Python Hashmap Equivalent? Guide to Python Dictionaries
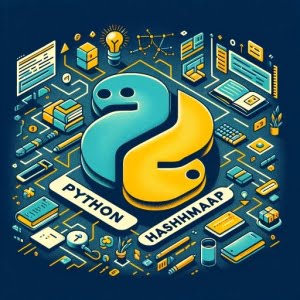
Stumped by hashmaps in Python? You’re not alone. Many developers find themselves puzzled when it comes to understanding hashmaps in Python. But, consider Python’s hashmap as a powerful toolbox – versatile and handy for various tasks.
Whether you’re storing data for quick retrieval, managing complex data structures, or even debugging, understanding how to use hashmaps in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of using hashmaps in Python, from the basics to more advanced techniques. We’ll cover everything from creating and using Python dictionaries, handling nested dictionaries, as well as alternative approaches.
Let’s dive in and start mastering Python hashmaps!
TL;DR: What is a Hashmap in Python?
In Python, a hashmap is implemented as a dictionary. It’s a mutable, iterable, and unordered collection of key-value pairs, which allows for quick data storage and retrieval.
Here’s a simple example:
my_dict = {'apple': 1, 'banana': 2}
print(my_dict['apple'])
# Output:
# 1
In this example, we’ve created a dictionary my_dict
with two key-value pairs. We then print the value associated with the key ‘apple’, which outputs ‘1’.
This is just the tip of the iceberg when it comes to Python hashmaps. Continue reading for a more in-depth exploration of Python dictionaries, including advanced usage scenarios and alternative approaches.
Table of Contents
- Diving into Python Hashmaps: Creating and Using Dictionaries
- Advanced Python Hashmaps: Dictionary Comprehensions and Nested Dictionaries
- Exploring Alternatives to Python Hashmaps
- Handling Common Issues with Python Hashmaps
- Python Data Types and Data Structures: The Building Blocks
- Hash Functions: The Heart of Python Hashmaps
- Python Hashmaps: The Gateway to Advanced Topics
- Wrapping Up: Mastering Python Hashmaps
Diving into Python Hashmaps: Creating and Using Dictionaries
Python hashmaps, also known as dictionaries, are one of the most flexible built-in data types in Python. They store data in the form of key-value pairs, which makes it easy to set, retrieve, and delete data.
Creating a Python Dictionary
Creating a dictionary in Python is quite straightforward. Here’s a simple example:
fruit_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
print(fruit_dict)
# Output:
# {'apple': 1, 'banana': 2, 'cherry': 3}
In this example, we’ve created a dictionary fruit_dict
with three key-value pairs. Each key represents a type of fruit, and each value represents a corresponding quantity.
Using a Python Dictionary
To retrieve a value from a dictionary, you can use the key associated with that value. Let’s try to fetch the quantity of ‘banana’ from our fruit_dict
:
print(fruit_dict['banana'])
# Output:
# 2
As you can see, by using the key ‘banana’, we quickly fetched the corresponding value ‘2’ from our dictionary.
Advantages and Potential Pitfalls
One of the main advantages of using dictionaries is their speed and efficiency in data retrieval. However, there are a few potential pitfalls to keep in mind. For instance, dictionaries are mutable, which means they can be changed after they are created. This can lead to unexpected behavior if not handled carefully. Additionally, dictionaries do not maintain any order of elements, which can be a problem if the order is important for your application.
Advanced Python Hashmaps: Dictionary Comprehensions and Nested Dictionaries
As you become more comfortable with Python hashmaps or dictionaries, you can start exploring more complex uses. Two such advanced techniques are dictionary comprehensions and handling nested dictionaries.
Python Dictionary Comprehensions
Dictionary comprehensions are a concise way to create dictionaries. They are similar to list comprehensions but with the additional requirement of defining a key:
squares = {x: x**2 for x in range(6)}
print(squares)
# Output:
# {0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
In this example, we create a dictionary squares
where each key-value pair represents a number (from 0 to 5) and its square. This is a much more efficient way of creating a dictionary when compared to adding each key-value pair individually.
Handling Nested Dictionaries
Nested dictionaries are dictionaries that contain other dictionaries. They can be used to store more complex data structures:
employees = {
'employee1': {'name': 'John', 'age': 30, 'job': 'Engineer'},
'employee2': {'name': 'Sarah', 'age': 35, 'job': 'Designer'}
}
print(employees['employee1'])
print(employees['employee1']['name'])
# Output:
# {'name': 'John', 'age': 30, 'job': 'Engineer'}
# John
In this example, we have a dictionary employees
where each key is an employee ID and each value is another dictionary containing details about the employee. We can retrieve the details of an employee by using their ID, and further access specific details by using the corresponding keys.
These advanced techniques can help you handle more complex data structures and write more efficient code when working with Python hashmaps.
Exploring Alternatives to Python Hashmaps
While Python dictionaries (hashmaps) are powerful and versatile, Python also offers other data structures that can be used depending on the specific needs of your application. Let’s delve into some of these alternatives, namely lists, tuples, and sets, and understand their usage and effectiveness.
Python Lists
Lists in Python are ordered collections of items. They are mutable, meaning their content can be changed after creation. Here’s a simple example of a list:
fruits = ['apple', 'banana', 'cherry']
print(fruits)
# Output:
# ['apple', 'banana', 'cherry']
In this example, we create a list fruits
with three items. Unlike a dictionary, a list maintains the order of the elements.
Python Tuples
Tuples in Python are similar to lists in that they can store multiple items. However, tuples are immutable, meaning their content cannot be changed after creation:
fruits_tuple = ('apple', 'banana', 'cherry')
print(fruits_tuple)
# Output:
# ('apple', 'banana', 'cherry')
In this example, we create a tuple fruits_tuple
. We cannot add or remove items from this tuple after it’s created.
Python Sets
Sets in Python are unordered collections of unique items. They are mutable, but you cannot have duplicate items in a set:
fruits_set = {'apple', 'banana', 'cherry', 'apple'}
print(fruits_set)
# Output:
# {'cherry', 'apple', 'banana'}
In this example, we create a set fruits_set
. Notice that the duplicate ‘apple’ is removed in the output.
Choosing the Right Data Structure
Each of these data structures has its advantages and disadvantages. Dictionaries are excellent for quick data retrieval and storage, but they do not maintain order. Lists and tuples maintain order, but data retrieval can be slower. Sets are useful when you need to store unique items, but they are unordered.
Choosing the right data structure depends on the specific requirements of your application. Understanding the differences can help you make an informed decision and write more efficient code.
Handling Common Issues with Python Hashmaps
While Python dictionaries are highly efficient, developers may occasionally encounter issues. Let’s discuss some common problems, including KeyError, and provide solutions and tips to handle them.
Dealing with KeyError
A KeyError occurs when you try to access a key that doesn’t exist in the dictionary. Here’s an example:
fruit_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
print(fruit_dict['mango'])
# Output:
# KeyError: 'mango'
In this example, we try to access the key ‘mango’ in fruit_dict
, but it doesn’t exist, resulting in a KeyError.
Solving KeyError
One way to prevent a KeyError is to use the dictionary’s get()
method. This method returns the value for a key if it exists, and a default value otherwise. Here’s how to use it:
print(fruit_dict.get('mango', 'Not found'))
# Output:
# Not found
In this example, because ‘mango’ doesn’t exist in fruit_dict
, the get()
method returns the default value ‘Not found’.
Other Considerations
When working with Python dictionaries, it’s also important to consider the mutable nature of dictionaries. Since dictionaries can be altered after creation, you need to be careful when copying dictionaries or using them as default function arguments.
Understanding these common issues and their solutions can help you avoid pitfalls and work more effectively with Python hashmaps.
Python Data Types and Data Structures: The Building Blocks
To fully grasp the concept of Python dictionaries (hashmaps), it’s essential to understand Python’s basic data types and data structures. Let’s take a step back and delve into these fundamental concepts.
Python Data Types
Python has several built-in data types, including integers, floats, strings, and booleans. Each of these data types has unique properties and behaviors. For instance, an integer is a whole number without a decimal point, while a float is a number that includes a decimal point.
Python Data Structures
Data structures in Python are collections of data that provide a way to organize and store data efficiently. These include lists, tuples, sets, and, of course, dictionaries. Each of these data structures has its own strengths and weaknesses, making them suitable for different tasks.
Hash Functions: The Heart of Python Hashmaps
A hash function is a function that takes input of a certain type and returns an integer. In the context of Python dictionaries, a hash function is used to calculate a unique hash value for each key. This hash value is then used to store and retrieve the key-value pair.
Here’s a simple demonstration of Python’s built-in hash function:
print(hash('apple'))
# Output:
# 5908993871830677928
In this example, we use the hash()
function to calculate the hash value for the string ‘apple’. The output is a unique integer which represents the hash value of ‘apple’.
Understanding the role of hash functions in Python dictionaries is crucial as it forms the basis of how data is stored and retrieved in a Python hashmap. It’s this mechanism that allows Python dictionaries to offer quick data retrieval regardless of the size of the dictionary.
Python Hashmaps: The Gateway to Advanced Topics
Python dictionaries, or hashmaps, are not just a standalone concept. They are a foundational tool that plays a critical role in several advanced areas, including data analysis, web development, and more. Understanding Python hashmaps can open doors to these broader topics.
Relevance of Dictionaries in Data Analysis
In data analysis, Python dictionaries can be used to store and manipulate data efficiently. For instance, they can be used to create frequency distributions, where the keys are unique items in a dataset and the values are counts of these items.
Python Dictionaries in Web Development
In web development, Python dictionaries are often used to handle data sent and received in HTTP requests. For example, when you send data to a server using a POST request, the data is often sent as a JSON object, which is similar to a Python dictionary.
Exploring JSON Handling in Python
JSON (JavaScript Object Notation) is a popular data format that is often used for transmitting data in web applications. Python has built-in support for JSON with the json
module. This module provides functions to convert between JSON and Python dictionaries, making it easy to work with JSON data in Python.
Understanding Data Manipulation
Data manipulation involves changing data to make it easier to read or analyze. Python dictionaries can be used for various data manipulation tasks, such as filtering data, transforming data, and aggregating data.
Further Resources for Python Hashmap Mastery
To deepen your understanding of Python hashmaps and related concepts, you can explore the following resources:
- Python Dictionary Manipulation – Tips and Tricks – Dive deep into Python dictionaries with this informative guide, packed with examples and tips.
Making Your Data Beautiful – Pretty Printing Python Dictionaries – Master the art of presenting dictionaries in a well-organized manner in Python.
Dealing with Missing Keys in Python – The DefaultDict Approach – Dive into Python defaultdict and understand its role in data processing.
Python.org’s Official documentation on dictionaries – This is the official guide from Python about dictionaries, providing comprehensive explanations and examples of their usage.
Guide on Python dictionaries – This guide from Real Python provides a deep dive into Python dictionaries, exploring the data type’s various operations, methods, and their uses.
Python for Data Analysis – This book by Wes McKinney, the creator of pandas, is a definitive guide to using Python for data analysis, including comprehensive sections about data structures like dictionaries.
These resources provide in-depth explanations and practical examples to help you master Python hashmaps and apply them in various contexts.
Wrapping Up: Mastering Python Hashmaps
In this comprehensive guide, we’ve explored the ins and outs of Python dictionaries, also known as hashmaps, from their basic usage to more advanced techniques. We’ve also delved into the underlying concepts, such as hash functions and Python’s data types and data structures, to provide a solid foundation for understanding Python hashmaps.
We began with the basics, learning how to create and use Python dictionaries. We then ventured into more advanced territory, exploring dictionary comprehensions and handling nested dictionaries. Along the way, we tackled common challenges, such as KeyError, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to data handling in Python, comparing dictionaries with other data structures like lists, tuples, and sets. Here’s a quick comparison of these data structures:
Data Structure | Speed | Order Preservation | Uniqueness of Elements |
---|---|---|---|
Dictionary (Hashmap) | Fast | No | Keys are unique |
List | Moderate | Yes | No |
Tuple | Moderate | Yes | No |
Set | Fast | No | Yes |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your data handling skills, we hope this guide has given you a deeper understanding of Python hashmaps and their capabilities. With their balance of speed and versatility, Python hashmaps are a powerful tool for data handling in Python. Happy coding!