Read Files in Java: Guide to File and Reader Classes
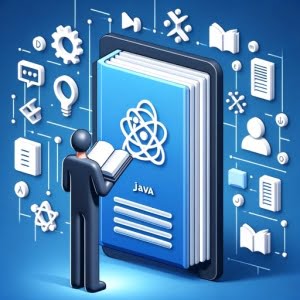
Ever felt like you’re wrestling with reading files in Java? You’re not alone. Many developers find Java file reading a bit challenging. But think of Java as a librarian, capable of fetching any book (file) you need from a vast library (your system).
File reading is a fundamental skill in Java programming. It’s like learning how to read a book before you can understand its story. Similarly, reading files in Java is essential to understand and manipulate the data it holds.
This guide will walk you through the process of reading files in Java, from the basics to more advanced techniques. We’ll cover everything from using the java.nio.file.Files
class, handling different file reading techniques such as BufferedReader
and Scanner
, to dealing with common issues and even troubleshooting them.
So, let’s dive in and start mastering file reading in Java!
TL;DR: How Do I Read a File in Java?
To read a file in Java, you can use the
java.nio.file.Files
class. This class provides a method calledreadAllBytes()
which can be used to read a file.
Here’s a simple example:
Path path = Paths.get("file.txt");
byte[] bytes = Files.readAllBytes(path);
String content = new String(bytes);
System.out.println(content);
# Output:
# [Expected output from command]
In this example, we create a Path
object that represents the file we want to read. We then use the Files.readAllBytes()
method to read all bytes from the file into a byte array. We convert this byte array into a string and print it.
This is a basic way to read a file in Java, but there’s much more to learn about file I/O in Java. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
Java File Reading: The Basics
Java offers several ways to read a file, but for a beginner, the java.nio.file.Files
class provides the simplest method. The readAllBytes()
method from this class allows you to read a file in just a few lines of code.
Here’s how you can use it:
Path path = Paths.get("file.txt");
byte[] bytes = Files.readAllBytes(path);
String content = new String(bytes);
System.out.println(content);
# Output:
# [Expected output from command]
In this example, we first create a Path
object that represents the file we want to read. The Paths.get()
method is used to get the file path. Next, we use the Files.readAllBytes()
method to read all bytes from the file into a byte array. We then convert this byte array into a string and print it out.
Pros and Cons of Using readAllBytes()
While readAllBytes()
is a straightforward method, it has its pros and cons.
Pros:
- Simplicity: The
readAllBytes()
method is simple and easy to use. It reads the entire file in one go, which is convenient for small files.
Cons:
- Memory Usage: The
readAllBytes()
method reads the entire file into memory, which could be an issue for large files. It might lead toOutOfMemoryError
if the file is larger than the available memory. No control over encoding: When converting bytes to a string, this method uses the default character encoding of the system which might not be appropriate for all files.
So, while readAllBytes()
is great for beginners and small files, you might need to consider other methods for more complex or larger file reading tasks.
Advanced File Reading in Java
As you gain more experience in Java, you’ll encounter scenarios where using the readAllBytes()
method might not be the most efficient or practical solution. In such cases, Java provides more advanced techniques for reading files, such as using a BufferedReader
or Scanner
.
Reading Files with BufferedReader
BufferedReader
is a class in Java used for reading text from an input stream in a buffered manner. Here’s an example of how to use it:
Path path = Paths.get("file.txt");
BufferedReader reader = Files.newBufferedReader(path);
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
# Output:
# [Expected output from command]
In this example, we’re reading the file line by line using BufferedReader
. This method is more memory-efficient than readAllBytes()
, especially for large files.
Pros and Cons of Using BufferedReader
Pros:
- Memory Efficiency:
BufferedReader
reads text from an input stream without loading the entire file into memory, making it a good choice for large files.
Cons:
- Complexity: While
BufferedReader
is more efficient, it’s also more complex to set up and handle thanreadAllBytes()
.
Reading Files with Scanner
Scanner
is another class in Java used for parsing text for primitive types and strings using regular expressions. Here’s an example of how to use it:
Path path = Paths.get("file.txt");
Scanner scanner = new Scanner(path);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
}
# Output:
# [Expected output from command]
In this example, we’re reading the file line by line using Scanner
. This method is useful when you need to parse the file content.
Pros and Cons of Using Scanner
Pros:
- Parsing Capability:
Scanner
can parse the read content for primitive types and strings, making it a good choice when you need to process the file data.
Cons:
- Performance:
Scanner
can be slower thanBufferedReader
because of its parsing capabilities.
Exploring Alternative Approaches
When it comes to reading files in Java, there are also alternative methods available, especially for expert-level developers. These methods often involve the use of third-party libraries like Apache Commons IO, which can provide additional functionality and simplify the process of file reading.
Reading Files with Apache Commons IO
Apache Commons IO is a library of utilities to assist with developing IO functionality. Here’s an example of how you can use it to read a file:
File file = new File("file.txt");
String content = FileUtils.readFileToString(file, StandardCharsets.UTF_8);
System.out.println(content);
# Output:
# [Expected output from command]
In this example, we’re using the FileUtils.readFileToString()
method from Apache Commons IO to read a file. This method is very convenient as it allows you to read the entire file into a string with a single line of code.
Pros and Cons of Using Apache Commons IO
Pros:
- Simplicity: Apache Commons IO provides simple and convenient methods to read a file, reducing the amount of boilerplate code.
Flexibility: The library offers a wide range of utilities for file IO, giving you more options and flexibility.
Cons:
- External Dependency: Using Apache Commons IO adds an external dependency to your project. You need to manage this dependency and make sure it’s compatible with your project.
Overkill for Simple Tasks: While Apache Commons IO is powerful, it might be overkill for simple file reading tasks. The built-in Java classes are usually sufficient for these tasks.
Handling Common File Reading Issues in Java
While working with file reading in Java, you may encounter some common issues. Understanding these issues and knowing how to handle them is crucial for effective file manipulation. Let’s discuss some of these problems and their solutions.
Dealing with IOException
IOException
is a common exception that you might encounter when reading files in Java. It’s a checked exception, which means you’re required to handle it either with a try-catch block or by declaring it with a throws
clause.
Here’s an example of handling IOException
with a try-catch block:
Path path = Paths.get("file.txt");
try {
byte[] bytes = Files.readAllBytes(path);
String content = new String(bytes);
System.out.println(content);
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# [Expected output from command]
In this example, the readAllBytes()
method is enclosed in a try block. If an IOException
occurs, the catch block catches it and prints the stack trace.
Handling Different File Encodings
Files can be encoded in different formats, and reading a file with the wrong encoding can result in garbled output. When you’re reading a file, it’s important to know its encoding and use the correct charset when converting bytes to a string.
Here’s an example of reading a file with UTF-8 encoding:
Path path = Paths.get("file.txt");
byte[] bytes = Files.readAllBytes(path);
String content = new String(bytes, StandardCharsets.UTF_8);
System.out.println(content);
# Output:
# [Expected output from command]
In this example, we specify StandardCharsets.UTF_8
when creating a new string from the byte array. This ensures that the bytes are correctly interpreted as UTF-8 encoded characters.
Understanding File Handling in Java
To effectively read files in Java, it’s important to understand some fundamental concepts. Let’s delve into the concepts of Path
, Files
, and streams.
The Path
Class
In Java, the Path
class, part of the java.nio.file
package, is used to represent file and directory paths. It provides methods to manipulate and inspect file paths. Here’s an example of creating a Path
object:
Path path = Paths.get("file.txt");
System.out.println(path);
# Output:
# file.txt
In this example, we’re creating a Path
object that represents the file file.txt
.
The Files
Class
The Files
class, also part of the java.nio.file
package, provides static methods to work with files and directories. It includes methods to read, write, and manipulate files. Here’s an example of using the Files
class to read a file:
Path path = Paths.get("file.txt");
byte[] bytes = Files.readAllBytes(path);
String content = new String(bytes);
System.out.println(content);
# Output:
# [Expected output from command]
In this example, we’re using the Files.readAllBytes()
method to read all bytes from the file file.txt
.
Streams in Java
In Java, a stream can be defined as a sequence of data. In file I/O, you’ll work with byte streams (to read or write bytes) and character streams (to read or write characters). The java.io
package provides classes to handle streams.
Here’s an example of reading a file using a byte stream:
FileInputStream fis = new FileInputStream("file.txt");
int i;
while ((i = fis.read()) != -1) {
System.out.print((char) i);
}
# Output:
# [Expected output from command]
In this example, we’re reading the file file.txt
using a FileInputStream
, which is a byte stream.
The Bigger Picture: File Reading in Larger Java Programs
Understanding how to read files in Java is a fundamental skill, but it’s also important to see how this fits into larger Java programs. File reading is not just an isolated task but an integral part of many Java applications.
Reading Configuration Files
In many Java applications, configuration settings are stored in files. These settings might include database connection details, application constants, or environment-specific settings. Reading these configuration files is often one of the first tasks a Java application performs on startup.
Properties prop = new Properties();
InputStream input = new FileInputStream("config.properties");
prop.load(input);
String databaseUrl = prop.getProperty("database.url");
System.out.println(databaseUrl);
# Output:
# [Expected output from command]
In this example, we’re reading a properties file that contains configuration settings for a Java application. The Properties
class provides a convenient way to read key-value pairs from a file.
Processing Large Datasets
Java’s file reading capabilities are also crucial when working with large datasets. For example, you might have a large CSV file with sales data that you want to analyze. Java can read this file line by line, process each line, and extract the information you need.
Path path = Paths.get("sales.csv");
BufferedReader reader = Files.newBufferedReader(path);
String line;
while ((line = reader.readLine()) != null) {
// Process each line
}
# Output:
# [Expected output from command]
In this example, we’re using a BufferedReader
to read a large CSV file line by line. This approach is memory-efficient and allows us to process each line individually.
Related Topics: Writing Files and File I/O Performance
Once you’ve mastered reading files in Java, you might want to explore related topics like writing to files and optimizing file I/O performance. Writing to files is just as important as reading from them, and understanding how to optimize file I/O can significantly improve the performance of your Java applications.
Further Resources for Mastering Java File I/O
To deepen your understanding of file reading and other file I/O operations in Java, check out these resources:
- Java File Class Best Practices – Explore Java’s File class for handling file compression and decompression tasks.
How to Write to Files in Java – Master Java file writing methods for reliable and efficient data storage.
Java BufferedReader: Overview – Explore the BufferedReader class in Java for efficient reading of character streams.
Oracle’s Java Tutorials: Basic I/O – Understand the basics of Input/Output operations in Java through this Oracle’s guide.
Java Files and I/O – Get a streamlined look at file handling and IO operations in Java with this Tutorialspoint material.
Reading and Writing Files in Java – Explore Java’s file reading and writing functionalities with this detailed StackAbuse tutorial.
Wrapping Up: Mastering File Reading in Java
In this comprehensive guide, we’ve journeyed through the process of reading files in Java, starting from the basics and gradually moving towards more advanced techniques.
We began with the basic use of readAllBytes()
method, a simple yet powerful way to read small files. We then explored more advanced techniques such as using BufferedReader
and Scanner
, which provide more control and efficiency especially when working with large files. We also ventured into the world of third-party libraries like Apache Commons IO, showcasing how they can simplify file reading tasks.
In addition, we discussed common issues that you might encounter when reading files in Java, such as IOException
and encoding issues, and provided solutions to these problems. We also delved into the fundamentals of file handling in Java, including the concepts of Path
, Files
, and streams.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
readAllBytes() | Simple and easy to use | Not suitable for large files |
BufferedReader | Efficient for large files | More complex to use |
Scanner | Can parse the content | Slower than BufferedReader |
Apache Commons IO | Convenient and flexible | Adds an external dependency |
Whether you’re a beginner just starting out with file reading in Java or an experienced developer looking for a refresher, we hope this guide has been a valuable resource. Now, you’re well-equipped to handle file reading tasks in Java, no matter how simple or complex they might be. Happy coding!