Python Pickle Module | Usage Guide (With Examples)
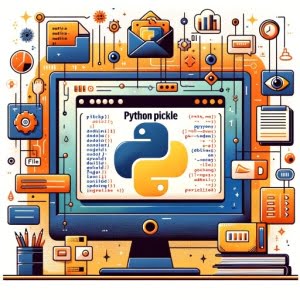
Ever felt puzzled by Python’s pickle module? Imagine it as a time capsule, a tool that allows you to preserve complex Python objects for future use.
This guide is your roadmap to mastering Python’s pickle module. We’ll unravel the mystery of pickle, taking you from basic usage to advanced techniques, all while keeping things light, engaging, and easily digestible.
So, grab your coding hat, and let’s dive into the world of Python serialization with pickle!
TL;DR: What Is Python Pickle?
Python pickle is a module used for serializing and deserializing Python objects. It’s a way to convert Python objects into a format that can be easily written to, and read from, disk or sent over a network.
Here’s a simple example:
import pickle
data = {'key': 'value'}
pickle_data = pickle.dumps(data)
print(pickle.loads(pickle_data))
# Output:
# {'key': 'value'}
In this code, we first import the pickle module. We then create a dictionary and use pickle.dumps()
to serialize the dictionary. The serialized data is stored in pickle_data
. Finally, we use pickle.loads()
to deserialize the data, bringing our dictionary back to life.
Intrigued? Keep reading to gain a more detailed understanding and explore advanced usage scenarios of Python’s pickle module.
Table of Contents
- The Pickle Basics: Serialization and Deserialization
- Pickling Python’s Custom Classes
- Exploring Alternatives to Python Pickle
- Troubleshooting Common Pickle Problems
- Understanding Python’s Object Model and Serialization
- Pickle Beyond Python: Real-world Applications
- Further Resources for Python Modules
- Pickle Unpacked: A Recap
The Pickle Basics: Serialization and Deserialization
Python’s pickle module is all about serialization and deserialization. But what does that mean? Serialization is the process of transforming Python objects into a format (a stream of bytes) that can be saved to disk or sent over a network. Deserialization is the reverse process, converting that stream of bytes back into a Python object.
Let’s see this in action with a simple dictionary.
import pickle
# A simple dictionary
my_dict = {'Python': 'Fun', 'Pickle': 'Tasty'}
# Serialize the dictionary
serialized_dict = pickle.dumps(my_dict)
print(serialized_dict)
# Output:
# b'\x80\x04\x95\x1c\x00\x00\x00\x00\x00\x00\x00}\x94(\x8c\x06Python\x94\x8c\x03Fun\x94\x8c\x06Pickle\x94\x8c\x05Tasty\x94u.'
# Deserialize the dictionary
deserialized_dict = pickle.loads(serialized_dict)
print(deserialized_dict)
# Output:
# {'Python': 'Fun', 'Pickle': 'Tasty'}
In this example, we first created a dictionary my_dict
. We then serialized it using pickle.dumps()
, which returned a byte stream. This stream was printed, showcasing the serialized form of our dictionary. Finally, we used pickle.loads()
to deserialize the byte stream back into a Python dictionary.
One major advantage of pickle is its ability to handle complex Python objects, including nested structures like lists within dictionaries. However, pickle is not without its pitfalls. For instance, pickle data can be manipulated to execute arbitrary code during deserialization, posing a potential security risk. Therefore, it’s crucial to only unpickle data you trust. In the following sections, we’ll delve deeper into more advanced uses and alternatives to pickle.
Pickling Python’s Custom Classes
Pickle’s real power shines when dealing with more complex Python objects like custom classes. Let’s create a simple custom class and see how pickle handles it.
import pickle
class MyClass:
def __init__(self, name):
self.name = name
# Instantiate MyClass
my_instance = MyClass('Pickle Master')
# Serialize the object
serialized_obj = pickle.dumps(my_instance)
print(serialized_obj)
# Output:
# b'\x80\x04\x95\x1b\x00\x00\x00\x00\x00\x00\x00\x8c\x08__main__\x94\x8c\x07MyClass\x94\x93\x94)\x81\x94}\x94\x8c\x04name\x94\x8c\x0cPickle Master\x94sb.'
# Deserialize the object
deserialized_obj = pickle.loads(serialized_obj)
print(deserialized_obj.name)
# Output:
# 'Pickle Master'
In this example, we created a custom class MyClass
with a single attribute name
. We then created an instance of MyClass
, serialized it with pickle.dumps()
, and printed the resulting byte stream. Finally, we deserialized the byte stream back into a Python object using pickle.loads()
. Notice that the deserialized object retains its state (the name
attribute).
While pickle’s ability to handle custom classes is powerful, it’s important to remember that it’s not without its quirks. For instance, pickle does not store class or function definitions, only the object’s state. If the class or function definition changes between pickling and unpickling, the deserialized object may behave differently. As a best practice, ensure that your code’s environment is consistent when pickling and unpickling.
Exploring Alternatives to Python Pickle
While Python’s pickle module is a powerful tool for serialization and deserialization, it’s not the only game in town. Let’s explore some alternative approaches to serialize Python objects, such as the json
module and third-party libraries like dill
.
Embracing JSON for Serialization
The json
module is a popular alternative to pickle for serializing simple Python data types. Its main advantage is interoperability – JSON data can be read by virtually any programming language. Here’s a simple example:
import json
# A simple dictionary
my_dict = {'Python': 'Fun', 'Pickle': 'Tasty'}
# Serialize the dictionary
serialized_json = json.dumps(my_dict)
print(serialized_json)
# Output:
# '{"Python": "Fun", "Pickle": "Tasty"}'
# Deserialize the JSON
deserialized_json = json.loads(serialized_json)
print(deserialized_json)
# Output:
# {'Python': 'Fun', 'Pickle': 'Tasty'}
In this example, we used json.dumps()
to serialize a dictionary into a JSON formatted string and json.loads()
to deserialize the JSON back into a Python dictionary. However, unlike pickle, the json
module can’t handle complex Python objects like custom classes out-of-the-box.
Dill: Pickle’s Powerful Cousin
For more complex scenarios, you might want to consider dill
, a third-party library that extends pickle’s capabilities. dill
can serialize a wider range of Python objects, including functions and classes.
import dill
def my_function(name):
return f'Hello, {name}'
# Serialize the function
serialized_func = dill.dumps(my_function)
# Deserialize the function
deserialized_func = dill.loads(serialized_func)
print(deserialized_func('World'))
# Output:
# 'Hello, World'
In this example, we used dill.dumps()
to serialize a function and dill.loads()
to deserialize it. Note that dill
can handle a wider variety of Python objects than pickle, but it’s a third-party library and may not be available in all environments.
Method | Advantages | Disadvantages |
---|---|---|
Pickle | Handles complex Python objects | Potential security risks |
JSON | Interoperability with other languages | Limited to simple data types |
Dill | Handles a wide range of Python objects | Third-party library |
While pickle is a versatile tool for Python serialization, it’s important to consider the alternative methods available based on your specific use case. Whether you choose pickle, JSON, or dill, each comes with its own set of advantages and trade-offs.
Troubleshooting Common Pickle Problems
While Python’s pickle module is a powerful tool, it’s not without its quirks. Let’s discuss some common issues you might encounter during pickling and how to resolve them.
Dealing with UnpicklingErrors
One common issue is the UnpicklingError
, which occurs when pickle encounters invalid data during deserialization. This can happen if the pickled data is corrupted or if it’s not pickled data at all.
import pickle
# Corrupted pickle data
bad_data = b'not pickle data'
try:
pickle.loads(bad_data)
except pickle.UnpicklingError:
print('Failed to unpickle data.')
# Output:
# Failed to unpickle data.
In this example, we tried to unpickle a byte string that wasn’t pickled data, resulting in an UnpicklingError
. Always make sure your data is valid before attempting to unpickle it.
Pickling Errors with Certain Types of Objects
Pickle can handle most Python objects, but there are exceptions. For instance, pickle can’t serialize functions with closures or objects that reference non-picklable objects.
import pickle
# A function with a closure
def outer_func(name):
def inner_func():
return f'Hello, {name}'
return inner_func
my_func = outer_func('World')
try:
pickle.dumps(my_func)
except TypeError as e:
print(e)
# Output:
# cannot pickle 'function' object
In this example, we tried to pickle a function with a closure, resulting in a TypeError
. If you need to serialize such objects, consider using third-party libraries like dill
that support a wider range of Python objects.
Remember, while pickle is a powerful tool, it’s not a one-size-fits-all solution. Always consider the nature of the data you’re working with and choose the right tool for the job.
Understanding Python’s Object Model and Serialization
Before we delve deeper into the mechanics of Python’s pickle module, it’s crucial to understand the fundamentals that underpin it – Python’s object model and the concept of serialization.
Python’s Object Model
Everything in Python is an object, from simple integers to complex custom classes. Each object in Python has a unique id, a type, and a value. The id is a unique identifier for the object, the type defines what operations can be performed on the object, and the value is the data stored in the object.
# A simple integer
num = 42
print(id(num))
print(type(num))
print(num)
# Output:
# 140733193383680
# <class 'int'>
# 42
In this example, we created an integer object and used id()
, type()
, and print()
to display its id, type, and value, respectively.
The Concept of Serialization
Serialization is the process of transforming an object’s state into a format that can be stored or transmitted and then reconstructed later. In the context of Python, serialization allows us to convert Python objects into a byte stream that can be saved to disk or sent over a network. Deserialization is the reverse process, converting the byte stream back into a Python object.
import pickle
# A simple dictionary
my_dict = {'Python': 'Fun', 'Pickle': 'Tasty'}
# Serialize the dictionary
serialized_dict = pickle.dumps(my_dict)
# Deserialize the dictionary
deserialized_dict = pickle.loads(serialized_dict)
print(deserialized_dict)
# Output:
# {'Python': 'Fun', 'Pickle': 'Tasty'}
In this example, we used pickle.dumps()
to serialize a dictionary into a byte stream and pickle.loads()
to deserialize the byte stream back into a dictionary.
Understanding Python’s object model and the concept of serialization is key to mastering the pickle module. With these fundamentals in mind, let’s explore how pickle leverages these concepts to serialize and deserialize Python objects.
Pickle Beyond Python: Real-world Applications
Python’s pickle module isn’t just a fascinating tool for developers to play with – it has practical applications in real-world scenarios, particularly in data storage and networking.
Pickle in Data Storage
Pickle’s serialization capabilities make it a powerful tool for data storage. By converting Python objects into a byte stream, pickle allows you to store complex data structures like nested dictionaries and custom classes in a database or a file on disk. You can then retrieve the data later and convert it back into a Python object, preserving the object’s state.
import pickle
class MyClass:
def __init__(self, name):
self.name = name
# Instantiate MyClass
my_instance = MyClass('Pickle Master')
# Serialize the object and write it to a file
with open('my_instance.pkl', 'wb') as f:
pickle.dump(my_instance, f)
# Later on...
# Read the file and deserialize the object
with open('my_instance.pkl', 'rb') as f:
loaded_instance = pickle.load(f)
print(loaded_instance.name)
# Output:
# 'Pickle Master'
In this example, we created an instance of a custom class and used pickle.dump()
to serialize it and write it directly to a file. We then used pickle.load()
to read the file and deserialize the object, preserving its state.
Pickle in Networking
Pickle’s ability to convert Python objects into a byte stream also makes it useful in networking. You can serialize a Python object, send the byte stream over a network, and then deserialize the byte stream back into a Python object on the receiving end.
import pickle
import socket
# A simple dictionary to send over the network
data = {'Python': 'Fun', 'Pickle': 'Tasty'}
# Serialize the dictionary
serialized_data = pickle.dumps(data)
# Send the serialized data over a network (omitting the networking code for brevity)
# socket.send(serialized_data)
# On the receiving end...
# Receive the serialized data (omitting the networking code for brevity)
# received_data = socket.recv(1024)
# Deserialize the data
# deserialized_data = pickle.loads(received_data)
# print(deserialized_data)
# Output (on the receiving end):
# {'Python': 'Fun', 'Pickle': 'Tasty'}
In this example, we serialized a dictionary and sent it over a network. On the receiving end, we received the byte stream and deserialized it back into a dictionary. Note that we omitted the actual networking code for brevity.
While pickle is a powerful tool, it’s not the only serialization tool available in Python. We encourage you to explore related concepts like Python’s json
module and database storage, and to delve deeper into Python’s rich ecosystem of data serialization tools. Whether you’re storing data, sending it over a network, or just playing with Python objects, understanding serialization is a valuable skill in a developer’s toolkit.
Further Resources for Python Modules
To further your knowledge on Python Modules, consider using the following resources:
- Python Modules Best Practices Simplified – Explore modules that enhance your ability to work with APIs and web services.
Python Deep Copy: Cloning Objects with deepcopy() – Dive deep into object cloning and copying techniques in Python.
Multithreading in Python: A Quick Introduction – Discover Python’s “threading” module for multithreading.
Python Pickle Module – A Guide for Python’s pickle module, an essential serialization and deserialization tool.
Serialization Techniques in Python – Medium article that provides an overview of various serialization techniques in Python.
Mastering Python Serialization and Pickling – An In-Depth Guide to master the art of serialization and pickling in Python.
Empowering yourself with an understanding of Python Modules will allow you to write more modular and maintainable code.
Pickle Unpacked: A Recap
We’ve journeyed through the intricacies of Python’s pickle module, from its basic usage to advanced techniques. We’ve seen how pickle can serialize and deserialize Python objects, transforming them into a byte stream that can be stored or transmitted and then reconstructed later. We’ve also explored common issues and solutions, such as dealing with UnpicklingError
and pickling errors with certain types of objects.
# A quick recap of pickle in action
import pickle
class MyClass:
def __init__(self, name):
self.name = name
# Instantiate MyClass
my_instance = MyClass('Pickle Master')
# Serialize the object
serialized_obj = pickle.dumps(my_instance)
# Deserialize the object
deserialized_obj = pickle.loads(serialized_obj)
print(deserialized_obj.name)
# Output:
# 'Pickle Master'
In this code, we created an instance of a custom class, serialized it using pickle.dumps()
, and then deserialized it using pickle.loads()
. The deserialized object preserved its state, demonstrating pickle’s power.
We’ve also delved into alternative approaches to Python object serialization, such as the json
module and third-party libraries like dill
. Each method has its own strengths and trade-offs, and the choice depends on your specific use case.
Method | Advantages | Disadvantages |
---|---|---|
Pickle | Handles complex Python objects | Potential security risks |
JSON | Interoperability with other languages | Limited to simple data types |
Dill | Handles a wide range of Python objects | Third-party library |
Whether you’re dealing with simple data types or complex custom classes, Python offers a rich ecosystem of serialization tools. Mastering these tools, starting with pickle, is a valuable skill in any Python developer’s arsenal.