Coding with Online Java Editors/IDEs | Developer’s Guide
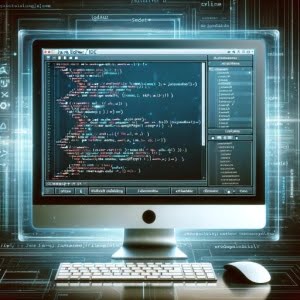
Ever found yourself needing to write and run Java code but without access to your usual development environment? You’re not alone. Many developers find themselves in situations where they need a portable and convenient solution for their Java coding needs.
Think of an online Java editor/IDE as a portable workstation – a tool that allows you to write, compile, and run Java code from anywhere, at any time, directly in your web browser. This means no more being tied down to a specific machine or having to install and configure a local development environment.
In this guide, we’ll dive deep into the world of online Java editors/IDEs. We’ll explore what they are, how they work, and how to choose the best one for your needs. We’ll cover everything from basic usage for beginners to advanced features for more experienced users, as well as alternative approaches for experts.
Let’s get started on your journey to mastering the online Java editor/IDE!
TL;DR: What is an Online Java Editor/IDE?
An
online Java editor/IDE
is a web-based platform that provides a comprehensive environment for you to write, edit, and manage your Java code directly in your web browser, without the need for installing any software on your local machine. Some examples include platforms like –Replit IDE
,Codiva.io
,Online IDE
.
Here’s a simple example of how you might use an online Java editor/IDE like Replit IDE
:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, we’ve written a basic ‘Hello, World!’ program in Java. This code can be written, compiled, and run directly in an online Java editor/IDE, with the output displayed in the browser.
This is just a basic introduction to online Java editors/IDEs. There’s a lot more to learn about these powerful tools, including how to choose the best one for your needs, how to use their advanced features, and how to troubleshoot common issues. Continue reading for a detailed comparison and review of popular online Java editors/IDEs.
Table of Contents
- Getting Started with Online Java Editors/IDEs
- Pros and Cons of Online Java Editors/IDEs
- Leveraging Advanced Features of Online Java Editors/IDEs
- Comparing Online Java Editors/IDEs
- Exploring Alternative Online Java Environments
- Comparing Alternatives to Online Java Editors/IDEs
- Navigating Troubles in Online Java Editors/IDEs
- Best Practices for Using Online Java Editors/IDEs
- Understanding IDEs and Their Importance
- IDE vs. Editor: What’s the Difference?
- Broadening Your Horizon: Online Java Editor/IDE and More
- Wrapping Up: Online Java Editors/IDEs
Getting Started with Online Java Editors/IDEs
Using an online Java editor/IDE is a straightforward process, even for beginners. Let’s walk through the steps of writing, compiling, and running a simple Java program in an online environment.
Step 1: Access the Online Editor/IDE
First, open your preferred online Java editor/IDE in your web browser. For this example, we’ll use Repl.it
, but the process is similar for other platforms like JDoodle or Codiva.
Step 2: Write Your Java Program
Next, write your Java program in the code editor. Here’s a simple ‘Hello, World!’ program:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
This program defines a class named ‘HelloWorld’ and a main method that prints ‘Hello, World!’ to the console.
Step 3: Compile and Run Your Program
After writing your program, click the ‘Run’ button (or equivalent) in the online editor/IDE. This will compile and run your program, with the output displayed in the console.
# Output:
# Hello, World!
And there you have it! You’ve written, compiled, and run a Java program in an online editor/IDE.
Pros and Cons of Online Java Editors/IDEs
Online Java editors/IDEs offer several advantages over traditional desktop IDEs, especially for beginners. They’re accessible from any machine with a web browser, require no installation or setup, and often include built-in tutorials and resources for learning Java. They’re also great for quick prototyping and sharing code with others.
However, online editors/IDEs also have some limitations. They may not offer as many features as desktop IDEs, and their performance can be affected by your internet connection. Additionally, while they’re generally secure, they may not be the best choice for working with sensitive data.
In the end, the best choice depends on your specific needs and circumstances. If you’re a beginner, an online Java editor/IDE can be a great way to get started with Java programming.
Leveraging Advanced Features of Online Java Editors/IDEs
As you become more comfortable with Java programming, you’ll want to start using some of the advanced features that online Java editors/IDEs offer. These features can significantly enhance your coding experience and productivity.
Code Completion
Code completion, also known as autocompletion, is a feature that suggests and inserts code as you type. This feature can save you time, reduce typing errors, and even help you learn new APIs.
Here’s an example of how code completion works in an online Java editor/IDE:
public class HelloWorld {
public static void main(String[] args) {
System.out. // At this point, the IDE might suggest 'println', 'print', 'printf', etc.
}
}
As you type System.out.
, the IDE suggests possible completions like println
, print
, and printf
. You can then select the suggestion you want, and the IDE will insert it for you.
Debugging
Online Java editors/IDEs often include debugging tools that let you step through your code, inspect variables, and understand exactly what your program is doing. These tools can be invaluable when you’re trying to find and fix bugs in your code.
Here’s an example of how you might use a debugger in an online Java editor/IDE:
public class HelloWorld {
public static void main(String[] args) {
int x = 5;
int y = x + 2;
System.out.println(y);
}
}
# Output:
# 7
In this example, you could use a debugger to step through the code line-by-line, inspect the values of x
and y
at each step, and see how the value of y
is calculated.
Version Control
Many online Java editors/IDEs integrate with version control systems like Git, allowing you to track changes to your code, collaborate with others, and even deploy your code to production. This feature can be a game-changer if you’re working on a team or on a large, complex project.
Here’s an example of how you might use version control in an online Java editor/IDE:
# Clone a Git repository
git clone https://github.com/your-username/your-repository.git
# Make changes to your code
# Commit your changes
git commit -m "Your commit message"
# Push your changes to the repository
git push origin master
In this example, you clone a Git repository, make changes to your code, commit your changes, and push your changes to the repository. All of this can be done directly in the online Java editor/IDE.
Comparing Online Java Editors/IDEs
Different online Java editors/IDEs offer different sets of advanced features. For example, Replit IDE
offers real-time collaboration, Online IDE
provides a simple and straightforward interface, and Codiva.io
supports automatic compilation and error reporting. It’s important to explore different options and choose the one that best fits your needs.
Exploring Alternative Online Java Environments
While online Java editors/IDEs provide an accessible and user-friendly platform for coding, there are other online alternatives for writing and running Java code. These alternatives can offer more advanced features, more control, or better integration with other tools and services.
AWS Cloud9
AWS Cloud9 is a cloud-based integrated development environment (IDE) that lets you write, run, and debug your code with just a browser. It includes a code editor, debugger, and terminal. Cloud9 also provides seamless integration with other AWS services.
Here’s an example of running a Java program in AWS Cloud9:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
After writing this program in the Cloud9 editor, you can run it directly in the Cloud9 terminal and see the output there.
Google Cloud Shell
Google Cloud Shell is an interactive shell environment for Google Cloud Platform. It provides command-line access to your GCP resources directly from your browser. You can use it to deploy applications, manage projects, and work with virtual machines. Google Cloud Shell also includes a web-based editor with support for Java.
Here’s an example of running a Java program in Google Cloud Shell:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
After writing this program in the Cloud Shell editor, you can run it directly in the Cloud Shell terminal and see the output there.
Comparing Alternatives to Online Java Editors/IDEs
Cloud-based development environments like AWS Cloud9 and Google Cloud Shell offer some advantages over online Java editors/IDEs. They provide a more comprehensive and integrated development environment, including access to a full Unix shell, direct integration with cloud services, and persistent workspace storage. However, they may be more complex and overkill for simple Java programs.
Online Java editors/IDEs, on the other hand, are simpler and more focused. They provide a straightforward and intuitive interface for writing, compiling, and running Java code. They are accessible from any web browser and require no setup or configuration. However, they may lack some of the advanced features and integrations of a full-featured cloud-based IDE.
Ultimately, the best choice depends on your specific needs and circumstances. Whether you choose an online Java editor/IDE, a cloud-based IDE, or some other alternative, the important thing is to find a tool that supports your workflow and makes you more productive.
While online Java editors/IDEs are powerful tools, they do come with their own set of challenges. Let’s discuss some common issues you might encounter and how to navigate them.
Network Latency
Since online Java editors/IDEs operate over the internet, they’re subject to network latency. This means your coding experience might be less responsive compared to a local IDE, especially if your internet connection is slow or unstable.
Limited Processing Power
Online Java editors/IDEs typically run on shared servers, which means they might not offer the same level of processing power as a local IDE running on your own machine. This could slow down compilation and execution of more complex programs.
Security Concerns
When you’re coding online, you’re sending your code over the internet, which raises potential security concerns. It’s important to ensure that the online Java editor/IDE you’re using employs strong security measures, such as encryption and secure coding practices.
Best Practices for Using Online Java Editors/IDEs
Here are some tips to help you mitigate these issues and get the most out of your online Java editor/IDE:
- Use a Reliable Internet Connection: A stable and fast internet connection can help mitigate network latency. If you’re experiencing lag, you might want to try switching to a more reliable network.
Code Efficiently: To cope with limited processing power, try to write efficient code. Avoid unnecessary computations, and break down complex tasks into simpler ones.
Secure Your Code: Be mindful of security when using an online Java editor/IDE. Avoid storing sensitive data in your code, and make sure to use secure connections (HTTPS) when accessing the editor/IDE.
Remember, while online Java editors/IDEs offer great convenience, they also require some considerations. By understanding these issues and how to navigate them, you can make the most of your online coding experience.
Understanding IDEs and Their Importance
IDE stands for Integrated Development Environment. It’s a software application that provides a comprehensive set of tools for programmers. These tools often include a text editor for writing code, a compiler or interpreter to transform code into executable programs, and a debugger to find and fix errors.
Here’s a simple example of using an IDE to write, compile, and run a Java program:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, you write the Java program in the IDE’s text editor, then use the IDE’s compiler to transform the Java code into an executable program. Finally, you run the program in the IDE, and the output ‘Hello, World!’ is displayed.
IDEs are particularly useful for Java programming because they can handle the complexity of Java’s compilation process, provide helpful code completion and error checking, and offer powerful debugging tools.
IDE vs. Editor: What’s the Difference?
You might be wondering: What’s the difference between an IDE and a text editor? After all, both can be used to write code.
The main difference lies in their features and complexity. A text editor is a simple tool for writing and editing text files, including code files. It might offer features like syntax highlighting and line numbers, but it doesn’t compile or run code.
Here’s an example of writing a Java program in a text editor:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In this example, you can write the Java program in the text editor, but you can’t compile or run it directly in the editor. You would need to use a separate tool to do that.
An IDE, on the other hand, is a more powerful tool that integrates a text editor with other development tools, such as a compiler, debugger, and version control system. This integration makes it easier to write, test, and debug code, especially for complex programming languages like Java.
Broadening Your Horizon: Online Java Editor/IDE and More
Online Java editors/IDEs are just one piece of the puzzle in the Java development ecosystem. They are incredibly useful tools, especially for beginners and those who need to code on the go. However, to become a proficient Java developer, it’s important to understand how these tools fit into the broader context of Java development.
Exploring Java Frameworks
Java frameworks are pre-written code libraries that provide a foundation for your own applications. They can significantly speed up your development process by providing solutions for common programming tasks. Some popular Java frameworks you might want to explore include Spring, Hibernate, and Apache Struts.
Mastering Build Tools
Build tools are used to automate the process of compiling, testing, and packaging Java applications. They can save you a lot of time and effort, especially for larger projects. Maven and Gradle are two of the most popular build tools in the Java ecosystem.
Navigating Deployment Strategies
Deployment is the process of making your Java application available for use. This can involve packaging your application, configuring servers, and managing updates. Understanding different deployment strategies can help you deliver your Java applications more effectively and reliably.
Further Resources for Java and IDEs
To continue your journey in mastering online Java editors/IDEs and the broader Java development ecosystem, consider exploring the following resources:
- Comprehensive Java Tutorial for Beginners – Get started with Java programming basics in this guide.
Online Java Compiler Overview – Explore online compilers for writing, compiling, and executing Java code in your browser.
Using JDoodle for Java Development – Discover how JDoodle enables coding and testing Java programs online.
Oracle’s Java Tutorials cover everything from the basics to advanced topics.
The Eclipse Foundation – Eclipse Che is a cloud-based IDE that supports Java and many other languages.
IntelliJ IDEA Guide provides a wealth of information on Java development tools and practices.
Wrapping Up: Online Java Editors/IDEs
In this comprehensive guide, we’ve delved into the world of online Java editors/IDEs, exploring their functionality, use cases, and the considerations that come with them.
We began with the basics, understanding what an online Java editor/IDE is and how to use it. We’ve seen how to write, compile, and run Java code directly in the browser, with platforms like Repl.it, JDoodle, and Codiva serving as our examples.
We ventured into more advanced territory, exploring the advanced features of online Java editors/IDEs, such as code completion, debugging, and version control. We also discussed alternative approaches, exploring cloud-based development environments like AWS Cloud9 and Google Cloud Shell.
Along the way, we tackled common challenges you might face when using online Java editors/IDEs, such as network latency, limited processing power, and security concerns, providing you with solutions and best practices for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Accessibility | Processing Power | Security |
---|---|---|---|
Online Java editor/IDE | High | Moderate | High |
Cloud-based IDE (e.g., AWS Cloud9, Google Cloud Shell) | High | High | High |
Whether you’re just starting out with online Java editors/IDEs or you’re looking to level up your Java programming skills, we hope this guide has given you a deeper understanding of online Java editors/IDEs and their capabilities.
With their accessibility, ease of use, and powerful features, online Java editors/IDEs are a valuable tool for any Java developer. Happy coding!