Python Wheel | Easy Software Packaging and Distribution
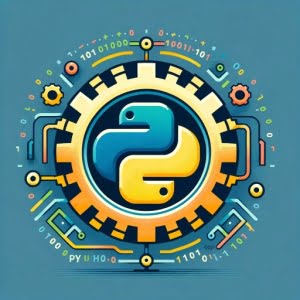
Are you struggling with distributing your Python software efficiently? You’re not alone. Many developers find this task challenging, but there’s a tool that can make this process smoother.
Like a well-oiled machine, Python wheels can streamline your software distribution. These wheels are a packaging standard that allows for faster installations and a more efficient distribution process.
In this guide, we’ll help you understand what Python wheels are, how to create them, and how to use them. We’ll explore everything from the basics of creating a Python wheel to more advanced topics, as well as alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering Python Wheel!
TL;DR: How Do I Create a Python Wheel?
To create a Python wheel, you first need to install the ‘wheel’ package using pip. Then, you can use the
'bdist_wheel'
command to create a wheel distribution. Here’s a simple example:
pip install wheel
python setup.py bdist_wheel
In this example, we first install the ‘wheel’ package using pip. Then, we use the 'bdist_wheel'
command to create a wheel distribution. This command will generate a .whl file, which is the wheel distribution of your Python software.
This is a basic way to create a Python wheel, but there’s much more to learn about Python wheels and their usage. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Creating a Python Wheel: The Basics
Creating a Python wheel is a straightforward process, but it requires a bit of understanding. Let’s break it down step by step.
The Setup.py File
The first thing you need is a setup.py file. This file is used by setuptools to package your Python software. Here’s a basic example of what a setup.py file might look like:
from setuptools import setup
setup(
name='Your-Package-Name',
version='0.1',
packages=['your_package'],
)
In this setup.py file, we define the name and version of our package, as well as the packages included in our software.
Creating the Wheel
Once you have your setup.py file, you can create a Python wheel using the ‘bdist_wheel’ command. But before you do that, you need to ensure that the ‘wheel’ package is installed. You can install it using pip:
pip install wheel
After installing the ‘wheel’ package, you can create your Python wheel:
python setup.py bdist_wheel
This command will generate a .whl file in a dist folder. This .whl file is the wheel distribution of your Python software.
Advantages and Potential Pitfalls
Creating a Python wheel has many advantages. It allows for faster installations and can include compiled extensions, making your software more efficient. But there are also potential pitfalls. For instance, a wheel created on one platform may not work on another, and it may include unnecessary files if not properly configured. We’ll look at how to address these issues in the advanced and troubleshooting sections.
Advanced Python Wheel Creation
As you become more comfortable with creating basic Python wheels, you can explore more advanced topics. These include creating universal wheels, pure Python wheels, and platform wheels.
Creating Universal Wheels
Universal wheels are wheels that are compatible with both Python 2 and Python 3. To create a universal wheel, you need to add a setup.cfg file with the following content to your project:
[bdist_wheel]
universal = 1
After adding this file, you can create your universal wheel just like you would create a basic Python wheel:
python setup.py bdist_wheel
Pure Python Wheels vs Platform Wheels
There are two types of Python wheels: pure Python wheels and platform wheels. Pure Python wheels contain only Python code and are platform-independent, while platform wheels contain compiled code and are platform-dependent.
You can tell whether a wheel is pure Python or platform by looking at its filename. A pure Python wheel’s filename ends with ‘py2.py3-none-any.whl’, while a platform wheel’s filename ends with something like ‘cp36-cp36m-macosx_10_6_intel.whl’.
Here’s an example of creating a platform wheel:
python setup.py bdist_wheel --plat-name macosx_10_6_intel
This command will generate a platform wheel that’s compatible with the specified platform.
Best Practices
When creating Python wheels, there are a few best practices to keep in mind. Always ensure that your wheel includes only the necessary files and that it’s compatible with the platforms you’re targeting.
If your software is compatible with both Python 2 and Python 3, consider creating a universal wheel. And if your software contains only Python code, create a pure Python wheel to ensure maximum compatibility.
Exploring Alternative Python Distribution Methods
While Python wheels have become the standard for Python software distribution, there are other methods you might consider, particularly if you’re distributing source code or dealing with complex dependencies. Two of these methods are source distributions (sdist) and eggs.
Source Distributions (sdist)
Source distributions package your Python software’s source code into a .tar.gz file. To create a source distribution, you can use the ‘sdist’ command:
python setup.py sdist
This command will generate a .tar.gz file in a dist folder. This file contains the source code of your Python software.
# Output:
# running sdist
# running egg_info
# writing top-level names to sample.egg-info/top_level.txt
# writing sample.egg-info/PKG-INFO
# writing dependency_links to sample.egg-info/dependency_links.txt
# reading manifest file 'sample.egg-info/SOURCES.txt'
# writing manifest file 'sample.egg-info/SOURCES.txt'
# running check
# creating sample-1.0
# creating sample-1.0/sample.egg-info
# making hard links in sample-1.0...
# hard linking setup.py -> sample-1.0
# hard linking sample.egg-info/PKG-INFO -> sample-1.0/sample.egg-info
# hard linking sample.egg-info/SOURCES.txt -> sample-1.0/sample.egg-info
# hard linking sample.egg-info/dependency_links.txt -> sample-1.0/sample.egg-info
# hard linking sample.egg-info/top_level.txt -> sample-1.0/sample.egg-info
# Writing sample-1.0/setup.cfg
# creating dist
# Creating tar archive
# removing 'sample-1.0' (and everything under it)
While source distributions are a great way to distribute source code, they require the end user to compile any extensions and install any dependencies, which can be a disadvantage compared to wheels.
Python Eggs
Python eggs are a legacy distribution format that predates wheels. They include compiled extensions and metadata, similar to wheels.
Creating an egg is similar to creating a wheel or source distribution:
python setup.py bdist_egg
This command will generate a .egg file in a dist folder.
While eggs are less common today, they can still be useful in certain situations. However, they have been largely replaced by wheels due to their lack of support for Python 3 and other limitations.
Choosing the Right Distribution Method
When distributing your Python software, it’s important to choose the right method. Wheels are the standard and offer many advantages, but source distributions and eggs can be useful in certain situations.
Consider your software’s requirements, your target audience, and the platforms you’re targeting when making your decision.
Troubleshooting Python Wheel Creation
Creating Python wheels is generally a smooth process, but like any technical task, you might encounter issues. Let’s discuss some common problems and their solutions.
Compatibility Issues
One common issue is compatibility. A wheel created on one platform might not work on another. This is particularly relevant for wheels containing compiled extensions.
If you’re encountering compatibility issues, consider creating a pure Python wheel or a universal wheel. You can also specify the platform when creating your wheel:
python setup.py bdist_wheel --plat-name macosx_10_6_intel
This command creates a wheel that’s compatible with the specified platform.
Errors During Wheel Creation
You might also encounter errors during the wheel creation process. These could be due to missing dependencies, errors in your setup.py file, or other issues.
If you’re encountering errors, the first step is to read the error message. Python’s error messages are usually informative and can guide you towards the solution. You might need to install a missing package, fix an error in your setup.py file, or address another issue.
Here’s an example of an error you might encounter and how to solve it:
python setup.py bdist_wheel
# Output:
# error: invalid command 'bdist_wheel'
This error message indicates that the ‘wheel’ package is not installed. You can solve this issue by installing the ‘wheel’ package:
pip install wheel
Tips for Creating Python Wheels
Finally, here are some tips to help you create Python wheels more effectively:
- Always test your wheel on the platforms you’re targeting.
- Include only the necessary files in your wheel to keep it lightweight.
- Use the ‘bdist_wheel’ command instead of the ‘bdist’ command to ensure that your wheel includes compiled extensions.
- If your software is compatible with both Python 2 and Python 3, consider creating a universal wheel.
The Genesis of Python Wheels
To fully appreciate the value of Python wheels, it’s important to understand the history and fundamentals of Python packaging and distribution.
The Evolution of Python Packaging
Python packaging has evolved significantly over the years. In the early days, distributing Python software was a complex task. Developers had to manually manage dependencies and compilation of extensions, which was time-consuming and error-prone.
The introduction of setuptools and distutils simplified the process, but it was still far from perfect. The distribution packages they created, known as eggs and source distributions, had their own limitations. Eggs, for instance, lacked support for Python 3 and had other issues.
The Birth of Python Wheels
Python wheels were introduced to address these limitations. The term ‘wheel’ is a play on the phrase ‘reinventing the wheel’, as the goal was to create a better distribution standard that solved the problems of the existing methods.
Wheels are binary distribution packages, meaning they include compiled code. This allows for faster installations compared to source distributions, which need to compile code during installation. Wheels can also include Python bytecode, which is platform-independent and can be executed faster than source code.
The Advantages of Python Wheels
Python wheels offer several advantages over the previous distribution methods. They allow for faster installations, can include compiled extensions, and are easier to create. They are also platform-independent, meaning a wheel created on one platform can be installed on another (as long as it’s a pure Python wheel).
The Role of Python Wheels in Solving Distribution Problems
Python wheels have played a significant role in simplifying Python software distribution. They’ve made it easier for developers to package their software, and for users to install it. They’ve also improved installation speed and efficiency, making Python software more accessible to a wider audience.
In summary, Python wheels are a result of the continuous evolution of Python packaging and distribution. They’ve addressed many of the problems of previous methods, simplifying and streamlining the distribution process.
Python Wheels and Broader Software Distribution
Python wheels have a significant role in the broader context of Python software distribution. They streamline the process, making software easier to package and faster to install.
Python Wheels and pip Installations
pip, Python’s package installer, uses wheels extensively. When you run a command like pip install numpy
, pip downloads a wheel if available and installs it. This is much faster than downloading and compiling the source code.
pip install numpy
# Output:
# Collecting numpy
# Downloading numpy-1.21.2-cp38-cp38-manylinux_2_12_x86_64.manylinux2010_x86_64.whl (15.8 MB)
# |████████████████████████████████| 15.8 MB 4.2 MB/s
# Installing collected packages: numpy
# Successfully installed numpy-1.21.2
In the output, you can see that pip downloaded a .whl file (a Python wheel) and installed it.
Python Wheels and PyPI
The Python Package Index (PyPI), the official repository for Python software, supports and encourages using wheels. When you upload a package to PyPI, you can upload it as a wheel. This makes it available for pip to install, making your software more accessible to the Python community.
Further Resources for Python Wheel Mastery
To deepen your understanding of Python wheels, here are some additional resources:
- Python Installation Guide for Ubuntu – All you need to know to get started with Python development on Ubuntu.
Installing Python on macOS – A Step-by-Step guide on how to install Python on macOS and set up a Python development environment.
Packaging Python Apps: A Guide to PyInstaller for Developers – Master the art of distributing Python applications as standalone executables with PyInstaller.
Python Packaging User Guide – This guide provides more details on packaging Python software, including creating wheels.
The Wheel project on PyPI – The official project page for the Wheel package on PyPI. It includes detailed documentation and usage examples.
Python Wheels – A website dedicated to Python wheels. It explains why wheels are beneficial and how to use them, and it includes a list of packages that offer wheels.
Wrapping Up: Mastering Python Wheels for Efficient Software Distribution
In this comprehensive guide, we’ve delved deep into the world of Python wheels, a standard for packaging Python software that streamlines distribution and installation.
We began with the basics, learning how to create a Python wheel from a simple setup.py file. We then ventured into more advanced territory, exploring the creation of universal wheels, pure Python wheels, and platform wheels. We also provided practical code examples and highlighted potential pitfalls to watch out for.
Along the way, we tackled common challenges you might face when creating Python wheels, such as compatibility issues and errors during the wheel creation process. We provided solutions and workarounds for each issue, and offered tips to help you create Python wheels more effectively.
We also looked at alternative approaches to Python software distribution, giving you a broader perspective.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Python Wheels | Fast installations, can include compiled extensions | Compatibility issues if not properly configured |
Source Distributions (sdist) | Great for distributing source code | Requires end user to compile extensions and install dependencies |
Python Eggs | Includes compiled extensions and metadata | Lacks support for Python 3, other limitations |
Whether you’re just starting out with Python wheels or you’re looking to level up your software distribution skills, we hope this guide has given you a deeper understanding of Python wheels and their capabilities.
With their balance of speed, efficiency, and ease of use, Python wheels are a powerful tool for Python software distribution. Now, you’re well equipped to enjoy those benefits. Happy coding!