Java Queue Explained: Your Guide to the Queue Interface
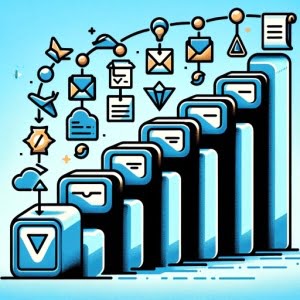
Ever found yourself grappling with Java Queue? You’re not alone. Many developers find it challenging to understand and implement Queue in Java. Think of it like a line at the grocery store – first in, first out. That’s the principle Java Queue operates on.
Java Queue is a powerful tool in a developer’s arsenal, allowing for efficient handling of elements in a ‘First In, First Out’ manner. This makes it a popular choice for various tasks, from data structure management to handling processes in operating systems.
In this guide, we’ll walk you through the process of using Java Queue, from the basics to more advanced techniques. We’ll cover everything from creating and manipulating a Queue, to more complex uses such as PriorityQueue and Deque, as well as troubleshooting common issues.
So, let’s dive in and start mastering Java Queue!
TL;DR: What is a Queue in Java?
A Queue in Java is a collection used to hold multiple elements prior to processing, defined with the syntax:
Queue<Integer> myQueue = new LinkedList<>();
. It follows the FIFO (First In, First Out) principle, meaning the first element that gets added is the first one to be removed.
Here’s a simple example:
Queue<Integer> q = new LinkedList<>();
q.add(1);
q.add(2);
System.out.println(q);
// Output:
// [1, 2]
In this example, we create a Queue of integers and add two elements to it: 1 and 2. When we print the Queue, we see the elements in the order they were added. This is a basic demonstration of how a Queue works in Java.
But there’s much more to Java Queue than this. Continue reading for a more detailed explanation, advanced usage, and how to troubleshoot common issues.
Table of Contents
- Creating and Manipulating a Java Queue: A Beginner’s Guide
- Intermediate Java Queue: PriorityQueue and Deque
- Expert-Level Java: Beyond Queue
- Troubleshooting Java Queue: Common Issues and Solutions
- Understanding the Java Queue Interface and FIFO Principle
- Java Queue in Real-World Applications: Multi-threading and Networking
- Wrapping Up
Creating and Manipulating a Java Queue: A Beginner’s Guide
Java’s Queue interface is part of the Java Collections Framework. It’s used to hold multiple elements that are about to be processed. The Queue follows the FIFO (First In, First Out) principle. Let’s start with the basics: creating a Queue and adding and removing elements.
Creating a Queue in Java
In Java, we can’t directly instantiate a Queue because it’s an interface. Instead, we use classes that implement the Queue interface. LinkedList is one such class. Here’s how to create a Queue using LinkedList:
Queue<Integer> myQueue = new LinkedList<>();
In this code, we’ve created a Queue named ‘myQueue’ that can hold integers. The ” is a Java feature called Diamond Operator. It allows the compiler to infer the type arguments, which makes the code cleaner and easier to read.
Adding Elements to a Queue
We add elements to a Queue using the add()
method. Let’s add some integers to our Queue:
myQueue.add(5);
myQueue.add(10);
myQueue.add(15);
System.out.println(myQueue);
// Output:
// [5, 10, 15]
We’ve added three integers to ‘myQueue’. When we print the Queue, we see the elements in the order they were added.
Removing Elements from a Queue
We remove elements from a Queue using the remove()
method. This method removes and returns the head of the Queue. Let’s remove an element:
int removedElement = myQueue.remove();
System.out.println("Removed element: " + removedElement);
System.out.println(myQueue);
// Output:
// Removed element: 5
// [10, 15]
We removed the head of the Queue, which is the integer 5. When we print the Queue, we see that 5 is no longer there.
That’s the basics of creating and manipulating a Queue in Java. In the following sections, we’ll delve into more complex uses and alternative approaches.
Intermediate Java Queue: PriorityQueue and Deque
As you become more comfortable with Java Queue, you’ll encounter more complex use cases. Two common ones are PriorityQueue and Deque. Let’s explore these in detail.
PriorityQueue in Java
A PriorityQueue is a special type of Queue that orders its elements based on their natural ordering or a custom Comparator provided during Queue construction. Let’s demonstrate this with an example:
Queue<Integer> priorityQueue = new PriorityQueue<>();
priorityQueue.add(15);
priorityQueue.add(10);
priorityQueue.add(20);
System.out.println(priorityQueue);
// Output:
// [10, 15, 20]
In this example, we created a PriorityQueue and added three integers. Although we added the integers in the order 15, 10, 20, the PriorityQueue reorders them based on their natural ordering (in this case, ascending numerical order).
Deque in Java
Deque (pronounced ‘deck’) stands for ‘Double Ended Queue’. It’s a type of Queue where you can add or remove elements from both ends. Deque has various implementations, and one of them is ArrayDeque. Let’s see it in action:
Deque<Integer> deque = new ArrayDeque<>();
deque.addFirst(10);
deque.addLast(20);
System.out.println(deque);
// Output:
// [10, 20]
In this example, we created a Deque and added an integer to the beginning (10) and end (20) of the Deque. When we print the Deque, we see the elements in the order they were added.
These are just a couple of the more advanced ways you can use Java Queue. As you continue your journey, you’ll encounter even more use cases and variations.
Expert-Level Java: Beyond Queue
While Java Queue is a versatile and commonly used data structure, there are situations where other data structures may be more suitable. Two such alternatives are Stack and List. Let’s explore these, along with examples and considerations for when to use each.
Stack in Java
A Stack is a data structure that follows the LIFO (Last In, First Out) principle, which is the opposite of Queue’s FIFO. This makes Stack suitable for scenarios where you want to access elements in the reverse order of their arrival. Here’s a basic example of a Stack in Java:
Stack<Integer> stack = new Stack<>();
stack.push(10);
stack.push(20);
System.out.println(stack);
// Output:
// [10, 20]
int poppedElement = stack.pop();
System.out.println("Popped element: " + poppedElement);
System.out.println(stack);
// Output:
// Popped element: 20
// [10]
In this example, we added two integers to the Stack and then removed the last one added (20), demonstrating the LIFO principle.
List in Java
A List in Java is a collection that holds an ordered sequence of elements. Unlike Queue, a List allows for random access to its elements, meaning you can access any element directly using its index. Here’s an example:
List<Integer> list = new ArrayList<>();
list.add(10);
list.add(20);
System.out.println(list);
// Output:
// [10, 20]
int firstElement = list.get(0);
System.out.println("First element: " + firstElement);
// Output:
// First element: 10
In this example, we added two integers to the List and then accessed the first one using its index (0).
When deciding between Queue, Stack, and List, consider the needs of your specific use case. Queue is perfect for FIFO operations, Stack for LIFO, and List for random access.
Troubleshooting Java Queue: Common Issues and Solutions
Working with Java Queue, like any other data structure, can sometimes throw up challenges. One common issue is encountering a NoSuchElementException. Let’s discuss this problem and how to solve it.
NoSuchElementException in Java Queue
This exception is thrown when you try to remove an element from an empty Queue. Here’s an example that will cause this exception:
Queue<Integer> queue = new LinkedList<>();
queue.remove();
// Output:
// Exception in thread "main" java.util.NoSuchElementException
In this example, we’ve created an empty Queue and then tried to remove an element from it. Because the Queue is empty, Java throws a NoSuchElementException.
How to Solve NoSuchElementException
To avoid this exception, always check if the Queue is empty before trying to remove an element. You can do this using the isEmpty()
method. Here’s how:
Queue<Integer> queue = new LinkedList<>();
if(!queue.isEmpty()) {
queue.remove();
} else {
System.out.println("Can't remove elements from an empty Queue!");
}
// Output:
// Can't remove elements from an empty Queue!
In this revised example, we first check if the Queue is empty. If it’s not, we remove an element. If it is, we print a message. As a result, we avoid the NoSuchElementException.
Remember, understanding the common issues and how to solve them is a crucial part of mastering Java Queue. Always ensure that your Queue operations consider these potential pitfalls.
Understanding the Java Queue Interface and FIFO Principle
To truly master Java Queue, it’s crucial to understand the underlying fundamentals: the Queue interface in Java and the principle of FIFO (First In, First Out).
The Queue Interface in Java
In Java, Queue is an interface that belongs to the Java Collections Framework. It extends the Collection interface and declares behavior specific to a queue data structure. The Queue interface includes methods for inserting, removing, and inspecting elements in a queue.
Here’s a brief overview of some key methods:
add(E e)
: Inserts the specified element into the queue. If the task is successful, add(E e) returns true, if not it throws an exception.offer(E e)
: Inserts the specified element into the queue. Returns true if the element was added to the queue, false otherwise.remove()
: Retrieves and removes the head of the queue. Throws an exception if the queue is empty.poll()
: Retrieves and removes the head of the queue. Returns null if the queue is empty.element()
: Retrieves, but does not remove, the head of the queue. Throws an exception if the queue is empty.peek()
: Retrieves, but does not remove, the head of the queue. Returns null if the queue is empty.
Queue<Integer> queue = new LinkedList<>();
queue.add(10);
System.out.println(queue.element());
// Output:
// 10
In this example, we created a Queue, added an integer, and then used the element()
method to retrieve the head of the queue. The element()
method does not remove the element, so the queue remains unchanged after the call.
FIFO: The Fundamental Principle of Queue
FIFO stands for ‘First In, First Out’, which is the fundamental principle of a queue. It means that the first element that gets added to the queue is the first one to be removed. This principle is what differentiates a queue from other data structures like stack or list.
In a real-world scenario, think of a queue as a line of people waiting for a bus. The person who arrives first gets on the bus first. The same applies to Java Queue – the first element added (or ‘arrives’) is the first one to be removed (or ‘gets on the bus’).
Understanding the Queue interface and the FIFO principle is a big step towards mastering Java Queue. With this foundation, you can better understand how to use Java Queue and its various methods in your code.
Java Queue in Real-World Applications: Multi-threading and Networking
Java Queue is not just a theoretical concept, it’s a practical tool used in many real-world applications. Two common use cases are multi-threading and networking applications. In addition, we’ll also suggest some related topics for further exploration.
Java Queue in Multi-threading
In multi-threading applications, Java Queue is often used to manage tasks. Each task is represented as an element in the Queue, and threads take tasks from the Queue to process. This ensures tasks are processed in the order they’re received and prevents any single thread from becoming a bottleneck.
Here’s a simplified example of how this might look in code:
Queue<Task> taskQueue = new LinkedList<>();
// Add tasks to the queue
for(int i = 0; i < 10; i++) {
taskQueue.add(new Task(i));
}
// Process tasks in separate threads
while(!taskQueue.isEmpty()) {
new Thread(new TaskRunner(taskQueue.remove())).start();
}
// Output:
// Tasks are processed in the order they were added to the queue
In this example, we create a Queue of tasks and add ten tasks to it. We then start a new thread for each task, removing the task from the Queue as we go. This ensures the tasks are processed in the order they were added to the Queue.
Java Queue in Networking Applications
In networking applications, Java Queue can be used to manage packets of data. Packets arrive at a server and are placed in a Queue. The server then processes the packets in the order they arrived.
While this is a more complex use case that goes beyond the scope of this guide, it’s a good example of how versatile Java Queue can be.
Related Topics: Java Deque and Java PriorityQueue
If you’re interested in going beyond the basics of Java Queue, two related topics worth exploring are Java Deque and Java PriorityQueue. We’ve touched on these briefly in this guide, but they each offer additional functionality and use cases that can be useful in more complex applications.
Further Resources for Mastering Java Queue
Ready to dive deeper into Java Queue? Here are some external resources that offer further reading and examples:
- Mastering Data Structures in Java – Understand the principles of object-oriented design and inheritance in Java data structures.
Java PriorityQueue Overview – Explore Priority Queue usage and examples in Java.
Exploring Java TreeMap – Master using TreeMap for scenarios requiring sorted mappings and range operations in Java.
Java Queue Interface – Gain a comprehensive understanding of the Java Queue interface.
Java Queue Tutorial – Learn about Java Queue and its methods.
Java Collections – PriorityQueue – In-depth exploration of Java PriorityQueue, expanding on advanced Queue usage.
These resources offer a wealth of information and practical examples that can help you further your understanding and mastery of Java Queue.
Wrapping Up
In this comprehensive guide, we’ve delved into the depths of Java Queue, a core component of the Java Collections Framework. From the basics to more advanced uses, we’ve explored how to create, manipulate, and troubleshoot Java Queues.
We began with the basics, learning how to create a Queue in Java, how to add elements, and how to remove elements. We then ventured into more advanced territory, discussing more complex uses of Java Queue, such as using PriorityQueue or Deque. We also explored alternative data structures, such as Stack or List, that can be used instead of a Queue.
Along the journey, we tackled common challenges you might face when using Java Queue, such as NoSuchElementException, and provided you with solutions to overcome these challenges. We also took a step back to understand the Queue interface in Java and its methods, as well as the principle of FIFO on which it operates.
Here’s a quick comparison of the data structures we’ve discussed:
Data Structure | Use Case | Principle |
---|---|---|
Queue | When elements need to be processed in the order they were added | FIFO |
Stack | When elements need to be processed in the reverse order of their arrival | LIFO |
List | When elements need to be accessed randomly | – |
Whether you’re just starting out with Java Queue or you’re looking to level up your data manipulation skills, we hope this guide has given you a deeper understanding of Java Queue and its capabilities.
Mastering Java Queue is a valuable skill for any Java developer. It’s a versatile tool that can be used in a wide range of applications, from data structure management to handling processes in operating systems. With this guide, you’re well on your way to becoming a Java Queue expert. Happy coding!