Python Eval Function: Complete Guide
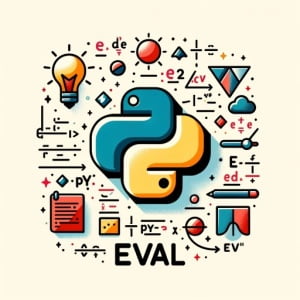
Ever found yourself puzzled by Python’s eval function? You’re not alone. Many developers find this function a bit tricky to comprehend. Think of Python’s eval function as a powerful calculator – it can evaluate expressions dynamically, making it a versatile tool in your Python toolkit.
Whether you’re looking to evaluate simple mathematical expressions or complex code snippets, understanding how to use Python’s eval function can significantly enhance your coding efficiency.
In this guide, we’ll explore the intricacies of Python’s eval function, from its basic usage to more advanced applications. We’ll also delve into potential pitfalls and discuss alternative approaches.
Let’s get started!
TL;DR: What is Python’s Eval Function?
Python’s
eval()
function is a powerful tool that evaluates expressions written as strings. It’s like a dynamic calculator within Python, capable of evaluating both simple and complex expressions.
Here’s a simple example:
x = 1
print(eval('x + 1'))
# Output:
# 2
In this example, we have a variable x
set to 1. We then use the eval function to evaluate the expression ‘x + 1’ which is written as a string. The eval function evaluates the string as a Python expression, adding 1 to the value of x
, and thus, the output is 2.
This is just a basic use of Python’s eval function. There’s much more to learn about this versatile function, including its advanced uses, potential pitfalls, and alternatives. So, stick around for a deeper dive!
Table of Contents
- Getting Started with Python’s Eval Function
- Leveraging Eval for Complex Expressions
- Exploring Alternatives to Python’s Eval Function
- Navigating Common Issues with Python’s Eval Function
- Python’s Dynamic Typing and String Manipulation
- Python’s Eval Function in Real-world Applications
- Wrapping Up: Mastering Python’s Eval Function
Getting Started with Python’s Eval Function
Python’s eval function is a built-in function that parses the expression passed to it and executes Python expression(s) within it. Let’s start with a basic example:
expression = '4 * (6 + 2) / 2'
result = eval(expression)
print(result)
# Output:
# 16.0
The expression ‘4 * (6 + 2) / 2’ is a string that gets evaluated by the eval function. It follows the usual mathematical rules of precedence, so the addition is performed first, followed by multiplication and then division, yielding the result 16.0.
The Power and Pitfalls of Eval
The eval function is incredibly powerful because it can dynamically evaluate Python expressions from strings. This can be handy in many situations, like creating a simple calculator app or parsing a user’s input.
However, with great power comes great responsibility. The eval function can pose security risks if not used carefully. Since it evaluates any Python expression, a malicious user could potentially pass code that can harm your system. Therefore, it’s crucial to use eval judiciously and only with trusted input.
In the next section, we’ll dive into more advanced uses of Python’s eval function, exploring its flexibility and potential in more complex scenarios.
Leveraging Eval for Complex Expressions
Python’s eval function isn’t limited to simple mathematical expressions. It can evaluate more complex expressions, and even function calls. Let’s take a look at an example:
expression = 'pow(2, 3)'
result = eval(expression)
print(result)
# Output:
# 8
In this example, we’re using the eval function to evaluate the string ‘pow(2, 3)’, which is a call to Python’s built-in pow
function with arguments 2 and 3. The eval function evaluates this string as a Python expression, effectively executing the pow
function and printing the result 8.
Eval with Different Scopes
The eval function can also be used with different scopes. It takes two optional dictionaries as arguments, the global and local symbol table. If provided, the expression is evaluated with those scopes. If not provided, the expression is evaluated in the current scope.
global_symbols = {'x': 2, 'y': 3}
local_symbols = {'y': 5, 'z': 8}
expression = 'x * y + z'
result = eval(expression, global_symbols, local_symbols)
print(result)
# Output:
# 18
In this example, the expression ‘x * y + z’ is evaluated with x
and y
from the global symbol table and z
from the local symbol table. Thus, the result is 18.
Handling Exceptions with Eval
Like any other Python function, the eval function can raise exceptions. For instance, if you try to evaluate a malformed expression, a SyntaxError
is raised. It’s good practice to handle such exceptions when using eval.
expression = '1 / 0'
try:
result = eval(expression)
except ZeroDivisionError:
print('Error: Division by zero is not allowed.')
# Output:
# Error: Division by zero is not allowed.
In this example, we’re trying to evaluate the expression ‘1 / 0’ which will cause a ZeroDivisionError
. By using a try-except block, we can catch this error and print a user-friendly error message instead of letting the program crash.
Remember, while Python’s eval function is a powerful tool, it’s not always the best solution. In the next section, we’ll explore some alternative approaches to eval.
Exploring Alternatives to Python’s Eval Function
While Python’s eval function is powerful and flexible, it’s not always the best choice, especially considering security and performance concerns. Let’s take a look at some alternatives and when you might want to use them.
Using literal_eval for Safe Evaluation
Python’s ast
module provides a function called literal_eval
that can evaluate a string containing a Python literal or container display. The key difference between eval
and literal_eval
is that literal_eval
is safe and can only evaluate Python literals.
import ast
expression = '[1, 2, 3]'
result = ast.literal_eval(expression)
print(result)
# Output:
# [1, 2, 3]
In this example, literal_eval
is used to evaluate a string containing a list display. Unlike eval
, literal_eval
would raise an exception if the string contained anything other than a Python literal.
Parsing Expressions with ast.parse
For more complex needs, you can use ast.parse
to parse a string into an abstract syntax tree (AST). You can then manipulate this AST and compile it into a code object, which can be executed using the exec
function.
import ast
expression = 'x = 2'
ast_node = ast.parse(expression)
exec(compile(ast_node, '', 'exec'))
print(x)
# Output:
# 2
In this example, ast.parse
is used to parse the expression ‘x = 2’ into an AST. This AST is then compiled into a code object, which is executed using exec
, setting the value of x
to 2.
Executing Code with exec
The exec
function is another built-in function that can execute Python code dynamically. Unlike eval
, exec
can execute statements as well as expressions, but it doesn’t return a value.
expression = 'x = 2'
exec(expression)
print(x)
# Output:
# 2
In this example, exec
is used to execute the statement ‘x = 2’, setting the value of x
to 2.
Remember, while these alternatives can be safer or more suitable than eval
in certain situations, they each have their own trade-offs. Always consider your specific needs and constraints when choosing how to evaluate or execute Python code dynamically.
While Python’s eval function is a powerful tool, it comes with its own set of challenges. Here, we’ll discuss some common issues you might encounter when using eval, along with their solutions.
Security Risks with Eval
One of the most significant risks of using eval is that it can evaluate any Python expression, potentially leading to code injection attacks. For example:
expression = 'os.system("rm -rf /")'
# eval(expression)
# Output:
# This would delete all files in your root directory!
In this example, if the eval function were to evaluate the expression, it would execute a command to delete all files in your root directory! Therefore, never use eval with untrusted input. If you need to evaluate expressions from an untrusted source, consider using ast.literal_eval
or parsing the expressions manually.
Performance Issues with Eval
The eval function can be slower than other methods of execution because it involves parsing and interpreting the expression. If performance is a concern, consider using compiled code or built-in functions instead.
Handling SyntaxErrors
If eval encounters a malformed expression, it raises a SyntaxError
. You can handle this with a try-except block.
expression = '1 /'
try:
result = eval(expression)
except SyntaxError:
print('Error: Malformed expression.')
# Output:
# Error: Malformed expression.
In this example, the eval function tries to evaluate the expression ‘1 /’, which is malformed. A SyntaxError
is raised, which we catch and print a user-friendly error message.
Remember, while Python’s eval function is incredibly powerful, it’s important to use it responsibly. Always consider potential issues and alternatives, and strive to write secure and efficient code.
Python’s Dynamic Typing and String Manipulation
Understanding Python’s dynamic typing and string manipulation fundamentals can provide valuable insight into how the eval function operates.
Dynamic Typing in Python
Python is a dynamically typed language, which means that the type of a variable is checked during runtime and not in advance. This flexibility allows Python to evaluate expressions from strings dynamically, which is the core functionality of the eval function.
Here’s a simple example:
x = 1
x = 'Hello'
print(x)
# Output:
# 'Hello'
In this example, the variable x
is initially an integer. Later, it’s reassigned to a string. Python’s dynamic typing allows this reassignment without any type errors.
String Manipulation in Python
Python provides robust features for string manipulation, which is crucial for the eval function. You can create, modify, and evaluate strings with Python’s built-in functions and methods.
expression = '2 * 3'
modified_expression = expression.replace('2', '4')
result = eval(modified_expression)
print(result)
# Output:
# 12
In this example, we initially have the string ‘2 * 3’. We then replace ‘2’ with ‘4’ using Python’s replace method. The modified string ‘4 * 3’ is then evaluated using the eval function, yielding the result 12.
By understanding these fundamentals, you can better appreciate the power and flexibility of Python’s eval function. In the following sections, we’ll explore how eval can be used in different Python applications and direct you to more resources for deepening your understanding.
Python’s Eval Function in Real-world Applications
Python’s eval function has a wide range of applications, from scripting to testing and debugging. Its ability to evaluate expressions dynamically makes it a powerful tool in various Python applications.
Scripting with Eval
In scripting, eval can be used to evaluate expressions dynamically, which can be especially useful when the expressions are not known until runtime.
# A simple script that asks for an expression and evaluates it
expression = input('Enter an expression: ')
result = eval(expression)
print(f'The result is {result}')
# Output (example):
# Enter an expression: 2 * 3
# The result is 6
In this example, the script asks the user for an expression, evaluates it using eval, and prints the result.
Testing and Debugging with Eval
Eval can also be used in testing and debugging to evaluate test expressions or debug code dynamically.
# A simple test function that uses eval to check the result of an expression
def test_expression(expression, expected_result):
result = eval(expression)
assert result == expected_result, f'Error: {expression} = {result}, expected {expected_result}'
# Testing the function
test_expression('2 * 3', 6)
# Output:
# No output if the test passes, an AssertionError if it fails
In this example, the test function uses eval to evaluate the test expression and checks if the result matches the expected result.
Exploring Related Concepts
If you’re interested in Python’s eval function, you might also want to explore related concepts like Python’s exec function and the AST (Abstract Syntax Tree) module. The exec function is similar to eval but can execute Python code dynamically. The AST module allows you to parse Python code into an abstract syntax tree, which can be analyzed or modified.
Further Resources for Mastering Python’s Eval Function
To deepen your understanding of Python’s eval function and related concepts, consider exploring the following resources:
- IOFlood’s Python Built-In Functions Article – Learn how built-in functions can enhance your code readability and efficiency.
Simplifying Iteration with Python’s zip() Function – Dive into zipping lists, tuples, and more with Python’s versatile “zip.”
Simplifying Range Generation with xrange() in Python – Learn about the benefits of “xrange” over “range” in Python 2.
Python’s Official Documentation on Eval – Official Python documentation for insights on the built-in eval function.
Python’s Official Documentation on the AST Module – Get to know about Python’s abstract syntax trees (AST) module.
Real Python’s Comprehensive Eval Function Tutorial can help you gain extensive knowledge on Python’s eval function.
Wrapping Up: Mastering Python’s Eval Function
In this comprehensive guide, we’ve explored the ins and outs of Python’s eval function. We’ve delved into its usage, from basic mathematical expressions to complex code evaluations, and discussed its potential pitfalls, such as security risks and performance issues.
We’ve seen how to use the eval function in different scenarios, from simple calculations to advanced expressions involving function calls and different scopes. We’ve also tackled common challenges you might encounter when using eval, such as handling exceptions and dealing with SyntaxErrors.
We ventured into alternative approaches to eval, such as using ast.literal_eval
, ast.parse
, and exec
. These alternatives can provide safer or more suitable solutions in certain situations, but like eval, they each come with their own trade-offs.
Here’s a quick comparison of these methods:
Method | Flexibility | Security | Performance |
---|---|---|---|
eval | High | Low | Moderate |
ast.literal_eval | Low | High | High |
ast.parse + exec | High | Moderate | High |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python’s eval function and its alternatives.
With its ability to evaluate expressions dynamically, Python’s eval function is a powerful tool in your Python toolkit. Happy coding!