How To Set A Java Timer: A Task Scheduling Guide
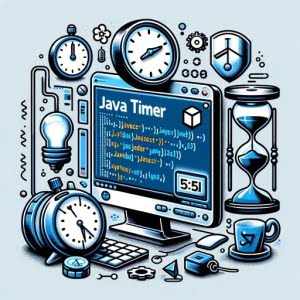
Are you finding it challenging to schedule tasks in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled timekeeper, Java’s Timer class allows you to schedule tasks for future execution in a background thread. These tasks can run on any system, even those without Java installed.
This guide will walk you through the ins and outs of using the Timer class in Java, from creating a basic timer to scheduling complex recurring tasks. We’ll explore Timer’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Java Timer!
TL;DR: How Do I Create and Use a Timer in Java?
In Java, you can create a timer with the following code:
Timer timer = new Timer();
to define the task you will simply create a TimerTask:TimerTask task = new TimerTask()
. Here’s a simple example:
Timer timer = new Timer();
TimerTask task = new TimerTask() {
public void run() {
System.out.println('Task executed');
}
};
timer.schedule(task, 5000);
// Output:
// 'Task executed'
In this example, we create a new Timer object and a TimerTask. The TimerTask is an abstract class that implements the Runnable interface, and we override its run()
method to define the task we want to execute. We then use the schedule
method of the Timer class to execute the task after a delay of 5000 milliseconds (or 5 seconds).
This is a basic way to use the Timer class in Java, but there’s much more to learn about scheduling tasks and handling complex scenarios. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with Java Timer
- Scheduling Recurring Tasks with Java Timer
- Exploring Alternatives to Java Timer
- Troubleshooting Common Java Timer Issues
- Understanding Multithreading in Java
- Applying Java Timer in Real-World Applications
- Further Resources for Mastering Java Timer
- Wrapping Up: Mastering Java Timer for Efficient Task Scheduling
Getting Started with Java Timer
The Java Timer class is a utility class used for scheduling tasks for future execution in a background thread. This can be highly useful when you want to execute certain tasks at a specific time in the future or at regular intervals.
Creating a Timer and a TimerTask
To use a Timer, we first need to create an instance of the Timer class. Additionally, we need to define the task we want to execute. This is done using the TimerTask class, which is an abstract class that implements the Runnable interface.
Here’s a simple example of how to create a Timer and a TimerTask:
Timer timer = new Timer();
TimerTask task = new TimerTask() {
public void run() {
System.out.println('Task executed');
}
};
In this code, we first create a new Timer object. Then, we define a TimerTask by creating an anonymous subclass of TimerTask and overriding its run()
method.
The run()
method is where we define the task we want to execute. In this example, the task is simply printing ‘Task executed’ to the console.
Scheduling a Task
Once we have a Timer and a TimerTask, we can schedule the task using the schedule
method of the Timer class. Here’s how to do it:
timer.schedule(task, 5000);
// Output:
// (After 5 seconds)
// 'Task executed'
The schedule
method takes two arguments: the task to execute (an instance of TimerTask) and the delay before execution (in milliseconds). In this example, the task will be executed once after a delay of 5000 milliseconds (or 5 seconds).
Understanding the Timer Class
The Timer class works by creating a single background thread. This thread is used to execute all scheduled TimerTasks. Once a TimerTask has been scheduled, it is not affected by changes to the system clock.
One of the advantages of the Timer class is its simplicity. It provides an easy way to schedule tasks for future execution. However, it also has some potential pitfalls. For example, if a TimerTask takes too long to execute, it can delay the execution of other TimerTasks. Also, if a TimerTask throws an unchecked exception, it can terminate the thread and cause other scheduled tasks to be cancelled.
Scheduling Recurring Tasks with Java Timer
The Timer class in Java not only allows you to schedule a task to be executed once after a delay, but it also provides a way to schedule tasks to be executed repeatedly at fixed intervals. This is done using the scheduleAtFixedRate
method.
Using the scheduleAtFixedRate
Method
Here’s an example of how to use the scheduleAtFixedRate
method to schedule a task to be executed every second (1000 milliseconds):
Timer timer = new Timer();
TimerTask task = new TimerTask() {
public void run() {
System.out.println('Task executed');
}
};
timer.scheduleAtFixedRate(task, 0, 1000);
// Output:
// 'Task executed'
// (after 1 second)
// 'Task executed'
// (after another second)
// 'Task executed'
// ... and so on every second
In this code, the scheduleAtFixedRate
method is called with three arguments: the task to execute, the delay before the first execution, and the period between subsequent executions. The task is executed immediately (since the delay is 0) and then every second.
schedule
vs scheduleAtFixedRate
While both schedule
and scheduleAtFixedRate
can be used to schedule recurring tasks, there are some key differences between them:
schedule
: This method schedules the next execution of a task relative to the time when the previous execution finished. If an execution of the task takes longer than the specified period, the next execution will be delayed.scheduleAtFixedRate
: This method schedules the next execution of a task relative to the time when the previous execution was supposed to finish. If an execution of the task takes longer than the specified period, the next execution will not be delayed.
In general, scheduleAtFixedRate
is more suitable for tasks that need to be executed at a consistent rate, such as updating a display or sending a regular status report. On the other hand, schedule
is more suitable for tasks where it’s important to maintain a certain period of time between the end of one execution and the start of the next, such as polling a remote server or database.
Exploring Alternatives to Java Timer
While the Java Timer class is a powerful tool for task scheduling, it’s not the only option available. There are alternatives that provide more flexibility and control, such as the ScheduledExecutorService.
ScheduledExecutorService: A Robust Alternative
The ScheduledExecutorService is an interface in Java that can schedule commands to run after a given delay or to execute periodically. Unlike Timer, it supports multiple threads, so it can execute multiple tasks concurrently.
Here’s an example of how to use the ScheduledExecutorService:
ScheduledExecutorService executor = Executors.newScheduledThreadPool(1);
Runnable task = () -> System.out.println('Task executed');
executor.scheduleAtFixedRate(task, 0, 1, TimeUnit.SECONDS);
// Output:
// 'Task executed'
// (after 1 second)
// 'Task executed'
// (after another second)
// 'Task executed'
// ... and so on every second
In this code, we first create a ScheduledExecutorService with a single thread. We then define a task (a Runnable) and schedule it to run every second, starting immediately.
Comparing Timer and ScheduledExecutorService
While both Timer and ScheduledExecutorService can be used for scheduling tasks, there are some key differences:
- Concurrency: Timer creates a single thread and executes all tasks on this thread. ScheduledExecutorService, on the other hand, can manage a pool of threads and execute tasks concurrently.
Error handling: If a TimerTask throws an unchecked exception, it terminates the thread and causes subsequent tasks to be cancelled. ScheduledExecutorService, however, isolates individual tasks and prevents a failing task from affecting others.
Flexibility: ScheduledExecutorService provides more flexible scheduling options, such as fixed delay (scheduleWithFixedDelay) and single-shot delay (schedule).
In general, ScheduledExecutorService is a more robust and flexible alternative to Timer. It’s especially useful when you need to execute multiple tasks concurrently or when you need more control over task scheduling and execution.
Troubleshooting Common Java Timer Issues
Even with its simplicity and utility, using the Java Timer class can sometimes be a bit tricky. Let’s explore some common issues you might encounter and discuss how to handle them effectively.
Handling Exceptions
One of the main issues with the Timer class is that if a TimerTask throws an unchecked exception, it can terminate the timer thread and cause subsequent tasks to be cancelled. To avoid this, you can wrap your task code in a try-catch block to handle any potential exceptions.
Here’s an example:
TimerTask task = new TimerTask() {
public void run() {
try {
// Task code
System.out.println('Task executed');
} catch (Exception e) {
// Handle exception
e.printStackTrace();
}
}
};
In this code, if an exception occurs during the execution of the task, it will be caught and handled by the catch block, preventing it from terminating the timer thread.
Dealing with Thread Safety
Since the Timer class uses a single background thread to execute all tasks, it’s important to ensure that your tasks are thread-safe. If a task modifies shared data, it should synchronize access to this data to prevent race conditions.
Here’s an example of how to synchronize access to shared data in a TimerTask:
int count = 0;
TimerTask task = new TimerTask() {
public void run() {
synchronized (this) {
count++;
System.out.println('Task executed ' + count + ' times');
}
}
};
In this code, the synchronized
keyword is used to ensure that only one thread can execute the block of code inside the synchronized
block at a time. This prevents multiple threads from modifying the count
variable simultaneously, which could lead to inconsistent results.
Remember, while the Timer class is a simple and easy-to-use tool for task scheduling in Java, it’s important to be aware of these potential issues and know how to handle them effectively.
Understanding Multithreading in Java
To fully grasp the Java Timer class’s functionality, it’s crucial to understand the concept of multithreading in Java. Multithreading is a core concept in Java, allowing multiple sequences of code (threads) to run concurrently within a single program.
The Role of Threads
In Java, every program has at least one thread – the main thread. However, additional threads can be created to perform tasks simultaneously. These threads can execute independently, making optimal use of CPU resources, especially in systems with multiple CPUs or cores.
Here’s a simple example of creating a new thread in Java:
Thread thread = new Thread() {
public void run() {
System.out.println('Hello from new thread');
}
};
thread.start();
// Output:
// 'Hello from new thread'
In this code, we create a new Thread object and override its run()
method to define the task we want to execute. We then start the thread using the start()
method, which causes the run()
method to be called in a separate thread.
How Timer Utilizes Background Threads
The Timer class in Java utilizes these principles of multithreading. When you create a Timer, it creates a single background thread. This thread is used to execute all tasks scheduled through that Timer.
When a task is scheduled using the Timer’s schedule
or scheduleAtFixedRate
method, it doesn’t block the main thread. Instead, it’s executed in the Timer’s background thread at the specified time or interval.
This ability to execute tasks in a separate thread without blocking the main thread makes the Timer class a valuable tool for task scheduling in Java.
Applying Java Timer in Real-World Applications
The Timer class in Java is not just a theoretical concept; it has practical applications in various real-world scenarios. Let’s explore some of them.
Scheduling Tasks in Web Applications
In web applications, the Timer class can be used to schedule tasks such as sending periodic emails, cleaning up old data, generating reports, and more. Here’s a simple example of using a Timer to send an email every day at a specific time:
TimerTask emailTask = new EmailTask(); // Assume EmailTask is a TimerTask that sends an email
Calendar scheduleTime = Calendar.getInstance();
scheduleTime.set(Calendar.HOUR_OF_DAY, 9); // 9 AM
scheduleTime.set(Calendar.MINUTE, 0);
scheduleTime.set(Calendar.SECOND, 0);
Timer timer = new Timer();
timer.scheduleAtFixedRate(emailTask, scheduleTime.getTime(), TimeUnit.MILLISECONDS.convert(1, TimeUnit.DAYS));
In this code, we first create a TimerTask (emailTask
) that sends an email. We then set the time we want to send the email (9 AM). We create a Timer and schedule the email task to run at the specified time every day.
Using Timer in Games
In game development, the Timer class can be used to control game mechanics, such as spawning enemies, controlling animations, or updating the game state. Here’s a simple example of using a Timer to update the game state every second:
TimerTask gameUpdateTask = new GameUpdateTask(); // Assume GameUpdateTask is a TimerTask that updates the game state
Timer timer = new Timer();
timer.scheduleAtFixedRate(gameUpdateTask, 0, 1000);
In this code, we create a TimerTask (gameUpdateTask
) that updates the game state. We then create a Timer and schedule the game update task to run every second.
Exploring Related Concepts
While the Timer class is a powerful tool, there are other related concepts that you might find useful, such as thread pools and Executors. These concepts offer more control and flexibility in managing and scheduling tasks.
Further Resources for Mastering Java Timer
For those looking to delve deeper into the Java Timer class and related concepts, here are some resources that you might find helpful:
- Best Practices for Java Classes – Understand proper handling of Java classes quickly.
Working with Colors in Java – Learn how to work with colors in Java to add visual appeal to your applications.
Java Randomization Techniques – Dive into Java’s random number generation for creating unpredictable outcomes.
Java Concurrency Tutorial – A guide to threads, Executors, and synchronization in Java.
Java Timer and TimerTask – Reminder Class Tutorials – A detailed tutorial on using the Timer and TimerTask classes.
Java ScheduledExecutorService Tutorial – An in-depth tutorial on an alternative to the Timer class.
Wrapping Up: Mastering Java Timer for Efficient Task Scheduling
In this comprehensive guide, we’ve delved deep into the world of Java Timer, a versatile class for scheduling tasks in Java. We’ve explored how to use this class to create timers, define tasks, and schedule them for future execution in a background thread.
We began with the basics of creating a Timer and a TimerTask and scheduling a task with a delay. We then ventured into more advanced territory, learning how to schedule recurring tasks and understanding the differences between the schedule
and scheduleAtFixedRate
methods.
Along the way, we tackled common issues you might encounter when using the Java Timer class, such as handling exceptions and ensuring thread safety. We also discussed the underlying concept of multithreading in Java and how the Timer class utilizes it.
We also ventured beyond the Timer class, exploring the more robust and flexible ScheduledExecutorService as an alternative for task scheduling in Java. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Timer | Simple and easy to use | Single thread, Can be terminated by unchecked exceptions |
ScheduledExecutorService | Supports multiple threads, More flexible scheduling options, Isolates individual tasks | More complex |
Whether you’re just starting out with Java Timer or you’re an experienced developer looking for a refresher, we hope this guide has given you a deeper understanding of how to use the Timer class effectively for task scheduling in Java.
With its simplicity and versatility, the Timer class is a powerful tool in any Java developer’s toolkit. Happy coding!