Java Tutorial: A Beginner’s Journey Into Programming
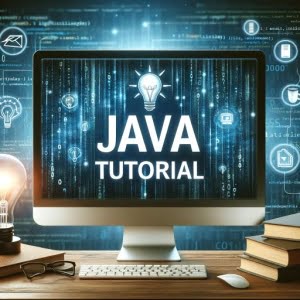
Are you eager to dive into the world of Java programming but unsure where to plunge? You’re not alone. Many aspiring developers find themselves at the edge, hesitant to jump into the vast ocean of Java. Think of this tutorial as your lifeboat, guiding you through the basics of Java and helping you navigate its more complex concepts.
Java, one of the most popular programming languages in the world, is like a universal language, spoken by millions of developers and understood by billions of devices. It’s the language behind many of the applications and websites you use daily.
This guide aims to provide a comprehensive introduction to Java, from setting up your environment, writing your first Java program, to understanding the basic syntax and structure. We’ll also delve into more complex Java topics such as object-oriented programming, data types, operators, loops, and arrays. So, let’s set sail on this exciting journey into the world of Java programming!
TL;DR: How Can I Start Learning Java?
To kickstart your Java learning journey, you first need to install the
Java Development Kit (JDK)
on your computer. Then, you can write a simple'Hello, World!'
program to get a feel for the basic syntax. This guide will walk you through these steps and much more.
Here’s a simple example of a ‘Hello, World!’ program in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
# Output:
# 'Hello, World!'
In this example, we’ve created a public class named ‘HelloWorld’. Inside this class, we have the main method, which is the entry point for any Java program. The System.out.println('Hello, World!');
line is used to print the text ‘Hello, World!’ to the console.
This is just the beginning of your Java adventure. Continue reading for a more detailed tutorial on Java programming, covering everything from setting up your environment to understanding and writing complex Java programs.
Table of Contents
- Setting Up Your Java Environment
- Writing Your First Java Program
- Understanding the Basic Syntax and Structure of a Java Program
- Deep Dive into Java: Intermediate Concepts
- Exploring Alternative Java Learning Resources
- Navigating Java Troubles: Common Issues and Solutions
- Best Practices for Debugging Java Programs
- Understanding Java: The Fundamentals
- Java in Action: Real-World Applications
- Expanding Your Java Horizons: Further Learning
- Wrapping Up: Mastering Java for Programming Success
Setting Up Your Java Environment
Before you can start writing Java programs, you need to set up your Java environment. This involves installing the Java Development Kit (JDK), which includes the Java Runtime Environment (JRE) and the tools you need to develop Java applications.
Here’s a step-by-step guide to installing the JDK:
- Visit the Oracle website and download the JDK installer for your operating system.
- Run the installer and follow the on-screen instructions.
- To verify the installation, open a command prompt or terminal window and type the following command:
java -version
You should see something like this:
java version '11.0.1' 2018-10-16 LTS
Java(TM) SE Runtime Environment 18.9 (build 11.0.1+13-LTS)
Java HotSpot(TM) 64-Bit Server VM 18.9 (build 11.0.1+13-LTS, mixed mode)
This output confirms that you have successfully installed the JDK and that your Java environment is set up correctly.
Writing Your First Java Program
Now that you have your Java environment set up, it’s time to write your first Java program. We’ll start with a simple ‘Hello, World!’ program.
Here’s the code for a basic ‘Hello, World!’ program in Java:
public class HelloWorld {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
# Output:
# 'Hello, World!'
To run this program, save it in a file named ‘HelloWorld.java’, then compile and run it using the following commands:
javac HelloWorld.java
java HelloWorld
The javac
command compiles the Java source file into bytecode, and the java
command runs the bytecode.
Understanding the Basic Syntax and Structure of a Java Program
Let’s break down the ‘Hello, World!’ program to understand the basic syntax and structure of a Java program:
public class HelloWorld
: This line defines a public class named ‘HelloWorld’. In Java, every application must contain a main class that wraps around the entire program.public static void main(String[] args)
: This line defines the main method. This is the entry point for any Java program.System.out.println('Hello, World!');
: This line prints the text ‘Hello, World!’ to the console.
In the next section, we’ll delve into more complex Java topics, such as object-oriented programming, data types, operators, loops, and arrays.
Deep Dive into Java: Intermediate Concepts
After getting our feet wet with basic Java programming, it’s time to dive deeper. This section will explore more complex Java topics such as object-oriented programming, data types, operators, loops, and arrays.
Object-Oriented Programming in Java
Java is fundamentally an object-oriented programming (OOP) language. It revolves around the concept of ‘objects’ which are instances of ‘classes’. These classes are blueprints that define the properties (variables) and behaviors (methods) of an object.
Here’s an example of a class and an object in Java:
public class Dog {
String breed;
int age;
String color;
void barking() {
}
void hungry() {
}
void sleeping() {
}
}
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.breed = 'bulldog';
myDog.age = 5;
myDog.color = 'black';
}
}
# Output:
# No output as the methods are empty.
In this example, Dog
is a class that includes properties like breed, age, and color, and methods like barking(), hungry(), and sleeping(). In the Main
class, we’ve created an object myDog
of the Dog
class and assigned values to its properties.
Java Data Types and Operators
Java has several data types used to store variables. They can be divided into two categories: primitive data types (e.g., int, char, boolean) and reference/object data types (e.g., String, Array, Class).
Here’s an example of Java data types and operators:
int myInt = 10;
char myChar = 'a';
boolean myBoolean = true;
String myString = 'Hello, World!';
# Output:
# No output as these are variable declarations.
In this example, we’ve declared four variables using different data types. An int
to store an integer, a char
to store a character, a boolean
to store a boolean value, and a String
to store a sequence of characters.
Loops and Arrays in Java
Loops are used in programming to repeat a specific block of code. In Java, we have three types of loops: for, while, and do-while.
Arrays are used to store multiple values in a single variable.
Here’s an example of a for loop and an array in Java:
int[] numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
System.out.println(number);
}
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, we’ve created an array of integers and used a for-each loop to iterate through each element of the array and print it to the console.
Understanding these advanced concepts is key to mastering Java programming. In the next section, we’ll explore alternative learning resources and approaches for learning Java.
Exploring Alternative Java Learning Resources
While this tutorial provides a comprehensive guide to Java, it’s important to explore other resources that can complement your learning journey. Let’s discuss some alternative approaches to learning Java, including online courses, books, and coding bootcamps.
Online Courses for Java
Online courses are an excellent way to learn Java at your own pace. They offer video lectures, quizzes, and assignments that provide hands-on experience. Websites like Coursera, Udemy, and Codecademy offer courses ranging from beginner to advanced levels.
Pros:
– Self-paced learning
– Hands-on assignments and projects
– Access to community forums for discussion and help
Cons:
– Some courses can be expensive
– Quality varies between courses
Java Programming Books
Books are a traditional yet effective way to learn Java. They provide in-depth explanations and cover a wide range of topics. Some popular Java books include ‘Head First Java’, ‘Effective Java’, and ‘Java: The Complete Reference’.
Pros:
– In-depth explanations
– Wide range of topics covered
Cons:
– Lack of interactive learning
– Some books can be outdated
Coding Bootcamps for Java
Coding bootcamps are intensive training programs that teach programming skills in a short time. They offer a structured curriculum and hands-on projects. However, they are more suitable for those who can commit full-time.
Pros:
– Structured curriculum
– Hands-on projects
– Job placement assistance
Cons:
– Intensive and time-consuming
– Can be expensive
It’s important to choose the learning resource that best fits your needs and learning style. You might find that a combination of these resources works best for you. Remember, the key to mastering Java or any programming language is practice and consistency. Happy coding!
As with learning any new skill, you’re bound to encounter some hurdles on your Java journey. But don’t worry, most issues beginners face when learning Java such as syntax errors, runtime errors, and logical errors are common and can be resolved with a bit of troubleshooting. Let’s discuss these issues and how to solve them.
Syntax Errors in Java
Syntax errors, also known as ‘compile-time errors’, occur when the code violates the language’s syntax rules. For example, forgetting a semicolon at the end of a statement or misspelling a keyword.
Here’s an example of a syntax error in Java:
public class Main {
public static void main(String[] args) {
System.out.println('Hello, World!')
}
}
# Output:
# Error: ';' expected
In this example, we forgot to add a semicolon at the end of the System.out.println('Hello, World!')
statement, which caused a syntax error.
Runtime Errors in Java
Runtime errors, also known as ‘exceptions’, occur during the execution of the program. These errors are typically caused by illegal operations such as dividing by zero or accessing a null object.
Here’s an example of a runtime error in Java:
public class Main {
public static void main(String[] args) {
int result = 10 / 0;
}
}
# Output:
# Exception in thread 'main' java.lang.ArithmeticException: / by zero
In this example, we tried to divide a number by zero, which is not allowed in Java and resulted in a runtime error.
Logical Errors in Java
Logical errors occur when the program compiles and runs without crashing, but it doesn’t produce the expected output. These errors are usually the hardest to detect and fix because they require you to trace and understand the logic of the code.
Here’s an example of a logical error in Java:
public class Main {
public static void main(String[] args) {
int result = 10 / 2;
System.out.println('The result is ' + result);
}
}
# Output:
# 'The result is 5'
In this example, let’s say we expected the result to be 20, but the output was 5. This indicates a logical error in our code.
Best Practices for Debugging Java Programs
Debugging is an essential skill for any programmer. Here are some best practices for debugging Java programs:
- Use a Debugger: Most Integrated Development Environments (IDEs) come with a built-in debugger that allows you to step through your code line by line and inspect variables.
- Print Debugging: You can print variables or messages to the console at different points in your code to track its execution.
- Code Review: Regularly reviewing your code can help you spot logical errors and improve code quality.
- Unit Testing: Writing unit tests for your code can help you catch errors and ensure your code is working as expected.
Remember, encountering errors is a normal part of the programming process. Don’t be discouraged by them. Instead, use them as learning opportunities to improve your problem-solving skills and deepen your understanding of Java.
Understanding Java: The Fundamentals
Java, developed by Sun Microsystems (now owned by Oracle), is a high-level, class-based, object-oriented programming language. It’s designed to be simple enough that many programmers can achieve fluency, yet powerful enough to develop complex applications.
Why is Java Important?
Java has been a dominant force in the world of programming since its introduction in 1995. It’s the language behind many of the applications and websites you use daily. Here’s why Java is so important:
- Platform Independence: Java’s motto is ‘write once, run anywhere’. This means you can write Java code on one machine and run it on any other machine that has a Java Virtual Machine (JVM), regardless of the underlying hardware and operating system.
Object-Oriented Programming: Java fully embraces the object-oriented programming (OOP) paradigm, making it easier to design modular programs and reusable code.
Robust and Secure: Java offers a number of features that enhance the security and robustness of applications, such as garbage collection, exception handling, and strong type checking.
Java vs Other Programming Languages
Java is often compared to other programming languages like C++, Python, and JavaScript. While each language has its strengths and weaknesses, Java stands out for its platform independence, robustness, and widespread use in enterprise environments.
Here’s a brief comparison:
Language | Speed | Syntax | Use Case |
---|---|---|---|
Java | Moderate | Verbose, strict syntax | Enterprise applications, Android apps |
C++ | Fast | Complex, flexible syntax | System software, game development |
Python | Slow | Simple, readable syntax | Data analysis, machine learning |
JavaScript | Moderate | Simple, flexible syntax | Web development |
Principles of Object-Oriented Programming in Java
Java is built around the principles of object-oriented programming. These principles include encapsulation, inheritance, polymorphism, and abstraction.
- Encapsulation is the technique of making the fields in a class private and providing access to them via public methods. It helps to control the way data is accessed or modified.
Inheritance is a mechanism where one class acquires the properties and behaviors of another class. It helps to promote code reusability.
Polymorphism allows a subclass to define its own unique behavior while still sharing the functionalities of its parent class.
Abstraction is a process of hiding the implementation details and showing only the functionality to the user.
Understanding these principles is key to mastering Java. They not only form the backbone of Java programming but also provide a clear structure for the code, making it easier to manage and maintain.
Java in Action: Real-World Applications
Java, with its versatility and widespread usage, finds its place in a variety of real-world applications. Let’s explore how Java is used in different domains and what makes it a popular choice among developers.
Java in Web Development
Java is extensively used in web development to build secure, robust, and scalable web applications. Java Servlets, Java Server Pages (JSP), and JavaServer Faces (JSF) are commonly used technologies in Java web development. Frameworks like Spring and Struts further simplify the process.
Java in Mobile App Development
Java is the primary language for Android app development. It provides a rich set of libraries and tools for building apps for the world’s most widely-used mobile platform. Android Studio, Google’s official IDE for Android app development, is built around Java.
Java in Game Development
Java is also used in game development, powering popular games like ‘Minecraft’. Java’s robustness, cross-platform capabilities, and the ability to create rich, interactive content make it a good choice for game developers.
Expanding Your Java Horizons: Further Learning
After mastering the basics of Java, you might want to explore related topics to further your learning. Here are some suggestions:
- Java Frameworks and Libraries: Frameworks like Spring and Hibernate and libraries like JUnit and Apache Commons can help you build powerful applications more efficiently.
Advanced Java Concepts: Topics like multithreading, generics, and annotations can deepen your understanding of Java.
Java Certification: Earning a Java certification from Oracle can validate your skills and increase your job prospects.
Further Resources for Java Mastery
Here are some additional resources to help you on your Java learning journey:
- Exploring Java Syntax – Explore essential syntax rules and constructs in Java programming language.
Java Code Samples – Learn by example with practical Java code snippets for common tasks and scenarios.
Java Playground Tools – Experiment with Java code snippets or test your programming skills in a sandbox environment.
Oracle’s Official Java Documentation: Comprehensive resource covering everything from basic concepts to advanced topics.
Java Code Geeks offers a wealth of tutorials, examples, and articles on Java programming.
Baeldung covers a wide range of Java topics from basic to advanced levels.
Wrapping Up: Mastering Java for Programming Success
In this comprehensive guide, we’ve journeyed through the world of Java programming, exploring its fundamentals, applications, and the resources available for learning and mastering this powerful programming language.
We began with the basics, understanding how to set up the Java environment and write a simple ‘Hello, World!’ program. This gave us a solid foundation to dive into more complex topics like object-oriented programming, data types, operators, loops, and arrays. Each step of the way, we provided practical code examples to illustrate these concepts and their applications.
Moving forward, we discussed alternative approaches to learning Java, including online courses, books, and coding bootcamps. We also addressed common issues beginners might encounter when learning Java and offered solutions and best practices for debugging Java programs.
Here’s a quick comparison of the learning resources we’ve discussed:
Learning Resource | Pros | Cons |
---|---|---|
Online Courses | Self-paced, hands-on assignments | Can be expensive, quality varies |
Books | In-depth explanations, wide range of topics | Lack of interactive learning, can be outdated |
Coding Bootcamps | Structured curriculum, job placement assistance | Intensive, can be expensive |
Whether you’re just starting out with Java or looking to level up your programming skills, we hope this guide has given you a deeper understanding of Java and its capabilities. With its robustness, platform independence, and widespread use in enterprise environments, Java is a powerful tool for any programmer. Now, you’re well equipped to enjoy the benefits of Java programming. Happy coding!