Python ‘urllib’ Library | Guide to Fetching URLs
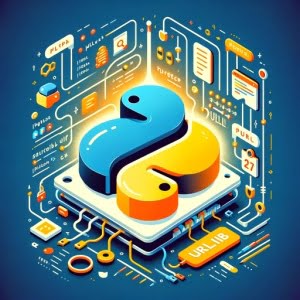
Ever found yourself struggling to fetch URLs in Python? You’re not alone. Many developers find Python URL fetching a bit challenging. But, think of urllib as your digital postman – it can deliver the content of any URL right to your Python script, making it a powerful tool in your Python toolkit.
In this guide, we’ll walk you through the process of using urllib in Python for fetching URLs, from the basics to more advanced techniques. We’ll cover everything from making simple URL requests using the urlopen
function, handling different types of URL responses, to troubleshooting common issues.
So, let’s get started and become proficient in fetching URLs with urllib in Python!
TL;DR: How Do I Use urllib to Fetch URLs in Python?
To fetch URLs in Python using urllib, you can use the
urlopen
function from the urllib.request module, such asdata = urlopen('http://example.com').read()
. This function allows you to read the contents of a URL and use it in your Python script.
Here’s a simple example:
from urllib.request import urlopen
data = urlopen('http://example.com').read()
print(data)
# Output:
# b'<!doctype html>
# <html>
# <head>
# <title>Example Domain</title>
# ...'
In this example, we import the urlopen
function from the urllib.request module. We then use urlopen
to fetch the contents of 'http://example.com'
, read it, and print it. This prints the HTML content of the page.
This is a basic way to use urllib to fetch URLs in Python, but there’s much more to urllib than just this. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Fetching URLs with urllib: The Basics
- urllib: Handling Redirects, Cookies, and Authentication
- Exploring Alternatives: requests and httplib2
- Troubleshooting urllib: Handling Errors and Timeouts
- urllib Under the Hood: HTTP Protocol and URL Structure
- urllib in the Real World: Web Scraping and API Clients
- Wrapping Up: Mastering urllib for Fetching URLs in Python
Fetching URLs with urllib: The Basics
The urlopen
Function: Your Key to URL Fetching
One of the simplest ways to fetch URLs in Python is by using the urlopen
function from the urllib.request module. This function allows you to open and read the contents of a URL, similar to how you would open and read a file in Python.
Here’s an example of how you can use the urlopen
function:
from urllib.request import urlopen
url = 'http://example.com'
response = urlopen(url)
data = response.read()
print(data)
# Output:
# b'<!doctype html>
# <html>
# <head>
# <title>Example Domain</title>
# ...'
In this example, we’re opening the URL 'http://example.com'
using urlopen
and storing the response in the response
variable. We then read the contents of the response using the read
method and store it in the data
variable. Finally, we print the data which outputs the HTML content of the page.
Advantages of urlopen
The urlopen
function is straightforward and easy to use, making it a great choice for beginners. It’s also versatile and powerful, capable of handling different types of URLs including HTTP, FTP, and file URLs.
Potential Pitfalls of urlopen
While urlopen
is a powerful tool, it’s important to remember that it doesn’t handle more complex tasks such as cookie handling or authentication. For these tasks, you might need to use more advanced urllib features or alternative Python libraries.
urllib: Handling Redirects, Cookies, and Authentication
urllib and Redirects
In the world of web browsing, redirects are commonplace. urllib can handle these without breaking a sweat. Here’s how you can use urllib to handle redirects:
from urllib.request import urlopen
url = 'http://example.com'
response = urlopen(url)
if response.geturl() != url:
print('Redirected to', response.geturl())
# Output:
# Redirected to http://www.iana.org/domains/example
In this example, geturl
is used to find the final URL after all redirects. If the final URL is different from the original URL, it means a redirect has occurred.
urllib and Cookies
Cookies are small pieces of data stored on the client side. urllib can handle cookies through the http.cookiejar
module. Here’s an example:
from urllib.request import build_opener, HTTPCookieProcessor
from http.cookiejar import CookieJar
opener = build_opener(HTTPCookieProcessor(CookieJar()))
response = opener.open('http://example.com')
# Output:
# <http.client.HTTPResponse object at 0x7f8b0c2a3d60>
In this example, HTTPCookieProcessor
is used with CookieJar
to handle cookies. The build_opener
function is then used to open URLs that will handle cookies.
urllib and Authentication
urllib also supports authentication through the HTTPBasicAuthHandler
:
from urllib.request import HTTPPasswordMgrWithDefaultRealm, HTTPBasicAuthHandler, build_opener
password_mgr = HTTPPasswordMgrWithDefaultRealm()
password_mgr.add_password(None, 'http://example.com', 'username', 'password')
auth_handler = HTTPBasicAuthHandler(password_mgr)
opener = build_opener(auth_handler)
response = opener.open('http://example.com')
# Output:
# <http.client.HTTPResponse object at 0x7f8b0c2a3d60>
In this example, HTTPPasswordMgrWithDefaultRealm
and HTTPBasicAuthHandler
are used to handle HTTP authentication.
Exploring Alternatives: requests and httplib2
While urllib is a versatile tool for fetching URLs in Python, it’s not the only game in town. There are other Python libraries that offer similar functionalities, such as requests
and httplib2
. Let’s take a closer look at these alternatives.
The requests Library
The requests
library is a popular choice among Python developers due to its simplicity and user-friendly interface. Here’s how you can use requests
to fetch a URL:
import requests
response = requests.get('http://example.com')
print(response.text)
# Output:
# '<!doctype html>
# <html>
# <head>
# <title>Example Domain</title>
# ...'
In this example, we use the get
function from requests
to fetch the URL and print its content. This is similar to how we used urlopen
in urllib, but with a simpler, more intuitive interface.
The httplib2 Library
httplib2
is another library for fetching URLs in Python. It offers more advanced features like automatic redirection and cookie handling. Here’s an example:
import httplib2
h = httplib2.Http('.cache')
response, content = h.request('http://example.com')
print(content)
# Output:
# b'<!doctype html>
# <html>
# <head>
# <title>Example Domain</title>
# ...'
In this example, we create an Http
object and use its request
method to fetch the URL. The request
method returns a response object and the content of the URL.
Comparing urllib, requests, and httplib2
While all three libraries can fetch URLs, they each have their strengths and weaknesses. urllib is versatile and comes with Python, but it can be complex to use for advanced tasks. requests
is simple and user-friendly, but it doesn’t come with Python and needs to be installed separately. httplib2
offers advanced features, but it’s not as popular or well-documented as the other two.
Troubleshooting urllib: Handling Errors and Timeouts
urllib and Error Handling
When fetching URLs with urllib, you might encounter errors. These could be due to network issues, server problems, or invalid URLs. Here’s how you can handle errors with urllib:
from urllib.request import urlopen
from urllib.error import URLError
try:
response = urlopen('http://example.com')
except URLError as e:
print(f'Failed to fetch http://example.com: {e.reason}')
# Output:
# Failed to fetch http://example.com: [Errno -2] Name or service not known
In this example, we use a try/except block to catch URLError
, which is raised when urlopen
fails to fetch a URL. We then print the reason for the error using the reason
attribute of the exception.
urllib and Timeouts
Sometimes, a server might take too long to respond to a request. In these cases, you can use the timeout
parameter of urlopen
to specify how long to wait for a response before giving up:
from urllib.request import urlopen
from urllib.error import URLError
try:
response = urlopen('http://example.com', timeout=5)
except URLError as e:
print(f'Timed out after 5 seconds: {e.reason}')
# Output:
# Timed out after 5 seconds: timed out
In this example, we set the timeout
parameter to 5 seconds. If urlopen
doesn’t get a response within 5 seconds, it raises URLError
.
urllib Under the Hood: HTTP Protocol and URL Structure
Understanding the HTTP Protocol
To fully grasp how urllib works, it’s crucial to understand the Hypertext Transfer Protocol (HTTP). HTTP is the foundation of any data exchange on the web, and urllib leverages this protocol to fetch URLs.
Here’s a basic example of an HTTP request and response:
from urllib.request import urlopen
response = urlopen('http://example.com')
print('HTTP Response Code:', response.getcode())
print('HTTP Response Headers:', response.getheaders())
# Output:
# HTTP Response Code: 200
# HTTP Response Headers: [('Content-Type', 'text/html; charset=UTF-8'), ('Date', 'Mon, 01 Nov 2021 00:00:00 GMT'), ...]
In this example, urlopen
sends an HTTP GET request to ‘http://example.com’. The server then responds with an HTTP response. The getcode
method returns the HTTP response code (200 in this case, which means ‘OK’), and the getheaders
method returns the HTTP response headers.
Unraveling the Structure of URLs
A URL (Uniform Resource Locator) is essentially a web address. It’s a reference to a web resource that specifies its location on a computer network (like the internet) and a mechanism for retrieving it, such as HTTP.
A typical URL looks like this: 'http://www.example.com/path?query#fragment'
. It consists of several parts:
- Scheme: This is the protocol used to access the resource. In our example, it’s
'http'
. - Netloc: This is the network location, which is usually the hostname. In our example, it’s
'www.example.com'
. - Path: This is the path to the resource on the server. In our example, it’s
'/path'
. - Query: This is a string of information to be passed to the resource. In our example, it’s
'query'
. - Fragment: This is an internal page reference. In our example, it’s
'fragment'
.
Understanding the structure of URLs is crucial when working with urllib, as different parts of the URL can be manipulated to fetch different resources.
urllib in the Real World: Web Scraping and API Clients
urllib for Web Scraping
Web scraping is the process of extracting information from websites. urllib, with its ability to fetch URLs, can be a powerful tool for web scraping. Here’s a basic example of how you can use urllib for web scraping:
from urllib.request import urlopen
url = 'http://example.com'
html_content = urlopen(url).read().decode('utf-8')
print(html_content)
# Output:
# '<!doctype html>
# <html>
# <head>
# <title>Example Domain</title>
# ...'
In this example, we’re fetching the HTML content of 'http://example.com'
and decoding it to a string. This HTML content can then be parsed and analyzed to extract useful information.
urllib for API Clients
APIs (Application Programming Interfaces) are a way for different software applications to communicate with each other. urllib can be used to make HTTP requests to APIs and fetch the responses. Here’s an example:
from urllib.request import urlopen
import json
url = 'http://api.example.com/data'
response = urlopen(url)
data = json.loads(response.read().decode('utf-8'))
print(data)
# Output:
# {'key': 'value', 'key2': 'value2', ...}
In this example, we’re fetching a JSON response from an API and decoding it to a Python dictionary. This dictionary can then be used in our Python script.
Further Resources for Mastering urllib
Want to dive deeper into urllib and related topics? Here are some resources to help you on your journey:
- IOFlood’s Python Web Scraping Guide – Master the art of web scraping while respecting website policies.
Sending POST Requests in Python with requests.post() covers data submission and interaction with web services through POST requests.
HTML Parsing in Python: A Quick Guide – Learn about Python’s HTML parsing capabilities for web data extraction.
Python’s Official urllib Documentation: A comprehensive guide to urllib’s functions and methods.
Real Python’s Guide to Web Scraping: A practical introduction to web scraping in Python, including examples with urllib.
Python Guides on Web Scraping – Peruse this Python guide offering an overview of web scraping scenarios and practices.
Wrapping Up: Mastering urllib for Fetching URLs in Python
In this comprehensive guide, we’ve delved into the depths of urllib, a powerful tool in Python for fetching URLs. We’ve covered everything from the basics to advanced features, providing you with the knowledge to use urllib effectively in your projects.
We began with the basics, learning how to fetch URLs using urllib’s urlopen
function. We then explored more advanced features, such as handling redirects, cookies, and authentication. We also discussed alternatives to urllib, like requests
and httplib2
, giving you a broader perspective of the tools available for fetching URLs in Python.
Along the way, we tackled common challenges you might face when using urllib, such as handling errors and timeouts. We provided solutions and workarounds for these issues, equipping you with the skills to troubleshoot any problems that might arise.
We also talked about some alternative libraries to achieve similar results. Here’s a table describing the various options:
Library | Speed | Versatility | Ease of Use |
---|---|---|---|
urllib | Fast | High | Moderate |
requests | Moderate | High | High |
httplib2 | Fast | Moderate | Moderate |
Whether you’re just starting out with urllib or looking to level up your URL fetching skills, we hope this guide has given you a deeper understanding of urllib and its capabilities.
With urllib, you can fetch any URL right to your Python script, making it a powerful tool for tasks like web scraping and API clients. Now, you’re well equipped to harness the power of urllib in your Python projects. Happy coding!