How to Throw Exceptions in Java | Detailed Tutorial
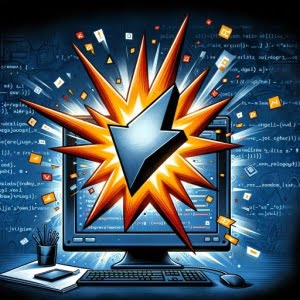
Are you finding it difficult to throw exceptions in Java? You’re not alone. Many developers find themselves in a quandary when it comes to handling exceptions in Java, but we’re here to help.
Think of Java’s exception handling as a referee in a game – enforcing rules and managing unexpected situations. Exception handling in Java is a powerful tool that allows us to control the flow of the program and maintain its robustness.
In this guide, we’ll walk you through the process of throwing exceptions in Java, from the basics to more advanced techniques. We’ll cover everything from using the throw
keyword, handling different types of exceptions, to discussing alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering Java exception handling!
TL;DR: How Do I Throw an Exception in Java?
To throw an exception in Java, you use the
throw
keyword followed by an instance of the exception:throw new Exception('This is an exception');
This is a fundamental aspect of error handling in Java, allowing you to control the flow of your program and handle unexpected situations.
Here’s an example:
public class Main {
public static void main(String[] args) {
try {
int[] arr = {1, 2, 3};
System.out.println(arr[3]);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("An exception occurred: " + e);
} finally {
System.out.println("This part always executes, exception or not.");
}
}
}
# Output:
# An exception occurred: java.lang.ArrayIndexOutOfBoundsException: Index 3 out of bounds for length 3
# This part always executes, exception or not.
In this example, attempting to access the non-existent fourth element of a three-element array triggers an ArrayIndexOutOfBoundsException
. The catch
block then handles this exception and prints an appropriate message. Regardless of whether or not an exception was caught, the code inside the finally
block is always executed.
This is an intermediate way to throw an exception in Java, but there’s much more to learn about error handling in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Throwing a Basic Exception in Java
- Throwing Different Types of Exceptions in Java
- Exploring Alternative Error Handling Methods in Java
- Troubleshooting Common Issues with Java Exceptions
- Understanding the Fundamentals of Java Exceptions
- Broadening the Scope: Exception Handling in Larger Java Applications
- Wrapping Up: Mastering Java Exceptions
Throwing a Basic Exception in Java
In Java, exceptions are events that disrupt the normal flow of the program. Throwing an exception is the process of creating an exception object and handing it off to the runtime system. To throw a basic exception in Java, you use the throw
keyword.
The throw
keyword in Java is used to explicitly throw an exception from a method or any block of code. We can throw either checked or unchecked exceptions. The throw
keyword is mainly used to throw custom exceptions.
Here is a simple example of throwing a basic exception in Java:
public class Main {
public static void main(String[] args) {
try {
throw new Exception('This is a basic exception');
} catch (Exception e) {
e.printStackTrace();
}
}
}
# Output:
# java.lang.Exception: This is a basic exception
# at Main.main(Main.java:4)
In this example, we’ve used the throw
keyword to throw a new instance of an Exception. The exception is then caught and handled in the catch block, where we print the stack trace to the console. This allows us to see where the exception occurred and can be very useful for debugging.
While the throw
keyword provides us with a powerful tool for handling unexpected situations, it’s important to use it carefully. Overuse of the throw
keyword can lead to code that is difficult to read and maintain. It’s also important to remember that not all exceptions should be caught – sometimes, it’s appropriate to let an exception bubble up to a higher level of your program where it can be handled more appropriately.
Throwing Different Types of Exceptions in Java
Java provides several types of exceptions to cater to specific error scenarios. Two commonly used exceptions are RuntimeException
and IOException
. Let’s delve into how to throw these exceptions and analyze their output.
Throwing a RuntimeException
RuntimeException
is the superclass of those exceptions that can be thrown during the normal operation of the Java Virtual Machine. Here’s how you can throw a RuntimeException
:
public class Main {
public static void main(String[] args) {
try {
throw new RuntimeException('This is a runtime exception');
} catch (RuntimeException e) {
e.printStackTrace();
}
}
}
# Output:
# java.lang.RuntimeException: This is a runtime exception
# at Main.main(Main.java:4)
In this example, we’ve thrown a RuntimeException
. It’s caught and handled in the same way as our previous Exception
.
Throwing an IOException
IOException
is used for handling input-output exceptions. Here’s an example:
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
throw new IOException('This is an IO exception');
} catch (IOException e) {
e.printStackTrace();
}
}
}
# Output:
# java.io.IOException: This is an IO exception
# at Main.main(Main.java:6)
In this case, we’ve thrown an IOException
. This type of exception is typically thrown when an input-output operation fails or is interrupted.
Throwing different types of exceptions allows you to handle specific error scenarios more effectively. It’s good practice to use the most specific exception applicable to the error situation. By doing so, you can provide more information about the error to the caller, making it easier to diagnose and fix the problem.
Exploring Alternative Error Handling Methods in Java
While throwing exceptions is a common way to handle errors in Java, there are alternative methods that can be used in certain situations. These include using assertions and error codes. Let’s explore these alternatives and their effectiveness.
Using Assertions
Assertions are used for debugging purposes. An assertion is a statement in Java which ensures the correctness of any assumptions that have been made in the program.
Here’s an example of using assertions in Java:
public class Main {
public static void main(String[] args) {
int value = 15;
assert value >= 20 : 'Value is less than 20';
}
}
# Output:
# Exception in thread 'main' java.lang.AssertionError: Value is less than 20
In this example, an AssertionError is thrown as the value is less than 20. Assertions are typically enabled during testing but disabled during deployment for performance reasons.
Using Error Codes
Another alternative approach is to use error codes. Instead of throwing an exception, a method may return an error code to indicate that an error occurred.
public class Main {
public static int divide(int a, int b) {
if (b == 0) {
return -1; // return error code
} else {
return a / b;
}
}
public static void main(String[] args) {
int result = divide(10, 0);
if (result == -1) {
System.out.println('Error occurred');
}
}
}
# Output:
# Error occurred
In this example, the divide
method returns an error code -1
when division by zero is attempted. This error code is then checked in the main
method to handle the error.
While error codes can be a simple and efficient way to handle errors, they can make code harder to read and maintain as it’s not immediately clear what an error code means. Therefore, they are generally not recommended for use in Java.
In conclusion, while throwing exceptions is the most common way to handle errors in Java, there are alternative methods available. The choice of method depends on the specific requirements of your program and the context in which the error handling is taking place. It’s important to understand the advantages and disadvantages of each method and choose the one that best fits your needs.
Troubleshooting Common Issues with Java Exceptions
Throwing exceptions in Java is a powerful tool for controlling the flow of your program and handling unexpected situations. However, there are common issues that you may encounter when working with exceptions. Let’s discuss these problems and provide some solutions and workarounds.
Dealing with Unhandled Exceptions
An unhandled exception occurs when an exception is thrown, but there is no appropriate catch block to handle it. This typically results in the termination of the program.
Here’s an example of an unhandled exception:
public class Main {
public static void main(String[] args) {
throw new RuntimeException('Unhandled exception');
}
}
# Output:
# Exception in thread 'main' java.lang.RuntimeException: Unhandled exception
# at Main.main(Main.java:3)
The solution to this problem is to add a catch block that can handle the exception:
public class Main {
public static void main(String[] args) {
try {
throw new RuntimeException('Now handled exception');
} catch (RuntimeException e) {
e.printStackTrace();
}
}
}
# Output:
# java.lang.RuntimeException: Now handled exception
# at Main.main(Main.java:4)
In this modified example, the RuntimeException is now caught and handled, preventing the program from terminating unexpectedly.
Understanding Exception Hierarchies
Java’s exception hierarchy can sometimes cause confusion. For example, if you have a catch block for Exception (which is a superclass of all exceptions), it will catch subclasses of Exception as well.
This can lead to unexpected behavior if you have multiple catch blocks for different exception types, as the first catch block that can handle the exception will be executed.
Here’s an example:
public class Main {
public static void main(String[] args) {
try {
throw new RuntimeException('This is a runtime exception');
} catch (Exception e) {
System.out.println('Caught by Exception catch block');
} catch (RuntimeException e) {
System.out.println('Caught by RuntimeException catch block');
}
}
}
# Output:
# Caught by Exception catch block
Even though we threw a RuntimeException, it was caught by the catch block for Exception. This is because Exception is a superclass of RuntimeException, and the catch block for Exception was listed first.
To avoid this problem, you should list catch blocks from most specific to least specific. That way, exceptions will be caught by the most specific catch block that can handle them.
public class Main {
public static void main(String[] args) {
try {
throw new RuntimeException('This is a runtime exception');
} catch (RuntimeException e) {
System.out.println('Caught by RuntimeException catch block');
} catch (Exception e) {
System.out.println('Caught by Exception catch block');
}
}
}
# Output:
# Caught by RuntimeException catch block
In this modified example, the RuntimeException is caught by the catch block for RuntimeException, as it is listed before the catch block for Exception.
Understanding the Fundamentals of Java Exceptions
Before we delve deeper into how to throw exceptions in Java, it’s essential to understand the fundamentals of exceptions in Java, including the exception hierarchy, checked and unchecked exceptions, and the theory behind exceptions.
Exception Hierarchy in Java
In Java, all exception classes are subtypes of the java.lang.Exception
class. The exception class is a subclass of the Throwable
class. Other than the exception class, there is another subclass called Error
which is used by the Java run-time system (JVM) to indicate errors that a reasonable application should not try to catch.
The exception class has two main subclasses: IOException
class and RuntimeException
class. IOException
class is used for handling input-output exceptions, and RuntimeException
class is used for runtime exceptions.
Checked vs Unchecked Exceptions
In Java, there are two types of exceptions: checked and unchecked exceptions.
- Checked exceptions are exceptions that are checked at compile time. If some code within a method throws a checked exception, then the method must either handle the exception or it must specify the exception using the
throws
keyword. Unchecked exceptions are exceptions that are not checked at compiled time. In Java exceptions under
Error
andRuntimeException
classes are unchecked exceptions, everything else underThrowable
is checked.
The Theory Behind Exceptions
Exceptions in Java provide a way to handle the runtime errors so that normal flow of the application can be maintained.
In theory, when an error occurs within a method, the method creates an object and hands it off to the runtime system. The object, called an exception object, contains information about the error, including its type and the state of the program when the error occurred. Creating an exception object and handing it to the runtime system is called throwing an exception.
After a method throws an exception, the runtime system attempts to find something to handle it. The set of possible ‘somethings’ to handle the exception is the ordered list of methods that had been called to get to the method where the error occurred. The list of methods is known as the call stack.
In conclusion, understanding the fundamentals of exceptions in Java, including the exception hierarchy, checked and unchecked exceptions, and the theory behind exceptions, is crucial before you start throwing exceptions in Java. This knowledge will help you to effectively handle exceptions and maintain the robustness of your Java applications.
Broadening the Scope: Exception Handling in Larger Java Applications
Throwing exceptions is not just a concept that is confined to small programs; it is a fundamental part of larger applications as well. It plays a crucial role in maintaining the robustness and reliability of an application. When dealing with larger applications, error handling becomes even more critical as a single unhandled exception can cause the entire application to crash.
Exploring Related Concepts
While this guide focuses on how to throw exceptions in Java, it’s just a single facet of error handling in Java. There are other related concepts that you should explore to get a comprehensive understanding of error handling in Java. These include:
- Try-Catch Blocks: These are used to handle exceptions by separating the code that might throw an exception from the code that handles the exception.
Finally Blocks: A finally block is a block of code that is executed regardless of whether an exception is thrown or not. It is often used to clean up resources.
Here is a simple example that uses a try-catch-finally block:
public class Main {
public static void main(String[] args) {
try {
throw new RuntimeException('This is a runtime exception');
} catch (RuntimeException e) {
System.out.println('Caught by RuntimeException catch block');
} finally {
System.out.println('Finally block is executed');
}
}
}
# Output:
# Caught by RuntimeException catch block
# Finally block is executed
In this example, the RuntimeException is caught by the catch block, and the finally block is executed regardless of whether an exception was thrown.
Further Resources for Mastering Java Exceptions
To deepen your understanding of exceptions and error handling in Java, here are some external resources that you might find helpful:
- Java Error Handling Best Practices – Understand Java error reporting mechanisms.
Exploring Try-Catch Mechanism in Java – Learn syntax and usage of try and catch statements.
Understanding NullPointerException in Java – Understand the NullPointerException in Java.
Oracle’s Java Tutorials on Exceptions provides a comprehensive overview of exceptions in Java.
Baeldung’s Guide on Java Exceptions provides a deep dive into Java exceptions, including multi-catch statements.
GeeksforGeeks’ Java Exception Handling Articles covers various aspects of exception handling in Java, including custom exceptions and best practices.
Wrapping Up: Mastering Java Exceptions
In this comprehensive guide, we’ve explored every nook and cranny of throwing exceptions in Java, providing you with the knowledge and tools you need to handle unexpected situations in your Java programs effectively.
We started off with the basics, learning how to throw a basic exception using the throw
keyword. We then delved into more advanced topics, such as throwing different types of exceptions like RuntimeException
and IOException
, and explored alternative approaches to handle errors in Java, such as using assertions and error codes.
Throughout our journey, we also tackled common issues that you might encounter when throwing exceptions, such as unhandled exceptions and issues with exception hierarchies, providing you with solutions and workarounds for each problem.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Throwing Exceptions | Direct, customizable error handling | Can lead to complex code if overused |
Using Assertions | Great for debugging | Should be disabled in production code |
Using Error Codes | Simple, efficient error handling | Can make code harder to read and maintain |
Whether you’re just starting out with Java or you’re a seasoned developer looking to deepen your understanding of exceptions, we hope this guide has been a valuable resource. With the knowledge you’ve gained, you’re now well-equipped to handle any unexpected situation that arises in your Java programs.
Remember, mastery comes with practice. So, keep coding, keep experimenting, and most importantly, don’t be afraid to make mistakes. After all, that’s what exceptions are for!