Python Threading: Multitasking Development Guide
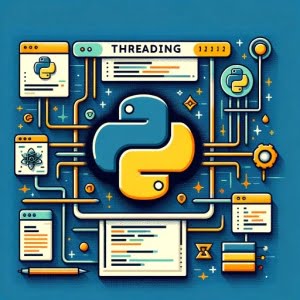
Are you finding it challenging to manage multiple tasks in your Python programs? You’re not alone. Many developers grapple with this task, but Python’s threading module can help you orchestrate multiple threads to work in harmony, much like a skilled conductor.
Whether you’re working on a web scraper that needs to fetch data from multiple URLs simultaneously, or a GUI application that needs to perform background tasks without freezing the user interface, understanding how to use threading in Python can significantly streamline your coding process.
This guide will walk you through the essentials of threading in Python, from basic usage to advanced techniques. We’ll cover everything from creating and managing threads, to more complex uses such as thread synchronization and communication between threads, and even some alternative approaches for concurrent programming in Python.
Let’s dive in and start mastering threading in Python!
TL;DR: How Do I Use Threading in Python?
To use threading in Python, you utilize the built-in
threading
module. This module allows you to create and manage threads, enabling your program to perform multiple tasks concurrently.
Here’s a simple example:
import threading
def print_numbers():
for i in range(10):
print(i)
def print_letters():
for letter in 'abcdefghij':
print(letter)
thread1 = threading.Thread(target=print_numbers)
thread2 = threading.Thread(target=print_letters)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
# Output:
# 0
# a
# 1
# b
# 2
# c
# 3
# d
# 4
# e
# 5
# f
# 6
# g
# 7
# h
# 8
# i
# 9
# j
In this example, we create two threads: thread1
and thread2
. thread1
is tasked with printing numbers from 0 to 9, while thread2
prints letters from ‘a’ to ‘j’. The start()
method initiates each thread, and the join()
method ensures that the main program waits for both threads to complete before proceeding.
This is a basic way to use threading in Python, but there’s much more to learn about managing threads and handling more complex scenarios. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Python Threading Basics: A Beginner’s Guide
- Python Threading: Advanced Techniques
- Exploring Alternatives to Threading in Python
- Troubleshooting Python Threading: Common Issues and Solutions
- Understanding Threading: The Fundamentals
- Python Threading: Beyond the Basics
- Wrapping Up: Mastering Threading in Python
Python Threading Basics: A Beginner’s Guide
Python threading is a powerful tool for managing multiple tasks simultaneously. It’s all about creating and managing threads using Python’s built-in threading
module. But what exactly are threads?
In the realm of programming, a thread is the smallest unit of execution. It’s like a separate flow of execution. Multiple threads within a process share the same data space with the main thread and can, therefore, share information or communicate with each other more easily than if they were separate processes.
Let’s dive into the basics of Python threading with a simple example:
import threading
def print_hello_five_times():
for i in range(5):
print('Hello')
def print_hi_five_times():
for i in range(5):
print('Hi')
thread1 = threading.Thread(target=print_hello_five_times)
thread2 = threading.Thread(target=print_hi_five_times)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
# Output:
# Hello
# Hi
# Hello
# Hi
# Hello
# Hi
# Hello
# Hi
# Hello
# Hi
In this example, we create two threads using the Thread
class from the threading
module. Each thread is associated with a function: print_hello_five_times
and print_hi_five_times
. The start()
method kicks off each thread, and join()
ensures the main program waits for these threads to complete before continuing.
This basic use of Python threading is a powerful way to handle multiple tasks concurrently. However, it’s worth noting that while threading can speed up certain tasks, it can also introduce complexity into your programs. For example, threads can lead to race conditions, where the order of execution can impact the program’s result. We’ll delve into these potential pitfalls and how to avoid them in later sections.
Python Threading: Advanced Techniques
As you become more comfortable with threads in Python, you’ll encounter scenarios that require more advanced techniques. Two such scenarios involve thread synchronization and communication between threads.
Thread Synchronization
Thread synchronization is defined as a mechanism which ensures that two or more concurrent threads do not simultaneously execute some particular program segment known as a critical section. Let’s consider a scenario where two threads are working on a shared data and we need to ensure that only one thread can manipulate the data at a time.
Here’s an example using threading.Lock
to illustrate this:
import threading
counter = 0
def increase_counter(lock):
global counter
lock.acquire()
try:
counter += 1
finally:
lock.release()
lock = threading.Lock()
threads = []
for i in range(100):
thread = threading.Thread(target=increase_counter, args=(lock,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(counter)
# Output:
# 100
In this example, we create 100 threads, each of which increases a shared counter by 1. We use a lock to ensure that only one thread can increase the counter at any given time, preventing race conditions.
Communication Between Threads
Sometimes, we need threads to communicate with each other or exchange data. Python’s queue
module provides several ways to do this. Here’s an example:
import queue
import threading
def worker(q):
while True:
item = q.get()
if item is None:
break
print(f'Working on {item}')
q.task_done()
q = queue.Queue()
threads = []
for i in range(5):
thread = threading.Thread(target=worker, args=(q,))
threads.append(thread)
thread.start()
for item in range(20):
q.put(item)
q.join()
for i in range(5):
q.put(None)
for thread in threads:
thread.join()
# Output:
# Working on 0
# Working on 1
# Working on 2
# ...and so on until...
# Working on 19
In this example, we create a queue and five worker threads. The worker threads continuously take items from the queue and process them. When there are no more items, we add five None
items to the queue, signaling the workers to exit.
These advanced techniques can help you handle more complex scenarios with Python threading, enhancing the efficiency and robustness of your programs.
Exploring Alternatives to Threading in Python
While threading is a powerful tool, Python offers other methods for concurrent programming. Understanding these alternatives can help you choose the right approach for your specific needs. Let’s explore two popular alternatives: multiprocessing and asyncio.
Multiprocessing
The multiprocessing module in Python is a powerful tool that allows you to create multiple processes, each with its own Python interpreter and memory space. This can be particularly beneficial in CPU-bound tasks where threading might be limited due to the Global Interpreter Lock (GIL).
Here’s an example of how you might use multiprocessing:
from multiprocessing import Process
def print_func(continent='Asia'):
print('The name of continent is : ', continent)
if __name__ == "__main__": # confirms that the code is under main function
names = ['America', 'Europe', 'Africa']
procs = []
proc = Process(target=print_func) # instantiating without any argument
procs.append(proc)
proc.start()
# instantiating process with arguments
for name in names:
proc = Process(target=print_func, args=(name,))
procs.append(proc)
proc.start()
# complete the processes
for proc in procs:
proc.join()
# Output:
# The name of continent is : Asia
# The name of continent is : America
# The name of continent is : Europe
# The name of continent is : Africa
In this example, we create a separate process for printing the name of each continent. Each process has its own memory space and Python interpreter.
Asyncio
Asyncio is a Python library that provides support for asynchronous IO through coroutines, multiplexing IO access over sockets and other resources, and implementing network protocols. It’s particularly useful for IO-bound tasks where you’re often waiting for input/output operations to complete.
Here’s a basic asyncio example:
import asyncio
async def main():
print('Hello')
await asyncio.sleep(1)
print('World')
asyncio.run(main())
# Output:
# Hello
# (waits for 1 second)
# World
In this example, we define a coroutine function main
that prints ‘Hello’, waits for 1 second, and then prints ‘World’. We run the coroutine using asyncio.run()
.
Both multiprocessing and asyncio offer powerful alternatives to threading for concurrent programming in Python. The choice between threading, multiprocessing, and asyncio depends on the nature of your task:
- For IO-bound tasks where you’re often waiting for input/output operations to complete, asyncio can be a great choice.
For CPU-bound tasks where you’re heavily utilizing the CPU, multiprocessing can help you bypass the limitations of the GIL and utilize multiple CPUs or cores on your machine.
For tasks that require shared memory space or lightweight task switching, threading can be the perfect fit.
Troubleshooting Python Threading: Common Issues and Solutions
While threading in Python can significantly enhance your programs, it can also introduce certain challenges. Two common issues you might encounter when using threading are race conditions and deadlocks.
Race Conditions
A race condition occurs when two or more threads can access shared data and they try to change it at the same time. As a result, the values of variables may be unpredictable and vary depending on the timings of context switches of the processes.
Here’s an example of a race condition:
import threading
counter = 0
def increase_counter():
global counter
counter += 1
threads = []
for i in range(100):
thread = threading.Thread(target=increase_counter)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(counter)
# Output:
# Varies between runs, but likely not 100
In this example, we’re increasing a shared counter with multiple threads. However, because we’re not using any synchronization mechanisms, the threads can interfere with each other, leading to a race condition and an unpredictable output.
Deadlocks
A deadlock is a situation where two or more threads are blocked forever, waiting for each other to release a resource. Deadlocks can occur when threads acquire locks without any ordering.
Here’s an example of a potential deadlock:
import threading
lock1 = threading.Lock()
lock2 = threading.Lock()
def thread_1():
with lock1:
with lock2:
print('Thread 1')
def thread_2():
with lock2:
with lock1:
print('Thread 2')
t1 = threading.Thread(target=thread_1)
t2 = threading.Thread(target=thread_2)
t1.start()
t2.start()
t1.join()
t2.join()
# Output:
# May cause a deadlock
In this example, Thread 1
acquires lock1
and then tries to acquire lock2
, while Thread 2
acquires lock2
and then tries to acquire lock1
. If both threads start at the same time, they can end up waiting for each other to release the locks, leading to a deadlock.
Understanding these common issues with Python threading and how to avoid them can help you write more robust multi-threaded programs. In the next sections, we’ll discuss the fundamental concepts of threading and its applications in real-world Python projects.
Understanding Threading: The Fundamentals
To fully grasp the concept of threading in Python, it’s essential to understand the basics of threading and its role in concurrent programming. Threading is a technique for decoupling tasks which are not sequentially dependent. It allows multiple activities to run concurrently in the same program.
What is Threading?
In simple terms, a thread is a separate flow of execution. This means that your program will have two things happening at once. But for most Python 3 implementations the different threads do not actually execute at the same time, they merely appear to.
Threads are lighter than processes, and share the same memory space, which allows for easier and faster data sharing. This shared memory space can also lead to conflicts when multiple threads access or manipulate the same data simultaneously, hence the need for concepts like locks and semaphores.
Here’s a simple example of threading in Python:
import threading
def print_numbers():
for i in range(10):
print(i)
def print_letters():
for letter in 'abcdefghij':
print(letter)
thread1 = threading.Thread(target=print_numbers)
thread2 = threading.Thread(target=print_letters)
thread1.start()
thread2.start()
thread1.join()
thread2.join()
# Output:
# 0
# a
# 1
# b
# 2
# c
# ...and so on...
In this example, we create two threads that run concurrently, printing numbers and letters respectively.
Threads vs Processes
While threads are part of the same process and share the same memory space, processes are completely separate from each other. Each process has its own memory space, which means that you need to use inter-process communication (IPC) mechanisms to share data between processes.
Processes are safer than threads as they don’t share memory with other processes. However, creating a new process requires more resources than creating a new thread, so they are more heavyweight.
In Python, you can use the multiprocessing
module to create processes, and the threading
module to create threads.
Understanding the fundamental concepts of threading and the difference between threads and processes is crucial to mastering Python threading and concurrent programming.
Python Threading: Beyond the Basics
Understanding Python threading is just the beginning. The real power of threading comes to light when applied to real-world Python projects. Let’s take a look at a couple of examples where threading can be particularly useful.
Threading in Web Scraping
Web scraping is a task that can greatly benefit from Python threading. When scraping data from multiple URLs, each URL can be handled by a separate thread, significantly speeding up the data gathering process.
Here’s a simplified example of how you might use threading to scrape multiple web pages:
import threading
import requests
from bs4 import BeautifulSoup
def scrape(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.title.text)
urls = ['http://example.com/page1', 'http://example.com/page2', 'http://example.com/page3']
for url in urls:
threading.Thread(target=scrape, args=(url,)).start()
In this example, we create a new thread for each URL we want to scrape. Each thread makes a request to its assigned URL, parses the HTML response, and prints the title of the page.
Threading in GUI Applications
Python threading is also useful in GUI applications. For instance, you might have a GUI application where you want to perform a long-running task without freezing the user interface. By running the task in a separate thread, your application remains responsive.
Here’s an example using Python’s tkinter
library:
import threading
import tkinter as tk
import time
def long_running_task():
time.sleep(5) # simulate long-running task
print('Task finished')
def on_button_click():
threading.Thread(target=long_running_task).start()
root = tk.Tk()
button = tk.Button(root, text='Start task', command=on_button_click)
button.pack()
root.mainloop()
In this example, clicking the button starts a long-running task in a separate thread, allowing the GUI to remain responsive.
Further Resources for Mastering Python Threading
If you’re interested in learning more about Python threading, here are some additional resources you might find helpful:
- Python Modules Efficiency Techniques – Dive deep into modules for scientific computing and data visualization.
Data Serialization Made Easy with Python Pickle – Learn how to store and retrieve Python objects using pickle.
Python Multiprocessing for Efficient Computations – Discover how to leverage multiple processors for efficient tasks.
Python’s official threading documentation provides an in-depth look at the threading module.
Real Python’s guide to threading is a comprehensive tutorial with plenty of examples.
Python Concurrency: An Intro to Threads and Processes by Toptal offers an introduction to threading and concurrent programming in Python.
Remember, mastering threading in Python is about practice. The more you use threading in your projects, the more comfortable you’ll become with it. Happy threading!
Wrapping Up: Mastering Threading in Python
In this comprehensive guide, we’ve delved into the world of threading in Python, a powerful tool for managing concurrent tasks in your programs.
We began with the basics, learning how to use Python’s built-in threading
module to create and manage threads. We then explored more advanced topics, such as thread synchronization and communication between threads. We also tackled common issues that you might encounter when using threading, such as race conditions and deadlocks, providing solutions to help you overcome these challenges.
We also looked at alternative approaches to concurrent programming in Python, such as multiprocessing and asyncio, giving you a sense of the broader landscape of tools for managing concurrent tasks in Python. Here’s a quick comparison of these methods:
Method | Ideal For | Complexity |
---|---|---|
Threading | IO-bound tasks, Shared memory space | Moderate |
Multiprocessing | CPU-bound tasks, Separate memory space | High |
Asyncio | IO-bound tasks, Single-threaded concurrency | High |
Whether you’re a beginner just starting out with threading in Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python threading and its capabilities.
With its balance of versatility, efficiency, and power, threading is a valuable tool in any Python developer’s toolkit. Happy threading!