Python Modules | Effective Use of Python Built-in Modules
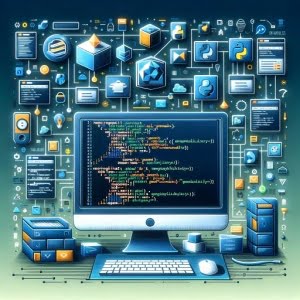
Understanding Python modules is crucial for efficient code organization and reuse at IOFLOOD. Modules enable us to structure our Python scripts into manageable sections, promoting modular programming practices. This article delves into what Python modules are, providing insights and examples to help our dedicated server customers improve their code maintainability.
This guide will walk you through Python modules and python built in modules, how to inmport libraries in python, and even compare the differences between python package vs module.
Understanding Python modules is key to writing clean, efficient code. So let’s dive in!
TL;DR: What are Python Modules?
Python modules are essentially libraries containing code designed to implement a set of functions, classes, or variables, which can then be utilized in other Python scripts. You can import libraries in Python with the syntax,
import [pythonModule]
, and utilize the module’s functions with the syntax,pythonModule.function()
.
Here’s a simple example of creating and using a Python module:
# mymodule.py
def hello():
print('Hello, world!')
# main.py
import mymodule
mymodule.hello()
# Output:
# 'Hello, world!'
In this example, we first create a Python module named ‘mymodule.py’ with a function ‘hello()’ that prints ‘Hello, world!’. Then, in our main script, we import this module and call the function. Once we run this python script, the output is ‘Hello, world!’.
If you’re curious to dive deeper into Python modules, their benefits, and advanced usage, keep reading. This guide is designed to take you from a beginner to an expert in Python modules. Let’s get started!
Table of Contents
Beginner’s Guide to Python Modules
Python modules are all about organization and code reusability. To begin, let’s understand how to create a simple Python module. A Python module is essentially a .py file containing Python definitions and statements. For instance, let’s create a module named ‘greetings.py’ and code a single function that prints a greeting:
# greetings.py
def greet():
print('Hello from the greetings module!')
Now, we can import this module into any other Python script and use the ‘greet()’ function. Here’s how you can do it:
# main.py
import greetings
greetings.greet()
# Output:
# 'Hello from the greetings module!'
By importing the ‘greetings’ module, we’re able to call the ‘greet()’ function defined in the module. This way, we can keep our code organized and avoid cluttering our main script with definitions.
Advanced Use of Python Modules
As you become more comfortable with Python modules, you can start exploring more complex uses. Let’s dive into some advanced techniques that can make your Python programming more efficient.
Importing Python Built In Module Functions
Firstly, you can import a specific function from a module using the from module import function
syntax. This can be particularly useful if you only need to use a specific function from a module, and not the entire module. Let’s use our ‘greetings’ module from the previous section as an example:
# main.py
from greetings import greet
greet()
# Output:
# 'Hello from the greetings module!'
Here, we’re only importing the ‘greet()’ function from the ‘greetings’ module. This allows us to call the function directly, without having to reference the module name.
When importing custom Modules, they may not always be in the working directory. You can find out how to import modules from other directiories with this guide!
Aliasing Python Modules
Secondly, you can use aliases to rename your modules when importing them. This is especially handy when dealing with modules with long names or if you want to avoid naming conflicts. Here’s an example:
# main.py
import greetings as g
g.greet()
# Output:
# 'Hello from the greetings module!'
In this example, we’re importing the ‘greetings’ module as ‘g’, which allows us to reference the module with this shorter alias.
Inspecting Python Modules with dir()
Finally, Python provides a built-in function dir()
which can be used to inspect the contents of a module. This can be useful when you want to see what functions, classes, or variables a module provides. Let’s inspect our ‘greetings’ module:
# main.py
import greetings
print(dir(greetings))
# Output:
# ['__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', 'greet']
The dir()
function returns a list of all the contents of the ‘greetings’ module. Among these, ‘greet’ is the function we defined.
These advanced techniques can help you use Python modules more effectively, making your Python programming more efficient and organized.
Python Packages vs Module
As you delve deeper into Python programming, you’ll discover that Python modules alone may not suffice for larger projects. This is where Python packages come into play.
A Python package is essentially a directory that contains multiple module files and a special python __init__.py
file, which allows Python to recognize the directory as a package.
Creating and Using Python Packages
Creating a Python package is straightforward. Let’s create a package named ‘greetings_package’ that contains our ‘greetings’ module:
/greetings_package
__init__.py
greetings.py
In this structure, ‘greetings_package’ is a directory that contains an empty python __init__.py
file and our ‘greetings.py’ module. Now, we can import the ‘greet()’ function from the ‘greetings’ module within this package:
# main.py
from greetings_package.greetings import greet
greet()
# Output:
# 'Hello from the greetings module!'
Here, we’re importing the ‘greet()’ function from the ‘greetings’ module within the ‘greetings_package’ package.
Comparing Python Package vs Module
Now, you might be wondering, when should you use a Python module and when should you use a Python package? Here’s a simple rule of thumb: use modules to organize related functions, classes, and variables, and use packages to organize related modules. While modules help you avoid repeating code, packages help you avoid repeating module names.
Python modules and packages are powerful tools for organizing your Python code. By understanding how to use them effectively, you can write clean, efficient, and maintainable code.
Troubleshooting Python Modules
As you work with Python modules, you may encounter a few common issues. Let’s discuss these challenges and provide solutions to help you overcome them.
Dealing with Python Module Import Errors
One of the most common problems when working with Python modules is import errors, or the no module named error. These errors typically occur when Python can’t find the module you’re trying to import. Here’s what an import error might look like:
# main.py
import non_existent_module
# Output:
# ImportError: No module named 'non_existent_module'
In this example, we’re trying to import a module that doesn’t exist, leading to an ImportError. To resolve this issue, ensure that the module you’re trying to import exists and is in the correct location. Python looks for modules in the directories defined in sys.path
, which includes the directory of the script you’re running and the site-packages directory where third-party packages are installed.
Resolving Python Module Name Clashes
Another common issue is name clashes. This happens when two modules have functions or classes with the same name. When you import these modules in the same script, calling the function or class may lead to unexpected behaviors. Here’s an example:
# greetings.py
def greet():
print('Hello from the greetings module!')
# salutations.py
def greet():
print('Hello from the salutations module!')
# main.py
import greetings
import salutations
greetings.greet()
salutations.greet()
# Output:
# 'Hello from the greetings module!'
# 'Hello from the salutations module!'
In this example, both ‘greetings’ and ‘salutations’ modules have a ‘greet()’ function. We can avoid the name clash by referencing the function with the module name.
Working with Python modules can sometimes be a challenge, but understanding these common issues and their solutions will help you navigate these challenges. Remember, the key to effective Python programming is writing clean, organized, and reusable code.
Understanding Modularity in Python
Modularity in programming is the practice of dividing a software system into separate, independent modules of subsystems. This concept is fundamental to efficient programming and is particularly relevant when working with Python modules.
Why is Modularity Important?
Modularity brings numerous benefits to the table. It enhances readability, as each module focuses on a single aspect of the system. It promotes code reusability, as modules can be used across different projects. It also simplifies maintenance and testing, as each module can be modified or tested independently.
# math_operations.py
def add(x, y):
return x + y
def subtract(x, y):
return x - y
# main.py
import math_operations
print(math_operations.add(5, 3))
print(math_operations.subtract(5, 3))
# Output:
# 8
# 2
In this example, we have a module ‘math_operations’ with two functions: ‘add()’ and ‘subtract()’. By grouping these related functions in a module, we can easily reuse them in different scripts, enhancing code reusability and organization.
Python’s Module System
Python’s module system is a prime example of implementing modularity. Each Python module is a separate file that can contain functions, classes, or variables. These modules can then be imported into other scripts, promoting code reusability and organization. Python’s module system is simple yet powerful, enabling programmers to write efficient and maintainable code.
Understanding the concept of modularity and Python’s module system is fundamental to mastering Python programming. With Python modules, you can write clean, organized, and reusable code, making your Python programming journey smoother and more enjoyable.
Python Modules in Larger Projects
Python modules become increasingly relevant as your projects grow in size and complexity. They allow you to structure your code in a way that’s manageable and scalable. For instance, in a large web application, you might have separate modules for user authentication, database operations, and user interface logic. This modular approach makes it easier to understand, test, and maintain your code.
# db_operations.py
def connect_to_db():
print('Connecting to database...')
def query_db():
print('Querying database...')
# main.py
import db_operations
db_operations.connect_to_db()
db_operations.query_db()
# Output:
# 'Connecting to database...'
# 'Querying database...'
In this example, we have a ‘db_operations’ module that handles database-related operations. By grouping these operations in a separate module, we can keep our main script clean and focused.
Exploring Python Libraries and the Python Standard Library
If you’re interested in diving deeper into Python’s modular ecosystem, you might want to explore Python libraries and the Python Standard Library. A Python library is a package of pre-written code that you can reuse in your projects, while the Python Standard Library is a collection of modules provided as part of Python’s standard distribution.
The Python Standard Library includes modules for various functionalities, including file I/O, system calls, string operations, Internet protocol handling, and more. Learning about these modules can greatly enhance your Python programming skills.
For more resources on Python modules, Python libraries, and the Python Standard Library, you can refer to the official Python documentation. This comprehensive guide will provide you with a deeper understanding of Python’s modular ecosystem and its benefits.
Further Info for Python Modules
To deepen your understanding of Python Modules and to master their application, we have gathered some free resources:
- Python Import Statement: Module Integration Simplified – Learn the fundamentals of Python’s “import” statements.
Python Threading for Concurrent Execution – Dive into concurrent programming and managing threads in Python.
Trinket’s Python Documents reference guide from covers Python essentials, perfect for beginners.
Python Modules: A Comprehensive Guide by Toppr delves into Python modules, exploring its functions and methods.
Programiz’s Tutorial on Python Modules
- Learn about Python modules to structure your program efficiently.
By thoroughly exploring these resources and leveraging Python Modules effectively, you can elevate your skills as a Python developer
Recap: Python Modules Tutorial
Python modules are the cornerstone of efficient Python programming. They allow you to keep your code organized, reusable, and maintainable. From creating a simple Python module to importing specific functions, Python modules offer a wide range of possibilities for structuring your code.
# greetings.py
def greet():
print('Hello from the greetings module!')
# main.py
import greetings
greetings.greet()
# Output:
# 'Hello from the greetings module!'
In the code above, we created a simple module ‘greetings.py’ with a function ‘greet()’, and then imported this module into our main script. This is the basic idea behind Python modules – creating reusable pieces of code that can be imported and used in other scripts.
For larger projects, Python packages come into play. They allow you to group related modules together, providing a higher level of organization. Remember, the key to effective Python programming is writing clean, organized, and reusable code.
Python Modules | Python Packages |
---|---|
Single .py files | Directories containing multiple .py files |
Used to organize related functions, classes, and variables | Used to organize related modules |
Import with import module | Import with from package import module |
Python modules and packages are powerful tools in your Python programming toolkit. By mastering these concepts, you can take your Python programming skills to the next level.