Bash ‘sort’ Command: How-to Organize Data in Files
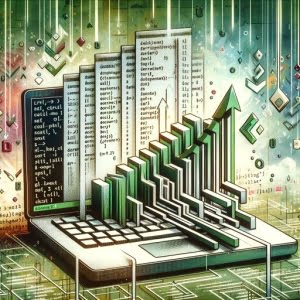
Are you finding it challenging to sort lines in text files using bash? Like a librarian organizing books, the bash ‘sort’ command can help you arrange lines in text and binary files. It’s a tool that, once mastered, can make your bash scripting tasks much easier and more efficient.
This guide will walk you through the basics to more advanced techniques of using the sort command in bash. We’ll explore the sort command’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the bash sort command!
TL;DR: How Do I Use the Sort Command in Bash?
To sort lines in a text file in bash, you use the
sort
command. It’s a simple yet powerful tool that can help you organize your data efficiently.
Here’s a simple example:
sort file.txt
# Output:
# Sorted lines of file.txt
In this example, we use the sort
command followed by the name of the file we want to sort (file.txt
). The command reads the file, sorts the lines, and then outputs the sorted lines.
This is just a basic way to use the
sort
command in bash, but there’s much more to learn about sorting lines in text and binary files. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Getting Started with Bash Sort
The sort
command in bash is a simple and efficient tool for organizing lines in text files. It reads the lines from the file, sorts them, and then outputs the sorted lines.
Let’s look at a basic example of how to use the sort
command:
# Here's a file named 'fruits.txt' with the following content:
# apple
# banana
# cherry
# date
# elderberry
# Now let's sort it using the sort command:
sort fruits.txt
# Output:
# apple
# banana
# cherry
# date
# elderberry
In this example, we’re using the sort
command followed by the name of the file we want to sort (fruits.txt
). The command reads the file, sorts the lines in alphabetical order, and then outputs the sorted lines.
The sort
command is a powerful tool that can help you organize your data efficiently. However, it’s important to understand its limitations. For instance, the sort
command sorts lines based on the ASCII value of characters, which might not always give you the expected result when sorting numbers or special characters. We’ll delve into these nuances in the advanced use section.
Exploring Advanced Bash Sort Features
As you become more comfortable with the sort
command in bash, it’s time to explore some of its advanced features. These include different flags that can be used to modify the way the command sorts lines in a file. Let’s discuss three important flags: -r
for reverse order, -n
for numerical sort, and -f
for case-insensitive sort.
Reverse Order with -r
The -r
flag is used to sort lines in reverse order. Here’s an example:
# Let's sort the 'fruits.txt' file in reverse order:
sort -r fruits.txt
# Output:
# elderberry
# date
# cherry
# banana
# apple
In this example, the sort -r
command sorts the lines in fruits.txt
in reverse alphabetical order.
Numerical Sort with -n
The -n
flag is used for numerical sort. It’s especially useful when dealing with numbers. Here’s an example:
# Here's a file named 'numbers.txt' with the following content:
# 10
# 2
# 1
# 20
# 3
# Now let's sort it using the sort command with the -n flag:
sort -n numbers.txt
# Output:
# 1
# 2
# 3
# 10
# 20
In this example, the sort -n
command sorts the lines in numbers.txt
in ascending numerical order.
Case-Insensitive Sort with -f
The -f
flag is used for case-insensitive sort. Here’s an example:
# Here's a file named 'case.txt' with the following content:
# Apple
# banana
# Cherry
# Date
# elderberry
# Now let's sort it using the sort command with the -f flag:
sort -f case.txt
# Output:
# Apple
# banana
# Cherry
# Date
# elderberry
In this example, the sort -f
command sorts the lines in case.txt
in a case-insensitive manner.
These flags can greatly enhance the utility of the sort
command in bash. They allow you to control the sorting process in a more granular way, which can be especially useful when dealing with complex data.
Alternative Sorting Methods in Bash
While the sort
command is a powerful tool for organizing data in bash, there are other methods you can use to sort lines in text files. Two such methods include using the awk
command and perl
script.
Sorting with Awk
Awk
is a versatile text processing language that can be used for a variety of tasks, including sorting. Here’s an example of how you can use awk
to sort lines in a file:
# Here's a file named 'fruits.txt' with the following content:
# apple
# banana
# cherry
# date
# elderberry
# Now let's sort it using the awk command:
awk '{ print $0 }' fruits.txt | sort
# Output:
# apple
# banana
# cherry
# date
# elderberry
In this example, we’re using awk
to print each line ($0
refers to the entire line) and then piping (|
) the output to the sort
command. The result is the same as if we had used the sort
command directly.
While this may seem redundant, awk
becomes incredibly useful when you need to sort based on specific fields in a line or perform complex transformations before sorting.
Sorting with Perl
Perl
is another powerful text processing language. It’s more complex than awk
but also more powerful. Here’s an example of sorting with perl
:
# Here's a file named 'fruits.txt' with the following content:
# apple
# banana
# cherry
# date
# elderberry
# Now let's sort it using the perl script:
perl -e 'print sort <>' fruits.txt
# Output:
# apple
# banana
# cherry
# date
# elderberry
In this example, the perl -e
command executes the provided script, which reads from the file (“), sorts the lines, and then prints them.
Both awk
and perl
provide more control over the sorting process than the sort
command alone, but they also have a steeper learning curve. If your sorting needs are complex, it might be worth learning these tools. However, for most sorting tasks, the sort
command is more than capable and easier to use.
Addressing Common Bash Sort Issues
While the sort
command in bash is robust and reliable, you may occasionally encounter issues or unexpected results. Let’s discuss some of these common challenges and how to overcome them.
Sorting with Different Locales
One common issue arises when sorting data in different locales. The sort
command uses your system’s locale settings to determine the order of characters. This can lead to unexpected results when sorting data that includes special or non-English characters.
Here’s an example:
# Let's say we have a file named 'words.txt' with the following content:
# zebra
# ångström
# æther
# penguin
# If we sort it using the sort command, we might get unexpected results:
sort words.txt
# Output (might vary depending on your system's locale settings):
# penguin
# zebra
# ångström
# æther
In this example, the sort
command doesn’t place ångström
and æther
at the beginning of the sorted list, as you might expect if you’re used to English alphabetical order.
To address this issue, you can set the LC_ALL
environment variable to C
before running the sort
command. This tells the command to use the traditional C locale, which sorts characters based on their ASCII values.
Here’s how you can do it:
# Sort the 'words.txt' file using the C locale:
LC_ALL=C sort words.txt
# Output:
# penguin
# zebra
# ångström
# æther
In this example, the sort
command sorts the lines in words.txt
based on their ASCII values, which places ångström
and æther
after zebra
and penguin
.
Remember, troubleshooting is an integral part of working with any command in bash. The key is to understand the command’s behavior and how it interacts with your system’s settings and the data you’re working with.
Bash Scripting and Sorting Fundamentals
To fully grasp the power of the bash sort
command, it’s essential to understand the fundamentals of bash scripting and the concept of sorting.
Bash Scripting Basics
Bash (Bourne Again Shell) is a command-line interpreter or shell. It allows users to interact with the operating system by executing commands. Bash scripting is writing a series of commands for the bash shell to execute. It’s a powerful tool for automating tasks on Unix or Linux based systems.
Here’s a simple bash script example:
#!/bin/bash
# This is a comment
# Print 'Hello, World!'
echo 'Hello, World!'
# Output:
# Hello, World!
In this script, #!/bin/bash
indicates that the script should be run using the bash shell. The echo
command is used to print ‘Hello, World!’ to the terminal.
Understanding Sorting
Sorting is arranging items in a particular order – ascending or descending. It’s a fundamental concept in computer science and data processing. In the context of bash scripting, sorting is often used to organize lines in text files for easier data analysis.
The bash sort
command is a powerful tool for this purpose. It reads a file line by line, sorts the lines based on certain criteria (like alphabetical or numerical order), and then outputs the sorted lines. The sort
command’s behavior can be modified using various flags, as we’ve seen in previous sections.
Understanding these fundamentals can help you better appreciate the utility of the bash sort
command and how it can be used to efficiently process and analyze data.
The Relevance of Bash Sort in Real-World Applications
The bash sort
command is not just a tool for organizing data—it’s a key player in many real-world applications, such as data analysis and log file management.
Sorting in Data Analysis
In data analysis, sorting is often the first step in understanding your data. It can reveal patterns and anomalies that might not be immediately apparent. For instance, sorting a dataset of customer transactions by date could help you identify seasonal trends or unusual activity.
Log File Management with Bash Sort
In log file management, the sort
command can help you make sense of large, unwieldy log files. For example, you could sort a server log file by IP address to group together all requests from a particular user. This could help you identify patterns of use or detect malicious activity.
Exploring Related Concepts
If you’ve mastered the sort
command and are looking for more ways to enhance your bash scripting skills, consider exploring related concepts like regular expressions and file handling in bash. Regular expressions can help you match and manipulate text with precision, while file handling techniques can enable you to read, write, and modify files efficiently.
Further Resources for Bash Sort Mastery
To deepen your understanding of the sort
command and related concepts, consider checking out the following resources:
- GNU Coreutils: Sort invocation: This is the official manual for the
sort
command from GNU. It’s a comprehensive resource that covers all the command’s features in detail. The Art of Command Line: This is a GitHub repository that offers practical tips and tricks for mastering the command line. It covers a wide range of topics, including sorting and other data manipulation techniques.
Bash Academy: This is an online academy dedicated to teaching bash scripting. It offers a range of courses, from beginner to advanced, that can help you hone your scripting skills.
Wrapping Up: Mastering Bash Sort for Efficient Data Manipulation
In this comprehensive guide, we’ve journeyed through the world of the bash sort
command, a powerful tool for organizing lines in text and binary files.
We started with the basics, learning how to use sort
for simple sorting tasks. We then ventured into more advanced territory, exploring the command’s various flags and how they can be used to modify the sorting process. We also tackled common challenges, such as sorting with different locales, providing you with solutions and workarounds for each issue.
We didn’t stop at the sort
command. We also looked at alternative approaches to sorting lines in text files, such as using the awk
command and perl
script. These tools can provide more control over the sorting process, especially for complex data.
Here’s a quick comparison of these methods:
Method | Complexity | Control Over Sorting Process |
---|---|---|
Bash Sort | Low | Moderate |
Awk | Moderate | High |
Perl | High | High |
Whether you’re just starting out with bash scripting or you’re looking to level up your data manipulation skills, we hope this guide has given you a deeper understanding of the sort
command and its capabilities.
With its balance of simplicity and power, the bash sort
command is a key player in many real-world applications, such as data analysis and log file management. Now, you’re well equipped to enjoy those benefits. Happy scripting!