Removing Items from Lists in Python: Easy Techniques
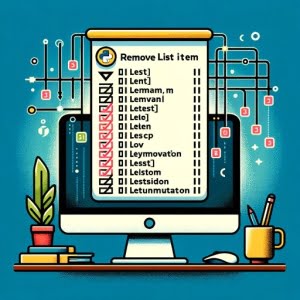
Easily removing items from lists in Python is a hurdle we often encounter while scripting for use at IOFLOOD. This process is especially important during script setup, for maintaining clean and consistent data sets. In this article, we will share our best practices and examples to help our dedicated hosting customers manage their data with ease.
Whether you’re a beginner just starting out with Python, or an intermediate user looking to deepen your understanding, this guide has something for you. We’ll start with the basics and gradually move on to more advanced techniques, ensuring you have a comprehensive understanding of how to remove items from a list in Python.
So, let’s dive in and start making items disappear from your Python lists!
TL;DR: How Do I Remove an Item from a List in Python?
To remove an item f rom a list in Python you can use the
remove()
method with the syntax,sample_list.remove('sample_item')
. Here’s a simple example:
my_list = ['apple', 'banana', 'cherry']
my_list.remove('banana')
print(my_list)
# Output:
# ['apple', 'cherry']
In this example, we have a list called my_list
with three items. We use the remove()
method to remove ‘banana’ from the list. When we print the list, ‘banana’ is no longer present, leaving us with ['apple', 'cherry']
.
Stay tuned for more detailed explanations and advanced usage scenarios. We’ll explore other methods to remove items from a list in Python, discuss their advantages and disadvantages, and provide practical examples to help you understand these concepts better.
Table of Contents
Basic Use: Python list.remove()
The remove()
method is the most straightforward way to remove an item from a list in Python. This method searches for the first occurrence of the given item in the list and removes it. If the item is not found, it raises a ValueError
.
Here’s how you can use the remove()
method:
fruits = ['apple', 'banana', 'cherry', 'banana']
fruits.remove('banana')
print(fruits)
# Output:
# ['apple', 'cherry', 'banana']
In this example, we have a list named fruits
with ‘banana’ appearing twice. When we call fruits.remove('banana')
, the first occurrence of ‘banana’ is removed from the list. The modified list now contains one ‘banana’ instead of two.
Advantages of the remove()
Method
The remove()
method is simple and easy to use, making it a great tool for beginners. It doesn’t require you to know the index of the item you want to remove, just the item itself.
Potential Pitfalls of the remove()
Method
However, the remove()
method only removes the first occurrence of the specified item. If the item appears multiple times in the list, subsequent occurrences remain. Also, if you try to remove an item that’s not in the list, Python raises a ValueError
, which can cause your program to crash if not handled properly.
Advanced Techniques: Item Removal
Python’s pop()
Function
Apart from the remove()
method, Python offers another built-in function to remove items from a list: the pop()
function. The pop()
function removes an item from a specific index in the list and returns it. If no index is specified, it removes and returns the last item in the list.
Here’s an example of how to use the pop()
function:
fruits = ['apple', 'banana', 'cherry']
removed_item = fruits.pop(1)
print(fruits)
print('Removed item:', removed_item)
# Output:
# ['apple', 'cherry']
# Removed item: banana
In this example, we call fruits.pop(1)
, which removes the item at index 1 (‘banana’) from the list and returns it. The modified list now only contains ‘apple’ and ‘cherry’. The removed item (‘banana’) is printed out separately.
Removing Items with List Comprehension
List comprehension is a powerful Python feature that allows you to create and manipulate lists in a concise way. You can use list comprehension to create a new list that excludes certain items from the original list.
Here’s how you can use list comprehension to remove items from a list:
fruits = ['apple', 'banana', 'cherry']
fruits = [fruit for fruit in fruits if fruit != 'banana']
print(fruits)
# Output:
# ['apple', 'cherry']
In this example, we create a new list that includes all items from the fruits
list that are not ‘banana’. The new list is then assigned back to the fruits
variable, effectively removing all occurrences of ‘banana’ from the list.
Additional Methods: Loops and filter()
Removing Items with For Loops
Python’s for
loop is a versatile tool that can also be used to remove items from a list. This method involves iterating over the list and copying all the items you want to keep to a new list.
Here’s an example of how to use a for loop to remove items from a list:
fruits = ['apple', 'banana', 'cherry']
new_list = []
for fruit in fruits:
if fruit != 'banana':
new_list.append(fruit)
fruits = new_list
print(fruits)
# Output:
# ['apple', 'cherry']
In this example, we create a new list and fill it with all items from the fruits
list that are not ‘banana’. We then assign the new list back to the fruits
variable, effectively removing ‘banana’ from the list.
Python’s filter()
Function
The filter()
function is a higher-order function used to create a new list that excludes certain items from the original list. The filter()
function takes two arguments: a function and a list. The function is applied to each item in the list, and only the items for which the function returns True
are included in the new list.
Here’s how you can use the filter()
function to remove items from a list:
fruits = ['apple', 'banana', 'cherry']
fruits = list(filter(lambda fruit: fruit != 'banana', fruits))
print(fruits)
# Output:
# ['apple', 'cherry']
In this example, we use the filter()
function with a lambda function that returns True
for all items that are not ‘banana’. The filter()
function returns a new list that includes only these items, which is then assigned back to the fruits
variable.
Troubleshooting Issues: Item Removal
Dealing with Non-Existent Items
One common issue that you may encounter when trying to remove an item from a list is that the item doesn’t exist in the list. If you try to remove a non-existent item using the remove()
method, Python will raise a ValueError
.
fruits = ['apple', 'cherry']
try:
fruits.remove('banana')
except ValueError:
print('Item not found in list')
# Output:
# Item not found in list
In this example, we try to remove ‘banana’ from a list that doesn’t contain ‘banana’. Python raises a ValueError
, but we catch it with a try/except block and print a custom error message.
Removing All Occurrences of an Item
Another issue is when you want to remove all occurrences of an item from a list. The remove()
method only removes the first occurrence of an item, so it won’t work if the item appears multiple times in the list.
fruits = ['apple', 'banana', 'cherry', 'banana']
while 'banana' in fruits:
fruits.remove('banana')
print(fruits)
# Output:
# ['apple', 'cherry']
In this example, we use a while loop to repeatedly remove ‘banana’ from the list until it’s no longer in the list. The modified list now only contains ‘apple’ and ‘cherry’.
Understanding Python Collections
Python’s list is a built-in data type that can be used to store multiple items in a single variable. Lists are one of the four built-in data types in Python used to store collections of data. The other three are Tuple, Set, and Dictionary.
For more info, we have wrriten an in-depth article on Tuples, you can read it here!
Understanding Python Lists
Lists are created by placing all the items (elements) inside square brackets []
, separated by commas. An item can be of any data type (strings, numbers, or even other lists), and a single list can contain items of different types.
# A list with items of different data types
my_list = ['apple', 1, 3.14, ['a', 'b', 'c']]
print(my_list)
# Output:
# ['apple', 1, 3.14, ['a', 'b', 'c']]
In this example, my_list
is a list that contains a string, an integer, a float, and another list.
Mutability of Python Lists
One important characteristic of Python lists is that they are mutable. This means that you can change their content without changing their identity. You can modify a list by adding, removing, or changing its elements.
Importance of List Manipulation
List manipulation, including removing items from a list, is a fundamental skill in Python programming. It’s especially important in areas such as data analysis and machine learning, where you often need to clean and preprocess your data before using it.
Understanding how to remove items from a list in Python, as well as the underlying concepts, will help you manipulate lists effectively and write more efficient Python code.
Related Concepts: List Management
Once you’ve mastered how to remove items from a list in Python, you might want to explore other related concepts. For example, you can learn about list slicing, which allows you to access subsets of your list, or list sorting, which enables you to arrange the items in your list in a certain order.
Here’s a simple example of list slicing:
fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry']
print(fruits[1:4])
# Output:
# ['banana', 'cherry', 'date']
In this example, fruits[1:4]
returns a new list that includes the items at index 1, 2, and 3 of the fruits
list.
Further Resources for Python
To deepen your understanding of Python lists and list manipulation, you can refer to Python’s official documentation, online tutorials, or Python programming books. Practice is key when it comes to programming, so make sure to write your own code and experiment with different methods of list manipulation.
Here are some resources that you might find helpful:
- Step-by-Step Tutorial: Working with Python Lists: Follow along with this detailed tutorial to learn how to manipulate and utilize Python lists effectively.
Removing Duplicates from a List in Python: This tutorial provides different techniques to remove duplicate elements from a list in Python, including using a set, list comprehension, and the OrderedDict class.
Sorting a List in Python: This guide explains how to sort a list in different ways in Python, such as using the sorted() function, the sort() method, and custom sorting with a key parameter.
Python Documentation: Data Structures: This official Python tutorial provides an in-depth explanation of various data structures such as lists, tuples, sets, and dictionaries, and how to use them effectively in Python.
Python Reference: List: This comprehensive reference guide covers the different methods and functionalities available for working with lists in Python, including list manipulation, sorting, searching, and more.
How to Remove an Item from the List in Python: An article on GeeksforGeeks that illustrates various techniques to remove items from a list in Python.
Recap: Removing Items in Python List
In this guide, we explored various methods to remove items from a list in Python. We started with the basic remove()
method, which is simple and easy to use but only removes the first occurrence of the specified item.
We then moved on to more advanced techniques such as the pop()
function and list comprehension, which offer more flexibility but require a better understanding of Python.
We also discussed alternative approaches like using a for loop or the filter()
function, which can be useful in certain scenarios.
Furthermore, we covered some common issues you might encounter when removing items from a list in Python, such as trying to remove a non-existent item, and provided solutions and workarounds for these issues.
In summary, Python offers several ways to remove items from a list, each with its own advantages and disadvantages. The best method to use depends on your specific needs and your understanding of Python. By mastering these methods, you’ll be able to manipulate Python lists effectively and write more efficient Python code.