Mastering If Statements in Java: Basics and Beyond
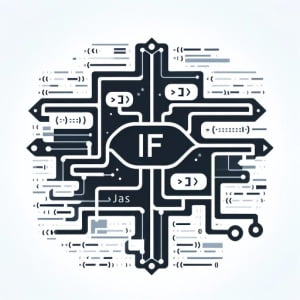
Are you finding it challenging to get your head around if statements in Java? You’re not alone. Many developers, especially beginners, often find themselves puzzled when it comes to handling if statements in Java. Think of an if statement as a traffic cop, directing the flow of your program based on certain conditions. It’s a fundamental concept that forms the backbone of many programming tasks.
This guide will walk you through the ins and outs of using if statements in Java, from the basics to more advanced techniques. We’ll cover everything from the syntax of if statements, how to use them in different scenarios, to common pitfalls and how to avoid them.
So, let’s dive in and start mastering if statements in Java!
TL;DR: How Do I Use an If Statement in Java?
In Java, an “if statement” is used to test a condition. The syntax is
if (condition) {code to execute}
. If the condition is true, the code within the curly braces{}
is executed.
Here’s a simple example:
int x = 10;
int y = 5;
if (x > y) {
System.out.println("x is greater than y");
}
// Output:
// 'x is greater than y'
In this example, we’ve used an if statement to compare two variables, x
and y
. The condition x > y
is true, so the code within the if statement is executed, and ‘x is greater than y’ is printed to the console.
This is a basic way to use if statements in Java, but there’s much more to learn about controlling the flow of your program. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Use of If Statements in Java
The if statement in Java is a fundamental control flow statement, which allows the program to make decisions based on certain conditions. It follows a simple syntax:
if (condition) {
// code to be executed if the condition is true
}
The condition
in an if statement is a boolean expression, which means it can either be true
or false
. If the condition is true, the code block inside the if statement gets executed. If it’s false, the code block is skipped.
Here’s a simple code example:
int age = 20;
if (age >= 18) {
System.out.println("You are eligible to vote.");
}
// Output:
// 'You are eligible to vote.'
In this example, the condition age >= 18
is true, so the string ‘You are eligible to vote.’ is printed to the console.
While if statements are incredibly useful, they do come with some potential pitfalls. For example, if you forget to use braces {}
, only the first line of code after the if statement will be considered part of the if block. This can lead to unexpected results. Always remember to enclose the code you want to execute within braces.
In the next section, we’ll take a look at more complex uses of if statements in Java.
Intermediate-Level If Statements
Once you’ve mastered the basics of if statements in Java, it’s time to explore more complex uses. Two such techniques are nested if statements and the use of logical operators.
Nested If Statements
A nested if statement is an if statement inside another if statement. It allows you to test for multiple conditions and execute different code blocks depending on the outcome. Here’s an example:
int score = 85;
if (score >= 50) {
if (score >= 75) {
System.out.println("Excellent!");
} else {
System.out.println("Good job, but you can do better.");
}
} else {
System.out.println("You need to work harder.");
}
// Output:
// 'Excellent!'
In this example, we first check if the score
is greater than or equal to 50. If it is, we then check if it’s also greater than or equal to 75. Depending on the results of these tests, we print different messages to the console.
If Statements with Logical Operators
Logical operators such as &&
(and), ||
(or), and !
(not) can be used in the condition of an if statement to test multiple criteria at once. Here’s an example:
int age = 20;
boolean isCitizen = true;
if (age >= 18 && isCitizen) {
System.out.println("You are eligible to vote.");
}
// Output:
// 'You are eligible to vote.'
In this example, the if statement checks two conditions: whether age
is greater than or equal to 18, and whether isCitizen
is true. Only if both conditions are true does it print ‘You are eligible to vote.’ to the console.
These advanced techniques allow for more complex decision-making in your Java programs. However, they also require careful attention to detail to avoid errors and unexpected behavior.
Exploring Alternatives to If Statements in Java
While if statements are a crucial part of controlling the flow of your program, Java offers other control flow statements that can serve as alternatives or complements to if statements. Two such alternatives are switch statements and ternary operators.
The Power of Switch Statements
A switch statement can be used instead of a complex if-else chain when you need to select one of many code blocks to be executed.
int day = 3;
String dayOfWeek;
switch (day) {
case 1:
dayOfWeek = "Monday";
break;
case 2:
dayOfWeek = "Tuesday";
break;
case 3:
dayOfWeek = "Wednesday";
break;
// ...and so on for the rest of the week
}
System.out.println(dayOfWeek);
// Output:
// 'Wednesday'
In this example, the switch statement checks the value of day
, and depending on that value, assigns a different string to dayOfWeek
. This is much cleaner and more readable than a long chain of if-else statements.
Ternary Operators: A Shorter Alternative
The ternary operator is a shorter form of an if-else statement. It’s a one-liner that tests a condition and returns a value based on whether the condition is true or false.
int score = 85;
String result = (score >= 50) ? "Pass" : "Fail";
System.out.println(result);
// Output:
// 'Pass'
In this example, the ternary operator checks if score
is greater than or equal to 50. If it is, it assigns the string ‘Pass’ to result
. If not, it assigns ‘Fail’. This is much shorter and more concise than an equivalent if-else statement.
While these alternatives offer their own advantages, they also have their own drawbacks. Switch statements can be more verbose for simple conditions, and ternary operators can be harder to read for complex conditions. It’s essential to choose the right tool for the job based on your specific situation.
Troubleshooting If Statements in Java
While if statements are a cornerstone of Java, they can sometimes lead to unexpected results. Let’s explore some common pitfalls and best practices to avoid them.
The Importance of Braces
Forgetting to use braces {}
is a common mistake. In Java, if you don’t use braces after an if statement, only the first line of code will be considered part of the if block. Here’s an example:
int x = 10;
int y = 5;
if (x > y)
System.out.println("x is greater than y");
System.out.println("This will print regardless of the condition.");
// Output:
// 'x is greater than y'
// 'This will print regardless of the condition.'
In this example, the second print statement executes regardless of whether x
is greater than y
because it’s not enclosed in braces. Always remember to use braces when you want multiple lines of code to be part of the if block.
Using the Correct Comparison Operator
Another common pitfall is using the wrong comparison operator. For example, using =
(assignment) instead of ==
(equality) can lead to unexpected results.
int x = 10;
if (x = 5) {
System.out.println("This will cause a compile error.");
}
In this example, using x = 5
in the if statement will cause a compile error because =
is an assignment operator, not a comparison operator. To check if x
is equal to 5, you should use x == 5
.
Being aware of these common mistakes and following these best practices can help you avoid errors and write more robust Java code.
Unveiling Java Control Flow
Control flow is a fundamental concept in programming that refers to the order in which the program’s code executes. In Java, this is primarily controlled by control flow statements, which include if statements, switch statements, for loops, while loops, and more.
If Statements: The Traffic Cops of Java
Among these, if statements play a pivotal role. They act like traffic cops, directing the flow of your program based on certain conditions. If a condition is true, the code within the if statement executes. If not, the code is skipped, and the program moves on to the next part of the code.
int temperature = 25;
if (temperature > 30) {
System.out.println("It's a hot day!");
}
// No output, as the condition is false
In this example, the condition temperature > 30
is false, so the print statement within the if statement is skipped, and there’s no output.
The Role of Boolean Expressions
The conditions in if statements are boolean expressions, meaning they evaluate to either true
or false
. These expressions can involve comparisons (like x > y
), logical operators (like x > y && x < z
), or method calls that return a boolean value (like string.isEmpty()
).
Understanding control flow and the role of if statements in Java is crucial to writing effective and efficient code. As you gain more experience, you’ll find that mastering these concepts can greatly enhance your problem-solving skills in programming.
If Statements in the Larger Context
If statements don’t exist in isolation. They’re often used in conjunction with other control flow statements, such as loops and methods, to create more complex and powerful programs.
If Statements and Loops
For example, you can use if statements within loops to perform certain actions only when specific conditions are met.
for (int i = 1; i <= 10; i++) {
if (i % 2 == 0) {
System.out.println(i + " is even.");
}
}
// Output:
// '2 is even.'
// '4 is even.'
// '6 is even.'
// '8 is even.'
// '10 is even.'
In this example, the if statement checks if the current number i
is even. If it is, it prints a message to the console. This happens on each iteration of the loop, allowing us to check and respond to different conditions at each step.
If Statements and Methods
If statements can also be used within methods to control the method’s behavior based on its inputs.
public void greet(String name) {
if (name == null || name.isEmpty()) {
System.out.println("Hello, stranger!");
} else {
System.out.println("Hello, " + name + "!");
}
}
// Usage:
// greet(null);
// greet("Alice");
// Output:
// 'Hello, stranger!'
// 'Hello, Alice!'
In this example, the greet
method checks if the input name
is null or empty. If it is, it prints a generic greeting. If not, it prints a personalized greeting. This allows the method to respond appropriately to different inputs.
Further Resources for Mastering Java Control Flow
If you’re interested in diving deeper into control flow in Java, here are some resources that you might find helpful:
- Demystifying Loops in Java – Discover how loops contribute to code modularity and reusability in Java.
Java Else-If – Explore the else-if ladder in Java for handling multiple conditional branches.
Using Break in Java – Learn how to use break to terminate loop execution based on certain conditions.
Oracle’s Java Tutorials covers all aspects of Java, including in-depth discussions on control flow.
Java Conditions and If Statements by W3Schools explains how to use conditions and ‘if’ statements in Java.
Learn Java course includes several lessons and projects that will help you master control flow in Java.
Wrapping Up: Mastering If Statements in Java
In this comprehensive guide, we’ve navigated through the world of if statements in Java, a cornerstone of control flow in programming.
We began with the basics, understanding the syntax and usage of if statements, and how they serve as the traffic cops of your Java programs. We then delved into more complex uses, exploring nested if statements and the use of logical operators to test multiple conditions simultaneously.
Along the way, we’ve tackled common pitfalls, such as forgetting to use braces or using the wrong comparison operator, and provided best practices to avoid these errors. We’ve also explored alternative control flow statements, such as switch statements and ternary operators, giving you a broader perspective on controlling the flow of your program.
Here’s a quick comparison of the methods we’ve discussed:
Method | Complexity | Use Case |
---|---|---|
If Statements | Low to Moderate | Testing single or multiple conditions |
Switch Statements | Moderate | Selecting one of many code blocks to execute |
Ternary Operators | Low | Testing a condition and returning a value based on the result |
Whether you’re a beginner just starting out with if statements or an experienced developer looking to brush up on your skills, we hope this guide has been a helpful journey through the ins and outs of if statements in Java.
Mastering if statements and control flow is a crucial step in becoming a proficient Java programmer. Now, you’re well equipped to handle any condition your program might throw at you. Happy coding!