Learn Python Functions | Python “def” examples and usage
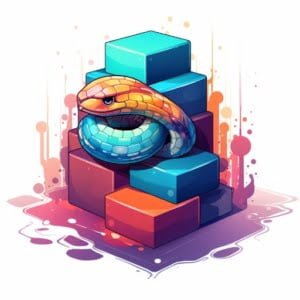
Imagine you’re constructing a building. You wouldn’t start by creating each brick from scratch, would you? Instead, you’d use pre-made bricks, each one identical and perfectly shaped for its purpose. This makes the building process much more efficient and the final structure more robust. This is the essence of Python functions.
Python functions are the ‘building blocks’ of Python programming. They allow you to write a block of code once and reuse it wherever you need it, making your code more organized, more readable, and more efficient. But what exactly are Python functions? How do they work? How can they be used to improve your Python programming skills?
In this comprehensive guide, we’ll delve deep into Python functions. We’ll explore what they are, how to declare and use them, the different types of functions available in Python, and some advanced function concepts. By the end of this guide, you’ll have a solid understanding of Python functions and be ready to use them to enhance your coding efficiency and readability. So, let’s get building!
TL;DR What are Python functions?
Python functions are reusable blocks of code that perform a specific task. They are declared using the
def
keyword and can be called anywhere in your program. Python offers both built-in functions for common tasks and allows you to create your own user-defined functions for custom tasks. Example:
# Define the function
def greet(name):
print(f"Hello, {name}!")
# Call the function
greet("Alice")
Table of Contents
Unveiling Python Functions
Just as bricks are fundamental to a building, Python functions are fundamental to Python programming. But what exactly are Python functions? In the simplest terms, a Python function is a block of reusable code designed to perform a specific task. Whenever you need to perform that task in your program, you can call the function instead of writing the same code again. This not only streamlines your code but also significantly enhances your coding efficiency.
The Power of Python Functions
Python functions bring a multitude of benefits to your coding practice. One of the main benefits is code reusability. Rather than writing the same code multiple times, you can write a function once and call it wherever you need it. This not only saves time but also makes your code easier to understand and maintain.
Another significant benefit of Python functions is that they help you create modular code. This means you can break down a complex problem into smaller, more manageable parts. Each function serves as a module that performs a specific task, simplifying the process of understanding and debugging your code.
Declaring a Python Function
Declaring a Python function is straightforward. You use the def
keyword, followed by the function name and parentheses ()
. The code block within every function is initiated with a colon :
and is indented.
Here’s the basic syntax of a Python function:
def function_name():
# code
In the above example, function_name
is the name of the function. The code inside the indented block is the code that will be executed whenever the function is called.
Python functions are incredibly powerful tools that can significantly enhance your coding efficiency and readability. By understanding and utilizing Python functions, you can elevate your Python programming skills to new heights.
Exploring Types of Functions in Python
Python functions can be broadly classified into two types: built-in functions and user-defined functions. Each type has its own unique characteristics and uses.
Built-in Functions
Python comes pre-packaged with a suite of built-in functions that are readily available for use. These functions cater to common programming needs. For instance, the print()
function is used to display data on the screen, the len()
function returns the length of an object, and the type()
function discloses the type of an object.
Utilizing these functions is as simple as calling them in your program. Here’s an example:
print("Hello, world!")
In the above example, print
is a built-in function that displays the string “Hello, world!” on the screen.
User-Defined Functions
Apart from the built-in functions, Python also empowers you to create your own functions. These are referred to as user-defined functions. You can create a user-defined function using the def
keyword, followed by the function name and parentheses ()
.
Here’s an example of a user-defined function:
def greet():
print("Hello, world!")
In this example, greet
is a user-defined function that displays the string “Hello, world!” on the screen. To call this function, you simply use its name followed by parentheses:
greet()
The power of user-defined functions lies in their ability to create custom functions that perform specific tasks as per your requirements. This means you can create functions that do exactly what you want, providing a high degree of customization and flexibility.
Built-in vs User-Defined Functions: A Comparison
The fundamental difference between built-in and user-defined functions is that built-in functions are pre-defined in Python, while user-defined functions are defined by the user. This means that while built-in functions offer convenience, user-defined functions offer customization.
Another point of difference is that to use certain built-in functions, you may need to import the respective module. For example, to use the sqrt()
function to calculate the square root of a number, you need to import the math
module:
import math
print(math.sqrt(16))
Contrarily, user-defined functions do not require any imports (unless they use a built-in function from a module).
In conclusion, both built-in and user-defined functions have their own strengths. Built-in functions provide convenience and efficiency, while user-defined functions offer customization and flexibility. By understanding and utilizing both types of functions, you can enhance your effectiveness and versatility as a Python programmer.
Diving into Python Function Parameters and Arguments
The power of Python functions multiplies when you start to work with parameters and arguments. But what exactly are these concepts, and how do they function in Python? Let’s unravel this.
Deciphering Function Parameters
Function parameters are the names listed in the function definition. They act as placeholders for the values that the function requires to execute its task. When you define a function, you specify the parameters in the parentheses after the function name.
Consider this function with parameters:
def greet(name):
print(f'Hello, {name}!')
In this example, name
is a parameter. When you call this function, you can pass a value for this parameter.
Decoding Function Arguments
Function arguments are the actual values that are passed to the function when it is invoked. In essence, arguments are the values that replace the parameters when the function is executed.
Here’s an example of calling a function with an argument:
greet('Alice')
In this example, ‘Alice’ is an argument that is passed to the greet
function. When the function is executed, it substitutes ‘Alice’ for the name
parameter and prints ‘Hello, Alice!’.
Embracing an Unspecified Number of Arguments
Python also permits an unspecified number of arguments using ‘*args’. This means you can pass any number of arguments to the function, and Python will gather them into a tuple.
Here’s an example:
def add(*args):
return sum(args)
print(add(1, 2, 3, 4, 5))
In this example, the add
function accepts any number of arguments, sums them up, and returns the total. When you call add(1, 2, 3, 4, 5)
, it returns the sum of these numbers, which is 15.
Parameters vs Arguments: The Distinction
Often, the terms ‘parameter’ and ‘argument’ are used interchangeably. However, they denote slightly different things. A parameter is a variable listed in the function definition, while an argument is the actual value that is passed to the function. In other words, parameters appear in function definitions, while arguments appear in function calls.
The Significance of Function Parameters
Function parameters play a vital role in enhancing the flexibility and adaptability of functions. By using parameters, you can create functions that can perform a task with a variety of inputs, thereby making your functions more versatile. For instance, the greet
function above can greet any name, not just ‘Alice’. This makes it a flexible and reusable piece of code.
To sum up, understanding and utilizing function parameters and arguments is an integral part of working with Python functions. They not only make your functions more adaptable and flexible but also enable you to write cleaner and more efficient code.
Delving into Advanced Python Function Concepts
After mastering the basics of Python functions, it’s time to explore some advanced concepts. These include arbitrary keyword arguments, docstrings, anonymous functions, and more. These concepts can elevate your Python code, making it more efficient and effective.
Unleashing Arbitrary Keyword Arguments
Python provides the flexibility to pass an arbitrary number of keyword arguments to a function using **kwargs
. These arguments are gathered into a dictionary, which can then be processed within the function.
Consider this example:
def greet(**kwargs):
for key, value in kwargs.items():
print(f'{key} : {value}')
greet(name='Alice', age=25, country='USA')
def greet(**kwargs):
for key, value in kwargs.items():
print(f'{key} : {value}')
greet(name='Alice', age=25, country='USA')
In this example, name
, age
, and country
are keyword arguments. The greet
function consolidates these arguments into a dictionary and then prints each key-value pair.
Understanding Docstrings in Python Functions
Docstrings, an abbreviation for documentation strings, serve to document Python functions. They are written as a string at the start of a function and provide a description of the function’s purpose.
def greet(name):
"""This function greets the person passed in as a parameter"""
print(f'Hello, {name}!')
Here’s a function with a docstring for illustration:
def greet(name):
"""This function greets the person passed in as a parameter"""
print(f'Hello, {name}!')
In this example, the string """This function greets the person passed in as a parameter"""
is a docstring that elucidates what the greet
function does.
Embracing Anonymous Functions in Python
Python also supports anonymous functions, otherwise known as lambda functions. These are compact, one-line functions that are not defined with the conventional def
keyword.
Here’s an instance of a lambda function:
greet = lambda name: print(f'Hello, {name}!')
In this example, greet
is a lambda function that accepts one argument name
and prints a greeting message.
Lambda functions are particularly useful when you require a small function for a short duration and don’t wish to define a full function with the def
keyword.
Exploring Nested Functions
Python also allows the definition of functions within other functions. These are known as nested functions. Nested functions can access variables of the enclosing scope, making them beneficial for certain tasks.
Here’s an instance of a nested function:
def outer_function():
def inner_function():
print('Hello, world!')
inner_function()
outer_function()
In this example, inner_function
is a nested function defined within the outer_function
. When you call outer_function()
, it defines inner_function
and then calls it, printing ‘Hello, world!’.
Nested functions are highly beneficial for encapsulating code that is exclusively used by the enclosing function, thereby enhancing code organization and readability.
Harnessing the Power of Anonymous Functions
Anonymous functions, or lambda functions, are a potent feature of Python. They enable you to write swift, one-off functions without the need to define a full function with the def
keyword. This can make your code more concise and cleaner, especially when you’re working with functions like map
, filter
, and reduce
that take a function as an argument.
In conclusion, understanding and employing these advanced Python function concepts can significantly boost your Python programming skills. They not only equip you with more tools to tackle problems but also aid you in writing cleaner and more efficient code.
Unraveling Recursion in Python Functions
As we delve deeper into Python functions, we come across a fascinating concept known as recursion. Recursion is a problem-solving technique where a function calls itself, much like a mirrored reflection that goes on indefinitely. It’s like inception, but for functions!
The Concept of Recursion
In Python, a recursive function is a function that solves a problem by solving a smaller instance of the same problem. It might sound a bit mind-bending, but it’s a powerful concept once understood.
Imagine standing at the base of a staircase, intending to reach the top. You could solve this problem by taking one step, then addressing the ‘smaller’ problem of reaching the top from your current step. This is the essence of recursion – solving a problem by solving smaller instances of the same problem.
The Mechanism of Recursion
In a recursive function, the function calls itself to address the problem. This is known as a recursive call. The function continues to call itself until it reaches a condition where it can return a result without any further recursion. This condition is known as the base case.
Here’s an example of a recursive function in Python:
def countdown(n):
if n <= 0:
print('Blast off!')
else:
print(n)
countdown(n-1)
In this example, countdown
is a recursive function that counts down from a number n
to 0. It accomplishes this by printing the number n
and then calling itself with the argument n-1
. The base case is when n
is less than or equal to 0, at which point it prints ‘Blast off!’ and halts calling itself.
The Significance of the Base Case
The base case is a critical component of any recursive function. It’s the condition that stops the function from calling itself indefinitely. Without a base case, a recursive function would keep calling itself ad infinitum, leading to an infinite loop and eventually a stack overflow error.
In the countdown
function above, the base case is n <= 0
. When this condition is met, the function ceases calling itself and prints ‘Blast off!’. This halts the recursion and allows the function to return.
Recursive Functions in Action
Recursion is a potent tool that can simplify complex problems. For instance, consider calculating the factorial of a number. The factorial of a number n
is the product of all positive integers less than or equal to n
. This problem can be elegantly solved using recursion:
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n-1)
In this example, factorial
is a recursive function that calculates the factorial of a number n
. It does this by returning the product of n
and the factorial of n-1
. The base case is when n
is 1, at which point it returns 1.
The Might of Recursion
Recursion is a mighty concept that can simplify complex problems and make your code cleaner and more elegant. However, it’s also a concept that can be challenging to grasp. The key to understanding recursion is to break it down into smaller parts – mirroring how a recursive function breaks a problem down into smaller parts!
In conclusion, understanding and utilizing recursion is an advanced aspect of working with Python functions. It not only equips you with a powerful tool to solve complex problems but also aids in writing cleaner and more efficient code.
Wrapping Up Python Functions: Building Blocks of Python Programming
We’ve embarked on an extensive exploration into the world of Python functions in this post. From understanding what they are and how they work, to diving into the different types of functions available in Python, we’ve covered it all. We’ve also unraveled some advanced function concepts, including arbitrary keyword arguments, docstrings, and anonymous functions, and delved into the powerful concept of recursion.
Python functions are akin to the building blocks of Python programming. They foster code reusability and facilitate the creation of modular code, significantly enhancing your coding efficiency and readability. By gaining a comprehensive understanding and making thoughtful use of Python functions, you can elevate the cleanliness, efficiency, and effectiveness of your Python code.
Whether you’re leaning on built-in functions for their convenience or crafting your own user-defined functions for a custom solution, Python functions serve as a potent tool in your programming arsenal. With the added knowledge of advanced concepts like recursion and anonymous functions, you’re equipped to tackle complex problems in a simpler and more elegant manner.
For more on Python’s diverse capabilities, check out our cheat sheet here.
So, when you next find yourself in a coding rut, remember the power of Python functions. They’re like your secret weapon, ready to be deployed. Use them wisely, and you’ll be well on your way to becoming a Python programming pro, building sturdy and efficient programs one function at a time!