PyInstaller | How To Make Standalone Python Executables
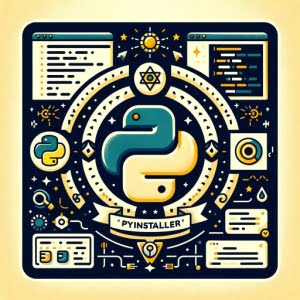
Are you finding it challenging to convert your Python programs into standalone executables? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled craftsman, PyInstaller is a handy utility that can seamlessly mold your Python code into a standalone executable. These executables can run on any system, even those without Python installed.
This guide will walk you through using PyInstaller to create standalone Python executables. We cover installation, basic use, and advanced techniques, perfect for beginners and seasoned Python experts alike.
So, let’s dive in and start mastering PyInstaller!
TL;DR: How Do I Use PyInstaller to Create a Standalone Executable?
To create a standalone executable using PyInstaller, you can use it from the command line. Navigate to your program’s directory and run
pyinstaller your_program.py
. This will create a standalone executable in adist
folder.
Here’s a simple example:
# Navigate to the directory containing your_program.py
# Run the following command
pyinstaller your_program.py
# Output:
# A 'dist' folder is created in the current directory, containing your_program as a standalone executable.
In this example, we navigate to the directory containing your_program.py
and run the pyinstaller your_program.py
command. This command tells PyInstaller to create a standalone executable of your_program.py
. The executable is then stored in a newly created dist
folder within the current directory.
This is a basic way to create a standalone executable using PyInstaller. However, there’s much more to learn about PyInstaller, including more detailed instructions and advanced usage scenarios. So, keep reading to become a PyInstaller pro!
Table of Contents
Basic Usage: PyInstaller for Beginners
If you’re new to PyInstaller, don’t worry. We’ll start with the basics. Using PyInstaller from the command line is straightforward. Here’s how you can convert your Python program into a standalone executable.
First, you need to navigate to your program’s directory. You can do this using the cd
command followed by the path to your directory. For instance, if your program is in a directory named ‘my_program’ on your desktop, you would navigate to it like this:
# Change directory to 'my_program' on the desktop
cd Desktop/my_program
Once you’re in the correct directory, you can tell PyInstaller to create a standalone executable of your program. Let’s say your program is named ‘hello_world.py’. You would use the following command:
# Create a standalone executable of 'hello_world.py'
pyinstaller hello_world.py
# Output:
# A 'dist' folder is created in the current directory, containing 'hello_world' as a standalone executable.
In this example, pyinstaller hello_world.py
is the command that tells PyInstaller to create a standalone executable of ‘hello_world.py’. The resulting executable is placed in a ‘dist’ folder within the current directory. If the ‘dist’ folder does not exist, PyInstaller will create it.
And that’s it! You’ve just created your first standalone executable using PyInstaller. In the next section, we’ll explore some more advanced uses of PyInstaller.
Advanced Features of PyInstaller
Once you’ve mastered the basic usage of PyInstaller, you can start exploring its more advanced features. These include creating a single file executable, including additional files, and specifying an icon for your executable.
Single File Executable
By default, PyInstaller creates a directory of files for each program. However, you can instruct it to bundle everything into a single executable file with the --onefile
option. Here’s how you can do it:
# Create a single file executable of 'hello_world.py'
pyinstaller --onefile hello_world.py
# Output:
# A 'dist' folder is created in the current directory, containing 'hello_world' as a single file executable.
Including Additional Files
Sometimes, your program may depend on additional files such as images or data files. You can include these files in your executable using the --add-data
option. The syntax is source;destination
.
# Include 'image.png' in the executable
pyinstaller --add-data 'image.png;.' hello_world.py
# Output:
# A 'dist' folder is created in the current directory, containing 'hello_world' as an executable along with 'image.png'.
Specifying an Icon
You can also customize the icon of your executable using the --icon
option followed by the path to your icon file.
# Specify an icon for the executable
pyinstaller --icon=my_icon.ico hello_world.py
# Output:
# A 'dist' folder is created in the current directory, containing 'hello_world' with 'my_icon.ico' as its icon.
In this section, we’ve explored some of the more advanced uses of PyInstaller. With these techniques, you can create more sophisticated standalone executables tailored to your specific needs.
Alternative Tools: cx_Freeze & py2exe
While PyInstaller is a powerful tool, it’s not the only game in town. Other utilities, such as cx_Freeze and py2exe, also allow you to create standalone executables from Python programs. Let’s take a brief look at these alternatives and see how they stack up against PyInstaller.
Exploring cx_Freeze
cx_Freeze is similar to PyInstaller in many ways. It supports both Python 2 and Python 3 and can create executables for Windows, Mac, and Linux. However, it’s known for its simplicity and ease of use. Here’s a basic example of how to use cx_Freeze:
# cx_Freeze setup script
from cx_Freeze import setup, Executable
setup(name='hello_world',
version='0.1',
description='My GUI application!',
executables=[Executable('hello_world.py')])
# Command to run
# python setup.py build
# Output:
# A 'build' directory is created containing the standalone executable.
In this example, we create a setup script for cx_Freeze and then run python setup.py build
to create the executable. The resulting executable is placed in a ‘build’ directory.
Diving into py2exe
py2exe, on the other hand, is a utility that can convert Python scripts into executable Windows programs. However, it only supports Python 2. Here’s a basic example of how to use py2exe:
# py2exe setup script
from distutils.core import setup
import py2exe
setup(console=['hello_world.py'])
# Command to run
# python setup.py py2exe
# Output:
# A 'dist' directory is created containing the standalone executable.
In this example, we create a setup script for py2exe and then run python setup.py py2exe
to create the executable. The resulting executable is placed in a ‘dist’ directory.
Comparing PyInstaller, cx_Freeze, and py2exe
While all three utilities allow you to create standalone executables, they have their strengths and weaknesses. PyInstaller is known for its robustness and support for many libraries, while cx_Freeze is praised for its simplicity. py2exe, while only supporting Python 2, is a popular choice for creating Windows executables due to its ease of use.
Ultimately, the best tool depends on your specific needs and the complexity of your Python program.
Troubleshooting Issues with PyInstaller
While PyInstaller is a powerful tool, you may encounter some issues when using it. Some common problems include missing modules and compatibility issues. In this section, we’ll discuss these issues and provide solutions to help you overcome them.
Missing Modules
One common issue is PyInstaller failing to include some modules that your program depends on. This can happen if PyInstaller is unable to detect these dependencies automatically. If your program imports a module dynamically (i.e., the module name is not explicitly mentioned in the source code), PyInstaller may miss it.
To solve this, you can use the --hidden-import
option followed by the name of the module.
# Include a hidden import
pyinstaller --hidden-import=missing_module hello_world.py
# Output:
# A 'dist' folder is created in the current directory, containing 'hello_world' as an executable with 'missing_module' included.
In this example, --hidden-import=missing_module
tells PyInstaller to include ‘missing_module’ in the executable, even if it’s not able to detect it automatically.
Compatibility Issues
Another common issue is compatibility problems with certain libraries. Some libraries may use features that are not compatible with PyInstaller, causing the creation of the executable to fail.
In such cases, you can often find a workaround by searching for your issue along with ‘PyInstaller’ on online forums or issue trackers. The PyInstaller community is active and helpful, and it’s likely that someone else has encountered the same issue and found a solution.
Remember, PyInstaller is a powerful tool, but like any tool, it may require some tweaking and troubleshooting to get it to work perfectly with your specific program. With these tips, you’ll be well-equipped to tackle any issues that come your way.
Core Concepts of PyInstaller
To fully master PyInstaller, it helps to understand how it works. At its core, PyInstaller does two things: it collects your program’s dependencies and bundles them into an executable.
Dependency Collection
When you run PyInstaller, it first analyzes your Python script to find all the modules it imports. It does this by actually running your script (in a controlled manner) and observing which modules get imported. This is why PyInstaller can handle complex imports that static analysis tools might miss.
Once PyInstaller knows which modules your script needs, it collects these modules, along with their dependencies, into a folder. Here’s a simplified example of what this process might look like:
# Running PyInstaller on your script
pyinstaller hello_world.py
# Output:
# PyInstaller creates a 'build' directory, which contains a 'hello_world' directory, which in turn contains all the modules and dependencies needed by 'hello_world.py'.
In this example, pyinstaller hello_world.py
instructs PyInstaller to analyze hello_world.py
and collect its dependencies. The dependencies are placed in a ‘build/hello_world’ directory.
Bundling into an Executable
After collecting the dependencies, PyInstaller then bundles them, along with your script, into an executable. This executable contains a small bootstrap code and a bundled Python interpreter. When run, the bootstrap code unpacks the bundled interpreter and dependencies into a temporary folder and runs your script.
# The resulting executable from the previous step
./dist/hello_world
# Output:
# The 'hello_world' executable runs and produces the same output as the original 'hello_world.py' script.
In this example, running ./dist/hello_world
executes the standalone hello_world
program, which behaves exactly as the original hello_world.py
script.
The Power of Standalone Executables
So, why go through all this trouble to create standalone executables? The main advantage is that they can run on any system, even if Python is not installed. This makes it easy to distribute your Python programs to end users, who may not have Python installed or may not know how to install Python packages. It’s a powerful way to share your Python programs with the world.
Use Cases of Standalone Executables
Standalone executables are not just about running Python programs on systems without Python installed. They have wider applications, especially when it comes to software distribution and installation.
Software Distribution
Creating standalone executables significantly simplifies software distribution. You can share your program as a single file that runs on any system, without requiring the user to install Python or any dependencies. This is particularly useful for distributing software to end users who may not be familiar with Python or its ecosystem.
Software Installation
Standalone executables can also simplify software installation. Instead of requiring users to install Python, then install your program and its dependencies via pip, you can provide a single executable that users can run immediately. This can drastically reduce the complexity of the installation process, making your software more accessible to a wider audience.
Further Resources for Mastering PyInstaller
To delve deeper into PyInstaller and standalone executables, here are a few resources that you might find helpful:
- IOFlood’s Guide to Python Installation on Ubuntu explores the compatibility of Python with Ubuntu and how to ensure a seamless development experience.
Building and Distributing Python Packages with Wheels – Master the art of creating and distributing Python packages in wheel format for efficiency.
Jupyter Notebook Installation Made Easy – A quick tutorial on Jupyter Notebook installation and unleashing its potential for your projects.
The official PyInstaller documentation provides a comprehensive overview of PyInstaller’s features and usage.
The Hitchhiker’s guide to PyInstaller offers a detailed walkthrough of creating standalone executables with PyInstaller.
The Hitchhiker’s Guide to Packaging explores the broader topic of Python packaging, including creating standalone executables.
These resources can provide further insights into PyInstaller and the broader context of Python packaging and distribution.
Wrapping Up: Mastering PyInstaller
In this comprehensive guide, we’ve explored how to use PyInstaller to create standalone executables from Python programs.
We’ve seen how to use PyInstaller from the command line, with a simple command like pyinstaller your_program.py
creating a standalone executable in a ‘dist’ folder. We’ve also delved into more advanced usage, such as creating a single file executable, including additional files, and specifying an icon.
We’ve discussed common issues that you might encounter when using PyInstaller, such as missing modules and compatibility issues, and provided solutions to help you overcome these challenges. And we’ve looked at alternatives to PyInstaller, such as cx_Freeze and py2exe, giving you a sense of the broader landscape of tools for creating standalone executables.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
PyInstaller | Robust, supports many libraries | May require troubleshooting for some programs |
cx_Freeze | Simple and easy to use | Less robust than PyInstaller |
py2exe | Easy to use for Windows executables | Only supports Python 2 |
Whether you’re a beginner just starting out with PyInstaller or an experienced developer looking to level up your PyInstaller skills, we hope this guide has given you a deeper understanding of how to create standalone executables with PyInstaller and the power of this tool. Happy coding!