Python Bytes Data Type | bytes() Function Guide
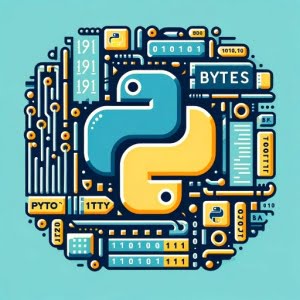
Are you finding it challenging to understand Python bytes? You’re not alone. Many developers find themselves puzzled when it comes to handling bytes in Python, but we’re here to help.
Think of Python bytes as a secret code – a tool that can unlock efficient data handling in your Python applications. Bytes in Python are a sequence of integers in the range of 0-255, and they are a fundamental aspect of managing binary data in Python.
This guide will walk you through the basics of Python bytes, how to use them, and why they’re important. We’ll cover everything from creating and using bytes in Python, to converting bytes to other data types and vice versa, and even troubleshooting common issues.
So, let’s dive in and start mastering Python bytes!
TL;DR: What are Python Bytes and How Do I Use Them?
Python bytes are a sequence of integers in the range of 0-255. You can create bytes in Python using the
bytes()
function or a bytes literal.
Here’s a simple example:
data = bytes([120, 110, 100, 97, 111])
print(data)
# Output:
# b'xndao'
In this example, we’re creating a bytes object in Python using the bytes()
function. We pass a list of integers, each representing a byte. When we print the data
variable, we get a bytes object represented as a string.
This is just a basic introduction to Python bytes. There’s much more to learn about creating, manipulating, and using bytes in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with Python Bytes
Python bytes are a sequence of integers in the range of 0-255. They are an immutable sequence data type, meaning once a bytes object is created, it cannot be changed. Let’s dive in and see how to create and use Python bytes.
Creating Bytes in Python
There are a couple of ways to create bytes in Python. The simplest way is to use the bytes()
function or a bytes literal, like so:
data = bytes([65, 66, 67])
print(data)
# Output:
# b'ABC'
In this example, we’re creating a bytes object in Python using the bytes()
function. The bytes()
function takes a list of integers, each representing a byte. When we print the data
variable, we get a bytes object represented as a string.
Accessing and Modifying Python Bytes
As bytes are immutable, you cannot modify them. However, you can access individual bytes using indexing, similar to how you would with a list or a string.
data = bytes([65, 66, 67])
print(data[0])
# Output:
# 65
In this example, we’re accessing the first byte in the data
object. When we print it, we get the integer value of the byte.
Advantages of Using Bytes
Bytes are extremely useful for handling binary data. They are compact (each byte takes up only 8 bits of memory), and they are faster to process than text data. This makes them ideal for tasks such as reading binary files or sending data over a network.
Potential Pitfalls with Python Bytes
While bytes are powerful, they can also be a bit tricky to work with. One common pitfall is trying to modify a bytes object, which will result in a TypeError. Remember, bytes are immutable!
Another potential issue is encoding. Bytes represent raw binary data, while strings represent text. Converting between the two (known as encoding and decoding) can sometimes result in errors if not done correctly. We’ll cover this in more detail in the Intermediate Level section.
Exploring Advanced Python Bytes
As you get more comfortable with Python bytes, you can start exploring more complex uses. A common intermediate-level task involving bytes is converting them to other data types and vice versa. This is known as encoding and decoding.
Encoding and Decoding with Python Bytes
Encoding is the process of converting a string into bytes, while decoding is the opposite – converting bytes into a string. This is done using the encode()
and decode()
methods in Python.
Here’s an example of encoding a string into bytes:
string = 'Hello, World!'
encoded = string.encode('utf-8')
print(encoded)
# Output:
# b'Hello, World!'
In this example, we’re encoding the string ‘Hello, World!’ into bytes using the UTF-8 encoding. The encode()
method returns a bytes object.
Now, let’s decode the bytes back into a string:
decoded = encoded.decode('utf-8')
print(decoded)
# Output:
# 'Hello, World!'
Here, we’re decoding the bytes object back into a string using the UTF-8 encoding. The decode()
method returns a string.
Working with Other Data Types
Bytes can also be converted to and from other data types. For example, you can convert a bytes object to a list of integers like so:
data = bytes([65, 66, 67])
list_of_integers = list(data)
print(list_of_integers)
# Output:
# [65, 66, 67]
In this example, we’re converting the bytes object to a list of integers. Each integer represents a byte in the bytes object.
Remember, encoding and decoding can sometimes result in errors if not done correctly, especially when dealing with non-ASCII characters. Always specify the correct encoding when encoding or decoding bytes.
Alternative Methods for Handling Bytes in Python
As you continue to work with bytes in Python, you may find that the basic bytes()
function doesn’t always meet your needs. Fortunately, Python provides several other ways to handle bytes, including the bytearray
type.
Working with Bytearrays in Python
A bytearray is like a bytes object, but mutable. That means you can change the values of a bytearray without creating a new object. This can be more efficient in some cases. Here’s how you create a bytearray:
arr = bytearray([65, 66, 67])
print(arr)
# Output:
# bytearray(b'ABC')
In this example, we’re creating a bytearray object in Python using the bytearray()
function. The bytearray()
function takes a list of integers, each representing a byte. When we print the arr
variable, we get a bytearray object represented as a string.
You can modify a bytearray using indexing, similar to how you would with a list:
arr[0] = 68
print(arr)
# Output:
# bytearray(b'DBC')
In this example, we’re changing the first byte in the arr
object. When we print it, we see that the first byte has been changed.
Advantages and Disadvantages of Bytearrays
Bytearrays have several advantages over bytes. They are mutable, so you can modify them without creating a new object. This can be more efficient in terms of memory usage, especially for large amounts of data.
However, bytearrays also have some disadvantages. They are not hashable, so they can’t be used as dictionary keys. They also take up slightly more memory than bytes, due to their mutability.
When deciding whether to use bytes or bytearray, consider the needs of your program. If you need to modify your binary data frequently, a bytearray might be the way to go. If your data is mostly static, or if you need to use it as a dictionary key, you might be better off with bytes.
Troubleshooting Common Python Bytes Issues
As you work with Python bytes, you may encounter some common issues. Let’s discuss these problems and their solutions to help you navigate through your Python bytes journey smoothly.
Dealing with UnicodeDecodeError
One common issue when working with bytes is the UnicodeDecodeError
. This occurs when you try to decode a bytes object that contains non-ASCII characters using the ASCII encoding. Here’s an example:
non_ascii_bytes = bytes([128, 129, 130])
try:
string = non_ascii_bytes.decode('ascii')
except UnicodeDecodeError:
print('Cannot decode bytes using ASCII.')
# Output:
# Cannot decode bytes using ASCII.
In this example, we’re trying to decode a bytes object that contains non-ASCII characters using the ASCII encoding. This raises a UnicodeDecodeError
, which we catch and print a message.
To avoid this error, always use the correct encoding when decoding bytes. If your bytes object contains non-ASCII characters, you should use an encoding that can handle these characters, such as ‘utf-8’.
Modifying Immutable Bytes
Another common pitfall is trying to modify a bytes object. As we’ve discussed, bytes are immutable, so trying to change them will result in a TypeError. Here’s an example:
data = bytes([65, 66, 67])
try:
data[0] = 68
except TypeError:
print('Cannot modify bytes.')
# Output:
# Cannot modify bytes.
In this example, we’re trying to change the first byte in the data
object. This raises a TypeError
, which we catch and print a message.
To avoid this error, if you need to modify your binary data frequently, consider using a bytearray instead. Bytearrays are mutable, so you can change them without creating a new object.
Understanding Python Bytes and Binary Data
To truly master Python bytes, it’s essential to understand what bytes are and why they’re so important in computing. Let’s delve into the fundamentals of Python’s bytes data type and binary data in general.
What are Bytes?
In computing, a byte is the basic unit of information storage and processing. It’s composed of 8 bits, each bit representing a binary value of 0 or 1. This means a single byte can represent 256 (2^8) different values.
In Python, bytes are represented as a sequence of integers in the range of 0-255. This makes Python bytes an efficient way to handle binary data.
Here’s an example of creating a bytes object in Python:
data = bytes([65, 66, 67])
print(data)
# Output:
# b'ABC'
In this example, we’re creating a bytes object in Python using the bytes()
function. The bytes()
function takes a list of integers, each representing a byte. When we print the data
variable, we get a bytes object represented as a string.
Why are Bytes Important?
Bytes are fundamental to computing for several reasons. They’re used to represent all types of data, from text and images to executable code. They’re also used for data transmission over networks.
In Python, bytes are especially important for handling binary data. They’re used for reading binary files, sending and receiving data over networks, and more. Understanding how to work with bytes in Python is crucial for any developer working with binary data.
Python Bytes: Beyond Basic Usage
Python bytes play a crucial role beyond just basic data handling. They are integral to file handling, networking applications, and more. Let’s explore these areas to understand the full potential of Python bytes.
Python Bytes in File Handling
When dealing with binary files such as images, audio files, or any non-text data, Python bytes are the go-to data type. They allow for efficient reading, writing, and manipulation of binary data.
Here’s an example of reading a binary file using Python bytes:
with open('example.bin', 'rb') as file:
data = file.read()
print(data)
# Output:
# b'Binary file content...'
In this example, we open a binary file in read-binary mode (‘rb’) and read its content into a bytes object.
Python Bytes in Networking Applications
Python bytes are also widely used in networking applications. When sending and receiving data over a network, the data is transmitted as bytes. This makes Python bytes essential for network programming.
Here’s an example of sending data over a network using Python bytes:
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(('localhost', 12345))
message = 'Hello, World!'.encode('utf-8')
sock.send(message)
sock.close()
# Note: This will send the message 'Hello, World!' to a server running on localhost on port 12345.
In this example, we create a socket, connect to a server, encode a string message into bytes, and send it over the network.
Further Resources for Mastering Python Bytes
- Getting Started With Python Built-In Functions Tutorial explores Python’s all of built-in functions.
List Manipulation with Python’s pop() Method – Dive into manipulating lists and dictionaries with the “pop” operation.
Python hasattr() Function: Attribute Existence Checking – Explore Python’s “hasattr” function for checking object attributes.
Python’s Official Documentation on Bytes offers a thorough guide on the built-in bytes functions.
Working with Binary Data in Python – Get insight into handling binary data in Python with DevDungeon’s tutorial.
Bytes and Bytearray Tutorial in Python 3 – Grab key knowledge about Bytes and Bytearray in Python 3 with this instructive video guide.
Wrapping Up: Mastering Python Bytes for Efficient Data Handling
In this comprehensive guide, we’ve embarked on a journey to understand Python bytes, a fundamental aspect of handling binary data in Python.
We began with the basics, learning how to create and use Python bytes. We then ventured into more advanced territory, exploring complex tasks such as encoding and decoding bytes and converting bytes to other data types.
Along the way, we tackled common challenges you might face when working with bytes, such as UnicodeDecodeError
and immutability of bytes, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to handling bytes in Python, such as using bytearray. This mutable sequence of integers offers more flexibility in certain scenarios, but also comes with its own considerations.
Here’s a quick comparison of these methods:
Method | Flexibility | Use Case |
---|---|---|
Python Bytes | Limited | When data is static |
Python Bytearray | High | When frequent modification is needed |
Whether you’re just starting out with Python bytes or you’re looking to level up your binary data handling skills, we hope this guide has given you a deeper understanding of Python bytes and their capabilities.
With its balance of efficiency and versatility, Python bytes are a powerful tool for handling binary data in Python. Happy coding!