Python HTML Parser Guide | BeautifulSoup and More
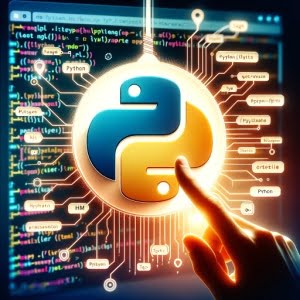
Are you finding it challenging to parse HTML in Python? You’re not alone. Many developers find themselves in a maze when it comes to handling HTML parsing in Python, but we’re here to help.
Think of Python as your personal web archaeologist, capable of digging through HTML documents to unearth the data you need. It’s a powerful tool that, when used correctly, can make your data extraction tasks a breeze.
This guide will walk you through the process of HTML parsing in Python, from the basics to more advanced techniques. We’ll cover everything from using BeautifulSoup for simple parsing tasks to handling more complex scenarios with other libraries like lxml and html.parser.
So, let’s get started and master HTML parsing in Python!
TL;DR: How Do I Parse HTML in Python?
Python’s BeautifulSoup library is commonly used for HTML parsing. You can parse an HTML document in Python by creating a BeautifulSoup object and passing the HTML document as a string to it, like
print(BeautifulSoup(html_doc, 'html.parser').prettify())
. Here’s a simple example:
from bs4 import BeautifulSoup
html_doc = """<html><head><title>The Dormouse's story</title></head>
<body><p class='title'><b>The Dormouse's story</b></p></body></html>"""
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.prettify())
# Output:
# <html>
# <head>
# <title>
# The Dormouse's story
# </title>
# </head>
# <body>
# <p class="title">
# <b>
# The Dormouse's story
# </b>
# </p>
# </body>
# </html>
In this example, we’re using BeautifulSoup to parse an HTML document. We create a BeautifulSoup object (soup) and pass the HTML document as a string to it. The ‘html.parser’ argument tells BeautifulSoup to use Python’s built-in HTML parser. The prettify()
method then formats the parsed HTML content in a way that’s easier to read.
This is a basic way to parse HTML in Python using BeautifulSoup, but there’s much more to learn about HTML parsing in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with BeautifulSoup
BeautifulSoup is a Python library that is used for web scraping purposes to pull the data out of HTML and XML files. It creates a parse tree from page source code that can be used to extract data in a hierarchical and more readable manner.
Parsing HTML with BeautifulSoup
Here’s how you can use BeautifulSoup for HTML parsing:
from bs4 import BeautifulSoup
# HTML document as a string
html_doc = """<html><head><title>The Title</title></head>
<body><p id='firstpara' align='center'>This is paragraph <b>one</b>.
<p id='secondpara' align='blah'>This is paragraph <b>two</b>.</p>
</body></html>"""
# Creating a BeautifulSoup object
soup = BeautifulSoup(html_doc, 'html.parser')
# Accessing tags
print(soup.title)
print(soup.p)
# Output:
# <title>The Title</title>
# <p id="firstpara" align="center">This is paragraph <b>one</b>.</p>
In this code block, we first import the BeautifulSoup class from the bs4 module. Then we create an instance of this class to parse our HTML document. The ‘html.parser’ argument tells BeautifulSoup to use Python’s built-in HTML parser.
We can then access the HTML tags in this parsed tree using dot notation. For instance, soup.title
gives us the content of the title tag and soup.p
gives us the content of the first p tag.
Pros and Cons of BeautifulSoup
BeautifulSoup is a powerful library that makes HTML parsing in Python much easier. It’s capable of handling malformed markup and provides simple, Pythonic idioms for navigating, searching, and modifying a parse tree.
However, it’s not the fastest library out there and can be a bit slow when dealing with large documents. It also doesn’t support XPath expressions out of the box, which can be a downside if you’re coming from a language like PHP or JavaScript where this is standard.
Advanced HTML Parsing Techniques with Python
As you get comfortable with basic HTML parsing in Python, you might find yourself needing to handle more complex scenarios. BeautifulSoup provides a variety of methods to help you deal with nested tags, search for specific elements, and handle different encodings.
Dealing with Nested Tags
HTML documents often contain nested tags. BeautifulSoup makes it easy to navigate through these nested structures. Here’s an example:
from bs4 import BeautifulSoup
html_doc = """<html><head><title>The Title</title></head>
<body><p id='firstpara' align='center'>This is paragraph <b>one</b>.
<p id='secondpara' align='blah'>This is paragraph <b>two</b>.</p>
</body></html>"""
soup = BeautifulSoup(html_doc, 'html.parser')
# Accessing nested tags
print(soup.body.b)
# Output:
# <b>one</b>
In this example, soup.body.b
gives us the first ‘b’ tag nested within the ‘body’ tag.
Searching for Specific Elements
BeautifulSoup provides powerful methods for searching the parse tree. You can find tags that match specific filters, navigate the tree based on tag names, and more. Here’s how you can find all ‘p’ tags in the document:
# Find all 'p' tags
p_tags = soup.find_all('p')
for tag in p_tags:
print(tag)
# Output:
# <p id="firstpara" align="center">This is paragraph <b>one</b>.</p>
# <p id="secondpara" align="blah">This is paragraph <b>two</b>.</p>
Handling Different Encodings
BeautifulSoup automatically converts incoming documents to Unicode and outgoing documents to UTF-8. You don’t have to think about encodings, unless the document doesn’t specify an encoding and BeautifulSoup can’t detect one. Then you’ll get a UnicodeDecodeError.
# To avoid this, you can specify an encoding when creating the BeautifulSoup object:
soup = BeautifulSoup(html_doc, 'html.parser', from_encoding='iso-8859-1')
In this example, we’re telling BeautifulSoup to use the ‘iso-8859-1’ encoding when parsing the document.
Exploring Alternative Libraries for HTML Parsing
While BeautifulSoup is a popular choice for HTML parsing in Python, it’s not the only option. Other libraries such as lxml and html.parser can also be used for HTML parsing. Here’s a brief overview of these alternative approaches.
Parsing HTML with lxml
lxml is a library for processing XML and HTML. It’s very fast and easy to use. Here’s how you can parse an HTML document with lxml:
from lxml import html
# HTML document as a string
html_doc = """<html><head><title>The Title</title></head>
<body><p id='firstpara' align='center'>This is paragraph <b>one</b>.
<p id='secondpara' align='blah'>This is paragraph <b>two</b>.</p>
</body></html>"""
# Parse the document
tree = html.fromstring(html_doc)
# Accessing tags
print(tree.xpath('//title/text()'))
print(tree.xpath('//p/text()'))
# Output:
# ['The Title']
# ['This is paragraph ', '.
', 'This is paragraph ', '.
']
In this example, we’re using the html.fromstring
function to parse our HTML document. We then use the xpath
method to access the content of the title and p tags.
Parsing HTML with html.parser
html.parser is a built-in Python library for parsing HTML. It’s not as fast as lxml, but it doesn’t require any additional installations. Here’s an example of how to use it:
from html.parser import HTMLParser
# Create a subclass and override the handler methods
class MyHTMLParser(HTMLParser):
def handle_starttag(self, tag, attrs):
print("Encountered a start tag:", tag)
def handle_endtag(self, tag):
print("Encountered an end tag :")
def handle_data(self, data):
print("Encountered some data :", data)
# Instantiate the parser and fed it some HTML
parser = MyHTMLParser()
parser.feed('<html><head><title>Test</title></head><body><h1>Parse me!</h1></body></html>')
# Output:
# Encountered a start tag: html
# Encountered a start tag: head
# Encountered a start tag: title
# Encountered some data : Test
# Encountered an end tag :
# Encountered an end tag :
# Encountered a start tag: body
# Encountered a start tag: h1
# Encountered some data : Parse me!
# Encountered an end tag :
# Encountered an end tag :
# Encountered an end tag :
In this example, we’re creating a custom parser by subclassing HTMLParser
and overriding its methods. We then use the feed
method to parse an HTML document.
Making the Right Choice
Choosing the right library for HTML parsing depends on your specific needs.
- BeautifulSoup is a great choice for beginners due to its simplicity and ease of use.
lxml is a good option if you need speed and don’t mind installing an additional library.
html.parser, while not as fast or easy to use as the other two, is a built-in option that doesn’t require any additional installations.
Troubleshooting Common HTML Parsing Issues
While HTML parsing in Python is generally straightforward, you may encounter some common issues. Let’s discuss how to deal with malformed HTML, handle different encodings, and manage dynamic content.
Dealing with Malformed HTML
Not all HTML is well-formed, and this can cause issues when parsing. BeautifulSoup is quite forgiving and can handle malformed HTML. However, for particularly problematic documents, you might need to clean up the HTML before parsing it. Here’s an example:
from bs4 import BeautifulSoup
from bs4 import SoupStrainer
malformed_html = '<html><head><title>Page Title</head><body>Body content'<p>Paragraph'</p>'
# Only parse part of the document
tag = SoupStrainer('p')
soup = BeautifulSoup(malformed_html, 'html.parser', parse_only=tag)
print(soup.prettify())
# Output:
# <p>
# Paragraph
# </p>
In this example, we’re using the SoupStrainer
class to parse only part of the document. This can be useful when dealing with large documents or if you’re only interested in certain parts of the document.
Handling Different Encodings
As mentioned before, BeautifulSoup automatically converts incoming documents to Unicode and outgoing documents to UTF-8. However, if the document doesn’t specify an encoding and BeautifulSoup can’t detect one, you’ll get a UnicodeDecodeError. To avoid this, you can specify an encoding when creating the BeautifulSoup object:
soup = BeautifulSoup(html_doc, 'html.parser', from_encoding='iso-8859-1')
Dealing with Dynamic Content
HTML parsing can get tricky when dealing with dynamic content, such as JavaScript-generated HTML. In these cases, you might need to use a tool like Selenium to render the JavaScript before parsing the HTML. Here’s a basic example:
from selenium import webdriver
from bs4 import BeautifulSoup
# Create a new instance of the Firefox driver
driver = webdriver.Firefox()
# Go to the page that we want to scrape
driver.get('http://www.example.com')
# Parse the HTML with BeautifulSoup
soup = BeautifulSoup(driver.page_source, 'html.parser')
# Close the browser
ndriver.quit()
In this example, we’re using Selenium to render the JavaScript on a page before parsing the HTML with BeautifulSoup. This allows us to scrape dynamic content that would otherwise be inaccessible with standard HTML parsing techniques.
Understanding HTML Parsing Fundamentals
HTML parsing is a method used to extract information from a website by translating the HTML file into a readable format. This process is a fundamental aspect of many applications on the internet, including web scraping, web automation, and data extraction.
What is HTML Parsing?
HTML parsing involves taking in an HTML file and outputting a structured format that can be manipulated and analyzed. The parser reads through the HTML document and identifies the different elements (like tags, attributes, and their values), creating a parse tree—a tree data structure that represents the document.
from bs4 import BeautifulSoup
# HTML document as a string
html_doc = """<html><head><title>The Title</title></head>
<body><p id='firstpara' align='center'>This is paragraph <b>one</b>.</p>
</body></html>"""
# Creating a BeautifulSoup object
soup = BeautifulSoup(html_doc, 'html.parser')
# Accessing tags
print(soup.title)
print(soup.p)
# Output:
# <title>The Title</title>
# <p id="firstpara" align="center">This is paragraph <b>one</b>.</p>
In this example, we’re using BeautifulSoup to parse an HTML document. The parser identifies the different elements in the HTML string and creates a parse tree that we can navigate and extract information from.
Why is HTML Parsing Useful?
HTML parsing is extremely useful for web scraping, where you need to extract information from a website. It’s also used in web automation (where bots imitate human actions), and in creating dynamic web pages.
Underlying Concepts of HTML Parsing
HTML parsing relies on understanding the structure of HTML documents. HTML documents are structured as a tree of nodes, with the ‘html’ tag as the root, and other tags (like ‘head’, ‘body’, ‘title’, ‘div’, ‘span’, etc.) as child nodes. This structure is known as the Document Object Model (DOM).
HTML parsers work by reading this tree structure, identifying the different nodes, and creating a parse tree that represents the HTML document. This parse tree can then be used to extract information, modify the HTML, or generate a visual representation of the page.
Understanding these fundamentals of HTML parsing will help you get the most out of Python’s HTML parsing libraries and handle more complex HTML parsing tasks.
Expanding Your HTML Parsing Skills
Understanding HTML parsing in Python and its libraries like BeautifulSoup, lxml, and html.parser is just the beginning. There are many ways you can apply these skills in larger projects and further enhance your Python expertise.
HTML Parsing in Web Scraping
HTML parsing is a crucial part of web scraping, where it’s used to extract valuable data from websites. With Python’s HTML parsing libraries, you can navigate through the HTML of a webpage, locate the data you need, extract it, and even store it for later use.
from bs4 import BeautifulSoup
import requests
# Make a request to the website
r = requests.get('http://www.example.com')
# Parse the HTML content
soup = BeautifulSoup(r.text, 'html.parser')
# Find a specific element using its tag name and (optional) its attributes
first_paragraph = soup.find('p')
print(first_paragraph.text)
# Output:
# 'This is the first paragraph of the webpage.'
In this example, we’re using the requests
library to retrieve the HTML content of a webpage. We then parse this content with BeautifulSoup and use the find
method to locate the first paragraph on the page.
HTML Parsing in Data Mining
In data mining, HTML parsing can be used to collect and analyze data from the web. This can involve anything from sentiment analysis on customer reviews to trend identification based on news articles.
HTML Parsing in Web Automation
Web automation involves programming a software or bot to interact with web pages just like a human would. HTML parsing plays a key role in this by allowing the bot to understand the structure of the web pages it interacts with.
Further Resources for Mastering HTML Parsing in Python
If you’re interested in learning more about HTML parsing in Python, here are some valuable resources:
- Python Web Scraping for Data Extraction – Explore web scraping for data collection, analysis, and research.
URL Operations with Python urllib – Learn how to fetch data from the web and work with URLs in Python using urllib.
Making HTTP Requests with Python’s curl – Learn how to connect to websites and fetch data with Python’s “curl.”
Beautiful Soup Documentation – The official documentation for BeautifulSoup covers how to use the library effectively.
Python lxml Tutorial provides an in-depth look at how to use the lxml library for HTML and XML parsing.
Web Scraping with Python tutorial on Real Python goes beyond HTML parsing to cover the entire process of web scraping.
Remember, mastering HTML parsing in Python opens up a world of possibilities in web scraping, data mining, and web automation. Happy coding!
Wrapping Up: Mastering HTML Parsing in Python
In this comprehensive guide, we’ve explored the ins and outs of HTML parsing in Python, providing you with the knowledge you need to extract valuable data from HTML documents.
We began with the basics, learning how to use Python’s BeautifulSoup library for simple HTML parsing tasks. We then delved into more advanced techniques, such as dealing with nested tags, searching for specific elements, and handling different encodings.
Along the way, we tackled common issues you might encounter when parsing HTML in Python, such as dealing with malformed HTML and dynamic content, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to HTML parsing in Python, comparing BeautifulSoup with other libraries like lxml and html.parser.
Here’s a quick comparison of these libraries:
Library | Ease of Use | Speed | Flexibility |
---|---|---|---|
BeautifulSoup | High | Moderate | High |
lxml | Moderate | High | Moderate |
html.parser | Moderate | Moderate | Low |
Whether you’re just starting out with HTML parsing in Python or you’re looking to refine your skills, we hope this guide has given you a deeper understanding of how to effectively parse HTML documents in Python.
With its balance of ease of use, speed, and flexibility, Python is a powerful tool for HTML parsing. Now, you’re well equipped to tackle any HTML parsing task that comes your way. Happy coding!