Python defaultdict | Usage, Examples, and Alternatives
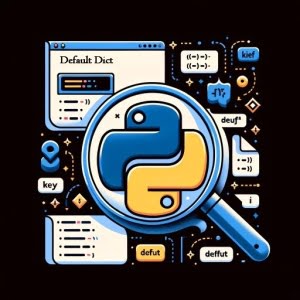
Are you finding it challenging to work with collections in Python? You’re not alone. Many developers find themselves puzzled when it comes to managing collections efficiently. But, think of Python’s defaultdict as a diligent secretary – always ready to manage your collections without a hitch.
Whether you’re dealing with simple lists or complex nested data structures, understanding how to use defaultdict in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of using defaultdict in Python, from the basics to more advanced techniques. We’ll cover everything from specifying default values, using it with different data types, to alternative methods to achieve the same results.
Let’s dive in and start mastering Python defaultdict!
TL;DR: What is defaultdict in Python and How to Use It?
defaultdict is a container in Python’s collections module that provides default values for nonexistent keys. It’s a subclass of the built-in dict class in Python, but it overrides one method and adds one writable instance variable.
Here’s a simple example:
from collections import defaultdict
d = defaultdict(int)
d['key'] += 1
print(d)
# Output:
# defaultdict(<class 'int'>, {'key': 1})
In this example, we import the defaultdict from the collections module. We then create a defaultdict with int as the default factory. This means that if a key is not found in the dictionary, it will be created with a default value of 0 (since int() returns 0). We then increment the value of the key ‘key’ by 1 and print the dictionary. The output shows that the key ‘key’ has been created with a value of 1.
This is just a basic usage of defaultdict in Python. There’s much more to learn about defaultdict, including how to use it with different data types and in nested data structures, as well as alternative methods to achieve the same results. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Python defaultdict: The Basics
How to Use defaultdict in Python
Before we dive into the details, it’s essential to understand what defaultdict is. In Python, defaultdict is a container that provides default values for nonexistent keys, which means you won’t get a KeyError when you try to access a key that doesn’t exist in the dictionary.
To use defaultdict in Python, you need to import it from the collections module, like so:
from collections import defaultdict
Specifying Default Values in defaultdict
The defaultdict takes a function or a default factory as an argument when you create it. This function or default factory is used to provide a default value for a key that doesn’t exist.
Here’s a simple example:
d = defaultdict(int)
In this case, int is the default factory, and it returns 0 when called with no arguments. So, if you try to access a key that doesn’t exist in the dictionary, it will be created with a default value of 0.
Let’s see it in action:
d = defaultdict(int)
d['key'] += 1
print(d)
# Output:
# defaultdict(<class 'int'>, {'key': 1})
Here, we’re incrementing the value of the key ‘key’ by 1. Since ‘key’ doesn’t exist in the dictionary, it’s created with a default value of 0 (from the int default factory), and then incremented by 1.
Advantages of Using defaultdict
The main advantage of using defaultdict over a regular dictionary is that it allows you to provide default values for keys, which can help avoid KeyError exceptions. It also simplifies your code and makes it more readable.
Pitfalls of Using defaultdict
While defaultdict is incredibly useful, it’s important to note that it might not always be the best solution. For example, if you need to check whether a key exists in the dictionary before performing an operation, using a regular dictionary might be a better option. Also, remember that the default factory must be a function that can be called with no arguments.
Python defaultdict: Advanced Techniques
Using defaultdict with Different Data Types
The power of defaultdict becomes more apparent when you start using it with different data types. For instance, you can use it with lists, sets, or even other dictionaries.
Consider this example where we use defaultdict with a list:
from collections import defaultdict
d = defaultdict(list)
d['key'].append(1)
print(d)
# Output:
# defaultdict(<class 'list'>, {'key': [1]})
In this example, we’re using list as the default factory. This means that if a key doesn’t exist in the dictionary, it will be created with a default value of an empty list. We then append the number 1 to the ‘key’, and print the dictionary. The output shows that the key ‘key’ has been created with a value of a list containing 1.
Using defaultdict in Nested Data Structures
Another powerful feature of defaultdict is its ability to be used in nested data structures. This can be particularly useful when you’re dealing with complex data.
Here’s an example of how you can use defaultdict in a nested data structure:
from collections import defaultdict
nested_dict = defaultdict(lambda: defaultdict(int))
nested_dict['outer']['inner'] += 1
print(nested_dict)
# Output:
# defaultdict(<function <lambda> at 0x7f1c6c2c6c20>, {'outer': defaultdict(<class 'int'>, {'inner': 1})})
In this example, we’re creating a defaultdict with a lambda function as the default factory. This lambda function returns another defaultdict with int as the default factory. We then increment the value of the key ‘inner’ inside the key ‘outer’ by 1, and print the dictionary. The output shows a nested defaultdict, with ‘outer’ as the outer key and ‘inner’ as the inner key, which has a value of 1.
These advanced techniques can help you manage complex data structures more efficiently and with less code.
Alternative Methods to Python defaultdict
While defaultdict is a powerful tool, Python offers other ways to achieve similar results. Let’s explore some of these alternatives and their pros and cons.
Using dict.get() Method
The dict.get() method is one way to provide default values for keys in a dictionary. This method returns the value for a key if it exists in the dictionary; otherwise, it returns a default value.
Here’s an example:
d = {}
value = d.get('key', 0)
print(value)
# Output:
# 0
In this example, we’re trying to get the value for the key ‘key’ from the dictionary d. Since ‘key’ doesn’t exist in the dictionary, the dict.get() method returns the default value of 0.
The advantage of the dict.get() method is its simplicity. However, it can make your code more verbose if you’re frequently accessing keys that might not exist in the dictionary.
Using try/except Blocks
Another alternative to defaultdict is using try/except blocks to handle KeyError exceptions.
Consider this example:
d = {}
try:
value = d['key']
except KeyError:
value = 0
print(value)
# Output:
# 0
In this case, we’re trying to access the key ‘key’ from the dictionary d inside a try block. If ‘key’ doesn’t exist in the dictionary, a KeyError exception is raised, and we handle it in the except block by setting the value to 0.
The advantage of using try/except blocks is that they make it explicit that you’re handling potential KeyError exceptions. However, they can also make your code more complex and harder to read, especially if you’re dealing with nested data structures.
In conclusion, while defaultdict is a powerful tool for managing collections in Python, it’s not always the best solution. Depending on your specific needs, you might find that using the dict.get() method or try/except blocks is a better approach.
Troubleshooting Python defaultdict: Common Issues and Solutions
While Python’s defaultdict is a powerful tool, it’s not without its quirks. Let’s delve into some common issues you might encounter when using defaultdict and how to resolve them.
Handling Type Errors
One common issue when using defaultdict is encountering a TypeError. This can happen if you try to use a non-callable object as the default factory.
Consider this example:
from collections import defaultdict
d = defaultdict('default')
# Output:
# TypeError: first argument must be callable or None
In this case, we’re trying to use the string ‘default’ as the default factory. However, since ‘default’ is not callable, a TypeError is raised.
To fix this issue, you can use a callable object as the default factory. If you want to use a specific string as the default value, you can use a lambda function:
from collections import defaultdict
d = defaultdict(lambda: 'default')
value = d['key']
print(value)
# Output:
# 'default'
In this corrected example, we’re using a lambda function that returns the string ‘default’ as the default factory. When we try to access the key ‘key’ that doesn’t exist in the dictionary, it’s created with a default value of ‘default’.
Dealing with Key Errors
While defaultdict is designed to prevent KeyError exceptions, you might still encounter them in some cases. For example, if you try to access a key from the dictionary before you’ve assigned a default factory.
Here’s an example:
from collections import defaultdict
d = defaultdict()
value = d['key']
# Output:
# KeyError: 'key'
In this case, we’re trying to access the key ‘key’ from the dictionary before we’ve assigned a default factory. As a result, a KeyError is raised.
To avoid this issue, always make sure to assign a default factory when you create a defaultdict:
from collections import defaultdict
d = defaultdict(int)
value = d['key']
print(value)
# Output:
# 0
In this corrected example, we’re assigning int as the default factory when we create the defaultdict. Thus, when we try to access the key ‘key’ that doesn’t exist in the dictionary, it’s created with a default value of 0 (the result of calling int() with no arguments).
Remember, while defaultdict can simplify your code and make it more efficient, it’s crucial to understand its quirks and how to troubleshoot common issues. With the right knowledge and understanding, you can use defaultdict to effectively manage collections in Python.
Python’s Collections Module and defaultdict
Understanding Python’s Collections Module
Python’s collections module is a powerful toolkit that offers container data types alternatives to Python’s general purpose built-in containers. It includes several classes that can make your life as a developer significantly easier.
One of these classes is defaultdict, a dictionary subclass that provides a default value for nonexistent keys.
The defaultdict Data Type
As mentioned, defaultdict is a subclass of the built-in dict class in Python. It overrides one method and adds one writable instance variable. The added instance variable is a callable object that provides default values for nonexistent keys, and the overridden method is missing(), which defaultdict uses to handle missing keys.
Here’s a simple example:
from collections import defaultdict
d = defaultdict(int)
print(d['key'])
# Output:
# 0
In this example, we’re creating a defaultdict with int as the default factory, which means it returns 0 when called with no arguments. When we try to access the key ‘key’ that doesn’t exist in the dictionary, it’s created with a default value of 0.
When to Use defaultdict Over Regular Dictionaries
While regular dictionaries are powerful and flexible, they have one key limitation: they raise a KeyError when you try to access a key that doesn’t exist in the dictionary. This is where defaultdict comes in.
With defaultdict, you can provide a default value for keys, which can help avoid KeyError exceptions and simplify your code. This makes defaultdict particularly useful when you’re dealing with collections of items, such as lists or sets, and you need to initialize your collection before you can perform any operations on it.
However, it’s important to note that defaultdict might not always be the best solution. For example, if you need to check whether a key exists in the dictionary before performing an operation, using a regular dictionary might be a better option.
defaultdict in Real-World Applications
defaultdict in Data Processing
In the world of data processing, defaultdict can be a game-changer. Its ability to provide default values for nonexistent keys makes it a powerful tool for handling large datasets. Whether you’re grouping data, counting occurrences, or building complex data structures, defaultdict can help you write more efficient and readable code.
For example, you can use defaultdict to count the occurrences of words in a text:
from collections import defaultdict
text = 'one fish two fish red fish blue fish'
words = text.split()
counter = defaultdict(int)
for word in words:
counter[word] += 1
print(counter)
# Output:
# defaultdict(<class 'int'>, {'one': 1, 'fish': 4, 'two': 1, 'red': 1, 'blue': 1})
In this example, we’re splitting the text into words, and then using a defaultdict with int as the default factory to count the occurrences of each word. The output shows the number of times each word appears in the text.
defaultdict in Web Scraping
Web scraping is another area where defaultdict can be incredibly useful. When you’re scraping data from a website, you often need to handle missing data. defaultdict allows you to provide default values for missing data, which can help you avoid errors and simplify your code.
Exploring Other Data Structures in the Collections Module
While defaultdict is a powerful tool, it’s just one of the many data structures available in Python’s collections module. Other data structures, such as namedtuple, deque, and Counter, also offer unique features that can help you write more efficient and readable code. Exploring these data structures can help you further improve your Python skills.
Further Resources for Mastering Python defaultdict
If you’re interested in learning more about defaultdict and other data structures in Python’s collections module, here are some resources you might find helpful:
- Simplifying Data Management with Python Dictionaries – Explore advanced uses of Python dictionaries to take your coding skills to the next level.
Harnessing the Power of Hash Tables in Python – Master the art of using hashmaps in Python for efficient data storage.
Enhancing Your Code with Python Dictionary Methods – Dive deep into Python dictionary methods to simplify data management.
Python’s collections module documentation – The official Python documentation is always a great place to start. It provides a comprehensive overview of all the data structures in the collections module, including defaultdict.
Real Python’s guide to Python’s collections module – This guide provides a detailed overview of Python’s collections module, with plenty of examples and explanations.
defaultdict in Python by GeeksforGeeks – This article demonstrates the use of defaultdict in Python with examples.
Remember, mastering defaultdict and other data structures in Python’s collections module can significantly improve your coding skills and open up new possibilities for what you can achieve with Python.
Wrapping Up: Mastering Python defaultdict
In this comprehensive guide, we’ve explored the ins and outs of Python defaultdict, a container in Python’s collections module that provides default values for nonexistent keys.
We began with the basics, learning how to create a defaultdict and specify default values. We then delved into more advanced usage, such as using defaultdict with different data types and in nested data structures. Along the way, we tackled common issues you might encounter when using defaultdict, such as TypeErrors and KeyErrors, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to managing collections in Python, comparing defaultdict with other methods like the dict.get() method and try/except blocks. Here’s a quick comparison of these methods:
Method | Simplicity | Readability | Efficiency |
---|---|---|---|
defaultdict | High | High | High |
dict.get() | High | Moderate | Moderate |
try/except | Low | Low | High |
Whether you’re a beginner just starting out with Python defaultdict or an experienced Python developer looking to level up your collections management skills, we hope this guide has given you a deeper understanding of defaultdict and its capabilities.
With its balance of simplicity, readability, and efficiency, defaultdict is a powerful tool for managing collections in Python. Happy coding!