Python List Comprehension | Usage Guide (With Examples)
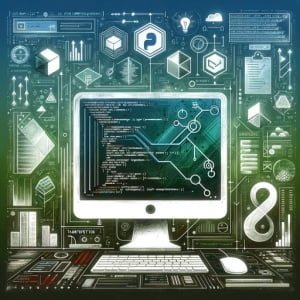
Ever felt like you’re wrestling with Python lists? It’s time to say goodbye to that struggle. Picture a master chef preparing a gourmet meal – that could be you, but with Python lists. This is possible thanks to a feature called list comprehension.
In this article, we will guide you through the ins and outs of Python list comprehension. Just like a culinary journey, we’ll start with the basics and move towards more advanced techniques. By the end, you’ll be serving up Python lists with the flair of a Michelin-starred chef.
So, let’s dive in and get started with mastering Python list comprehension!
TL;DR: How Do I Use Python List Comprehension?
Python list comprehension is a compact method of creating a new list from an existing one. For instance, if you want to create a new list from an old list of numbers you would use,
newList = [n**2 for n in oldList]
. It’s like a formula for creating lists in a more efficient and readable way.
Here’s a simple example:
numbers = [1, 2, 3, 4, 5]
squares = [n**2 for n in numbers]
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In the example above, we create a list of numbers and then use list comprehension to create a new list (squares
), where each element is the square of the corresponding element in the original numbers
list. We then print the new list, and the output is a list of the squares of the numbers 1 to 5.
Don’t stop here, though! There’s a lot more to Python list comprehension than this. Read on for more detailed examples and advanced usage scenarios.
Table of Contents
Intro to Python List Comprehension
List comprehension in Python is a powerful tool, but like any tool, it’s most effective when you know how to use it. Let’s break down the basics.
Step-by-Step Guide to Python List Comprehension
Imagine you have a list of numbers and you want to create a new list containing the square of each number. Here’s how you can do it using list comprehension:
numbers = [1, 2, 3, 4, 5]
squares = [n**2 for n in numbers]
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In the code above, n**2 for n in numbers
is our list comprehension. It reads as ‘for each number n
in our list numbers
, square it (n**2
)’. The result is a new list squares
containing the square of each number from the original list.
Pros and Cons of Using List Comprehension
Like everything in programming, list comprehension has its pros and cons.
Advantages
- Conciseness: List comprehension allows you to create new lists in a single line of code, making your code cleaner and easier to read.
Speed: List comprehension is often faster than traditional loops, especially with larger lists.
Disadvantages
Readability: While list comprehension is concise, it can be harder to read and understand for beginners compared to traditional loops.
Complexity: When used with complex conditions and multiple loops, list comprehension can become convoluted and difficult to debug.
In conclusion, list comprehension is a powerful tool for creating new lists in Python. However, like any tool, it’s important to use it wisely and understand its limitations.
Advanced Uses of List Comprehension
Now that you’re comfortable with the basics, let’s explore some more advanced uses of Python list comprehension. Specifically, we’ll look at using conditions and nested loops.
List Comprehension with Conditions
You can use conditions in list comprehension to filter the elements of the list. Let’s say we want to create a new list containing only the even numbers from our original list. Here’s how we can do it:
numbers = [1, 2, 3, 4, 5]
evens = [n for n in numbers if n % 2 == 0]
print(evens)
# Output:
# [2, 4]
In the code above, n for n in numbers if n % 2 == 0
is our list comprehension. It reads as ‘for each number n
in our list numbers
, include n
in the new list if n
is even’. The result is a new list evens
containing only the even numbers from the original list.
List Comprehension with Nested Loops
You can also use nested loops in list comprehension to create lists from multiple sequences. Let’s say we have two lists and we want to create a new list containing the product of each pair of numbers from the original lists. Here’s how we can do it:
numbers1 = [1, 2, 3]
numbers2 = [4, 5, 6]
products = [n*m for n in numbers1 for m in numbers2]
print(products)
# Output:
# [4, 5, 6, 8, 10, 12, 12, 15, 18]
In the code above, n*m for n in numbers1 for m in numbers2
is our list comprehension. It reads as ‘for each number n
in our first list numbers1
and each number m
in our second list numbers2
, include the product n*m
in the new list’. The result is a new list products
containing the product of each pair of numbers from the original lists.
As you can see, Python list comprehension is a powerful tool that can handle complex tasks with ease. However, keep in mind that as your list comprehension becomes more complex, so does the difficulty of reading and debugging your code. Use these advanced techniques wisely!
Going Beyond: Python List Creation
While list comprehension is a powerful tool, Python offers other ways to create lists as well. Let’s explore some of these alternative approaches, their benefits, drawbacks, and when to use them.
Traditional Loops for List Creation
Before list comprehension, traditional loops were commonly used to create lists in Python. Here’s how we can create a list of squares using a traditional for loop:
numbers = [1, 2, 3, 4, 5]
squares = []
for n in numbers:
squares.append(n**2)
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In the code above, we start by creating an empty list squares
. Then, for each number n
in our list numbers
, we square it (n**2
) and append it to squares
. The result is the same as our earlier list comprehension example.
Pros and Cons of Traditional Loops
- Advantages: Traditional loops are easy to read and understand, especially for beginners. They also allow for more complex operations and easier debugging.
Disadvantages: Compared to list comprehension, traditional loops require more lines of code and are generally slower.
Using the map() Function for List Creation
Python’s built-in map()
function is another alternative for creating lists. Here’s how we can create a list of squares using map()
:
numbers = [1, 2, 3, 4, 5]
squares = list(map(lambda n: n**2, numbers))
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In the code above, map(lambda n: n**2, numbers)
applies the lambda function lambda n: n**2
to each number n
in our list numbers
. The map()
function returns a map object, which we convert to a list using the list()
function. The result is the same as our earlier examples.
Pros and Cons of the map() Function
- Advantages: The
map()
function is fast and allows for parallel processing, making it a good choice for large data sets. Disadvantages: The
map()
function can be harder to read and understand compared to list comprehension or traditional loops, especially for beginners. It also requires a function as input, which can be inconvenient for complex operations.
In conclusion, while list comprehension is a powerful tool for creating lists in Python, it’s not the only tool. Depending on your specific needs and the complexity of your operations, traditional loops or the map()
function might be a better choice.
Troubleshooting Errors in Python Lists
Like any programming concept, Python list comprehension can bring its share of errors and obstacles. Let’s look at some common issues you might encounter and how to resolve them.
Common Errors in List Comprehension
One common mistake is forgetting the square brackets or the syntax inside them. For example, consider the following erroneous code:
numbers = [1, 2, 3, 4, 5]
squares = n**2 for n in numbers
# Output:
# SyntaxError: invalid syntax
In the code above, we forgot to enclose our list comprehension in square brackets. As a result, Python throws a SyntaxError
. The correct code should be squares = [n**2 for n in numbers]
.
Optimization Tips for List Comprehension
While list comprehension is generally faster than traditional loops, there are ways to optimize it further:
- Avoid unnecessary calculations: If your list comprehension involves a complex calculation that is repeated, consider calculating it once and storing the result instead of calculating it each time.
Use built-in functions: Python’s built-in functions are usually faster than custom code. If a built-in function can do what you need, use it!
Limit list size: List comprehension creates a new list in memory. If you’re working with a large data set, consider using a generator expression or a different approach to save memory.
In conclusion, while Python list comprehension is a powerful tool, it’s not without its pitfalls. However, with a good understanding of the syntax and some best practices, you can avoid common errors and optimize your code.
Key Concepts of List Comprehension
To truly master Python list comprehension, it’s important to understand its roots and how it relates to other Python concepts. Let’s dive a little deeper.
The Mathematics Behind List Comprehension
List comprehension in Python is based on a concept from mathematics called set-builder notation. In mathematics, set-builder notation is a shorthand used to describe sets. For example, the set of all x such that x > 0 is written as {x | x > 0}.
Python list comprehension is a way to bring this mathematical concept into programming. In fact, the syntax of list comprehension in Python is very similar to set-builder notation. For example, the list of all squares of n for n in numbers is written as [n**2 for n in numbers]
.
How List Comprehension Relates to Python Lists and Loops
List comprehension is a way to create Python lists using loops and conditions. However, instead of writing out the full loop syntax, list comprehension allows you to create lists in a single line of code.
Here’s a comparison of creating a list of squares using a traditional for loop and using list comprehension:
# Using a traditional for loop
numbers = [1, 2, 3, 4, 5]
squares = []
for n in numbers:
squares.append(n**2)
print(squares)
# Output:
# [1, 4, 9, 16, 25]
# Using list comprehension
numbers = [1, 2, 3, 4, 5]
squares = [n**2 for n in numbers]
print(squares)
# Output:
# [1, 4, 9, 16, 25]
As you can see, both methods produce the same result. However, list comprehension is more concise and often faster, especially with larger lists.
In conclusion, Python list comprehension is a powerful tool that combines the concepts of lists, loops, and conditions into a concise and efficient syntax. Understanding these fundamentals is key to mastering list comprehension.
Practical Uses of List Comprehension
List comprehension isn’t just for small scripts or single tasks. It can be a powerful tool in larger Python projects, helping to keep your code clean and efficient.
For example, you might use list comprehension in a data analysis project to clean your data, filtering out missing or irrelevant values. Or, in a web scraping project, you could use list comprehension to extract specific elements from your scraped data.
# Example of using list comprehension in a web scraping project
# Suppose 'data' is a list of scraped web pages, and we want to extract all URLs
urls = [link for page in data for link in page.get_links() if 'http' in link]
# The resulting 'urls' list contains all URLs from the scraped web pages
In the code above, we use list comprehension with a nested loop and a condition to extract all URLs from a list of scraped web pages. This is just one example of how list comprehension can be used in larger projects.
Exploring Further: Python List Tools
If you’re interested in further exploring Python’s powerful features for handling lists and sequences, consider looking into generators and lambda functions.
- Generators are a type of iterable, like lists. However, unlike lists, they generate their elements on the fly, which can save memory when dealing with large sequences.
Lambda functions are small anonymous functions defined with the
lambda
keyword. They can be used wherever function objects are required, and they’re especially useful in combination with functions likemap()
andfilter()
.
Further Resources for Handling Lists in Python
If you’re interested in learning more about list comprehension in Python and exploring various list methods, here are a few resources that you might find helpful:
- Python Lists: Building Blocks for Effective Coding: Discover Python lists as the fundamental building blocks for effective coding and unlock their potential for efficient data management and manipulation.
Tutorial on Joining a List into a String in Python: This IOFlood tutorial discusses various methods for joining the elements of a list into a string in Python.
Guide to Python List Methods: This guide by IOFlood explores the built-in methods available for lists in Python.
Python List Comprehension: A Comprehensive Guide: A comprehensive guide on w3schools.com explaining list comprehension in Python with examples.
List Comprehension in Python: An article on GeeksforGeeks covering the concept of list comprehension in Python with examples.
List Comprehension: Elegant and Efficient: A Real Python tutorial exploring list comprehension in Python, providing concise and efficient examples.
These resources will provide you with detailed explanations and examples to understand how to join a list into a string and explore the various methods available for working with lists in Python.
Recap: Python List Comprehension
In this guide, we’ve journeyed through the world of Python list comprehension, from its basic usage to more advanced techniques. We’ve seen how it allows us to create new lists from existing ones in a concise and efficient manner, often in just a single line of code.
We’ve also explored alternative methods for creating lists in Python, such as traditional loops and the map()
function, and discussed their pros and cons. While list comprehension is a powerful tool, it’s not always the best choice, and Python provides a variety of tools to suit different needs and preferences.
Here’s a quick comparison of the methods we’ve discussed:
Method | Conciseness | Speed | Readability | Complexity |
---|---|---|---|---|
List Comprehension | High | High | Medium | Medium |
Traditional Loops | Low | Medium | High | Low |
map() Function | Medium | High | Low | High |
Remember, the best tool depends on your specific situation. Consider factors like the size of your data, the complexity of the operation, and the readability of your code.
Finally, we’ve touched on some related Python concepts like generators and lambda functions, and provided resources for further exploration. Python is a rich language with many powerful features, and there’s always more to learn.
In conclusion, Python list comprehension is a versatile and powerful tool that can make your code cleaner, more efficient, and more Pythonic. Whether you’re a Python newbie or a seasoned pro, mastering list comprehension is a valuable addition to your Python toolkit.