Python Pop() Method: Complete Guide
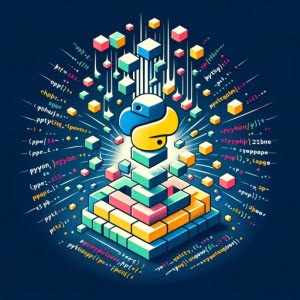
Are you finding it challenging to remove items from lists or dictionaries in Python? You’re not alone. Many developers find themselves puzzled when it comes to using the pop method in Python, but we’re here to help.
Think of Python’s pop method as a skilled magician – making elements disappear from your data structures with a simple command. It’s a powerful tool that can simplify your data manipulation tasks significantly.
In this guide, we’ll walk you through the process of mastering the pop method in Python, from its basic usage to more advanced techniques. We’ll cover everything from simple examples to dealing with complex scenarios and even alternative approaches.
So, let’s dive in and start mastering Python’s pop method!
TL;DR: How Do I Use the Pop Method in Python?
The pop method in Python is used to remove an item at the specified position in a list or dictionary and return it. For example,
item = my_list.pop(1)
will remove the item at index 1 frommy_list
.
Here’s a simple example:
my_list = [1, 2, 3]
item = my_list.pop(1)
print(item)
print(my_list)
# Output:
# 2
# [1, 3]
In this example, we have a list my_list
with elements 1, 2, and 3. We use the pop method to remove the item at index 1 (which is the number 2). The pop method returns this item, which we store in the variable item
. When we print item
, we see the number 2. When we print my_list
, we see that it now only contains the numbers 1 and 3.
This is just a basic usage of the pop method in Python. Continue reading for more detailed examples and advanced usage scenarios.
Table of Contents
- Understanding Python’s Pop Method
- Python Pop Method with Nested Data Structures
- Exploring Alternatives to Python’s Pop Method
- Troubleshooting Common Issues with Python’s Pop Method
- Python Data Structures: Lists and Dictionaries
- Indexing and the Pop Method
- Expanding Your Python Skills: Beyond Pop
- Wrapping Up: Mastering Python’s Pop Method
Understanding Python’s Pop Method
The pop method in Python is a built-in function that removes an item from a list or dictionary at a specific position, also known as the index, and returns it. This method can be extremely useful when you need to manipulate data structures in Python.
Using Pop with Python Lists
Here’s how you can use the pop method with a Python list:
# Create a list
my_list = ['apple', 'banana', 'cherry']
# Use the pop method
item = my_list.pop(1)
# Print the item and the updated list
print(item)
print(my_list)
# Output:
# 'banana'
# ['apple', 'cherry']
In this example, we created a list called my_list
with the elements ‘apple’, ‘banana’, and ‘cherry’. We then used the pop method to remove the item at index 1 (which is ‘banana’) and stored the returned item in the variable item
. When we print item
, we see ‘banana’. When we print my_list
, we see that it now only contains ‘apple’ and ‘cherry’.
Using Pop with Python Dictionaries
The pop method can also be used with Python dictionaries. However, instead of using an index, we use the key of the item we want to remove. Here’s an example:
# Create a dictionary
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Use the pop method
item = my_dict.pop('age')
# Print the item and the updated dictionary
print(item)
print(my_dict)
# Output:
# 25
# {'name': 'Alice', 'city': 'New York'}
In this example, we created a dictionary called my_dict
with the key-value pairs ‘name’: ‘Alice’, ‘age’: 25, and ‘city’: ‘New York’. We then used the pop method to remove the item with the key ‘age’ and stored the returned value in the variable item
. When we print item
, we see 25. When we print my_dict
, we see that it now only contains the key-value pairs ‘name’: ‘Alice’ and ‘city’: ‘New York’.
The pop method is a powerful tool for manipulating data structures in Python, but it’s important to remember that it modifies the original list or dictionary. If you need to keep the original data structure intact, you might want to consider using other methods or making a copy before using pop.
Python Pop Method with Nested Data Structures
As we dive deeper into Python, we often encounter more complex data structures, such as nested lists or dictionaries. The pop method remains a valuable tool even in these scenarios.
Pop Method with Nested Lists
Consider a nested list, which is essentially a list within a list. Here’s how you can use the pop method in this scenario:
# Create a nested list
nested_list = [['apple', 'banana'], ['carrot', 'daikon']]
# Use the pop method
item = nested_list[0].pop(1)
# Print the item and the updated nested list
print(item)
print(nested_list)
# Output:
# 'banana'
# [['apple'], ['carrot', 'daikon']]
In this example, we first target the inner list at index 0 (nested_list[0]
), and then use the pop method to remove the item at index 1. The pop method returns ‘banana’, which we store in the variable item
. The updated nested list now contains ‘apple’ in the first inner list and ‘carrot’ and ‘daikon’ in the second inner list.
Pop Method with Nested Dictionaries
Similarly, the pop method can be used with nested dictionaries. Here’s an example:
# Create a nested dictionary
nested_dict = {'Alice': {'age': 25, 'city': 'New York'}, 'Bob': {'age': 30, 'city': 'Chicago'}}
# Use the pop method
item = nested_dict['Alice'].pop('age')
# Print the item and the updated nested dictionary
print(item)
print(nested_dict)
# Output:
# 25
# {'Alice': {'city': 'New York'}, 'Bob': {'age': 30, 'city': 'Chicago'}}
In this example, we first target the inner dictionary with the key ‘Alice’ (nested_dict['Alice']
), and then use the pop method to remove the item with the key ‘age’. The pop method returns 25, which we store in the variable item
. The updated nested dictionary now contains only the ‘city’ key-value pair for ‘Alice’ and the original key-value pairs for ‘Bob’.
As you can see, the pop method is versatile and can handle even complex data structures in Python. However, remember that it modifies the original data structure, so use it wisely.
Exploring Alternatives to Python’s Pop Method
While the pop method is a powerful tool for manipulating data structures in Python, it’s not the only one. There are other methods, like remove
and del
, which can also be used to remove items from lists or dictionaries. Let’s explore these alternatives and their effectiveness.
The Remove Method
The remove
method removes the first occurrence of a value in a list. Unlike the pop method, it doesn’t work with dictionaries. Here’s an example:
# Create a list
my_list = ['apple', 'banana', 'cherry', 'banana']
# Use the remove method
my_list.remove('banana')
# Print the updated list
print(my_list)
# Output:
# ['apple', 'cherry', 'banana']
In this example, the remove method removes the first occurrence of ‘banana’ from my_list
. The updated list now contains ‘apple’, ‘cherry’, and ‘banana’ (the second occurrence).
One disadvantage of the remove method is that it raises a ValueError if the value is not found in the list. Also, it doesn’t return the removed item.
The Del Statement
The del
statement can be used to remove an item at a specific index in a list or a key-value pair in a dictionary. Here’s an example:
# Create a list
my_list = ['apple', 'banana', 'cherry']
# Use the del statement
del my_list[1]
# Print the updated list
print(my_list)
# Output:
# ['apple', 'cherry']
In this example, the del statement removes the item at index 1 from my_list
. The updated list now contains ‘apple’ and ‘cherry’.
One disadvantage of the del statement is that it raises an IndexError if the index is out of range. Also, like the remove method, it doesn’t return the removed item.
In conclusion, while the pop method is a versatile and powerful tool for manipulating data structures in Python, it’s not the only option. Depending on your specific needs, you might find the remove method or the del statement more suitable. As always, remember to consider the advantages and disadvantages of each method before making a decision.
Troubleshooting Common Issues with Python’s Pop Method
While Python’s pop method is a handy tool for manipulating data structures, it’s not without its potential pitfalls. In this section, we’ll discuss some common issues you might encounter when using the pop method and provide solutions and workarounds.
Dealing with IndexError
One common issue is the IndexError, which occurs when you try to pop an item at an index that doesn’t exist in the list. Here’s an example:
# Create a list
my_list = ['apple', 'banana', 'cherry']
# Try to pop an item at an index that doesn't exist
try:
item = my_list.pop(3)
except IndexError:
print('IndexError: pop index out of range')
# Output:
# 'IndexError: pop index out of range'
In this example, we tried to pop an item at index 3, which doesn’t exist in my_list
. Python raised an IndexError, which we caught with a try-except block and printed a custom error message.
Handling KeyError
Another common issue is the KeyError, which occurs when you try to pop an item with a key that doesn’t exist in the dictionary. Here’s an example:
# Create a dictionary
my_dict = {'name': 'Alice', 'age': 25}
# Try to pop an item with a key that doesn't exist
try:
item = my_dict.pop('city')
except KeyError:
print('KeyError: pop key not found')
# Output:
# 'KeyError: pop key not found'
In this example, we tried to pop an item with the key ‘city’, which doesn’t exist in my_dict
. Python raised a KeyError, which we caught with a try-except block and printed a custom error message.
In conclusion, while the pop method is a powerful tool for manipulating data structures in Python, it’s important to be aware of potential issues and know how to handle them. By using try-except blocks, you can catch errors and prevent your program from crashing. Remember, understanding the problem is the first step towards finding a solution.
Python Data Structures: Lists and Dictionaries
To fully grasp the power of the pop method in Python, it’s important to understand the fundamental data structures it operates on – lists and dictionaries.
Python Lists
A Python list is an ordered collection of items that can be of any type. The items in a list are indexed starting from 0. Here’s an example of a Python list:
# Create a list
my_list = ['apple', 'banana', 'cherry']
# Print the list
print(my_list)
# Output:
# ['apple', 'banana', 'cherry']
In this example, ‘apple’ is at index 0, ‘banana’ is at index 1, and ‘cherry’ is at index 2.
Python Dictionaries
A Python dictionary is an unordered collection of key-value pairs. Unlike lists, dictionaries are indexed by keys, which can be of any immutable type. Here’s an example of a Python dictionary:
# Create a dictionary
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Print the dictionary
print(my_dict)
# Output:
# {'name': 'Alice', 'age': 25, 'city': 'New York'}
In this example, ‘name’ is a key with the value ‘Alice’, ‘age’ is a key with the value 25, and ‘city’ is a key with the value ‘New York’.
Indexing and the Pop Method
Indexing plays a crucial role when using the pop method with Python lists. When you call my_list.pop(1)
, Python looks for the item at index 1 in my_list
and removes it. Similarly, when you call my_dict.pop('age')
, Python looks for the key ‘age’ in my_dict
and removes the corresponding key-value pair.
By understanding Python’s list and dictionary data types and the concept of indexing, you can better understand and use the pop method in your Python programming endeavors.
Expanding Your Python Skills: Beyond Pop
The pop method is a fundamental part of Python programming, particularly when it comes to data manipulation and web scraping. But Python’s capabilities go far beyond this method, and understanding related concepts can help you become a more proficient Python programmer.
Data Manipulation with Python
Python’s pop method is a powerful tool for manipulating lists and dictionaries, which are key data structures in Python. By learning how to effectively remove items from these data structures, you can handle and process data more efficiently, which is a critical skill in areas such as data analysis and machine learning.
Web Scraping with Python
Web scraping is another area where Python’s pop method can be useful. When you scrape data from the web, you often end up with nested lists or dictionaries that contain the scraped data. By using the pop method, you can easily remove unnecessary items and clean up your data.
Exploring Related Concepts
To further enhance your Python skills, consider exploring related concepts like list comprehension and dictionary manipulation. List comprehension is a concise way to create lists in Python, while dictionary manipulation involves various techniques to add, remove, or change items in a dictionary.
Further Resources for Python Pop Mastery
To deepen your understanding of Python’s pop method and related concepts, consider checking out the following resources:
- Beginner’s Guide to Python Built-In Functions – Master the art of leveraging built-in functions for efficient programming.
Hashing Data with Python’s hash() Function – Dive into hashing objects and data for quick comparisons in Python.
Working with Bytes in Python: A Quick Guide – Discover Python’s “bytes” data type for working with binary data.
The official Python Documentation provides explanations on Python’s data structures, including lists and dictionaries.
Real Python offers a wealth of tutorials and articles on various Python topics.
Python for Beginners provides a wide range of Python tutorials and guides, making it a great resource for Python learners.
Remember, mastering a programming language is a journey, and there’s always more to learn. So keep exploring, keep practicing, and keep pushing your Python skills to new heights.
Wrapping Up: Mastering Python’s Pop Method
In this comprehensive guide, we’ve delved into the ins and outs of Python’s pop method, a powerful tool for manipulating lists and dictionaries.
We began with the basics, learning how to use the pop method to remove items from lists and dictionaries. We then explored more advanced usage scenarios, such as dealing with nested lists and dictionaries.
Along the way, we tackled common challenges you might face when using the pop method, such as IndexError and KeyError, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to item removal in Python, comparing the pop method with other methods like remove
and del
.
Here’s a quick comparison of these methods:
Method | Works with | Returns removed item | Raises error if item not found |
---|---|---|---|
pop | Lists and dictionaries | Yes | Yes |
remove | Lists only | No | Yes |
del | Lists and dictionaries | No | Yes |
Whether you’re just starting out with Python or you’re looking to level up your data manipulation skills, we hope this guide has given you a deeper understanding of Python’s pop method and its capabilities.
With its balance of flexibility and power, the pop method is a crucial tool for any Python programmer. Keep practicing, keep exploring, and soon you’ll be popping items like a pro. Happy coding!