Python argparse | Command Line Library Tutorial
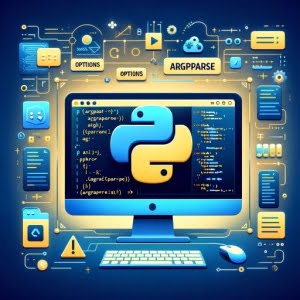
At IOFLOOD, we have began to implement Python’s argparse
module to expand the command-line interface functionality of our datacetner automation scripts. We decided on the argparse module mainly due to the way it handles command-line arguments and options in scripts. To assist our bare metal hosting customers in creating robust command-line interfaces for their scripts, we have provided our personal insights in today’s article.
In this guide, we’ll walk you through the basics of using argparse in Python, all the way up to advanced techniques. Whether you’re a Python newbie or a seasoned pro, we’ve got something for you.
So, let’s dive in and start mastering argparse together.
TL;DR: How Do I Use Argparse in Python?
Argparse in Python is a built-in module used to parse command-line arguments. Here’s a simple example of how to use it:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('--name')
args = parser.parse_args()
print(args.name)
# Output:
# Whatever value you passed in with --name when running the script
In this code block, we first import the argparse module. Then, we create an ArgumentParser object. We define an argument --name
that our script will accept. When we run our script from the command line and pass in --name
followed by a value, our script will recognize it and print out the value.
Stick around for a more in-depth understanding and advanced usage scenarios of argparse in Python!
Table of Contents
- Understanding Argparse: A Beginner’s Guide
- Mastering Argparse: Positional, Optional Arguments, and Sub-commands
- Exploring Alternatives to Argparse
- Troubleshooting Common Argparse Issues
- The ABCs of Command-line Arguments and Argparse
- Argparse: The Foundation of Python CLI Applications
- Argparse in Python: A Recap
Understanding Argparse: A Beginner’s Guide
Argparse is a built-in Python module that makes it easy to write user-friendly command-line interfaces. It understands command-line arguments and can generate help and usage messages for you. Let’s break it down with a simple code example.
import argparse
# Create the parser
parser = argparse.ArgumentParser(description='A simple demonstration of argparse')
# Add an argument
parser.add_argument('--name', type=str, help='Your name')
# Parse the arguments
args = parser.parse_args()
print(f'Hello, {args.name}!')
# Output:
# If you run the script like 'python script.py --name Anton', you'll get 'Hello, Anton!'
In this code, we first import the argparse module. We then create an ArgumentParser object and give it a description. This description will be displayed when someone uses the -h
or --help
option with our script.
Next, we add an argument --name
to our parser. We specify that it should be a string (type=str
) and provide a help message for it. This help message will be displayed alongside the argument in the -h
or --help
output.
Finally, we call parser.parse_args()
to do the actual parsing, and then we can use the parsed arguments in our script. In this case, we’re just printing out a friendly greeting.
Argparse’s advantages include its ability to parse both optional and positional arguments, generate error messages, and create informative help messages. However, it can be a bit verbose for simple scripts, and it doesn’t support subcommands as intuitively as some other libraries.
Mastering Argparse: Positional, Optional Arguments, and Sub-commands
Argparse allows you to handle various types of arguments. These include positional arguments, optional arguments, and even sub-commands. Let’s dive deeper into each one.
Positional Arguments
Positional arguments are the ones that must be included in the correct order. Here’s an example:
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('name')
args = parser.parse_args()
print(f'Hello, {args.name}!')
# Output:
# If you run the script like 'python script.py Anton', you'll get 'Hello, Anton!'
In this code, we define a positional argument name
. Unlike optional arguments, positional arguments don’t need --
before their name. When we run the script, we must provide the name
argument.
Optional Arguments
Optional arguments are those that you can choose to include. They are prefixed with --
or -
. We’ve already seen an example of this with --name
in our basic use case.
Sub-commands
Argparse also supports sub-commands. These are commands that have their own separate arguments.
import argparse
# Create the top-level parser
parser = argparse.ArgumentParser()
subparsers = parser.add_subparsers()
# Create the parser for the 'greet' command
parser_greet = subparsers.add_parser('greet')
parser_greet.add_argument('name')
# Create the parser for the 'goodbye' command
parser_goodbye = subparsers.add_parser('goodbye')
parser_goodbye.add_argument('name')
args = parser.parse_args()
if 'name' in args:
if args.command == 'greet':
print(f'Hello, {args.name}!')
elif args.command == 'goodbye':
print(f'Goodbye, {args.name}!')
# Output:
# If you run the script like 'python script.py greet Anton', you'll get 'Hello, Anton!'
# If you run the script like 'python script.py goodbye Anton', you'll get 'Goodbye, Anton!'
In this code, we first create a top-level parser. We then add subparsers to it. Each subparser is a separate command with its own arguments. In this case, we have a greet
command and a goodbye
command, each with a name
argument.
Understanding these different types of arguments and when to use them will help you make the most of argparse and create more versatile command-line interfaces.
Exploring Alternatives to Argparse
While argparse is an excellent module for parsing command-line arguments in Python, it’s not the only game in town. There are other methods you can use, such as the getopt
module or sys.argv
. Let’s take a look at these alternatives.
Parsing Command-line Arguments with Getopt
Getopt is another module for command-line parsing in Python. It’s more C-like, but it’s also more verbose than argparse.
import getopt
import sys
# Define our options
short_options = 'n:'
long_options = ['name=']
try:
arguments, values = getopt.getopt(sys.argv[1:], short_options, long_options)
except getopt.error as err:
print(str(err))
sys.exit(2)
for current_argument, current_value in arguments:
if current_argument in ('-n', '--name'):
print(f'Hello, {current_value}!')
# Output:
# If you run the script like 'python script.py --name Anton', you'll get 'Hello, Anton!'
In this code, we define our options, then use getopt.getopt()
to parse the command-line arguments. We then loop through the arguments and print a greeting if the --name
option is present.
Using sys.argv for Command-line Parsing
sys.argv
is a list in Python, which contains the command-line arguments passed to the script. It’s a straightforward method for command-line parsing, but it lacks the flexibility and features of argparse and getopt.
import sys
name = sys.argv[1]
print(f'Hello, {name}!')
# Output:
# If you run the script like 'python script.py Anton', you'll get 'Hello, Anton!'
In this code, we simply take the second element of sys.argv
(the first element is the script name) and print a greeting.
While each of these methods has its advantages, argparse is generally the most flexible and powerful. It’s also the most Pythonic and generally recommended for new Python scripts. However, knowing these alternatives can be useful, especially when maintaining older scripts or when argparse is not the right fit for your specific needs.
Troubleshooting Common Argparse Issues
While argparse is a powerful tool, you may encounter some common issues when using it. Let’s discuss these issues and provide some solutions and workarounds.
Handling Incorrect Argument Types
Argparse allows you to specify the type of an argument. If the user provides an argument of the wrong type, argparse will automatically generate an error message. However, you might want to handle these errors yourself to provide more user-friendly messages.
import argparse
def positive_int(value):
ivalue = int(value)
if ivalue <= 0:
raise argparse.ArgumentTypeError(f'{value} is an invalid positive int value')
return ivalue
parser = argparse.ArgumentParser()
parser.add_argument('--number', type=positive_int)
args = parser.parse_args()
print(f'You entered {args.number}')
# Output:
# If you run the script like 'python script.py --number -3', you'll get an error message: '-3 is an invalid positive int value'
In this code, we define a function positive_int()
that checks if a value is a positive integer. If not, it raises an ArgumentTypeError
. We then use this function as the type
for our --number
argument.
Dealing with Missing Arguments
By default, argparse treats arguments as optional unless you tell it otherwise. If you want to make an argument required, you can use the required
keyword argument.
import argparse
parser = argparse.ArgumentParser()
parser.add_argument('--name', required=True)
args = parser.parse_args()
print(f'Hello, {args.name}!')
# Output:
# If you run the script without the --name argument, you'll get an error message: 'the following arguments are required: --name'
In this code, we make the --name
argument required. If we run the script without providing this argument, argparse will automatically generate an error message.
These are just a few of the common issues you might encounter when using argparse. With a good understanding of how argparse works and some careful coding, you can handle these issues and create robust, user-friendly command-line interfaces.
The ABCs of Command-line Arguments and Argparse
Command-line arguments are parameters that are specified when running a script. In Python, these arguments are captured as strings in a list called sys.argv
. The first element of this list is always the name of the script itself. The following elements are the arguments that were passed.
import sys
print(sys.argv)
# Output:
# If you run the script like 'python script.py arg1 arg2', you'll get ['script.py', 'arg1', 'arg2']
In this code, we simply print out sys.argv
. If we run our script with arg1
and arg2
as arguments, we can see that sys.argv
captures these arguments as strings in a list.
While sys.argv
is a straightforward way to grab command-line arguments, it can get messy when you start dealing with multiple arguments, optional arguments, and flags. That’s where argparse comes in.
Argparse: Simplifying Command-line Parsing
Argparse makes it easy to write user-friendly command-line interfaces. It generates help and usage messages and issues errors when users give the program invalid arguments. Argparse is more sophisticated than manually parsing sys.argv
.
With argparse, you can specify the type of each argument, define required arguments, and even add a helpful description for each argument that will be displayed when the user runs your script with the -h
or --help
option.
Argparse simplifies the task of argument parsing and makes your script more robust and user-friendly. It’s a powerful tool that every Python programmer should have in their toolbox.
Argparse: The Foundation of Python CLI Applications
Argparse is more than just a module for parsing command-line arguments. It’s a foundation upon which you can build powerful command-line applications in Python. When you master argparse, you’re not just learning how to parse command-line arguments — you’re also learning the basics of how to interact with users on the command line, how to handle input, and how to generate informative help and error messages.
import argparse
parser = argparse.ArgumentParser(description='A Python CLI application')
parser.add_argument('--name', type=str, help='Your name')
args = parser.parse_args()
print(f'Welcome to the application, {args.name}!')
# Output:
# If you run the script like 'python app.py --name Anton', you'll get 'Welcome to the application, Anton!'
In this code, we’ve created a simple command-line application that greets the user. This is a basic example, but you can imagine how you might expand this to create a more complex application.
Exploring Related Concepts
Once you’re comfortable with argparse, you might want to explore related concepts. For example, you could learn about creating CLI tools in Python, handling user inputs more effectively, or even building interactive command-line interfaces.
Further Resources
To dive deeper into argparse and these related concepts, you might find the following resources helpful:
- Mastering Command Line Arguments in Python – Learn handling command-line inputs effortlessly in Python.
Python Get Environment Variable: Accessing Configuration Values – Learn how to retrieve specific environment variables in Python.
Python args: Handling Variable-Length Argument Lists – Explore args in Python for variable-length argument lists.
The Python documentation on argparse is a comprehensive guide to the module.
The Click library is a powerful tool for creating beautiful command-line interfaces in Python.
Python Prompt Toolkit is a library for building interactive command-line applications.
Remember, mastering argparse and command-line interfaces is a journey. Keep exploring, keep learning, and most importantly, keep coding!
Argparse in Python: A Recap
Throughout this guide, we’ve explored the use of argparse in Python, a powerful module for parsing command-line arguments. We’ve delved into handling different types of arguments, including positional arguments, optional arguments, and sub-commands. We’ve also discussed common issues you might encounter when using argparse, such as handling incorrect argument types and dealing with missing arguments, and provided solutions and workarounds for these issues.
We’ve looked at alternative approaches to command-line argument parsing, such as using the getopt
module or sys.argv
. While these methods have their advantages, we’ve seen that argparse is generally more flexible and powerful, and is the recommended choice for new Python scripts.
import argparse
# An example of argparse usage
parser = argparse.ArgumentParser(description='A Python CLI application')
parser.add_argument('--name', type=str, help='Your name')
args = parser.parse_args()
print(f'Welcome to the application, {args.name}!')
# Output:
# If you run the script like 'python app.py --name Anton', you'll get 'Welcome to the application, Anton!'
In the code above, we’ve created a simple command-line application that greets the user. This is a basic example, but it underscores the power and flexibility of argparse for creating user-friendly command-line interfaces.
Mastering argparse and understanding command-line argument parsing are essential skills for any Python developer. They form the foundation for creating powerful, interactive command-line applications. So keep exploring, keep practicing, and happy coding!