Python hashlib Module | Guide To Hashing
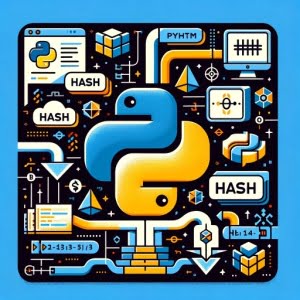
Are you finding it challenging to hash data in Python? You’re not alone. Many developers find themselves puzzled when it comes to handling data hashing in Python, but we’re here to help.
Think of Python’s hashlib module as a reliable vault – a tool that can securely transform your data into a fixed size sequence of bytes. It’s a versatile and handy tool for various tasks involving data integrity and security.
In this guide, we’ll walk you through the process of using Python’s hashlib module, from the basics to more advanced techniques. We’ll cover everything from simple hashing using different algorithms to hashing larger data like files, as well as alternative approaches.
Let’s dive in and start mastering Python’s hashlib module!
TL;DR: How Do I Use the hashlib Module in Python?
To hash data in Python, you can use the hashlib module’s hash functions, like
hashed_data = hashlib.sha256(data.encode())
. Here’s a simple example:
import hashlib
data = 'Hello, World!'
hashed_data = hashlib.sha256(data.encode())
print(hashed_data.hexdigest())
# Output:
# 'a591a6d40bf420404a011733cfb7b190d62c65bf0bcda32b57b277d9ad9f146e'
In this example, we’ve used the hashlib module’s sha256
function to hash the string ‘Hello, World!’. The encode()
function is used to convert the string into bytes, which is the required input for the sha256
function. The hexdigest()
function is then used to convert the hash object into a hexadecimal string, which is printed out.
This is a basic way to use the hashlib module in Python, but there’s much more to learn about data hashing. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with Python’s hashlib Module
- Hashing Larger Data with Python’s hashlib
- Exploring Alternatives: Python’s hmac Module
- Troubleshooting Python’s hashlib Module
- The Fundamentals of Data Hashing
- The Relevance of Data Hashing in Today’s Tech Landscape
- Wrapping Up: Mastering Python’s hashlib Module for Data Hashing
Getting Started with Python’s hashlib Module
Understanding hashlib’s Hash Functions: md5, sha1, and sha256
Python’s hashlib module provides a variety of hash functions, including md5
, sha1
, and sha256
. These functions are used to create hash objects, which are then used to generate hashes of data.
Let’s take a look at a simple code example using each of these functions:
import hashlib
data = 'Hello, World!'
# MD5
md5_hash = hashlib.md5(data.encode())
print('MD5:', md5_hash.hexdigest())
# SHA1
sha1_hash = hashlib.sha1(data.encode())
print('SHA1:', sha1_hash.hexdigest())
# SHA256
sha256_hash = hashlib.sha256(data.encode())
print('SHA256:', sha256_hash.hexdigest())
# Output:
# MD5: ed076287532e86365e841e92bfc50d8c
# SHA1: 2ef7bde608ce5404e97d5f042f95f89f1c232871
# SHA256: a591a6d40bf420404a011733cfb7b190d62c65bf0bcda32b57b277d9ad9f146e
In this example, we’re hashing the string ‘Hello, World!’ using the md5
, sha1
, and sha256
functions from the hashlib module. The encode()
function is used to convert the string into bytes, which is the required input for the hashing functions. The hexdigest()
function is then used to convert the hash object into a hexadecimal string, which is printed out.
Advantages and Pitfalls of Different Hash Functions
While all these functions serve the same basic purpose – to create a hash of data – they each have their advantages and potential pitfalls.
- MD5:
md5
is a widely used hash function that produces a 128-bit hash value. It’s commonly used for checksums and data integrity. However,md5
is considered to be broken in terms of collision resistance, which means it’s possible for two different inputs to produce the same hash. Therefore, it’s not recommended for functions where security is critical. SHA1:
sha1
produces a 160-bit hash value, making it stronger thanmd5
. However,sha1
is also considered to be broken in terms of collision resistance and is no longer recommended for functions where security is critical.SHA256:
sha256
is part of the SHA-2 family of cryptographic hash functions and is widely used in security applications and protocols. It produces a 256-bit hash value and is currently considered to be secure against collision attacks.
What has function should I use?
Unless there’s a specific reason like performance to use something else, we strongly recommend using sha256. While md5
and sha1
can be used for simple checksums and data integrity checks, sha256
should be used when a higher level of security is required.
Hashing Larger Data with Python’s hashlib
Hashing Files with the hashlib Module
Python’s hashlib module is not limited to just hashing strings – you can also use it to hash larger data, such as files. This can be useful for a variety of purposes, such as checking the integrity of a file or comparing two files to see if they’re identical.
Here’s an example of how you can hash a file using the sha256
function from the hashlib module:
import hashlib
def hash_file(filename):
h = hashlib.sha256()
with open(filename, 'rb') as file:
while True:
chunk = file.read(h.block_size)
if not chunk:
break
h.update(chunk)
return h.hexdigest()
print(hash_file('example.txt'))
# Output:
# 'd7d52d110e6e0657c118e3a6f77aef42f8ec9915b5a4e3a7f6beeb500e635f0c'
In this example, we’re creating a function called hash_file
that takes a filename as an argument. The function opens the file in binary mode and reads it in chunks of h.block_size
bytes. Each chunk is then passed to the update
method of the hash object to update the hash. Once all chunks have been read and hashed, the hexdigest
method is called to return the final hash as a hexadecimal string.
This approach is more efficient than reading the entire file into memory at once, especially for larger files. It also ensures that the file is properly closed after it has been read.
Best Practices for Hashing Files
When hashing files, it’s important to keep a few best practices in mind:
- Always open files in binary mode when hashing. This ensures that the data is read exactly as it is stored on disk, without any transformations.
Use a buffer to read large files in chunks, as shown in the example above. This reduces memory usage and can improve performance.
Always close files after you’re done with them. In Python, the best way to do this is by using the
with
statement, which automatically closes the file when it’s no longer needed.
By following these best practices, you can effectively and efficiently hash larger data with Python’s hashlib module.
Exploring Alternatives: Python’s hmac Module
Hashing Data with hmac
While the hashlib module is a powerful tool for hashing data, Python also provides other modules that offer additional functionality. One such module is hmac
– a module for generating keyed-hash message authentication codes.
Here’s an example of how you can use the hmac
module to hash data:
import hmac
message = 'Hello, World!'.encode()
key = 'secret'.encode()
h = hmac.new(key, message, digestmod='SHA256')
print(h.hexdigest())
# Output:
# 'f7ad406ce1b8838f04e7a129ea7a79f0f249ffab56e1f3a598d8b814067d307a'
In this example, we’re using the hmac
module’s new
function to create an hmac
object. This function takes three arguments: a key, a message, and a digestmod
. The key and message are both byte strings, and the digestmod
is the name of the hash function to use. In this case, we’re using ‘SHA256’. The hexdigest
method is then used to convert the hmac
object into a hexadecimal string.
Advantages and Disadvantages of hmac
The hmac
module provides an additional layer of security over the hashlib module by requiring a secret key to hash data. This makes it more difficult for an attacker to generate the same hash, even if they have the original data.
However, the hmac
module also has its drawbacks. It can be more complex to use than the hashlib module, especially for beginners. Furthermore, it requires the management of secret keys, which can add an additional layer of complexity to your code.
When to Use hmac
While the hmac
module can provide additional security, it’s not always necessary to use it. For simple checksums and data integrity checks, the hashlib module is usually sufficient. However, if you’re working on a project where security is a major concern, such as a password manager or a secure messaging app, the hmac
module can be a valuable tool.
Troubleshooting Python’s hashlib Module
Handling Common Issues in hashlib
While Python’s hashlib module is generally straightforward to use, you may encounter some issues along the way. One common issue is the UnicodeEncodeError
.
UnicodeEncodeError in hashlib
The UnicodeEncodeError
typically occurs when you try to hash a string that contains non-ASCII characters. This is because the hashlib functions require byte strings as input, and Python’s default encoding is ASCII.
Here’s an example of how this error might occur:
import hashlib
data = 'Hello, 世界!'
hashed_data = hashlib.sha256(data.encode())
print(hashed_data.hexdigest())
# Output:
# UnicodeEncodeError: 'ascii' codec can't encode characters in position 7-8: ordinal not in range(128)
In this example, we’re trying to hash the string ‘Hello, 世界!’, which contains non-ASCII characters. When we call the encode
method on the string, Python tries to encode it using the default ASCII encoding, which results in a UnicodeEncodeError
.
Solutions for UnicodeEncodeError
The solution to this issue is to specify an encoding that can handle non-ASCII characters when calling the encode
method. The ‘utf-8’ encoding is a good choice, as it can handle any character in the Unicode standard.
Here’s how you can fix the above code:
import hashlib
data = 'Hello, 世界!'
hashed_data = hashlib.sha256(data.encode('utf-8'))
print(hashed_data.hexdigest())
# Output:
# '2a6e9f9146a428ae8b763a26b5be9275fbb9c3a3c9c5c77a8534a25d39bc10c8'
In this fixed example, we’re specifying ‘utf-8’ as the encoding when calling the encode
method. This allows Python to correctly encode the string into bytes, which can then be hashed by the sha256
function.
Remember, whenever you’re dealing with strings that might contain non-ASCII characters, it’s a good practice to specify an encoding when converting them into bytes. This will help you avoid the UnicodeEncodeError
and ensure that your code works with any string.
The Fundamentals of Data Hashing
The Importance of Data Hashing in Computer Science
Data hashing is a fundamental concept in computer science, with wide-ranging applications in fields like data retrieval, security, and data integrity. At its core, data hashing is about transforming any form of data into a fixed-size sequence of bytes, regardless of the original data’s size or type.
Hashing serves several crucial functions. It ensures data integrity by allowing you to check if data has been tampered with. It also enables efficient data retrieval, as hash tables use hash functions to quickly locate data. In the realm of security, hashing is used to securely store passwords and for digital signatures.
Understanding Different Hashing Algorithms
There are various hashing algorithms, each with its own use cases, advantages, and disadvantages. Let’s examine three common ones you’ll encounter when using Python’s hashlib
module: md5
, sha1
, and sha256
.
import hashlib
data = 'Python hashlib'.encode()
# MD5 hash
md5_hash = hashlib.md5(data)
print('MD5:', md5_hash.hexdigest())
# SHA1 hash
sha1_hash = hashlib.sha1(data)
print('SHA1:', sha1_hash.hexdigest())
# SHA256 hash
sha256_hash = hashlib.sha256(data)
print('SHA256:', sha256_hash.hexdigest())
# Output:
# MD5: 5cfd210fed6b8b04eab269ecfe4c95c1
# SHA1: 65d2a1dd60a517eb27bfbf530cf6a543285bd8ac
# SHA256: 6dcd4ce23d88e2ee95838f7b014b6284a5bb6d76d2595947bd9f079d820d8b2a
In this example, we’re generating hashes for the string ‘Python hashlib’ using md5
, sha1
, and sha256
. Each algorithm produces a unique hash value, and each has its specific use cases.
- MD5: While
md5
is fast and produces a compact hash, it’s susceptible to collision attacks, where different inputs produce the same hash. Therefore, it’s not recommended for security-critical applications. SHA1:
sha1
is a step up frommd5
in terms of security, but recent years have exposed vulnerabilities, and it’s no longer considered secure against well-funded attackers.SHA256: Part of the SHA-2 family,
sha256
is currently recommended for most cryptographic applications. It’s slower and produces longer hashes thanmd5
andsha1
, but it offers significantly better security.
Understanding these algorithms and their use cases will help you choose the right tool for your hashing needs.
The Relevance of Data Hashing in Today’s Tech Landscape
Data Hashing in Cybersecurity and Data Integrity
Data hashing is not only a fundamental concept in computer science, but it also plays a significant role in today’s tech landscape, specifically in cybersecurity and data integrity.
In cybersecurity, hashing algorithms like those provided by Python’s hashlib module are used to securely store user passwords. Instead of storing the actual password, which could be stolen and misused, systems store the hash of the password. When a user enters their password, it’s hashed, and the hash is compared to the stored hash. This way, even if an attacker gains access to the stored hashes, they won’t be able to determine the actual passwords.
In terms of data integrity, hashing is used to ensure that data has not been tampered with during transmission. A hash of the data is sent along with the data itself. The recipient can then hash the received data and compare it to the received hash. If the two hashes match, the data has not been altered; if they don’t match, the data has been tampered with.
Exploring Related Concepts: Digital Signatures and HMAC
If you’re interested in data hashing, you might also want to explore related concepts like digital signatures and HMAC (Hash-Based Message Authentication Code).
Digital signatures use hashing and encryption to verify the authenticity of digital messages or documents. They provide a layer of validation and security, ensuring that a message was not altered in transit (integrity) and that it came from the person who claims to have sent it (authenticity).
HMAC is a specific type of message authentication code (MAC) that uses a cryptographic hash function and a secret cryptographic key. It’s used to verify both the data integrity and the authenticity of a message.
Further Resources for Mastering Python’s hashlib Module
To deepen your understanding of Python’s hashlib module and data hashing, here are some resources you might find helpful:
- Beginner’s Guide to Python Modules – Master the art of creating your own custom Python modules.
Python Turtle Graphics for Beginners – Learn how to use Python turtle for educational and creative purposes.
Deep Copying in Python: A Quick Guide – Learn about Python’s “copy” and “deepcopy” for handling objects.
Python’s Official Documentation on hashlib provides an overview of the hashlib module and its functions.
Python Cryptography Toolkit is a collection of cryptographic algorithms and protocols, including secure hash functions like SHA256 and HMAC, for Python use.
Python Security – This resource covers a broad range of security-related Python topics, including hashing and data integrity.
Wrapping Up: Mastering Python’s hashlib Module for Data Hashing
In this comprehensive guide, we’ve delved deep into the world of Python’s hashlib module, a powerful tool for hashing data in Python.
We started with the basics, learning how to use the hashlib module’s hash functions, including md5
, sha1
, and sha256
, to generate hashes of data. We provided code examples and discussed the advantages and potential pitfalls of each function.
We then moved onto more advanced topics, discussing how to use the hashlib module to hash larger data, such as files. We provided a code example, discussed the output, and shared best practices for hashing files.
We also explored alternative approaches to data hashing in Python, such as using the hmac module, and discussed their advantages, disadvantages, and use cases. We also dove into common issues one might encounter when using the hashlib module, such as the UnicodeEncodeError
, and provided solutions.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
hashlib’s md5, sha1 | Simple to use, suitable for checksums and data integrity | Not secure against collision attacks |
hashlib’s sha256 | Secure against collision attacks, suitable for security-critical applications | Slower than md5, sha1 |
hmac | Provides an additional layer of security by requiring a secret key | More complex to use, requires key management |
Whether you’re just starting out with Python’s hashlib module or you’re looking to deepen your understanding of data hashing, we hope this guide has been a valuable resource.
With its balance of simplicity, flexibility, and power, Python’s hashlib module is an invaluable tool for any Python programmer working with data hashing. Happy hashing!