Python Zip Function | Guide (With Examples)
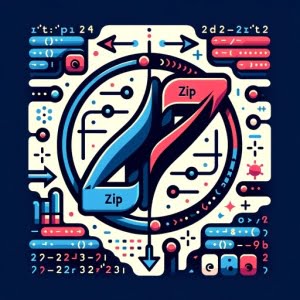
Ever wondered how to pair elements from different lists in Python? Like a perfect matchmaker, Python’s zip function can pair elements from different iterables.
This article will guide you through the ins and outs of the Python zip function, from basic usage to advanced techniques. Whether you’re a beginner just starting out with Python or an experienced developer looking to refine your skills, this guide is designed to help you master the Python zip function.
So, let’s dive in and explore the power of Python’s zip function together!
TL;DR: How Do I Use the Zip Function in Python?
The zip function in Python takes in iterables as arguments and returns an iterator that generates tuples. Here’s a simple example:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
zipped = zip(list1, list2)
print(list(zipped))
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
In this example, we have two lists: list1
with numbers and list2
with letters. We use the zip
function to pair each element from list1
with the corresponding element from list2
.
The result is a list of tuples, where each tuple contains a pair of corresponding elements from the two lists.
Stay tuned for more detailed explanations and advanced usage scenarios of the Python zip function!
Table of Contents
- Understanding the Basics of Python’s Zip Function
- Advanced Usage of Python’s Zip Function
- Exploring Alternative Approaches to Pairing Iterables
- Troubleshooting Common Issues with Python’s Zip Function
- Python Iterables and the Concept of Zipping
- The Relevance of Python’s Zip Function
- Further Resources for Zip and Other Functions
- Wrapping Up: Mastering the Python Zip Function
Understanding the Basics of Python’s Zip Function
The zip function in Python is a built-in function that allows us to combine corresponding elements from multiple iterable objects (like lists, tuples, or sets) into a single iterable. This function can take in any number of iterables and returns an iterator that generates tuples. Each tuple contains the i-th element from each of the argument sequences or iterables.
Let’s take a look at a basic example of how the zip function works:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
zipped = zip(list1, list2)
print(list(zipped))
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
In this example, list1
and list2
are our input iterables. The zip
function pairs the corresponding elements from these lists and returns an iterator of tuples. When we convert this iterator to a list and print it, we see a list of tuples where each tuple contains a pair of corresponding elements from list1
and list2
.
Advantages and Potential Pitfalls
The zip function is incredibly useful when you need to pair up elements from multiple lists. It’s a clean, efficient way to create pairs (or larger tuples) without needing to write a loop.
However, there are a few things to keep in mind when using the zip function. One of the main things to remember is that the zip function stops creating tuples when the shortest input iterable is exhausted. This means that if your input iterables are not the same length, the ‘extra’ elements from the longer iterables will not be included in the output.
We’ll explore this and other advanced scenarios in the next section, so stay tuned!
Advanced Usage of Python’s Zip Function
The Python zip function is versatile and can handle different types of iterables and uneven length iterables.
Dealing with Different Types of Iterables
First, let’s discuss how the zip function works with different types of iterables. The zip function is not limited to lists; it can handle any iterable, including tuples, sets, and even strings.
Let’s see an example:
list1 = [1, 2, 3]
tuple1 = ('a', 'b', 'c')
set1 = {'x', 'y', 'z'}
zipped = zip(list1, tuple1, set1)
print(list(zipped))
# Output:
# [(1, 'a', 'x'), (2, 'b', 'y'), (3, 'c', 'z')]
In this example, we have a list, a tuple, and a set. The zip function successfully pairs the corresponding elements from each iterable, returning an iterator of tuples.
Handling Uneven Length Iterables
When dealing with uneven length iterables, the zip function stops creating tuples when the shortest iterable is exhausted. This means that ‘extra’ elements from the longer iterables will not be included in the output.
Here’s an example:
list1 = [1, 2, 3, 4]
list2 = ['a', 'b', 'c']
zipped = zip(list1, list2)
print(list(zipped))
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
In this case, the number ‘4’ from list1
does not appear in the output because list2
does not have a corresponding element.
Best Practices
When using the zip function, it’s best to ensure your input iterables are of the same length to avoid missing out on any elements. If your iterables might be of different lengths and you want to include ‘extra’ elements, consider using the zip_longest
function from the itertools
module. We’ll explore this and other alternatives in the next section.
Exploring Alternative Approaches to Pairing Iterables
While the Python zip function is a powerful tool for pairing elements from iterables, it’s not the only method available. Let’s explore some alternative approaches, such as list comprehension and using the itertools module.
Pairing Iterables with List Comprehension
List comprehension is a Pythonic way to create lists based on existing lists (or other iterables). We can use it to pair elements from two lists in a similar way to the zip function.
Here’s an example:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
pairs = [(x, y) for x, y in zip(list1, list2)]
print(pairs)
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
In this code, we use list comprehension to create a list of tuples. Each tuple contains a pair of corresponding elements from list1
and list2
. The result is the same as using the zip function directly, but with an added layer of flexibility. With list comprehension, we can add conditions to filter the output or modify the elements before pairing them.
Pairing Iterables with itertools.zip_longest
The itertools
module provides a function called zip_longest
that works similarly to the zip function but with a key difference: it doesn’t stop at the shortest iterable. Instead, it fills in ‘missing’ values with a specified fillvalue.
Here’s how it works:
from itertools import zip_longest
list1 = [1, 2, 3, 4]
list2 = ['a', 'b', 'c']
zipped = zip_longest(list1, list2, fillvalue='N/A')
print(list(zipped))
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c'), (4, 'N/A')]
In this example, list1
is longer than list2
. The zip_longest
function pairs the elements as usual, but when it reaches the end of list2
, it continues to pair elements from list1
with the fillvalue
‘N/A’.
Comparison Table
Method | Advantages | Disadvantages |
---|---|---|
zip | Simple, built-in function | Stops at shortest iterable |
List comprehension with zip | Flexible, can add conditions or modify elements | More complex syntax |
itertools.zip_longest | Pairs all elements, doesn’t stop at shortest iterable | Requires importing itertools module |
In conclusion, while the Python zip function is a great tool for pairing elements from iterables, you might find list comprehension or itertools.zip_longest
more suitable for your needs depending on your specific use case.
Troubleshooting Common Issues with Python’s Zip Function
While Python’s zip function is a powerful tool, like any function, it may not always behave as expected. Let’s discuss some common issues you may encounter when using it, along with solutions and workarounds.
Dealing with Empty Iterables
One common issue is dealing with empty iterables. If one or more of your input iterables is empty, the zip function will return an empty iterator. Here’s an example:
list1 = [1, 2, 3]
list2 = []
zipped = zip(list1, list2)
print(list(zipped))
# Output:
# []
In this case, because list2
is empty, the zip
function returns an empty list. If you want to pair elements from list1
with a default value when list2
is empty, you can use the itertools.zip_longest
function, as discussed in the previous section.
Memory Issues
Another issue to consider is memory. The zip function returns an iterator, which is a lazy object that generates values on the fly. This is memory-efficient for large iterables, as it doesn’t require storing all the pairs in memory at once. However, if you convert the iterator to a list or another iterable, it will consume more memory. If you’re dealing with very large iterables and experiencing memory issues, consider working with the iterator directly, or using a tool like itertools.islice
to handle chunks of the iterator at a time.
Remember, Python’s zip function is a versatile tool, but it’s not the only one in your toolbox. Depending on your specific needs and the issues you’re facing, other functions or modules may be more appropriate. Always consider the characteristics of your data and the requirements of your project when choosing your tools.
Python Iterables and the Concept of Zipping
To fully grasp the Python zip function, it’s important to understand two fundamental concepts: Python’s iterable objects and the concept of zipping in programming.
Python’s Iterable Objects
In Python, an iterable is any object capable of returning its elements one at a time. Lists, tuples, strings, dictionaries, sets, and even files are all examples of iterable objects in Python.
Here’s an example of iterating over a list:
list1 = [1, 2, 3]
for i in list1:
print(i)
# Output:
# 1
# 2
# 3
In this code, we use a for loop to iterate over list1
. The loop runs once for each element in the list, and each time it runs, the variable i
is set to the next element in the list.
The Concept of Zipping in Programming
Zipping is a concept in programming where you ‘zip’ together two or more sequences by pairing up their corresponding elements. It’s like zipping up a zipper: you’re bringing together two separate things into one interconnected whole.
In Python, we achieve this using the built-in zip function. As we’ve seen in previous sections, the zip function takes in any number of iterables and returns an iterator that generates tuples. Each tuple contains the i-th element from each of the argument sequences or iterables.
By understanding Python’s iterable objects and the concept of zipping, we can better understand and use the Python zip function. With these fundamentals in mind, we’re ready to delve deeper into the zip function and explore its practical applications.
The Relevance of Python’s Zip Function
Python’s zip function is more than just a tool for pairing elements from different lists. It plays a crucial role in data manipulation and handling multiple sequences, making it a key part of any Python programmer’s toolkit.
Zip Function in Data Manipulation
In data analysis and manipulation, we often deal with structured data where each row represents an observation and each column represents a variable. Let’s say we have data on students’ names and their corresponding grades. We can store this data in two lists and use the zip function to pair each student with their grade:
students = ['Alice', 'Bob', 'Charlie']
grades = [85, 90, 95]
student_grades = dict(zip(students, grades))
print(student_grades)
# Output:
# {'Alice': 85, 'Bob': 90, 'Charlie': 95}
In this example, we use the zip function to pair each student’s name with their grade, then convert the resulting iterator of tuples into a dictionary.
Handling Multiple Sequences
When dealing with multiple sequences, the zip function shines. It allows us to handle multiple sequences concurrently, pairing up corresponding elements without the need for nested loops.
Expanding Your Knowledge
While the zip function is a powerful tool, it’s just one of many in Python’s arsenal. Other concepts like list comprehension and generator expressions offer different ways to manipulate and work with data.
Further Resources for Zip and Other Functions
We encourage you to explore the following concepts further to deepen your understanding and expand your skillset:
- Python Built-In Functions: A Quick Reference – Explore the essential built-in functions that Python offers for everyday tasks.
Python type() Function: Data Type Identification: Discover Python’s “type” function for determining the type of an object.
Python eval() Function: Dynamic Code Execution – Discover Python’s “eval” function for dynamic code execution.
Understanding Python Zip Function – An in-depth look at Python’s built-in zip function and how it can be used.
Python Library Functions Documentation – Official Python documentation on all of Python’s built-in functions.
Exploring Python Zip Function – Get hands-on with Python’s zip function with examples and practice exercises from W3Schools.
Remember, the best way to learn is by doing. So, don’t hesitate to experiment with these concepts and apply them in your projects.
Wrapping Up: Mastering the Python Zip Function
To recap, the zip
function in Python is an invaluable tool for pairing elements from multiple iterables, such as lists or tuples. We’ve explored its basic usage, where it pairs corresponding elements from the given iterables and returns an iterator that generates tuples.
We’ve also delved into some of the common issues you might encounter when using the zip
function, such as dealing with empty or uneven length iterables, and provided solutions and workarounds for these scenarios.
Additionally, we’ve looked at alternative approaches to pairing elements from iterables. List comprehension offers a flexible way to create lists based on existing lists, while the itertools.zip_longest
function from the itertools module can handle uneven length iterables without leaving out ‘extra’ elements.
Here’s a brief comparison of the methods discussed:
Method | Advantages | Disadvantages |
---|---|---|
zip | Simple, built-in function | Stops at shortest iterable |
List comprehension with zip | Flexible, can add conditions or modify elements | More complex syntax |
itertools.zip_longest | Pairs all elements, doesn’t stop at shortest iterable | Requires importing itertools module |
Ultimately, the best method depends on your specific needs and the nature of your data. The zip
function is a powerful tool, but it’s important to remember that Python offers a variety of tools and techniques for handling data. Don’t hesitate to explore and experiment with these to find the ones that work best for you.