Python Guide To Activate Venv / Virtual Environments
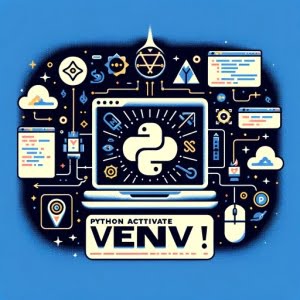
Are you finding it challenging to activate a virtual environment in Python? You’re not alone. Many developers find themselves puzzled when it comes to managing different Python versions and packages without affecting their main Python installation.
Think of a virtual environment as a sandbox – a safe space where you can play around with different Python versions and packages without any fear.
This guide will walk you through the process of activating a virtual environment in Python. We’ll explore the basic use, delve into more advanced techniques, and even discuss alternative approaches and common issues.
Let’s get started!
TL;DR: How Do I Activate a Virtual Environment in Python?
Activating a virtual environment in Python is straightforward. You can do this via the command
source venv/bin/activate
. This uses the ‘activate’ script located in the ‘Scripts’ directory of your virtual environment.
Here’s a simple example:
source venv/bin/activate
In this example, we’re using the source
command followed by the path to the ‘activate’ script within our virtual environment (named ‘venv’ in this case). Running this command in your terminal will activate the virtual environment, allowing you to work with the Python version and packages specific to that environment.
But there’s more to Python virtual environments than just activation. Continue reading for a more detailed guide on creating and managing virtual environments in Python, including advanced use cases and troubleshooting tips.
Table of Contents
- Creating and Activating a Python Virtual Environment
- Managing Multiple Python Virtual Environments
- Exploring Alternative Tools: pyenv and conda
- Troubleshooting Python Virtual Environments
- Understanding Python Virtual Environments
- Expanding Your Python Skills: Package Management and Beyond
- Wrapping Up: Mastering Python Virtual Environments
Creating and Activating a Python Virtual Environment
Starting with Python virtual environments is quite simple. Here’s a step-by-step guide to help you create and activate a virtual environment.
Creating a Virtual Environment
First, we need to create a virtual environment. You can do this using the venv
module that comes pre-installed with Python 3. Here’s how:
python3 -m venv my_env
In this command, python3 -m venv
is calling the venv
module, and my_env
is the name of the virtual environment you’re creating. You can replace my_env
with any name you like.
Activating the Virtual Environment
Once you’ve created the virtual environment, the next step is to activate it. You can do this using the activate
script located in the bin
directory of your virtual environment. Here’s how:
source my_env/bin/activate
In this command, source
is a shell command that reads and executes commands from the file specified as its argument, in this case, the activate
script.
When you run this command in your terminal, you’ll notice that the prompt changes to include the name of the activated virtual environment. This indicates that the virtual environment is now active, and any Python commands you run will use the environment’s settings and packages.
(my_env) $
This (my_env)
before your prompt means that my_env
is currently active, and you’re now working within that virtual environment. Any Python packages you install while the environment is active will be installed in this environment, not globally on your system.
Remember, you can replace my_env
with the name of your virtual environment. And that’s it! You’ve successfully created and activated a Python virtual environment.
Managing Multiple Python Virtual Environments
As your Python projects grow in number and complexity, you may find yourself needing to manage multiple virtual environments. Here’s how you can do it.
Creating Multiple Virtual Environments
First, let’s create a couple of new virtual environments. We’ll name them env1
and env2
:
python3 -m venv env1
python3 -m venv env2
These commands create two separate virtual environments in your current directory. Each environment has its own Python binary and can have its own independent set of installed Python packages.
Switching Between Virtual Environments
Activating a virtual environment is as simple as running the activate
script in its bin
directory, as we’ve seen before. So, to switch between env1
and env2
, you just need to deactivate the current environment and then activate the other one.
Here’s how you deactivate a virtual environment:
(my_env) $ deactivate
$
The deactivate
command will end your current session with the virtual environment, and the prompt will go back to normal, indicating no environment is currently active.
Now, you can activate env2
like this:
source env2/bin/activate
And your prompt will change to show that env2
is now active:
(env2) $
And there you have it! You’ve successfully switched from one virtual environment to another. By managing multiple virtual environments, you can maintain separate Python configurations for different projects, ensuring that each has exactly the dependencies it needs and nothing more.
Exploring Alternative Tools: pyenv and conda
While venv
is a great tool for managing Python virtual environments, it’s not the only one. There are other robust tools like pyenv
and conda
that offer additional features and can be a better fit for certain use cases.
pyenv: Version Management Made Easy
pyenv
is a Python version management tool. It allows you to install multiple versions of Python on your system and switch between them on a per-project basis. Here’s a simple example of how to install a specific Python version using pyenv
:
pyenv install 3.8.0
And here’s how you can set it as your default Python version:
pyenv global 3.8.0
In these commands, 3.8.0
can be replaced with any Python version you wish to install or set as default.
conda: A Package Manager and More
conda
is a package and environment management system. It allows you to create separate environments for your projects, similar to venv
, but it also handles package dependencies and can install packages from the conda package repository as well as from the Python Package Index (PyPI). Here’s an example of creating a new environment with conda
:
conda create --name my_env python=3.8
And here’s how you can activate it:
conda activate my_env
In these commands, my_env
is the name of the environment, and 3.8
is the Python version you want in that environment.
Comparing venv, pyenv, and conda
While all three tools can manage Python environments, they each have their strengths. venv
is built into Python and is straightforward to use, making it a great choice for beginners. pyenv
excels at managing multiple Python versions, and conda
is powerful and versatile, especially for data science projects where managing complex package dependencies is crucial.
Choosing the right tool depends on your specific needs. You may even find that using a combination of these tools works best for you.
Troubleshooting Python Virtual Environments
While working with Python virtual environments, you might encounter some common issues. Let’s discuss some of these problems and how to resolve them.
Issue: ‘No module named venv’
When trying to create a virtual environment, you might see a ‘No module named venv’ error. This usually means that the venv
module is not installed on your Python version. Here’s an example of this error:
python3 -m venv my_env
# Output:
# /usr/bin/python3: No module named venv
To resolve this issue, you need to install the python3-venv
package using apt
:
sudo apt-get install python3-venv
Issue: ‘Command not found’ After Activation
Sometimes, after activating a virtual environment, you might find that running Python or pip returns a ‘command not found’ error. This could mean that the virtual environment was not created correctly.
To resolve this issue, you could try recreating the virtual environment. Make sure to deactivate the current environment first:
(my_env) $ deactivate
$ python3 -m venv my_env
$ source my_env/bin/activate
Issue: Forgetting to Activate the Environment
It’s easy to forget to activate your virtual environment before starting work. If you find that changes you’re making don’t seem to have any effect, or you’re not seeing the packages you expect, check to make sure you’ve activated the correct environment.
Remember, you can always check which environment is currently active by looking at your command prompt, or by using the which python
command:
(my_env) $ which python
# Output:
# /path/to/my_env/bin/python
This command will show the path to the Python binary that will be used in the active session, which should be within your active virtual environment.
Working with Python virtual environments can be tricky, but once you’re familiar with these common issues and their solutions, you’ll be able to navigate your way through smoothly.
Understanding Python Virtual Environments
Before we dive deeper into the topic of activating Python virtual environments, it’s essential to understand what a virtual environment is and why it’s crucial for Python development.
What is a Virtual Environment?
In Python, a virtual environment is a self-contained directory tree that includes a Python installation of a particular version, plus a number of additional packages. Different applications can then use different virtual environments. To put it simply, a virtual environment is a tool that helps to keep dependencies required by different projects separate by creating isolated Python environments for them.
python3 -m venv my_env
This command creates a new virtual environment named ‘my_env’. Inside this directory, it houses its own Python binary and can have its own independent set of installed Python packages.
Why Use a Virtual Environment?
The main reason to use a virtual environment is to isolate your Python environment from the global Python environment. This isolation helps you avoid conflicts between Python packages and allows you to work with different versions of Python and Python packages without affecting other projects or the system Python installation.
How Does a Virtual Environment Work?
When you create a virtual environment, Python creates a directory for the new virtual environment and sets up a fresh Python environment in that directory. This environment includes a copy of the Python binary itself, a copy of the entire Python standard library, a copy of the pip installer, and (crucially) a new directory to hold installed packages.
When you activate the virtual environment using the source
command, your shell’s PATH is temporarily adjusted so that the Python binary and installed scripts in the virtual environment directory are found before the system-wide Python binary. This means that when you type python
, you get the Python binary in the virtual environment, not the system-wide Python binary.
source my_env/bin/activate
This command activates the virtual environment ‘my_env’, and your command prompt changes to show the name of the activated environment. Now, when you install new packages using pip, they are installed in the virtual environment’s directory, not in the system-wide directory. This keeps your global Python environment clean and manageable.
Understanding these fundamentals of Python virtual environments will help you better comprehend the advanced topics we’ll discuss later in this guide.
Expanding Your Python Skills: Package Management and Beyond
While understanding how to activate a Python virtual environment is crucial, it’s just the tip of the iceberg. There are many more related topics that can enhance your Python development experience. One such topic is managing Python packages within a virtual environment using pip.
Mastering Package Management with pip
pip
is the standard package manager for Python. It allows you to install and manage additional libraries and dependencies that are not distributed as part of the standard Python library. When working within a virtual environment, pip
will install packages into the environment’s package directory, keeping your global environment clean and manageable.
Here’s an example of installing a package (let’s say requests
) using pip
:
(my_env) $ pip install requests
This command installs the requests
package, which you can now import and use in your Python scripts within this environment.
import requests
response = requests.get('https://www.example.com')
print(response.status_code)
# Output:
# 200
In this Python script, we’re importing the requests
module and using it to send a GET request to ‘https://www.example.com’. The status code of the response is then printed out.
Further Resources to Master Python Environments
Ready to deepen your understanding of Python environments and package management? Here are some resources that can help:
- Easy Python 3 Installation on Ubuntu – Discover a hassle-free process of installing Python on your Ubuntu system.
Boosting Python Productivity with IPython – Tips and Tricks for IPython and explore how it elevates your Python coding experience
Simplifying Python Version Control with Version Managers – Dive into the world of version management and discover the flexibility it offers.
Python’s official venv documentation is a comprehensive guide to
venv
, straight from the creators of Python.The Hitchhiker’s Guide to Python is an excellent resource for Python best practices, including a section on virtual environments.
Real Python’s guide on pip thoroughly explains
pip
, Python’s package installer.
By exploring these resources and practicing what you’ve learned in this guide, you’ll be well on your way to mastering Python virtual environments and package management.
Wrapping Up: Mastering Python Virtual Environments
In this comprehensive guide, we’ve delved into the world of Python virtual environments. We’ve discovered how to activate a virtual environment in Python, the benefits of using such environments, and the techniques to manage multiple environments efficiently.
We began with the basics, learning how to create a virtual environment and activate it using the activate
script. We then moved onto more advanced territory, exploring how to manage multiple environments and switch between them. Along the way, we tackled common challenges you might encounter when working with Python virtual environments, providing you with solutions for each issue.
We also explored alternative tools for managing Python environments such as pyenv
and conda
, giving you a broader understanding of the available options. Here’s a quick comparison of these tools:
Tool | Pros | Cons |
---|---|---|
venv | Built into Python, simple to use | Basic, lacks some features of other tools |
pyenv | Excellent at managing multiple Python versions | Doesn’t handle package dependencies |
conda | Powerful, handles complex package dependencies | Can be overkill for simple projects |
Whether you’re a beginner just starting out with Python virtual environments or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of how to activate and manage Python virtual environments and the power of these tools. Happy coding!