Using .charAt() Function in Java | From Basics to Advanced
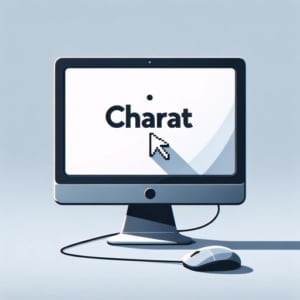
Ever found yourself needing to access a specific character in a string in Java? You’re not alone. Many developers find themselves in situations where they need to manipulate strings, and that’s where the charAt function comes into play. Think of the charAt function as a skilled librarian, able to help you find exactly the character you’re looking for in the vast library of a string.
In this guide, we will walk you through the use of the charAt function in Java, from the basics to more advanced techniques. We’ll cover everything from simple character access, handling different scenarios, to dealing with common issues and their solutions.
So, let’s dive in and start mastering the charAt function in Java!
TL;DR: How Do I Use the charAt Function in Java?
The charAt function in Java is used to return the character at a specific index in a string. You can use it like this:
char ch = str.charAt(1);
wherestr
is your string and1
is the index of the character you want to access.
Here’s a simple example:
String str = 'Hello';
char ch = str.charAt(1);
System.out.println(ch);
# Output:
# 'e'
In this example, we’ve created a string str
with the value ‘Hello’. We then use the charAt
function to get the character at index 1 (remember, Java uses zero-based indexing, so index 1 is the second character). The character is then printed to the console, resulting in the output ‘e’.
This is a basic way to use the charAt function in Java, but there’s much more to learn about accessing and manipulating characters in strings. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics of charAt in Java
- Handling Complex Scenarios with charAt in Java
- Exploring Alternatives to charAt in Java
- Troubleshooting Common Issues with charAt in Java
- Delving into Java’s String Class and String Indexing
- The Impact of charAt Function in Larger Java Projects
- Wrapping Up: Mastering the .charAt() Function in Java
Understanding the Basics of charAt in Java
The charAt
function in Java is a part of the String class and is used to retrieve a specific character from a string based on its position or index. The function takes an integer as input, which represents the index of the character you want to access. Remember, Java uses zero-based indexing, which means the first character in a string is at index 0, the second character is at index 1, and so forth.
Here’s a basic example of how to use the charAt
function:
String str = 'Hello, World!';
char ch = str.charAt(0);
System.out.println(ch);
# Output:
# 'H'
In this example, we have a string str
with the value ‘Hello, World!’. We then use the charAt
function to get the character at index 0, which is ‘H’. The character is then printed to the console, resulting in the output ‘H’.
Advantages of Using charAt
The charAt
function is straightforward and easy to use, making it a handy tool for beginners in Java. It allows you to access individual characters in a string, which can be useful for various tasks such as string manipulation and checking the presence of specific characters.
Potential Pitfalls of charAt
While the charAt
function is quite useful, it’s important to use it carefully. If you try to access an index that is out of the range of the string (either negative or greater than or equal to the length of the string), Java will throw a StringIndexOutOfBoundsException
. Always ensure that the index you’re trying to access is within the range of the string.
Handling Complex Scenarios with charAt in Java
As you get more comfortable with the charAt
function, you’ll find that it can be used in a variety of more complex scenarios. One such scenario is handling out-of-bounds indices, which can be a common pitfall when working with this function.
Dealing with Out-of-Bounds Indices
When you’re using the charAt
function, it’s important to ensure that the index you’re trying to access is within the range of the string. If the index is out of range, Java will throw a StringIndexOutOfBoundsException
. Here’s how you can handle this scenario:
String str = 'Hello, World!';
int index = 15;
if (index >= 0 && index < str.length()) {
char ch = str.charAt(index);
System.out.println(ch);
} else {
System.out.println('Index is out of bounds.');
}
# Output:
# Index is out of bounds.
In this example, we’re trying to access the character at index 15 in the string str
. However, the length of str
is only 13, so index 15 is out of bounds. To avoid a StringIndexOutOfBoundsException
, we first check if the index is within the range of the string. If it is, we use the charAt
function to get the character at that index. If it’s not, we print a message indicating that the index is out of bounds.
This is a best practice when using the charAt
function in Java, as it ensures that you’re always accessing valid indices and prevents potential runtime exceptions.
Exploring Alternatives to charAt in Java
While charAt
is a commonly used method to access characters in a string, Java provides other methods that can be used as alternatives, such as getChars
and toCharArray
methods.
Using getChars Method
The getChars
method is used to copy characters from a string into a destination character array. Here’s an example of how it works:
String str = 'Hello, World!';
char[] destination = new char[5];
str.getChars(0, 5, destination, 0);
System.out.println(destination);
# Output:
# Hello
In this example, we’re copying the first five characters from the string str
into the destination
character array. The getChars
method takes four parameters: the start index, the end index, the destination array, and the start position in the destination array.
Using toCharArray Method
The toCharArray
method converts a string into a new character array. Here’s how you can use it:
String str = 'Hello, World!';
char[] chars = str.toCharArray();
System.out.println(chars[0]);
# Output:
# H
In this example, we’re converting the string str
into a character array chars
using the toCharArray
method. We then print the first character of the array, which is ‘H’.
Comparing charAt, getChars, and toCharArray
Method | Advantage | Disadvantage |
---|---|---|
charAt | Simple and easy to use. | Throws StringIndexOutOfBoundsException if index is out of bounds. |
getChars | Can copy multiple characters at once. | More complex to use than charAt . |
toCharArray | Converts the entire string into a character array. | Can be inefficient for large strings. |
While charAt
is a simple and straightforward method to access characters in a string, getChars
and toCharArray
can be useful alternatives when dealing with multiple characters or when you need to convert a string into a character array. Choose the method that best suits your needs.
Troubleshooting Common Issues with charAt in Java
As with any function, using charAt
can sometimes lead to unexpected issues. One of the most common issues you might encounter is the StringIndexOutOfBoundsException
. This exception is thrown when trying to access an index that is out of the range of the string.
Handling StringIndexOutOfBoundsException
The StringIndexOutOfBoundsException
occurs when you try to access a character at an index that is either negative or greater than or equal to the length of the string. Here’s an example that throws this exception:
String str = 'Hello, World!';
try {
char ch = str.charAt(15);
System.out.println(ch);
} catch (StringIndexOutOfBoundsException e) {
System.out.println('Caught an exception: ' + e.getMessage());
}
# Output:
# Caught an exception: String index out of range: 15
In this example, we’re trying to access the character at index 15 in the string str
. However, the length of str
is only 13, so index 15 is out of bounds. When we try to access this index, Java throws a StringIndexOutOfBoundsException
. To handle this exception, we use a try-catch block. If the charAt
function throws an exception, we catch it and print a message to the console.
Tips for Using charAt Effectively
- Always ensure that the index you’re trying to access is within the range of the string.
- Use a try-catch block to handle potential exceptions when using the
charAt
function. - Remember that Java uses zero-based indexing. The first character in a string is at index 0, not 1.
By keeping these tips in mind, you can use the charAt
function effectively and avoid common issues.
Delving into Java’s String Class and String Indexing
To fully grasp the workings of the charAt
function, it’s crucial to understand the String class and the concept of string indexing in Java.
The String Class in Java
In Java, strings are objects that are instances of the String class. This class comes with a variety of methods to manipulate and perform operations on strings. The charAt
function is one of these methods. It allows us to access individual characters in a string based on their index.
Here’s a simple demonstration of creating a string object and using the charAt
function:
String str = new String('Hello, World!');
char ch = str.charAt(0);
System.out.println(ch);
# Output:
# 'H'
In this example, we’re creating a new string object str
with the value ‘Hello, World!’. We then use the charAt
function to get the character at index 0, which is ‘H’.
Understanding String Indexing in Java
String indexing is a way of accessing individual characters in a string. In Java, strings are zero-indexed, meaning the first character in a string is at index 0, the second character is at index 1, and so forth.
Here’s a visual representation of string indexing in Java:
String str = 'Hello';
// Index: 01234
char ch = str.charAt(2);
System.out.println(ch);
# Output:
# 'l'
In this example, we’re accessing the character at index 2 in the string str
. The character at this index is ‘l’, so ‘l’ is printed to the console.
By understanding the String class and string indexing, you can better understand and effectively use the charAt
function in Java.
The Impact of charAt Function in Larger Java Projects
The charAt
function, while simple, plays a significant role in larger Java programs and projects. It’s a fundamental part of string handling and manipulation in Java, which is a common task in many programs.
Exploring Related Concepts
Understanding the charAt
function opens the door to learning more about string manipulation and handling in Java. Concepts such as string concatenation, comparison, conversion, and searching are all related to and often involve the use of the charAt
function.
For example, you might need to use charAt
in a program that needs to count the number of occurrences of a specific character in a string. Or you might use it in a text processing program that needs to replace certain characters in a string.
Further Resources for Mastering Java String Handling
To deepen your understanding of the charAt
function and string handling in Java, here are some additional resources you might find helpful:
- Beginner’s Guide to Java Strings – Dive into the immutability of Java strings and its significance.
Java CompareTo Method – Understand how compareTo() returns an integer indicating the relative ordering of strings.
Java IndexOf Method – Learn about the indexOf() method’s parameters and return values for substring search.
Oracle’s Java Documentation on String provides in-depth information about the String class and its methods, including
charAt
.Java String Handling Tutorial by TutorialsPoint covers various aspects of string handling in Java.
Java String charAt Method by JavaTpoint provides a detailed explanation of the
charAt
method.
By exploring these resources and practicing with different string handling tasks, you can become proficient in using the charAt
function and handling strings in Java.
Wrapping Up: Mastering the .charAt() Function in Java
In this comprehensive guide, we’ve provided you with a detailed walkthrough on how to use the charAt function in Java. We’ve covered everything from the basics of accessing individual characters in a string to handling more complex scenarios and common issues.
We began with the basics, explaining how the charAt
function works and how to use it to access characters in a string. We then moved on to more advanced usage, discussing how to handle out-of-bounds indices and avoid the common StringIndexOutOfBoundsException
.
We also explored alternative methods to access characters in a string, such as using the getChars
and toCharArray
methods. Along the way, we provided practical code examples to illustrate these concepts and techniques.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantage | Disadvantage |
---|---|---|
charAt | Simple and easy to use. | Throws StringIndexOutOfBoundsException if index is out of bounds. |
getChars | Can copy multiple characters at once. | More complex to use than charAt . |
toCharArray | Converts the entire string into a character array. | Can be inefficient for large strings. |
Whether you’re a beginner just starting out with Java or an experienced developer looking for a refresher, we hope this guide has helped you gain a deeper understanding of the charAt function and its role in string manipulation in Java.
Mastering the charAt
function and other string manipulation techniques is a fundamental skill in Java programming. With this guide, you’re now well-equipped to handle character access in strings effectively. Happy coding!