Learn Python: Pretty Print a Dict with pprint()
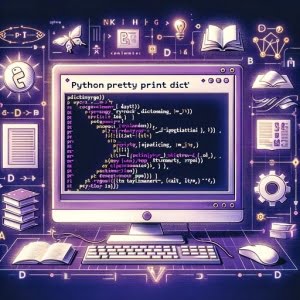
Ever found yourself in a maze of Python dictionaries, trying to decode the information? Consider Python’s pretty print function as your friendly librarian, ready to organize that information into a readable, structured format.
In this comprehensive guide, we’ll walk you through the process of pretty printing dictionaries in Python. We’ll start from the basics and gradually delve into more advanced techniques. So whether you’re a Python novice or a seasoned coder, there’s something for you to learn and apply.
TL;DR: How Do I Pretty Print a Dictionary in Python?
You can pretty print a dictionary in Python using the
pprint
module. Here’s a simple example:
import pprint
# Here's a simple dictionary
data = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
# Using pprint to pretty print the dictionary
pprint.pprint(data)
# Output:
{'key1': 'value1',
'key2': 'value2',
'key3': 'value3'}
In the above example, we first import the pprint
module. Then we define a simple dictionary called data
. Finally, we use the pprint
function from the pprint
module to print our data
dictionary in a pretty and readable format. The output is an organized and well-structured display of our dictionary.
Stick around for a more detailed exploration and advanced usage scenarios of pretty printing dictionaries in Python. Trust us, it’s worth your time!
Table of Contents
Pretty Print: A Beginner’s Guide
Python’s pprint
function is a handy tool for making your dictionaries more readable. But how does it work? Let’s explain it.
The pprint
function is part of the pprint
module. It takes a Python object (like our dictionary) as an argument and produces a string as output. It organizes the data in a way that’s easier to read and understand, especially when dealing with complex or nested dictionaries.
Let’s take a look at a simple example:
import pprint
# Our dictionary
data = {'apple': 'fruit', 'carrot': 'vegetable', 'cake': 'dessert'}
# Standard print
print('Standard Print:', data)
# Pretty Print
pprint.pprint('Pretty Print:', data)
# Output:
# Standard Print: {'apple': 'fruit', 'carrot': 'vegetable', 'cake': 'dessert'}
# Pretty Print: {'apple': 'fruit',
# 'carrot': 'vegetable',
# 'cake': 'dessert'}
In the above code, we first print out our dictionary using the standard print
function. The output is a single line of text, which can be hard to read if our dictionary gets larger. Then we use the pprint
function and the output is much clearer. Each key-value pair is on a separate line, making it easier to understand the structure of our dictionary.
The pprint
function is particularly useful when dealing with larger, more complex dictionaries. It’s like having your own personal librarian, organizing your data into a format that’s easy to read and understand.
Like any tool,
pprint
has its limitations. For instance, it may not handle non-string keys or non-serializable values as expected. We’ll delve into these potential pitfalls in our troubleshooting section.
Taming Complex Dictionaries with Pretty Print
As you delve deeper into Python, you’ll often encounter more complex dictionaries. These might include nested dictionaries or dictionaries with long strings. The pprint
function remains a reliable ally in making sense of these beasts. Let’s explore how.
Dealing with Nested Dictionaries
Nested dictionaries are dictionaries that contain other dictionaries as values. Here’s how pprint
can help make them more readable:
import pprint
# A nested dictionary
nested_dict = {
'fruit': {'apple': 'red', 'banana': 'yellow'},
'vegetable': {'carrot': 'orange', 'pea': 'green'},
'dessert': {'cake': 'delicious', 'broccoli': 'not a dessert'}
}
pprint.pprint(nested_dict)
# Output:
# {'dessert': {'broccoli': 'not a dessert', 'cake': 'delicious'},
# 'fruit': {'apple': 'red', 'banana': 'yellow'},
# 'vegetable': {'carrot': 'orange', 'pea': 'green'}}
In the above example, pprint
neatly organizes our nested dictionary, making it easier to understand the hierarchy and relationships within the data.
Handling Long Strings
Dictionaries with long strings can also benefit from pretty printing. pprint
automatically wraps long lines to make them more readable:
import pprint
# A dictionary with long strings
long_string_dict = {
'quote': 'In the face of ambiguity, refuse the temptation to guess.',
'author': 'The Zen of Python, by Tim Peters'
}
pprint.pprint(long_string_dict, width=40)
# Output:
# {'author': 'The Zen of Python, by Tim Peters',
# 'quote': 'In the face of ambiguity, refuse the temptation to guess.'}
In this example, we’ve added an additional argument, width
, to the pprint
function. This tells pprint
the maximum width of a line before it should wrap the text to a new line. As a result, our long strings are now much easier to read.
By mastering these advanced techniques, you’ll be better equipped to handle complex dictionaries in Python. Remember, pretty printing is not just about making your code look good – it’s about making it easier to understand and debug.
Alternative Pretty Printing Methods
While Python’s pprint
module is a powerful tool for pretty printing dictionaries, it’s not the only game in town. There are alternative methods that you might find useful, depending on your specific needs.
Let’s explore two of these alternatives: the json
module and third-party libraries like PyYAML
.
Pretty Printing with JSON
Python’s json
module offers a method called dumps()
that can pretty print dictionaries. It’s particularly useful when working with JSON data, as it can serialize Python objects into JSON formatted strings.
Here’s an example:
import json
# Our dictionary
data = {'apple': 'fruit', 'carrot': 'vegetable', 'cake': 'dessert'}
# Pretty print with json
print(json.dumps(data, indent=4))
# Output:
# {
# "apple": "fruit",
# "carrot": "vegetable",
# "cake": "dessert"
# }
In the above code, json.dumps()
takes two arguments: the object to be serialized and the indent
parameter. The indent
parameter specifies how many spaces to use as indentation, which makes the output more readable.
Pretty Printing with PyYAML
PyYAML
is a Python library that allows you to parse and generate YAML, a human-friendly data serialization standard. It can also pretty print dictionaries in a YAML-like format.
Here’s how to do it:
import yaml
# Our dictionary
data = {'apple': 'fruit', 'carrot': 'vegetable', 'cake': 'dessert'}
# Pretty print with PyYAML
print(yaml.dump(data, default_flow_style=False))
# Output:
# apple: fruit
carrot: vegetable
cake: dessert
In this example, yaml.dump()
takes two arguments: the object to be serialized and the default_flow_style
parameter. Setting default_flow_style
to False
ensures that our dictionary is printed in block style, which is more readable.
Both of these methods have their advantages. The
json
module is built into Python and is great for working with JSON data.PyYAML
, on the other hand, requires an additional installation but provides a more human-friendly output. Your choice will depend on your specific needs and the nature of your data.
Like any tool, Python’s pretty print function is not without its quirks. You may encounter issues when dealing with non-string keys or non-serializable values in your dictionaries.
But don’t fret – we’ve got some solutions and workarounds to help you navigate these potential pitfalls.
Handling Non-String Keys
Python dictionaries can have keys of any immutable type. But pretty printing dictionaries with non-string keys can lead to unexpected results. Here’s an example:
import pprint
# Dictionary with a non-string key
data = {1: 'one', 2: 'two', 3: 'three'}
pprint.pprint(data)
# Output:
# {1: 'one', 2: 'two', 3: 'three'}
In the above example, our dictionary keys are integers. The pprint
function prints the dictionary as expected, but it doesn’t quote the keys as it would with string keys. This might be confusing if you’re expecting all keys to be quoted.
Dealing with Non-Serializable Values
The pprint
function might also struggle with non-serializable values, like custom objects. Here’s an example:
import pprint
# A custom object
class MyClass:
pass
# Dictionary with a non-serializable value
data = {'my_class': MyClass()}
pprint.pprint(data)
# Output:
# {'my_class': <__main__.MyClass object at 0x7f9e1f4a3a90>}
In the above example, our dictionary contains a custom object as a value. The pprint
function can’t serialize this object into a string, so it prints the object’s memory address instead. This might not be helpful if you’re trying to understand the contents of the object.
In both of these cases, a possible workaround is to convert your keys or values to strings before pretty printing. This will ensure that they’re serialized correctly. But remember, this might not always be the best solution, especially if you need to preserve the original data types in your dictionary.
Understanding Python Dictionaries
Before we delve deeper into the art of pretty printing, let’s take a step back and understand the fundamentals. At the heart of this technique is Python’s dictionary data type.
Python Dictionaries: The Basics
A Python dictionary is a built-in data type that stores data in key-value pairs. It’s an unordered collection which means the data stored in a dictionary doesn’t have a specific order. Here’s a simple example:
# A simple dictionary
my_dict = {'name': 'John', 'age': 30, 'city': 'New York'}
# Print the dictionary
print(my_dict)
# Output:
# {'name': 'John', 'age': 30, 'city': 'New York'}
In this example, ‘name’, ‘age’, and ‘city’ are keys, and ‘John’, 30, and ‘New York’ are their respective values. Dictionaries are versatile and can store a wide range of data types as keys and values, making them a powerful tool in Python.
The Bigger Picture: Pretty Print in Real-World Applications
Now that you’ve mastered the art of pretty printing dictionaries in Python, let’s explore why this skill is so valuable in real-world applications.
Data Analysis and Debugging
In data analysis, you often work with large, complex datasets. Pretty printing can help you understand the structure of your data, making it easier to clean, analyze, and visualize. Similarly, in debugging, a well-structured display of your data can make it easier to spot errors or inconsistencies.
Logging
Logging is another area where pretty printing shines. When logging data, readability is key. Pretty printing can help you structure your log data in a way that’s easy to read and understand, saving you time and effort in the long run.
Expanding Your Horizons
Pretty printing dictionaries is just the tip of the iceberg. There’s a whole world of related concepts to explore. For instance, you might be interested in learning how to handle JSON data in Python, or how to format output for better readability. Each of these skills will make you a better Python programmer and open up new possibilities for your projects.
To deepen your understanding, we recommend exploring the following resources:
- Python Dictionary Guide: Unlocking Its Full Potential – Discover the hidden potential of Python dictionaries and their role in data-driven applications.
Using Ordered Dictionaries in Python: Order Matters – Master the art of working with OrderedDicts in Python.
Python Hashmap: Understanding the Basics of Hash Tables – Explore the concept of a hashmap in Python and its applications.
Article on Python Dictionary Methods – This Dev Genius article highlights 10 important Python dictionary methods and provides a detailed walkthrough on their usage.
Guide on Python Dictionary Methods – This guide discusses various dictionary methods in Python with examples to explain their particular usage and benefits.
Dictionary Methods in Python on Plain English – This article explains 12 dictionary methods in Python that aid in accessing and manipulating data with Python dictionaries.
The Python community is vast and active, and there’s a wealth of resources available to help you on your journey. Remember, the more you learn, the more powerful your Python skills will become.
Wrapping Up:
In our journey through the art of pretty printing dictionaries in Python, we’ve covered a lot of ground. We started with the basics, learning how to use Python’s pprint
function to make our dictionaries more readable.
We then delved into more complex scenarios, exploring how pprint
can handle nested dictionaries and long strings. We also learned about potential issues when dealing with non-string keys or non-serializable values, and how to troubleshoot these issues.
Beyond pprint
, we discovered alternative methods for pretty printing dictionaries, such as the json
module and the PyYAML
library. Each of these methods has its own strengths and can be a powerful tool in your Python toolkit.
Finally, we looked at the bigger picture, discussing the real-world applications of pretty printing and how it can benefit you in areas like data analysis, debugging, and logging. We also encouraged you to continue learning and exploring related concepts.
In summary, pretty printing dictionaries in Python is a valuable skill that can make your code more readable and your life as a Python programmer easier. Whether you’re using pprint
, json
, or PyYAML
, the key is to find the method that works best for you and your data. Happy coding!