basics to more advanced techniques. We’ll cover everything from simple file operations to handling directories, file paths, and file permissions, as well as alternative approaches.
Let’s dive in and start mastering the File class in Java!
TL;DR: What is the File Class in Java and How is it Used?
The
File
class in Java is a utility for file handling operations such as creating, reading, writing, and deleting files, instantiated with the syntax,File file = new File("filename.txt");
. It provides an abstraction that allows developers to interact with the file system in a platform-independent manner.
Here’s a simple example of creating a new file using the File class:
File file = new File("filename.txt");
boolean isCreated = file.createNewFile();
// Output:
// This will create a new file named 'filename.txt' in the project directory if it does not exist already.
In this example, we create a new instance of the File class with the filename as the parameter. The createNewFile()
method is then called, which creates a new file with the specified name in the project directory if it does not exist already. The method returns a boolean value indicating whether the file was created successfully.
This is a basic way to use the File class in Java, but there’s much more to learn about file handling. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- File Handling Basics with Java’s File Class
- Advanced File Handling with Java’s File Class
- Exploring Alternative Approaches to File Handling in Java
- Troubleshooting Common Issues with Java’s File Class
- Understanding File Handling in Java
- The Role of Java’s File Class in Larger Projects
- Wrapping Up: Mastering Java’s File Class for Efficient File Handling
File Handling Basics with Java’s File Class
The File class in Java is a fundamental tool for basic file operations such as creating, reading, writing, and deleting files. Let’s break down each of these operations and see how we can perform them using the File class.
Creating a New File
To create a new file, we instantiate the File class and call the createNewFile()
method. Here’s a simple example:
try {
File file = new File("newfile.txt");
boolean isCreated = file.createNewFile();
if(isCreated)
System.out.println("File has been created.");
else
System.out.println("File already exists.");
} catch (IOException e) {
e.printStackTrace();
}
// Output:
// 'File has been created.' or 'File already exists.'
In this block of code, we are creating a new file named ‘newfile.txt’. If the file is created successfully, it prints ‘File has been created.’, otherwise, it prints ‘File already exists.’.
Reading a File
To read a file, Java provides several ways, but one of the most common ways is using Scanner
class along with File class. Here’s how you can do it:
try {
File file = new File("newfile.txt");
Scanner reader = new Scanner(file);
while (reader.hasNextLine()) {
String data = reader.nextLine();
System.out.println(data);
}
reader.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
// Output:
// Contents of the 'newfile.txt' file
In this example, we create a Scanner
object and pass our file to it. Then, we read the file line by line using the hasNextLine()
and nextLine()
methods.
Writing to a File
To write to a file, we can use the FileWriter class in conjunction with the File class. Here’s a simple example:
try {
FileWriter writer = new FileWriter("newfile.txt");
writer.write("Hello, World!");
writer.close();
System.out.println("Successfully wrote to the file.");
} catch (IOException e) {
e.printStackTrace();
}
// Output:
// 'Successfully wrote to the file.'
In this example, we write ‘Hello, World!’ to our file. If the operation is successful, it prints ‘Successfully wrote to the file.’.
Deleting a File
To delete a file, we use the delete()
method of the File class. Here’s how to do it:
File file = new File("newfile.txt");
boolean isDeleted = file.delete();
if(isDeleted)
System.out.println("File deleted.");
else
System.out.println("Failed to delete the file.");
// Output:
// 'File deleted.' or 'Failed to delete the file.'
In this example, we delete ‘newfile.txt’. If the file is deleted successfully, it prints ‘File deleted.’, otherwise, it prints ‘Failed to delete the file.’.
These are the basic file operations you can perform using the File class in Java. In the following sections, we will dive into more advanced uses of the File class.
Advanced File Handling with Java’s File Class
While the basic operations cover a lot of ground, the File class in Java offers more advanced features that let you handle directories, manage file paths, and control file permissions. Let’s delve into these advanced uses of the File class.
Handling Directories
The File class allows you to create, list, and navigate directories. Here’s an example of creating a directory:
File dir = new File("myDirectory");
boolean isCreated = dir.mkdir();
if(isCreated)
System.out.println("Directory created.");
else
System.out.println("Directory already exists.");
// Output:
// 'Directory created.' or 'Directory already exists.'
In this example, we create a directory named ‘myDirectory’. If the directory is created successfully, it prints ‘Directory created.’, otherwise, it prints ‘Directory already exists.’.
Managing File Paths
File paths can be absolute or relative. The File class provides methods to retrieve and manipulate these paths. Here’s how you can retrieve the absolute path of a file:
File file = new File("newfile.txt");
String absolutePath = file.getAbsolutePath();
System.out.println("Absolute Path: " + absolutePath);
// Output:
// 'Absolute Path: ' followed by the absolute path of 'newfile.txt'
In this block of code, we retrieve the absolute path of ‘newfile.txt’ and print it.
Controlling File Permissions
You can check and modify the permissions of a file using the File class. Here’s an example of checking if a file is readable, writable, and executable:
File file = new File("newfile.txt");
System.out.println("Readable: " + file.canRead());
System.out.println("Writable: " + file.canWrite());
System.out.println("Executable: " + file.canExecute());
// Output:
// 'Readable: true or false'
// 'Writable: true or false'
// 'Executable: true or false'
In this example, we check if ‘newfile.txt’ is readable, writable, and executable, and print the results.
These are just a few examples of the advanced capabilities of the File class in Java. By understanding and using these features effectively, you can manage files and directories more efficiently in your Java applications.
Exploring Alternative Approaches to File Handling in Java
While the File class is a powerful tool, Java offers other ways to handle files too. Let’s explore some of these alternatives, namely the Files class and third-party libraries.
Java’s Files Class
The Files class, introduced in Java 7, provides methods for file operations such as reading, writing, and updating files. It also includes methods for dealing with symbolic links and file permissions. Here’s an example of reading a file using the Files class:
Path path = Paths.get("newfile.txt");
List<String> lines = Files.readAllLines(path, StandardCharsets.UTF_8);
for (String line : lines) {
System.out.println(line);
}
// Output:
// Contents of the 'newfile.txt' file
In this example, we read ‘newfile.txt’ using the Files class and print its contents. The Files class can simplify file operations and make your code cleaner and more readable.
Third-Party Libraries
Third-party libraries such as Apache Commons IO and Google’s Guava provide utilities for file handling. These libraries offer robust features and can simplify complex file operations. Here’s an example of reading a file using Apache Commons IO:
File file = new File("newfile.txt");
List<String> lines = FileUtils.readLines(file, "UTF-8");
for (String line : lines) {
System.out.println(line);
}
// Output:
// Contents of the 'newfile.txt' file
In this example, we read ‘newfile.txt’ using Apache Commons IO and print its contents. Third-party libraries can be a powerful tool for file handling, especially for complex or large-scale projects.
While the File class provides a wide range of functionalities, these alternative approaches can offer additional features and simplify your code. Understanding these alternatives allows you to choose the best approach for your specific needs.
Troubleshooting Common Issues with Java’s File Class
While the File class is a powerful tool for handling files, you may encounter some common issues, particularly when dealing with exceptions and different file systems. Let’s discuss these issues and their solutions.
Handling Exceptions
When working with the File class, you may encounter IOExceptions. These exceptions can occur when a file operation fails. Here’s an example of handling an IOException when creating a file:
try {
File file = new File("newfile.txt");
boolean isCreated = file.createNewFile();
if(isCreated)
System.out.println("File has been created.");
else
System.out.println("File already exists.");
} catch (IOException e) {
e.printStackTrace();
}
// Output:
// 'File has been created.' or 'File already exists.'
// If an IOException occurs, it prints the stack trace.
In this example, we handle the IOException using a try-catch block. If an IOException occurs, we print the stack trace to understand the issue better.
Dealing with Different File Systems
File systems can differ between operating systems. For instance, the path separators are different in Windows (\) and Unix (/). The File class provides methods to handle these differences, such as separatorChar
to get the system-dependent default name-separator character. Here’s an example:
char separator = File.separatorChar;
System.out.println("File separator: " + separator);
// Output:
// 'File separator: ' followed by the system-dependent default name-separator character
In this example, we retrieve the system-dependent default name-separator character and print it. By using these methods, you can write platform-independent code with the File class.
These are some of the common issues you may encounter when using the File class in Java. By understanding these issues and their solutions, you can use the File class more effectively in your Java applications.
Understanding File Handling in Java
File handling is a critical aspect of any programming language, and Java is no exception. It allows us to create, read, update, and delete files, which are essential operations for many applications.
The Role of the File Class
In Java, the File class plays a significant role in file handling. It serves as an abstraction that represents both files and directory pathnames in an abstract, system-independent manner. This means you can use the File class to perform operations on files regardless of the underlying file system.
Streams and Buffers: The Building Blocks
Two fundamental concepts in Java file handling are streams and buffers. A stream is a sequence of data. In Java, a stream is composed of bytes. It’s called a stream because it’s like a stream of water that continues to flow.
There are two main types of streams in Java:
- Byte streams (InputStream and OutputStream): They perform input and output of 8-bit bytes.
Character streams (Reader and Writer): They perform input and output for 16-bit unicode.
Here’s an example of using a byte stream to read a file:
try {
FileInputStream fis = new FileInputStream("newfile.txt");
int i;
while ((i = fis.read()) != -1) {
System.out.print((char) i);
}
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
// Output:
// Contents of 'newfile.txt'
In this example, we use a FileInputStream (a type of byte stream) to read ‘newfile.txt’ and print its contents. If an IOException occurs, we print the stack trace.
On the other hand, a buffer is a memory area that stores data temporarily. In file handling, buffers are often used when reading from or writing to a file, improving the speed of the input/output operation.
Understanding these fundamental concepts is key to mastering file handling in Java, and the File class is an integral part of this process.
The Role of Java’s File Class in Larger Projects
The File class in Java is not just for simple file operations; it plays a crucial role in larger projects as well. Whether you’re working on a web application, a network program, or a database management system, the File class can be an essential part of your toolkit.
File Class in Networking
In networking applications, you often need to send or receive files over the network. The File class allows you to read and write files, which you can then send or receive using network streams. Here’s a simple example of sending a file over a socket:
Socket socket = new Socket("localhost", 8080);
File file = new File("newfile.txt");
byte[] bytes = new byte[(int) file.length()];
FileInputStream fis = new FileInputStream(file);
BufferedInputStream bis = new BufferedInputStream(fis);
bis.read(bytes, 0, bytes.length);
OutputStream os = socket.getOutputStream();
os.write(bytes, 0, bytes.length);
os.flush();
socket.close();
// Output:
// This will send 'newfile.txt' over a socket to 'localhost' on port 8080.
In this example, we read ‘newfile.txt’ into a byte array and send it over a socket to ‘localhost’ on port 8080.
File Class in Database Management
In database management systems, you often need to import or export data. The File class allows you to read and write data to and from files, which can then be used to import or export data from your database. Here’s a simple example of exporting data to a file:
try (PrintWriter writer = new PrintWriter(new File("data.csv"))) {
StringBuilder sb = new StringBuilder();
sb.append("Name,Class,Score
");
sb.append("John,Doe,90
");
sb.append("Jane,Doe,95
");
writer.write(sb.toString());
System.out.println("Data exported.");
} catch (FileNotFoundException e) {
e.printStackTrace();
}
// Output:
// 'Data exported.'
In this example, we write some data to ‘data.csv’. This data could then be imported into a database.
Further Resources for Mastering Java’s File Class
To deepen your understanding of the File class in Java and its applications, here are some resources you might find useful:
- Exploring Java Classes – Master Java class concepts for organizing and encapsulating data and behavior.
Creating Files in Java – Explore Java techniques for creating files programmatically for data storage or manipulation
Reading Files in Java – Discover how to read files in Java for accessing and processing external data.
Official File Class Java Documentation provides a detailed explanation of the File class and its methods.
Baeldung’s Guide to Java’s File Class offers a comprehensive overview of the File class in Java, complete with examples.
Java File Class Tutorial by DigitalOcean delves into the File class in Java, covering its methods and their uses in detail.
By exploring these resources and understanding the role of the File class in larger projects, you can become proficient in file handling in Java and apply this knowledge to your own projects.
Wrapping Up: Mastering Java’s File Class for Efficient File Handling
In this comprehensive guide, we’ve explored the ins and outs of the File class in Java, a critical utility for managing files in Java applications. We’ve looked at its basic and advanced uses, alternative approaches, and common issues and their solutions.
We began with the basics, learning how to create, read, write, and delete files using the File class. We then delved into more advanced usage, such as handling directories, managing file paths, and controlling file permissions. We also discussed common issues you might encounter when using the File class, such as handling exceptions and dealing with different file systems, and provided solutions for these issues.
Along the way, we explored alternative approaches to file handling in Java, such as using the Files class and third-party libraries. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
File Class | Comprehensive, supports many operations | May require troubleshooting for some operations |
Files Class | Simplifies file operations, makes code cleaner | Less comprehensive than the File class |
Third-Party Libraries | Robust, can simplify complex operations | May require additional dependencies |
Whether you’re just starting out with the File class in Java or you’re looking to deepen your understanding of file handling, we hope this guide has been a valuable resource. With its wide range of functionalities, the File class is a powerful tool for file handling in Java. Now, you’re well equipped to manage files efficiently in your Java applications. Happy coding!